Building a RAG Chatbot Using LangChain: Key Steps and Considerations
Creating a Retrieval-Augmented Generation (RAG) chatbot using LangChain can significantly enhance user interaction and information retrieval. The primary goal of such a chatbot is to seamlessly merge large datasets with generative language models, allowing it to fetch relevant information quickly while generating coherent responses. Here’s how to go about building one effectively.
Understanding the Fundamentals of RAG Chatbots
Before diving into the technicalities, it's essential to grasp the concept of a RAG chatbot. RAG chatbots leverage the capabilities of a knowledge base while also generating responses that feel natural and contextually relevant. To achieve this, your chatbot will use the LangChain framework, which simplifies integrating various language models and data storage solutions.
Key Components for Building Your RAG Chatbot
To create a functional RAG chatbot, you'll need to focus on the following components:
- Data Sources: Collect a diverse dataset that your chatbot can refer to when answering queries.
- Language Model: Select a language model, like OpenAI’s GPT, that can generate fluent and relevant text.
- Contextual Embeddings: Use contextual embeddings to encode your data and effectively match the user's intent.
- Retrieval Mechanism: Implement a retrieval system that can fetch relevant data from the dataset based on user queries.
Step-by-Step Process
Follow these key steps to build your RAG chatbot using LangChain:
Step 1: Set Up Your Environment
Start by setting up your development environment, ensuring that you have Python and the necessary libraries installed. Use the following command to install LangChain if you haven’t already:
pip install langchain
Step 2: Prepare Your Dataset
Your dataset is crucial for retrieval accuracy. Make sure it's well-structured. You can organize your data in formats such as CSV or JSON. Here’s a simple structure you might consider:
Field | Description |
---|---|
Question | The query a user is likely to ask. |
Answer | Concise and accurate response to the query. |
Keywords | Relevant keywords for effective retrieval. |
Step 3: Implement the Retrieval Mechanism
Integrate a retrieval system that can efficiently fetch data from your dataset. LangChain provides various tools like vector stores to manage and search embeddings.
from langchain.embeddings import OpenAIEmbeddings
from langchain.document_retrievers import VectorStoreRetriever
embeddings = OpenAIEmbeddings()
retriever = VectorStoreRetriever(embedding_function=embeddings)
Step 4: Generating Responses
With the retrieval system in place, it's time to generate answers. You will need to pass the retrieved data into your language model for further processing. Here’s a basic implementation:
from langchain.chat_models import ChatOpenAI
chatbot = ChatOpenAI(model="gpt-3.5-turbo")
response = chatbot.generate(retrieved_data)
Step 5: Testing and Iteration
Once your chatbot is functional, it’s critical to test it rigorously. Conduct user testing and gather feedback. This iterative process allows you to fine-tune both the retrieval mechanism and response generation for better user satisfaction. Ensure error handling is incorporated into your code to manage unexpected inputs gracefully.
Best Practices for Enhancing Your RAG Chatbot
Consider implementing the following best practices to optimize your RAG chatbot:
- Regularly Update Your Dataset: Information changes quickly; ensure your dataset reflects the latest data.
- Monitor Performance: Analyze user interactions to identify trends and areas for improvement.
- Utilize User Feedback: Adapting based on user feedback can greatly improve the chatbot's effectiveness.
Building a RAG chatbot using LangChain empowers you to create a sophisticated tool that can greatly improve user experience. Keep iterating on your design while prioritizing user needs for optimal results.
The Role of Retrieval-Augmented Generation in Modern Chatbots
In recent years, chatbots have transformed the way businesses interact with their customers. One of the key advancements propelling this evolution is the concept of Retrieval-Augmented Generation (RAG). RAG chatbots not only generate responses based on the data they have been trained on, but they also retrieve relevant information from external sources, making them more effective and relevant in real-time conversations.
Understanding the mechanics of RAG is crucial for developers and organizations looking to implement sophisticated chatbots. At its core, RAG combines the strengths of traditional retrieval-based models and generative models. This hybrid approach enables chatbots to provide precise information while maintaining a natural conversational tone.
One of the main advantages of RAG chatbots is their ability to access and utilize large repositories of data. Unlike static chatbots that rely solely on pre-defined responses, RAG chatbots can fetch information from databases, APIs, or even the internet. This capability significantly enhances their relevance and applicability in various contexts.
For example, let's consider a customer service chatbot for an e-commerce platform. If a user inquires about the status of their order, the RAG approach allows the chatbot to not only generate a response based on its training data but also pull real-time information from the order management system. This results in a prompt and accurate reply that boosts customer satisfaction.
Moreover, the flexibility of RAG enables chatbots to function across different industries effectively. Whether it's healthcare, finance, or education, these chatbots adapt their responses based on the retrieved data while ensuring that the generated content aligns with the company’s voice and tone. This adaptability is particularly beneficial in highly regulated industries where accuracy is paramount.
Implementing a RAG chatbot involves several crucial steps:
- Data Collection: Gather domain-specific data that the chatbot will use to enhance its responses.
- Model Training: Utilize natural language processing (NLP) techniques to train the chatbot on both predefined responses and retrieval mechanisms.
- Integrating Retrieval Systems: Establish connections to external databases, APIs, or content management systems from which the chatbot can pull the latest information.
- Testing: Rigorously test the chatbot in various scenarios to ensure that it retrieves and generates information seamlessly.
- Monitoring and Feedback: Continuously monitor interactions and gather feedback to refine both the generative and retrieval portions of the chatbot.
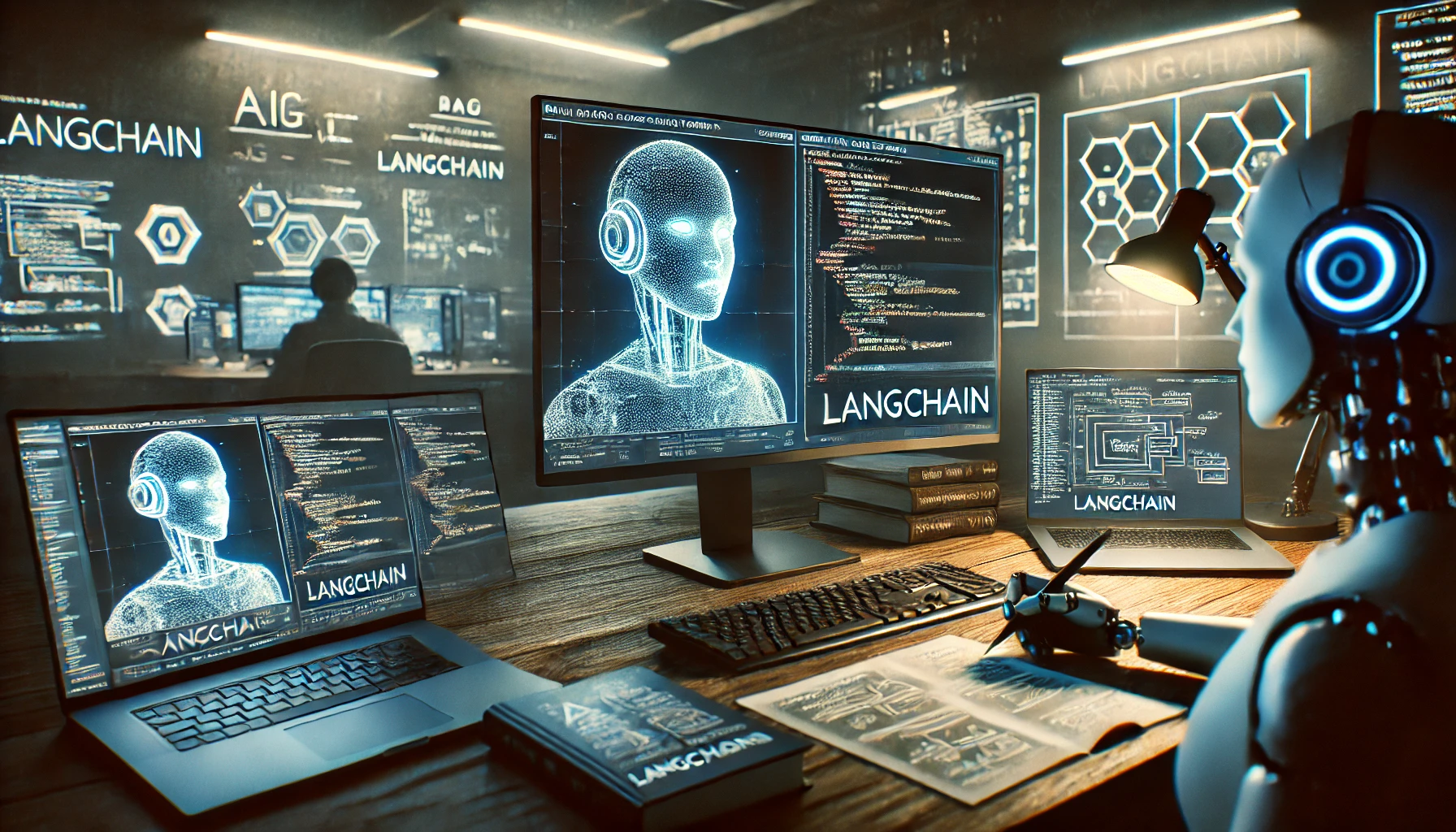
Nonetheless, while RAG presents exciting opportunities, it is not without challenges. One critical aspect is ensuring the quality and reliability of the retrieved information. Chatbots must discern between trustworthy sources and unreliable or misleading content. Employing robust filtering and validation mechanisms is essential for maintaining the integrity of the information provided.
Additionally, fostering a seamless user experience is vital. If a chatbot retrieves information that is difficult to understand or irrelevant, it can frustrate users. Therefore, designing intuitive conversations that guide users toward their desired information while making use of RAG is crucial for overall engagement.
Another challenge lies in computational efficiency. Implementing retrieval mechanisms can require significant resources, especially as the volume of data increases. Developers must balance the chatbot's responsiveness with the necessary data retrieval processes to maintain real-time interaction without lagging.
The future of chatbots guided by RAG looks promising. With advancements in artificial intelligence and machine learning, developers are continuously seeking ways to enhance the functionality of these conversational agents. Innovations like better indexing of retrieved data and improved NLP systems will likely push the boundaries of what RAG chatbots can achieve.
The role of Retrieval-Augmented Generation in modern chatbots is pivotal. By blending retrieval-based and generative methods, organizations can create chatbots that are not only interactive and engaging but also informative and precise. As this technology continues to evolve, the potential applications are boundless, paving the way for smarter, more effective customer interactions in various sectors.
Comparisons Between LangChain and Other Chatbot Frameworks
When it comes to building chatbots, several frameworks compete for attention, each with unique features, advantages, and challenges. Among these, LangChain has emerged as a popular choice, thanks to its flexibility and capabilities. Here, we’ll dissect how LangChain compares to other established chatbot frameworks like Rasa, Dialogflow, and Microsoft Bot Framework.
Flexibility and Customization
LangChain stands out for its modular architecture, allowing developers to customize their chatbots according to specific needs. Unlike Rasa, which offers a more defined pipeline approach, LangChain provides developers with the freedom to implement their own logic and integrate various components easily. This adaptability is crucial for businesses requiring tailored solutions.
On the other hand, Dialogflow, owned by Google, incorporates NLP (Natural Language Processing) directly into its service, making it user-friendly but somewhat limited for highly specialized projects. Developers may find themselves constrained by predefined intents and limited customization options. In contrast, LangChain allows for a more hands-on approach, enabling enhanced creativity in building complex bot functionalities.
Natural Language Processing Capabilities
Leveraging advanced NLP is a significant aspect of chatbot development. LangChain integrates various NLP libraries, supporting tasks ranging from entity recognition to sentiment analysis. Its open architecture allows seamless incorporation of third-party NLP models, giving developers the flexibility to choose the most suitable tools for their projects.
Conversely, frameworks like Dialogflow come with built-in NLP capabilities, making them easier for beginners. However, this convenience can turn into a disadvantage for those who want deeper customization. With LangChain, you can elevate user experiences by tailoring language understanding and generation more finely.
Deployment and Scalability
Deployment options are critical for businesses planning to scale their chatbot solutions. LangChain excels in offering a diverse set of deployment options, whether on-premises, cloud-based, or continuous integration pipelines. This flexibility facilitates easier scaling as the user base grows.
Compare this to the Microsoft Bot Framework, which requires integration within the Azure ecosystem for optimal performance. While Azure offers powerful cloud solutions, businesses not using Microsoft products may find integration challenging. LangChain’s versatile deployment framework arises as a significant plus, particularly for organizations with varied tech stacks.
Community and Support
Community support is vital for any framework. LangChain has gradually built a robust community, providing resources like forums, documentation, and plugin development that facilitate smoother debugging and enhancement processes. For instance, you can find rich community-contributed modules that extend functionality significantly.
Rasa also boasts an active community with countless tutorials and integrations available. However, some users report that support can be fragmented, given the decentralized nature of the community contributions. This discrepancy can lead to challenges when seeking help.
Integration with Other Tools
Integrating various APIs and services can be a cumbersome process for chatbot development. LangChain shines here, offering straightforward integrations with popular platforms like Slack, Facebook Messenger, and custom APIs. This level of integration can significantly speed up development times and expand a chatbot's capabilities.
Dialogflow natively supports multiple messaging platforms, but customization options remain limited, often necessitating workarounds for unique integrations. LangChain, by contrast, allows for more versatile API integrations, enabling developers to tailor their solutions from top to bottom.
Performance Metrics
When measuring performance and user engagement, the choice of framework plays a crucial role. LangChain allows for considerable optimization and fine-tuning of performance metrics. Developers can monitor conversations closely and tweak the system per user feedback, enhancing long-term user satisfaction.
Below is a comparative overview showcasing key differences among LangChain, Rasa, Dialogflow, and Microsoft Bot Framework:
Feature | LangChain | Rasa | Dialogflow | Microsoft Bot Framework |
---|---|---|---|---|
Customization | High | Medium | Low | Medium |
NLP Capabilities | Advanced | Advanced | Built-in | Advanced |
Deployment Options | Versatile | Cloud & On-Prem | Cloud | Azure-focused |
Community Support | Growing | Established | Growing | Established |
Integration with Other Tools | High | Medium | High | Medium |
Learning Curve | Moderate | Steep | Easy | Moderate |
Choosing the right framework depends on your specific requirements, skill levels, and project goals. Each has its strengths and weaknesses, yet LangChain stands out with its customization options and flexibility, making it an excellent choice for developers looking to create sophisticated, tailored chatbot solutions. Whether you're beginning a new project or needing to refine an existing chatbot, understanding these comparisons can guide you to decision-making that best serves your needs.
Enhancing User Experience with Customizable Chatbot Features
As organizations increasingly recognize the value of chatbots in improving customer interaction and satisfaction, the demand for customizable features has grown exponentially. Customizable chatbot features allow businesses to tailor their bots to the specific needs and preferences of their users. This approach not only enhances the user experience but also drives engagement and retention. Below, we delve into various customizable features that can significantly improve the user experience.
User Interface Personalization
One of the most impactful customizable features is the user interface (UI). A personalized UI can cater to different user demographics and preferences, creating a more engaging experience. By allowing users to choose themes, colors, and layout styles, businesses enable customers to interact in a manner that feels natural to them.
- Theme Selection: Users can select dark mode or light mode, aligning with their preferences.
- Font Size Adjustment: Enabling users to modify font sizes enhances readability.
- Language Options: Offering multilingual support improves accessibility for non-native speakers.
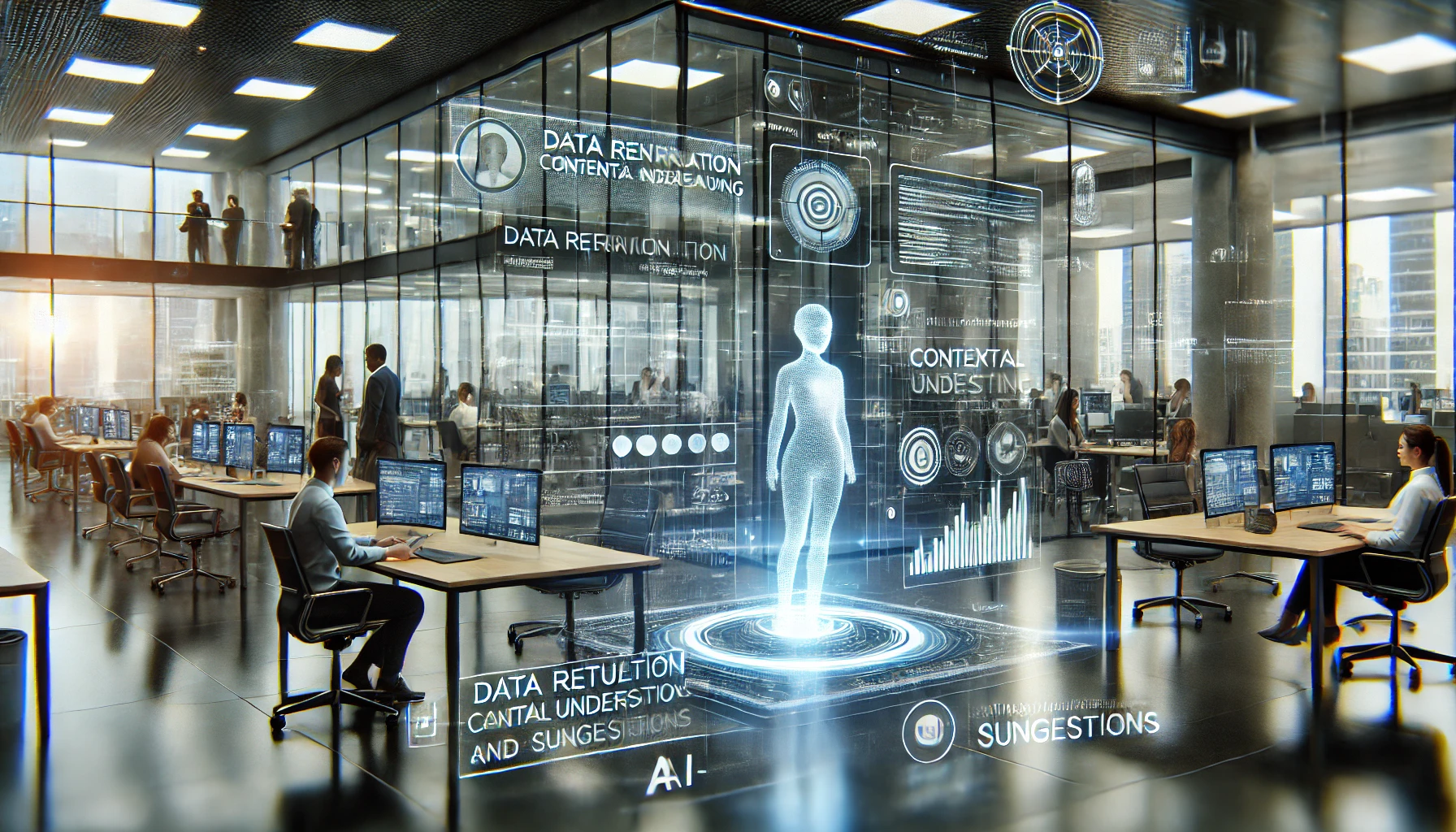
Conversation Flow Customization
Customizing the conversation flow is essential for enhancing user engagement. Businesses can curate paths that guide users through interactions based on their preferences or previous behaviors. This approach helps in creating a more relevant experience.
- Dynamic Content: Chatbots can serve different content based on user profiles and previous interactions.
- Branching Scenarios: Users can be directed down different conversation paths based on their responses, making conversations feel more organic.
- Contextual Awareness: memory features allows chatbots to remember past interactions, tailoring future conversations based on collected data.
Integration with Third-Party Services
Integrating with third-party tools can vastly enhance a chatbot’s capabilities. Users benefit when a chatbot can seamlessly interact with other services like calendars, e-commerce platforms, and CRM systems.
- Appointment Scheduling: Integrating with calendar software allows users to set appointments directly through the chatbot.
- E-commerce Functionality: Allow customers to browse products and even complete purchases without leaving the chat interface.
- Data Syncing: Keeping user data synchronized across platforms creates a cohesive experience.
Feedback and User Input
Enhancing user experience through feedback mechanisms is crucial. Chatbots should be able to prompt users for their opinions and make adjustments based on their responses.
- Satisfaction Surveys: After interactions, prompting users for feedback helps identify areas for improvement.
- Adaptive Learning: feedback allows chatbots to evolve and enhance their responses over time.
- User Preferences: Giving users the ability to modify their interaction preferences shapes higher satisfaction rates.
AI-Driven Personalization
Leveraging artificial intelligence can significantly enhance the personalization of chatbot interactions. By analyzing user data, AI can help create a more tailored experience.
- Recommendation Systems: Based on user behavior and preferences, chatbots can suggest products or information that are most relevant.
- Sentiment Analysis: Understanding user emotions can facilitate appropriate responses, enhancing overall satisfaction.
- Proactive Engagement: Chatbots can reach out to users with special offers or information at crucial touchpoints, showing a proactive approach.
Accessibility Features
In today’s digital age, accessibility is more important than ever. Customizable accessibility features can make a chatbot usable for individuals with disabilities, creating an inclusive experience.
- Voice Input/Output: Allowing users to interact using their voice can significantly help those with limited typing abilities.
- Screen Reader Compatibility: Ensuring that the chatbot interface works with screen readers aids visually impaired users.
- Keyboard Navigation: Providing shortcuts and keyboard commands can assist users who cannot use a mouse.
Customizable features into chatbots not only improves user experience but can also lead to increased loyalty and conversion rates. As businesses continue to invest in chatbot technology, focusing on personalization, integration, and accessibility will be vital in creating effective and engaging customer interactions.
Future Trends in Chatbot Development and AI Integration
The landscape of chatbot development and AI integration is evolving rapidly, presenting exciting opportunities for businesses and users alike. As advancements in technology continue to grow, so do the capabilities and functionalities of chatbots. Understanding the future trends in chatbot development can give organizations a competitive edge and improve customer experiences.
Enhanced Natural Language Processing
Natural Language Processing (NLP) has significantly progressed, thanks to deep learning advancements. This shift enables chatbots to understand context better, grasping nuances in human language. With improvements in sentiment analysis, chatbots can recognize emotions and respond more empathetically. This evolution leads to more meaningful interactions and a better user experience.
Voice-Activated Interfaces
Voice recognition technology is gaining traction. Users now expect not just text-based interactions but also the ability to engage with chatbots through voice. By integrating voice-activated interfaces, businesses can cater to a broader audience. Individuals on the go or those who prefer speaking over typing can benefit greatly from this technology. As voice technology continues to improve, the expectation for chatbots to provide seamless voice interactions will only increase.
Personalized User Experiences
Artificial Intelligence drives the ability of chatbots to deliver personalized experiences. Leveraging user data, chatbots can tailor responses based on past interactions, preferences, and behaviors. By utilizing machine learning algorithms, they can learn from each conversation, improving accuracy and relevance over time. Users are more likely to engage with chatbots that provide tailored recommendations, making personalization a key focus area in the coming years.
Multimodal Interactions
As chatbots evolve, the integration of multimodal interactions is becoming essential. Users increasingly engage with content across different platforms, and chatbots must accommodate these preferences. The ability to switch between text, video, or voice while interacting with a bot will enhance user satisfaction. By supporting multiple forms of input and output, chatbots can provide richer engagement experiences.
Augmented Intelligence
The concept of augmented intelligence, where AI supports human decision-making rather than replacing it, is gaining prominence in chatbot development. By leveraging AI capabilities, chatbots can aid users in making informed decisions. For instance, in sectors like finance or healthcare, chatbots can provide data-driven insights, helping professionals make better choices based on real-time information.
Integration with IoT Devices
The Internet of Things (IoT) is becoming a significant player in the chatbot space. With various devices interconnected, chatbots can serve as conduits between users and their smart devices. Whether it’s controlling home automation systems or providing updates from wearables, chatbots integrated with IoT can help streamline everyday tasks. This trend will lead to more intuitive interfaces, allowing users to manage their devices seamlessly through conversational interactions.
Robust Security Measures
With the rise of AI and chatbot technologies, security concerns become paramount. Future developments must prioritize robust security protocols to protect user data and privacy. As regulations like GDPR and CCPA set new standards, chatbots will need to ensure compliance while providing transparent data usage policies. Users will be more inclined to interact with bots that guarantee their information is secure.
Increased Use of Low-Code Platforms
Low-code and no-code platforms are making chatbot development more accessible. These solutions allow non-developers to create and customize chatbots without extensive coding knowledge. As more organizations adopt these platforms, the number of innovative bots entering the market will rise, supporting various industries. This democratization of chatbot creation can spark creativity and lead to more diverse applications.
Real-Time Analytics and Reporting
In the future, chatbots will increasingly rely on real-time analytics to enhance performance and user satisfaction. Businesses can leverage data gathered from interactions to optimize chatbot responses. By monitoring trends and user behaviors, organizations can make informed adjustments to improve their chatbots’ effectiveness. This shift towards data-driven insights will be crucial for staying relevant in a competitive market.
Challenges Ahead
Despite these promising trends, several challenges remain. Developing sophisticated chatbots requires continuous investment in both technology and human resources. Moreover, ensuring that bots can handle complex queries without human intervention presents a significant hurdle. Organizations must balance automation with the need for human oversight, striving for a harmonious interaction between bots and customers.
Embracing these future trends will empower businesses to develop innovative chatbot solutions that resonate with users. By focusing on enhanced user experiences and integrating advanced technologies, chatbots will continue to evolve, providing valuable services across various sectors. The future of chatbot development promises to transform the way we interact with machines, leading to more intuitive, efficient, and personalized customer experiences.
Key Takeaway:
Building a Retrieval-Augmented Generation (RAG) chatbot using LangChain opens up a realm of possibilities for enhancing user engagement and delivering precise information. This article explored key steps and crucial considerations for developing a RAG chatbot, emphasizing the importance of effectively combining retrieval systems with generative models. Such integration ensures that chatbots not only have the capability to generate human-like responses but also access relevant data quickly, significantly improving their accuracy and context relevance. As users increasingly demand personalized interactions, understanding how to leverage these systems becomes vital.
The article also delved into the unique benefits of LangChain compared to other chatbot frameworks. While various platforms can manage chatbots effectively, LangChain stands out for its robustness in handling complex queries and its adaptability. With features tailored for ease of customization, developers can craft unique experiences suited to their target audiences. This adaptability equips businesses with the flexibility needed to respond to market demands effectively.
Moreover, enhancing user experience is crucial in the competitive landscape of chatbot development. Customizable features in a LangChain-based chatbot enable businesses to tailor interactions according to user preferences. This personalization helps improve overall user satisfaction, fostering loyalty and ongoing engagement. As these techniques evolve, commonplace functionalities, like context retention and dynamic querying, are becoming integral components to the development of modern chatbots.
Looking toward the future, the trends in chatbot development and AI integration showcase a shift towards more sophisticated, intelligent solutions. Innovations in machine learning and natural language processing are expected to drive chatbots to understand context better and learn from user interactions. As businesses recognize the potential of RAG chatbots powered by LangChain, they are likely to invest more in training their systems, leading to even more refined and responsive user experiences.
Leveraging LangChain for building a RAG chatbot not only enhances operational efficiency but also enriches user interaction, setting a solid foundation for future advancements in AI chatbot technology. Embracing these methodologies and keeping an eye on emerging trends will ensure that developers and businesses thrive in this rapidly evolving digital ecosystem.
Conclusion
Creating a Retrieval-Augmented Generation (RAG) chatbot using LangChain represents a significant leap forward in how we interact with conversational AI. The journey of building such a chatbot requires meticulous attention to key steps and considerations that ensure its effectiveness and functionality. The architecture of RAG embraces the dual strengths of retrieving relevant information while generating nuanced responses. This integration allows chatbots to provide users with more precise and contextually aware answers, elevating the overall engagement and satisfaction of users.
A major advantage of employing LangChain lies in its robust capabilities compared to other chatbot frameworks available in the market. Unlike traditional frameworks, LangChain seamlessly connects various components involved in chatbot building, such as language models, data retrieval systems, and user interfaces. The comparative analysis highlights that while many existing platforms offer generic services, LangChain stands out by providing flexibility and easily adaptable modules that suit diverse requirements. By leveraging LangChain, developers can efficiently design chatbots that not only function as simple question-answering systems but also adapt to unique user needs, thereby creating a more personalized experience.
Customizable features significantly enhance user interaction with chatbots. The ability to tweak and refine chatbot behavior—ranging from conversational tone to response styles—empowers developers to align the chatbot with specific target audiences. This kind of personalization is pivotal in fostering a more meaningful dialogue between users and chatbots. When users feel that their needs are acknowledged through tailored features, their engagement levels increase, leading to better retention and satisfaction. Feature customization may also include integrating sentiment analysis that allows the bot to adjust its responses based on user emotions, effectively closing the gap between human-like interactions and automated responses.
Looking toward the future, the evolution of chatbot technology intertwined with artificial intelligence and machine learning promises exciting opportunities. Emerging trends indicate a strong focus on increasing chatbot intelligence through improved natural language understanding. As models grow in sophistication, they will gain the ability to process complex queries and utilize contextual histories to craft remarkably human-like responses. Additionally, advancements in voice recognition technology signal a shift toward more interactive and accessible chat experiences, driving further adoption across demographics.
Furthermore, integrating multi-modal capabilities will likely transform how users interact with chatbots. This means that chatbots not only understand and generate text but also incorporate images, sounds, and even video—creating richer, more engaging user experiences. The rise of such interaction forms reflects an essential push toward making technology more human-centric and immersive. By harnessing these innovations, businesses can elevate their customer service and support strategies through chatbots that resonate more deeply with user expectations.
It's critical to embrace these advancements while keeping ethical considerations at the forefront. As AI chatbots take on a more significant role in various domains including healthcare, education, and customer service, ensuring they operate within responsible frameworks becomes paramount. Issues such as data privacy, algorithmic bias, and transparency about AI capabilities need careful attention. Developers must build safeguards into their systems, protecting user privacy while ensuring the system functions responsibly.
In building a RAG chatbot with LangChain, developers must not only focus on technical execution but also prioritize creating an ethical, user-friendly environment. The emphasis should shift from merely deploying sophisticated technology to fostering a partnership that enhances human abilities. The ultimate goal is to bridge the gap between automated responses and genuine human-like interactions, creating chatbots that don't just respond but engage, inform, and assist in meaningful ways.
As you embark on this journey of building a RAG chatbot with LangChain, consider the evolving landscape of chatbot development. The technological foundation you choose today will shape the nature of conversations tomorrow. By prioritizing user experience, personalized features, and responsible AI practices, you will be well-positioned to create a chatbot that stands out in a competitive field and is poised for the future.