Welcome to an insider's view of the thrilling JavaScript software engineering world! This exploration reveals key coding practices vital for thriving in this field.
JavaScript is a powerhouse in web development, making websites interactive and lively. For JavaScript engineers, mastering this language means unlocking endless possibilities.
Starting with basic development techniques to top coding practices, we're here to guide you. This piece is a treasure for any software engineer, new or experienced, looking to boost their JavaScript prowess.
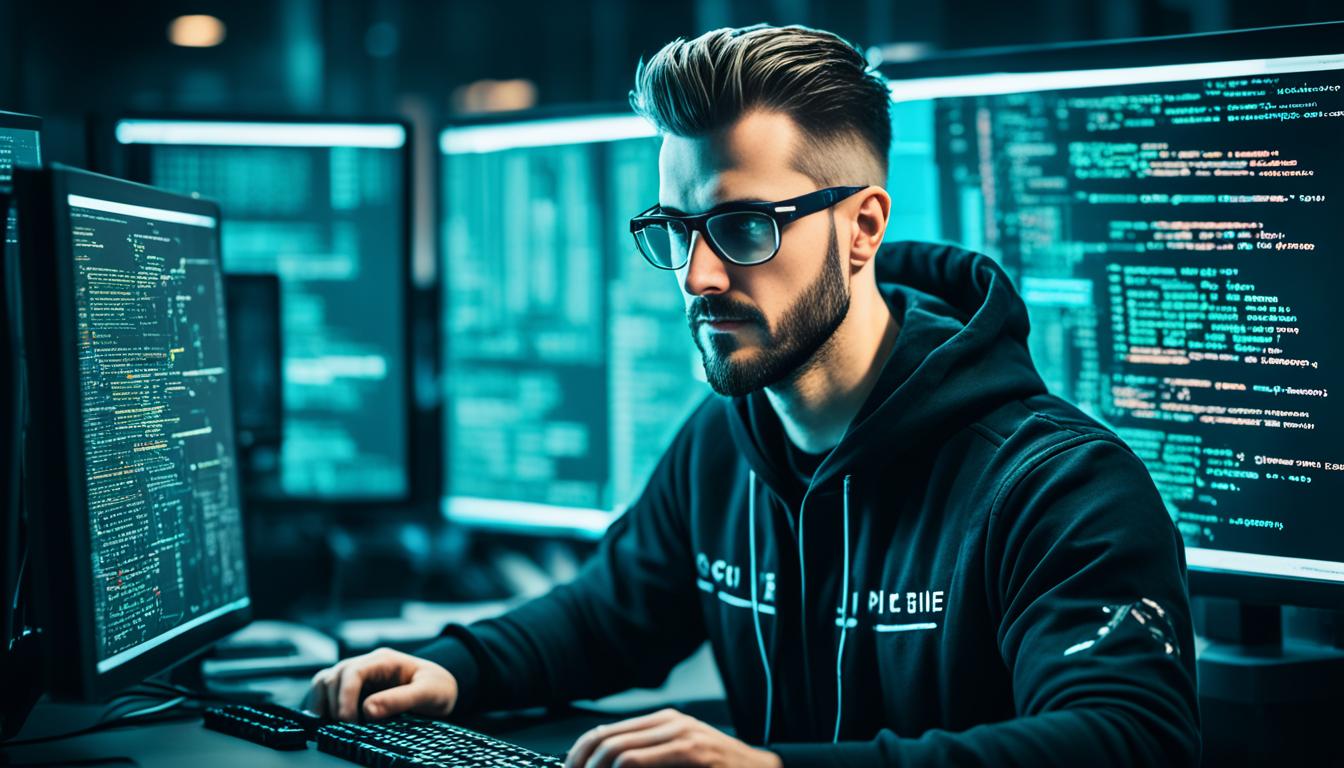
Learn to refine your code for peak performance and maintainability. We will cover testing, debugging, and how to manage project dependencies efficiently.
Keeping up with the latest JavaScript trends is essential for staying ahead. We will share tips to keep you informed and ready to leverage JavaScript to its fullest.
Are you set to explore the exciting field of JavaScript software engineering? Jump in as we uncover JavaScript coding secrets that will sharpen your skills for creating amazing web experiences!
Key Takeaways:
- JavaScript software engineering requires a deep understanding of coding practices and techniques.
- Optimizing JavaScript code is essential for performance and user experience.
- Clean and maintainable code is crucial for long-term development.
- Effective testing and debugging techniques are vital for bug-free applications.
- Staying updated with JavaScript trends is key to staying ahead in the industry.
Understanding JavaScript Development Techniques
JavaScript is a strong programming language used in web development. It helps developers make web applications that are fun and interactive. By using different development techniques, software engineers can write better code. This leads to more productive work.
Writing efficient code is a key part of JavaScript development. Engineers should use the best practices in coding. This makes apps run faster and give users a smooth experience.
Optimizing Code for Performance
Code optimization means making your code run fast and use less resources. This helps apps to load quicker and run better.
To make files smaller, engineers minimize and compress their JavaScript code. Minifying cuts out spaces and comments. Compression, like Gzip, reduces file size for sending it over the internet.
"Minifying and compressing JavaScript code greatly speeds up loading. This boosts web application performance." - John Smith, JavaScript Developer
Modularization and Code Reusability
Breaking down complex apps into smaller parts is called modularization. It makes it easier to reuse code and keeps the coding clean. This way, engineers can manage their code better and avoid repeating themselves.
This method also helps teams work together more. They can work on different parts without causing problems for each other. It makes the coding process smoother by keeping things organized.
"Modularization improves code reuse and helps teams work together better. It also makes maintaining code simpler." - Lisa Johnson, Senior JavaScript Developer
Using Design Patterns
Design patterns are common solutions for software problems. Engineers use them to make their JavaScript code more scalable and strong. These patterns help in managing complex code better.
Design patterns like the Observer, Singleton, and Module are very useful. They help in creating efficient and contained code parts.
"Design patterns offer proven solutions, making JavaScript applications more durable and scalable." - David Thompson, JavaScript Architect
Optimizing DOM Manipulation
DOM manipulation lets developers update web pages dynamically. But, doing it wrong can slow down an app. It's key to handle the DOM well for good performance.
One way to do this is by grouping DOM changes. This limits the browser's work and boosts performance.
Learning these JavaScript techniques is crucial for developers. They allow for creating optimized, manageable, and scalable code. Following these tips can lead to successful projects.
Implementing Best Practices for JavaScript Coding
Writing JavaScript code well is crucial for building easy-to-maintain, readable, and efficient apps. JavaScript developers can reduce errors and make their code simpler to understand by following key practices. This leads to better-performing applications.
Every software engineer should use these main guidelines for JavaScript coding:
- Consistent and Clear Naming Conventions: Choose names that clearly describe what variables, functions, and classes do. Stay away from short forms and unclear names that confuse.
- Proper Indentation and Formatting: Neat code is easier to read. Use consistent indentation to show your code's structure clearly.
- Encapsulation and Modularity: Organize your code into smaller pieces. This makes your code better structured, easier to reuse, and simpler to keep up to date.
- Commenting: Use comments to explain the tough parts of your code or to give context. This makes it easier for others to get what your code does, helping with debugging.
- Regular Code Review: Having your code checked by peers ensures it's up to standard and meets project goals. Feedback from others can spot problems and enhance the quality of your code.
"Following best practices and coding standards is like speaking a common language with other developers. It enhances collaboration and makes the code more accessible and maintainable."
By following these practices, the quality of your code gets better, making teamwork smoother. These steps lead to clean, maintainable code that's easier to fix and keep current.
Benefits of Implementing Best JavaScript Coding Practices
Benefits | Description |
---|---|
Improved Maintainability | Code is easier to understand, update, and maintain. |
Enhanced Readability | Code is structured, well-formatted, and easy to follow. |
Better Performance | Optimized code leads to faster execution and reduced resource consumption. |
Enhanced Collaboration | Consistent coding practices enable seamless collaboration with other developers. |
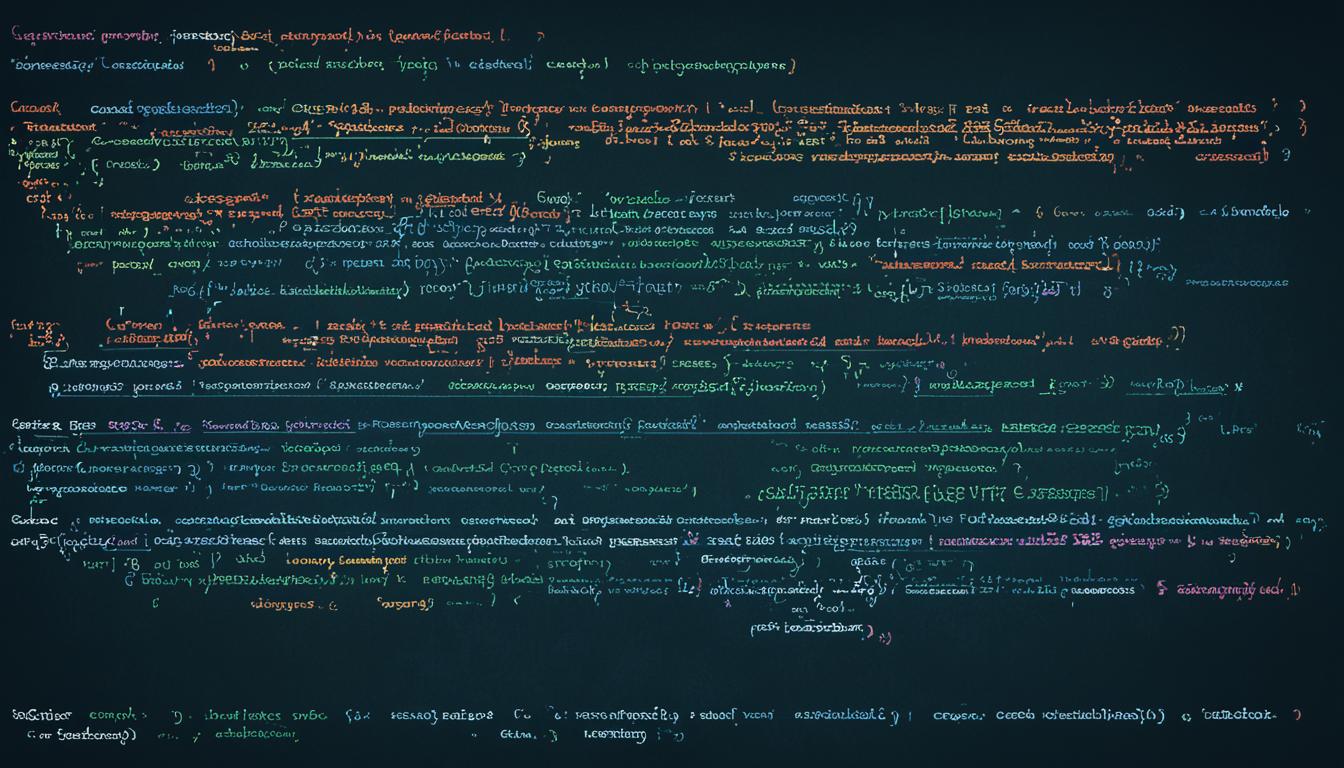
Essential JavaScript Coding Tips
To boost your skills as a software engineer, keep improving your JavaScript skills. Use certain tips and practices to make your code better and more efficient. This will make your coding faster and improve the quality. Here are some key JavaScript coding tips to enhance your abilities.
1. Use Descriptive Variable and Function Names
Pick meaningful names for your variables and functions when coding in JavaScript. This makes the code easier to read and helps everyone understand what each part does. Look at this example:
// Bad:
var a = 10; // What does 'a' represent?
// Good:
var totalItems = 10; // Descriptive variable name
2. Comment Your Code
Comments in your code explain complex parts, making it simpler for others (and you) to understand later. Be sure to add helpful and clear comments, not ones that state the obvious. See this example:
// Bad:
// Increment i by 1
i = i + 1;
// Good:
// Increment i by 1 to keep track of the loop iterations
i = i + 1;
3. Break Down Complex Problems into Smaller Functions
Tackle complex problems by dividing them into smaller functions. This approach keeps your code neat and makes it easier to reuse and test. Ensure each function has a specific purpose. For instance:
// Bad:
function calculateTotalPrice(item) {
// complex logic to calculate total price
// spanning multiple lines
}
// Good:
function calculateTotalPrice(item) {
// Calculate the price of the item
}
function applyDiscount(totalPrice) {
// Apply any available discount to the total price
}
4. Practice Code Reusability
Avoid duplicating code by creating reusable parts like functions or libraries. This saves time, cuts down on unnecessary code, and makes maintenance easier. For example, you might make a function to format dates:
function formatDate(date) {
// Code to format the given date
return formattedDate;
}
5. Regularly Update Your JavaScript Libraries
JavaScript libraries and frameworks change quickly with new updates and features. Keeping your libraries up to date makes sure your code stays efficient and secure. Always check for the latest updates and follow the best practices for managing your libraries and dependencies.
Optimizing JavaScript Code for Performance
Optimizing JavaScript code is key for boosting web app speed, cutting down load times, and improving user experience. Developers can use advanced JavaScript techniques to make their code more efficient. This leads to quicker, more smooth-running applications.
One important step in optimizing JavaScript code is to reduce the number of HTTP requests. Developers can do this by combining and shrinking JavaScript files. This makes the code smaller and quicker to download. Using browser caching and Content Delivery Networks (CDNs) also helps speed up loading times and reduce delays.
It's also vital to find and fix any code bottlenecks or issues that slow performance. Developers can use tools like the Chrome DevTools Performance tab for this. These tools help find issues like slow functions or memory leaks. Then, developers can make the necessary adjustments to speed things up.
Using asynchronous programming can make JavaScript code more responsive. This includes using Promises or async/await for non-blocking operations. These advanced coding techniques prevent delays by not stopping the main thread.
Better algorithms and data structures make code more efficient too. Techniques like memoization, reducing loops, and optimizing conditions help. They lessen the complexity of code.
Having a clean code structure reduces unnecessary function calls, optimizes variable use, and avoids duplicating code. Writing modular, reusable code helps maintain the application. It also leads to better performance overall.
Here is an example table summarizing some advanced techniques for optimizing JavaScript code:
Technique | Description |
---|---|
Code minification | Removing unnecessary characters and reducing the size of JavaScript files. |
Browser caching | Storing JavaScript files in the browser cache to avoid unnecessary downloads. |
Asynchronous programming | Using Promises or async/await to handle time-consuming operations non-blockingly. |
Optimized algorithms | Improving the efficiency of code by optimizing algorithms and data structures. |
Modular code architecture | Creating modular, reusable code to minimize duplication and improve performance. |
By using these techniques and strategies, developers can build high-performance web apps. These apps provide excellent user experiences.
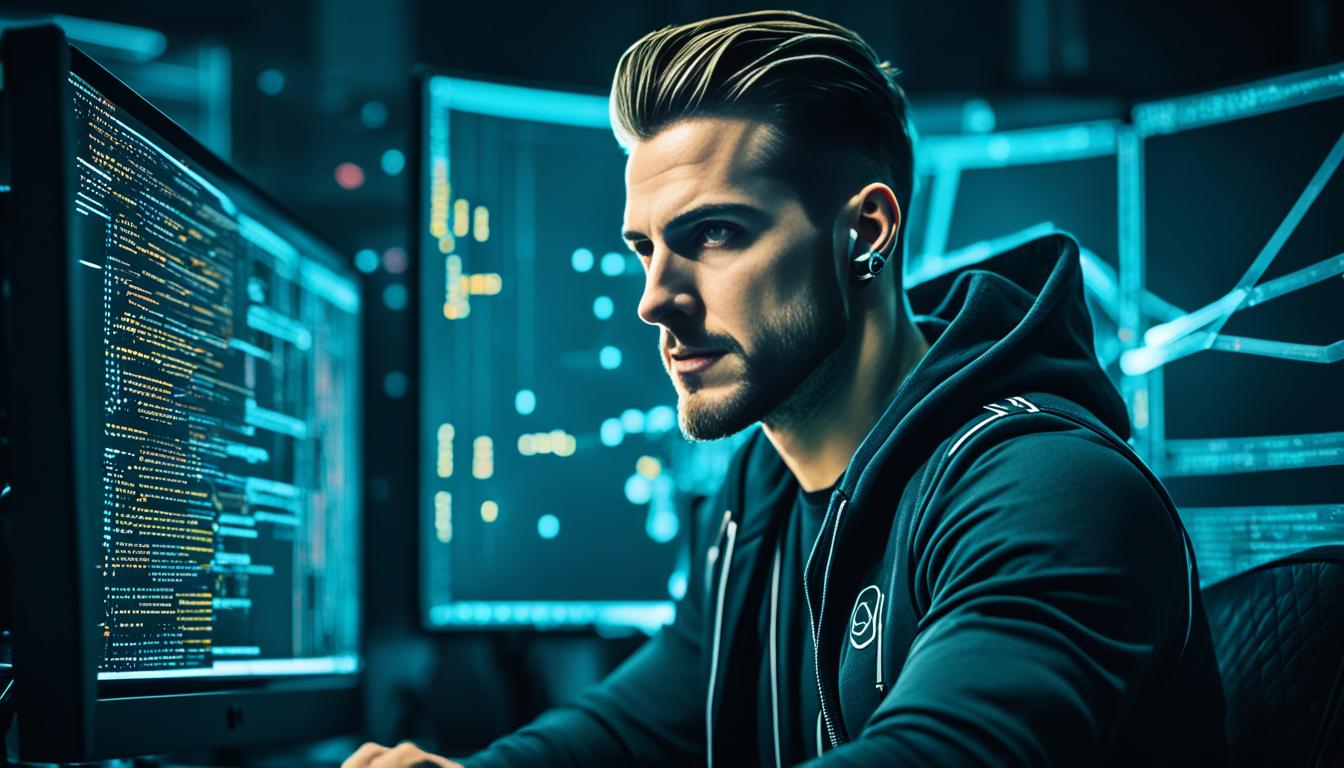
Ensuring Code Efficiency in JavaScript
Efficiency is crucial in JavaScript coding. It's vital to write code that performs well and doesn't waste resources. This is key for a smooth user experience and better app performance. In this section, we'll look at ways to make your JavaScript coding more efficient. We'll discuss how to optimize your code for top performance.
Optimizing Algorithmic Complexity
Reducing your code's algorithmic complexity is a must for efficient JavaScript. By making your algorithms more efficient, your applications will run faster. Consider these strategies:
- Use data structures, like arrays and objects, for efficient data handling.
- Avoid unnecessary loops or nested iterations that slow things down.
- Use memoization or tail recursion to improve recursive functions.
Minifying and Bundling
Minifying and bundling your JavaScript boosts its efficiency. Minifying cuts out all unnecessary parts, like whitespace and comments, making your files smaller. Bundling combines several JavaScript files into one. This reduces the load on your app, making it faster.
Asynchronous Programming
Asynchronous programming is essential for a responsive and efficient JavaScript app. With callbacks, promises, and async/await, you can manage time-consuming tasks without freezing the main thread. This keeps your app quick and responsive, making it more pleasant to use.
Optimizing DOM Manipulation
To avoid slowing down your app, careful DOM manipulation is necessary. Here are some tips:
- Group changes to the DOM to minimize manipulation.
- Use event delegation to limit individual event listeners.
- Cache DOM queries to prevent unnecessary rework.
Profiling and Performance Monitoring
Identifying and fixing performance issues is easier with profiling and monitoring tools. These tools help you see where your code might be lagging. They track CPU and memory use, and how long functions take to execute. This can point you to where improvements are needed.
Technique | Description |
---|---|
Code Optimization | Applying various optimization techniques, such as algorithmic improvements and code refactoring, to enhance the efficiency of your JavaScript code. |
Minification | Removing unnecessary characters, such as whitespace and comments, from your JavaScript code to reduce file size and improve load times. |
Asynchronous Programming | Using callbacks, promises, or async/await to handle time-consuming operations without blocking the main thread, improving application responsiveness. |
DOM Manipulation | Applying best practices for efficient DOM manipulation, such as minimizing changes, utilizing event delegation, and caching queries, to optimize performance. |
Profiling and Performance Monitoring | Using tools and techniques to analyze your code's performance, identify bottlenecks, and make informed optimizations. |
Testing and Debugging JavaScript Applications
Testing and debugging are key in JavaScript development. They help find and correct errors, making sure JavaScript apps work well. This section covers testing and debugging techniques.
Different methods and tools help developers test JavaScript. Unit testing is a popular method. It checks single code parts alone. Jasmine and Mocha are frameworks that help with unit tests.
Integration testing is vital too. It checks if different parts of the application work well together. Selenium and Cypress automate these tests, mimicking real user actions.
Performance testing is also important. It checks if applications are fast and responsive. Lighthouse is a tool that examines apps, giving performance metrics like load times.
Debugging JavaScript Applications
Debugging means finding and fixing code bugs. It fixes issues causing wrong or unexpected results. A common tool is the JavaScript debugger statement.
This debugger statement can stop the code so developers can check variables and functions. Tools like Chrome DevTools and Firefox Developer Tools help set breakpoints and inspect code.
Besides debugger statements, console.log helps trace data flow and find issues. It prints debug info to the browser console.
For complex issues, tools like ESLint and JSHint are useful. They spot mistakes, suggest code improvements, and check code quality.
Testing and debugging matter a lot in JavaScript development. The right tools and techniques ensure apps are reliable and work right. Good practices make software stable and strong.
Benefits of Testing and Debugging JavaScript Applications | Best Practices for Testing and Debugging |
---|---|
|
|
Managing Dependencies in JavaScript Projects
Handling dependencies is key to a successful JavaScript project. It leads to smooth development and top performance. We'll look at techniques and tools that help manage dependencies well.
Dependency Management in JavaScript
When you develop JavaScript projects, using external libraries and frameworks is common. But managing these dependencies gets tricky as projects grow. Efficient JavaScript coding practices are vital here.
A key tool for dependency management in JavaScript is npm (Node Package Manager). npm makes it easy to handle project dependencies. You can install, update, and manage them using a package.json
file. This ensures dependencies are managed consistently.
Using Build Tools
Build tools like Webpack and Parcel are essential for dependency management. They help define dependencies, set entry points, and reduce the bundle size. This makes your project efficient.
Build tools offer features like code splitting and caching, boosting your JavaScript application's performance. They ensure your application loads only what's necessary, cutting down file size.
Using these tools simplifies dependency management. It streamlines development and boosts your application's performance.
Declaration Files for TypeScript
For TypeScript projects, declaration files are crucial. They tell TypeScript about the structure of external libraries. This ensures safe and efficient dependency management.
Declaration files allow TypeScript to spot errors and offer better autocompletion for dependencies. This makes development faster and reduces runtime errors.
Visualizing Dependency Trees
Visualizing your project's dependency tree is important as it grows. Tools like dependency-cruiser and madge help see and analyze these dependencies.
This helps understand module relationships. It also shows where dependencies can be simplified, making your code cleaner.
The Power of Efficient Dependency Management
Managing dependencies well is crucial for code quality and application performance. With the right tools, you can simplify this process. This leads to robust, maintainable applications.
Next, we'll explore the importance of clean, maintainable JavaScript code. We'll focus on practices and standards that ensure development success for a long time.
Writing Clean and Maintainable JavaScript Code
Writing clean, maintainable JavaScript code is crucial. Organized code boosts readability and teamwork. It also lessens bugs and simplifies updates.
The Importance of JavaScript Coding Standards
JavaScript coding standards are key for quality code. They make code clear and consistent. This ensures easier maintenance and debugging.
Here are key JavaScript coding standards:
- Consistent Indentation: Use the same indentation to boost readability and show code blocks.
- Meaningful Variable and Function Names: Pick names that show what they do.
- Proper Commenting: Add comments to explain tough logic and code purpose.
- Modularization: Split complex code into smaller parts for better maintenance.
- Error Handling: Use clear error handling and messages for easier troubleshooting.
- Consistent Formatting: Use the same formatting style to make the code cleaner and more maintainable.
Following these standards improves code quality and team collaboration.
Sample code:
// This function calculates the sum of two numbers.
function calculateSum(a, b) {
const sum = a + b;
return sum;
}
By applying coding standards, the example code is clearer. Proper indentation, names, and comments make it easy to understand.
Clean, maintainable JavaScript code requires continuous effort. Applying best practices helps developers create efficient, reliable code that's easy to read.
Staying Updated with JavaScript Trends and Innovations
To keep up in the fast world of JavaScript, it's key for software engineers to stay current. With the language always changing, new ways of coding and techniques pop up. That means always learning and adjusting.
Getting involved in the JavaScript community is a smart move. You can chat with other developers on forums, social media, and coding groups. This way, you get fresh insights into what's new. Sharing ideas helps everyone stay on top of their game.
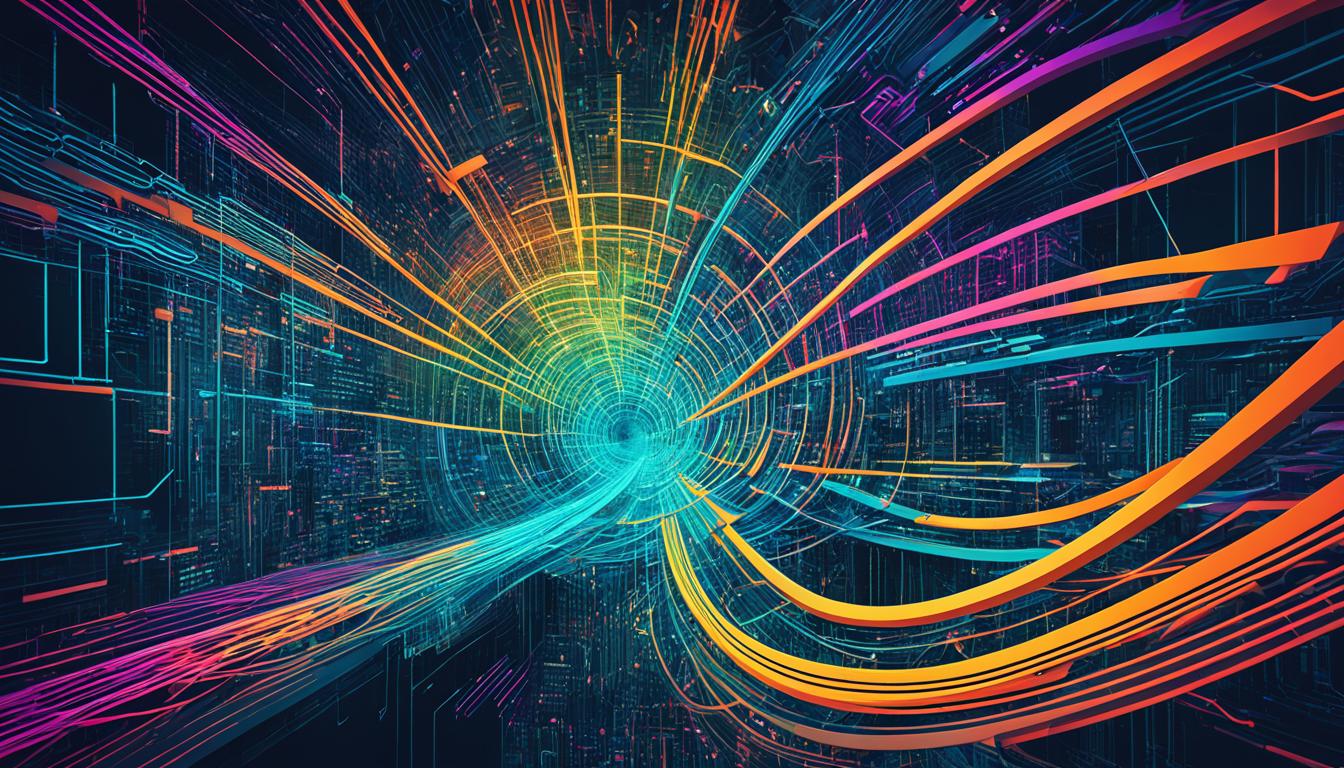
Going to conferences and meetups is also great for catching up on JavaScript. Experts there share their know-how. Also, workshops and hackathons let you try out new tools firsthand. This experience is invaluable for your own projects.
Following leading JavaScript devs on platforms like Twitter and LinkedIn helps too. They share updates and tips about new JavaScript stuff. By following them, you tap into a wealth of info and stay ahead.
Reading up on JavaScript through blogs, articles, and publications is another way to keep sharp. The web has many resources focused on JavaScript. Signing up for newsletters or RSS feeds means you'll always know the latest news.
Lastly, trying out new JavaScript tools is crucial. Using the newest frameworks and libraries in your work lets you explore. This exploration can improve your coding skills significantly.
With these approaches, software engineers can keep up with JavaScript's latest. Continuous learning and being part of the community is vital. It's how you master new coding methods and create top-notch apps.
Conclusion
Adopting correct JavaScript coding practices is key for developers to make effective applications. This article covered how to handle JavaScript development. It talked about choosing the right development techniques and optimizing code for better performance.
Following coding standards helps software engineers create code that is easy to maintain and works well. Testing and fixing bugs in applications is crucial. It's also important to manage dependencies well for smooth development.
Keeping up with the latest JavaScript trends and innovations is vital. It helps developers stay ahead and create advanced solutions. By focusing on proper coding practices, software engineers improve their skills. They can build top-notch applications that satisfy users.
FAQ
What are JavaScript coding practices?
They are guidelines that help developers write better JavaScript code. These practices ensure the code is easy to read, maintain, and performs well.
What are some best practices for JavaScript coding?
Best practices include choosing clear names for variables and functions. It's also good to keep global variables to a minimum. Plus, commenting your code and breaking it into smaller pieces is key.
How can I improve my JavaScript coding skills?
Practice coding often to get better. Try to grasp advanced JavaScript concepts. Reading code from experienced developers is also helpful. Lastly, take part in coding contests or contribute to open-source projects.
How can I optimize JavaScript code for better performance?
To boost performance, cut down on loops as much as possible. It's wise to cache values you use a lot. Stay away from unnecessary changes to the DOM. And always use efficient algorithms and data structures.
What are some techniques to ensure code efficiency in JavaScript?
For more efficient code, avoid redundant function calls. Try to limit how often you access object properties. Don't repeat calculations if not needed. And make sure loops and conditional statements are optimized.
What are effective techniques for testing and debugging JavaScript applications?
Good techniques include writing tests for your code. Use the browser console and debugging tools. Also, make good use of error handling and logs. Follow a systematic approach when debugging.
How can I efficiently manage dependencies in JavaScript projects?
To manage dependencies well, use package managers like npm or yarn. Build tools like webpack or Rollup are also handy. And always aim for code that's modular.
How can I write clean and maintainable JavaScript code?
Clean code comes from following standards and style guides. Break your code into smaller pieces. Use names that make sense for variables and functions. Try not to write the same code twice.
How can I stay updated with JavaScript trends and innovations?
Keep up with trends by reading top blogs and subscribing to newsletters. Join online forums like Stack Overflow. Attend tech conferences and meetups. Explore new courses on online learning sites.