JavaScript has grown into a powerful tool for creating websites. But it can do much more than make sites look good. Now, JavaScript can change the game in artificial intelligence with neural networks. This article explores how neural networks can boost your web-based AI projects. By using JavaScript, creating smart models becomes straightforward, helping you discover key insights.
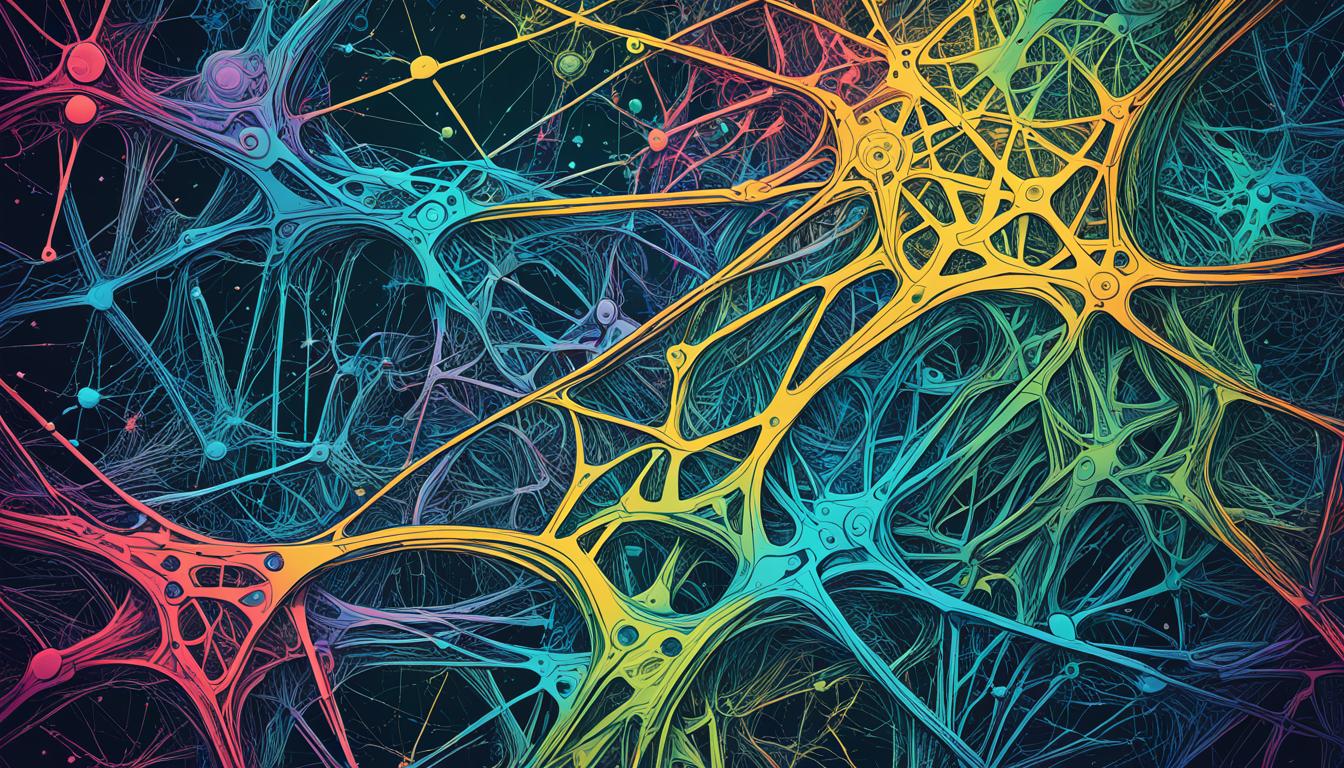
Key Takeaways:
- Neural networks in JavaScript offer a new frontier for web-based AI projects.
- Understanding the fundamentals of neural networks is crucial for implementation.
- JavaScript serves as a robust machine learning framework, enabling diverse applications.
- Implementing neural networks in JavaScript is achievable with proper guidance.
- Explore the wide range of neural network libraries available in JavaScript.
Understanding Neural Networks
Before diving into neural networks in JavaScript, it's key to grasp the basics. These models are like the human brain, using artificial neurons to process info. They recognize patterns in data to make predictions.
In the JavaScript world, neural networks help developers create smart apps. They can recognize images, understand language, and make decisions. This opens up many possibilities for solving complex problems.
Neural Network Algorithms in JavaScript
JavaScript supports various neural network algorithms for use in browsers. This includes backpropagation and feedforward networks, among others. Each algorithm is best for certain tasks.
For JavaScript devs, libraries like TensorFlow.js make neural networks easier to use. These tools offer powerful features for adding intelligence to apps. It simplifies working with complex data and making predictions.
JavaScript Neural Network Examples
Let's look at a basic JavaScript neural network example. We'll train it to identify handwritten digits using the MNIST dataset. This dataset has thousands of labeled images for training.
"Neural networks bring a new level to artificial intelligence, allowing machines to learn from data. With JavaScript, we can build AI apps in the browser. This guide will help you understand neural networks and see examples in action."
- Start with the MNIST dataset, preparing the images for training.
- Plan your neural network's design, detailing the layers and nodes.
- Use the training images to teach the neural network, fine-tuning as needed.
- Test the trained network to see how well it performs.
"Neural networks adapt to various tasks, from recognizing images to understanding language. By trying out JavaScript examples, devs can create powerful AI apps."
Running our JavaScript example lets developers see the neural network learning. It gradually gets better at predicting the correct digits.
Input Image | Predicted Digit |
---|---|
7 | |
3 | |
5 |
This example shows neural networks' accuracy in JavaScript. It correctly identifies the handwritten digits.
We now understand neural networks and JavaScript’s role in AI. With the right tools, devs can tackle complex challenges and build smart applications.
JavaScript as a Machine Learning Framework
JavaScript is more than just web development language. It's a powerful machine learning framework too. It has libraries and frameworks that help developers with machine learning tasks in the browser. We'll look into JavaScript's role as a machine learning framework. Plus, we'll check out resources for intelligent algorithms.
The Potential of JavaScript for Machine Learning
JavaScript's versatility and wide adoption make it great for machine learning. It works natively in web browsers, so no extra setup is needed. It can integrate with web applications easily. Using JavaScript means better performance and privacy through client-side computing.
Developers can use JavaScript for tasks like image and speech recognition, and natural language processing. There's a big need for AI-driven applications. JavaScript offers an easy, accessible way to meet this need with machine learning algorithms.
Libraries and Frameworks for JavaScript Machine Learning
JavaScript provides many libraries and frameworks for machine learning. These tools make development easier and offer ready-to-use algorithms. Here are some popular options:
- TensorFlow.js: Google's TensorFlow.js lets developers train and deploy models in the browser. It's good for deep learning tasks because of its intuitive APIs and performance.
- Brain.js: Brain.js is a simple neural network library. It's easy to create and train models with it. It works in browsers and Node.js, making it flexible for different projects.
- ConvNetJS: ConvNetJS focuses on convolutional neural networks (CNNs). It's efficient for image recognition and vision tasks.
Library/Framework | Description |
---|---|
TensorFlow.js | Google's comprehensive machine learning library for browser-based model training and deployment. |
Brain.js | A minimal neural network library for easy model creation and training in JavaScript. |
ConvNetJS | Focuses on CNNs, perfect for image recognition and computer vision. |
These libraries give a strong start for machine learning models in JavaScript. They have lots of documentation, examples, and a helpful community. This makes starting in machine learning easier without deep programming language knowledge.
"JavaScript's flexibility and extensive library ecosystem have transformed it into a capable machine learning framework, allowing developers to harness the power of AI directly in the browser."
With JavaScript and special libraries, developers can bring AI to their web apps. They can build systems that analyze data, make predictions, and automate decisions. JavaScript's easy access and versatility make it a thrilling platform for innovation in machine learning.
Implementing Neural Networks in JavaScript
We now have a strong base. It's time to dive into creating neural networks in JavaScript. This detailed guide gives you all you need to build a neural network from the ground up.
Let's get started:
Step 1: Understanding the Neural Network Architecture
Before coding, it's crucial to know about neural network architecture. A neural network has layers like the input layer, hidden layers, and an output layer. Each layer has nodes or neurons which pass on information. Knowing this helps you build and run your neural network well.
Step 2: Setting up your Development Environment
To code your neural network in JavaScript, a proper development setup is needed. Make sure you have a good text editor and a JavaScript runtime environment like Node.js. A good setup is key for a smooth coding journey.
Step 3: Building the Neural Network from Scratch
Now, it’s time to start building your neural network. First, decide on your network's structure. Choose the layers, neurons per layer, and activation functions. Then, code the weights and biases. These steps are the building blocks of your neural network.
Here's an example of JavaScript code for a simple neural network:
// Define the architecture of the neural network
const network = new NeuralNetwork({
inputNodes: 2,
hiddenLayers: [3],
outputNodes: 1,
activation: "sigmoid",
});
// Train the neural network with sample data
const trainingData = [
{ input: [0, 0], output: [0] },
{ input: [0, 1], output: [1] },
{ input: [1, 0], output: [1] },
{ input: [1, 1], output: [0] },
];
for (let i = 0; i
Step 4: Training and Testing the Neural Network
After building, you must train and test your neural network. Training means giving it data, then adjusting its weights and biases to reduce errors. Testing it with new data checks its accuracy. Training and testing are vital for its success.
Step 5: Fine-tuning and Optimizing the Neural Network
After testing, you should fine-tune and optimize your neural network. Try different settings and algorithms to get the best performance. This stage requires patience, as improvements can take time.
Following this tutorial, you're on your way to mastering neural networks in JavaScript. With time and experimentation, your skills in creating advanced models will grow.
Next, we'll look at some popular neural network libraries in JavaScript to help you further.
Exploring Neural Network Libraries in JavaScript
There are many neural network libraries in JavaScript to speed up development. These tools ease the creation of neural networks for web AI projects. We'll look at popular libraries and their features to help you pick the best one.
TensorFlow.js
TensorFlow.js is a top neural network library. Made by Google, it's got all you need to make machine learning models online. You can use models ready to go or build your own. TensorFlow.js is good for many AI jobs, working with deep learning too. It also works well with Python models.
Keras.js
Keras.js makes using Keras in JavaScript easy. It uses TensorFlow.js for a simple API, great for trying out new ideas fast. In Keras.js, making and training neural networks is straightforward. It's chosen by many for its ease of use. You can mix and match parts of it to fine-tune your models.
Brain.js
Brain.js is known for being simple and light. It has the basics for making neural networks in JavaScript. It can do different kinds of neural networks. Brain.js is perfect for newcomers or anyone who wants a simple option.
These libraries each have something special for various projects and needs. By checking them out, you can find one that fits your AI project well.
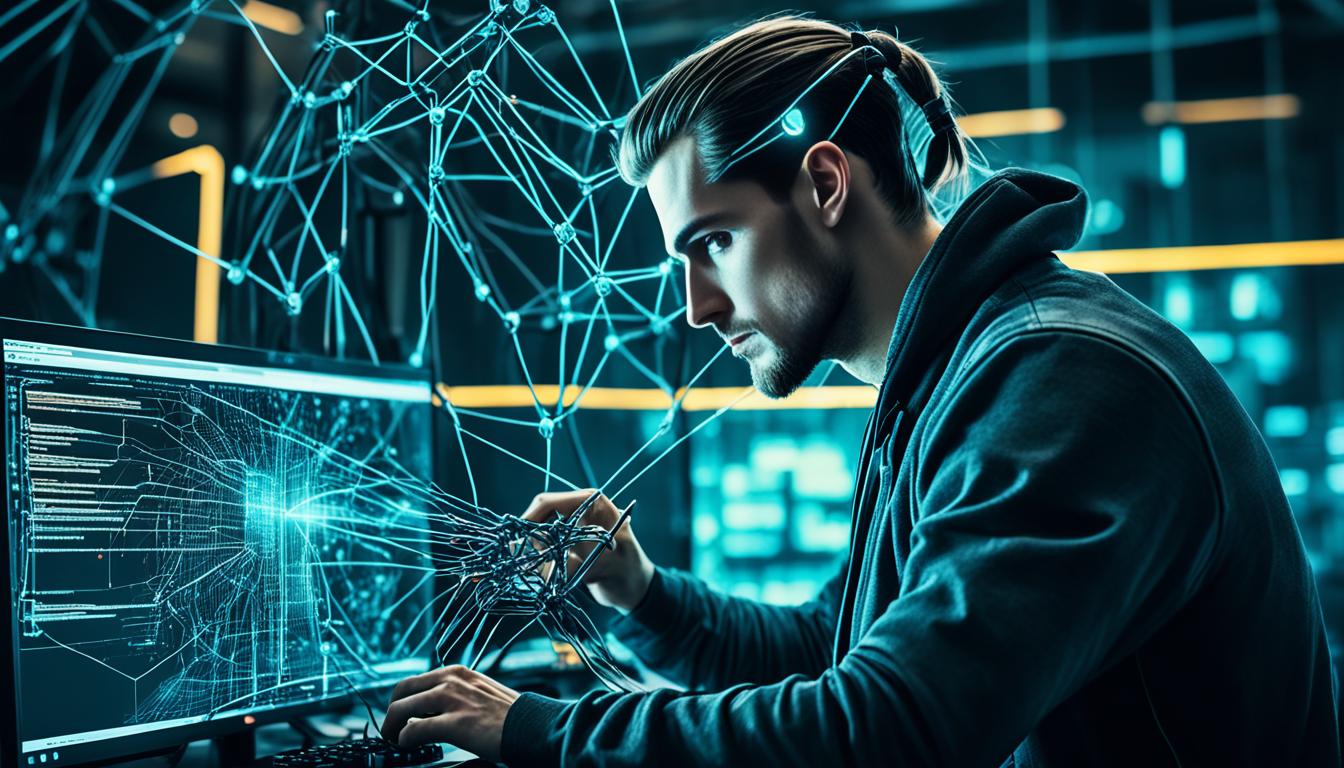
If you're interested in a comparison of these libraries, take a look at the table below:
Library | Features | Advantages | Limitations |
---|---|---|---|
TensorFlow.js | Support for traditional neural networks and deep learning architectures, seamless integration with TensorFlow ecosystem | Industry-standard, extensive documentation and community support | Steep learning curve for beginners |
Keras.js | High-level API, simplicity, easy prototyping | Smooth transition from Keras, wide range of pre-trained models available | May lack some advanced features compared to TensorFlow.js |
Brain.js | Simple building blocks, lightweight | Minimal learning curve, ideal for beginners | May not support all advanced neural network architectures |
Looking at the features, advantages, and limits of these libraries helps you choose right for your projects.
Deep Learning Capabilities in JavaScript
Deep learning is part of neural networks, which looks at complex patterns and structures. It lets machines handle tasks that need advanced thinking. JavaScript is key here. It has strong libraries for deep learning in web AI tasks. These tools help developers use deep learning's power more easily.
TensorFlow.js is a big deal in JavaScript deep learning. Google made this open-source tool. It helps if you know TensorFlow from Python. It lets you build and train deep neural networks right in your browser. You can even start with models that are already trained to make your AI projects better.
Brain.js focuses on JavaScript for deep learning too. It's made to be straightforward, with easy-to-use APIs. You can build neural networks, train with your data, and do things like figuring out feelings, recognizing images, or understanding language.
TensorFlow.js
TensorFlow.js blends deep learning with JavaScript. It lets developers make, train, and use deep learning models in the browser. This boosts AI applications on the web. You can pick from simple to advanced API levels, depending on what you need.
Here are its key features:
- Different model types, like CNNs and RNNs.
- Fast training and use with GPU boost, using your computer's own power.
- Models ready to go for common uses, saving you time and resources.
- Using pre-trained models for tasks that are alike, saving effort.
With TensorFlow.js, you can explore deep learning in JavaScript. This opens up new chances for AI on the web.
Brain.js
Brain.js is made specifically for neural networks in JavaScript. It makes deep learning simple, so you can bring AI into your JavaScript projects more easily.
Its main features include:
- It's easy to start and use, even if you're new to this.
- Clear APIs to build neural network setups and set training.
- Fast GPU use for quicker training and figuring things out.
- It works with learning on its own or from examples.
Brain.js helps you use deep learning in JavaScript. This makes smart web applications possible.
Comparing TensorFlow.js and Brain.js
Library | Key Features |
---|---|
TensorFlow.js |
|
Brain.js |
|
When comparing, TensorFlow.js has more features than Brain.js. It's a full platform with many model types, GPU speed, and ready-made models. Meanwhile, Brain.js is simpler and easier, great for a straightforward neural network approach.
Using deep learning with JavaScript, thanks to libraries like TensorFlow.js and Brain.js, transforms web applications using AI. Whether for recognizing pictures, understanding language, or other tasks, JavaScript is a strong and easy-to-use choice for deep learning.
Advantages of Neural Networks in JavaScript
Neural networks have changed how we handle AI projects online. They let developers fully use artificial intelligence's power. With JavaScript, these networks make smart applications possible.
Enhanced Performance and Speed
Neural networks in JavaScript work fast, especially with complex jobs. They combine JavaScript's speed and their own parallel processing. This gives quick responses and better performance.
They tackle big problems by breaking them into smaller pieces. This means neural networks can handle many tasks at once, improving AI applications.
Flexibility and Accessibility
JavaScript is a common language that is easy to use and versatile. With neural networks, developers can use machine learning easily. This makes developing simpler and lets AI apps work on all devices.
JavaScript's big community offers many tools and libraries for neural networks. This helps developers share ideas and improve AI models together.
Intuitive Development Environment
JavaScript is user-friendly for both new and expert developers. It has a straightforward style and plenty of libraries. Developers can quickly work with neural networks, making data easy to see and adjust in real-time. This helps in making AI models better.
Seamless Integration with Web Technologies
Neural networks and JavaScript together let developers add AI to web apps easily. They work well with HTML and CSS for making interactive user interfaces. So, AI models can be added to websites, chatbots, and more. This leads to better user experiences and personalized services.
Neural networks and JavaScript together open many doors for web AI projects. They bring great performance, ease of use, a friendly development setting, and easy integration with web tech. They are great for developers wanting to make smart and new solutions.
Let's look at the table below to see neural networks' unique benefits in JavaScript:
Advantage | Description |
---|---|
Enhanced Performance | Neural networks in JavaScript use parallel processing and modular structure for speed and efficient computing. |
Flexibility and Accessibility | JavaScript's wide use and neural networks mean no extra dependencies are needed for developers. |
Intuitive Development Environment | JavaScript's simple syntax and visualization help make and improve AI models easier. |
Seamless Integration | These networks fit well into web tech, enabling interactive and custom AI apps. |
Using neural networks in JavaScript lets developers create strong AI apps. These can change industries and make user experiences better.
Practical Applications of JavaScript Neural Networks
JavaScript neural networks help in many AI areas. They let developers build apps that handle complicated tasks. Here are examples of their use in the real world:
1. Image Recognition
Image recognition is a thrilling use for JavaScript neural networks. They learn to spot objects, faces, and patterns in photos very well. This tech can manage smart photo apps or facial recognition systems.
2. Natural Language Processing
In natural language processing (NLP), JavaScript neural networks are key. They understand text, which helps make language tools, sentiment systems, and chatbots. This technology is changing our conversations with machines.
3. Recommendation Systems
Recommendation systems use JavaScript neural networks a lot. They analyze what users like to offer personalized suggestions in shopping, movies, and more. They make these systems work better, keeping users happy.
4. Financial Predictions
Banks and financial groups use JavaScript neural networks for smart predictions. They look at past money data to forecast stocks and trends. This tech is crucial for smart finance choices.
5. Fraud Detection
To stop fraud, JavaScript neural networks check transactions for odd patterns. They help catch credit card fraud and false insurance claims fast. This technology is essential for keeping business and people safe.
JavaScript neural networks are super versatile and effective. They're used in recognizing images, processing languages, making suggestions, predicting finances, and detecting fraud. These networks are changing lots of industries. Developers use this smart tech to create exciting AI projects.
"JavaScript neural networks are powering cutting-edge applications, revolutionizing industries across the board." - AI Expert
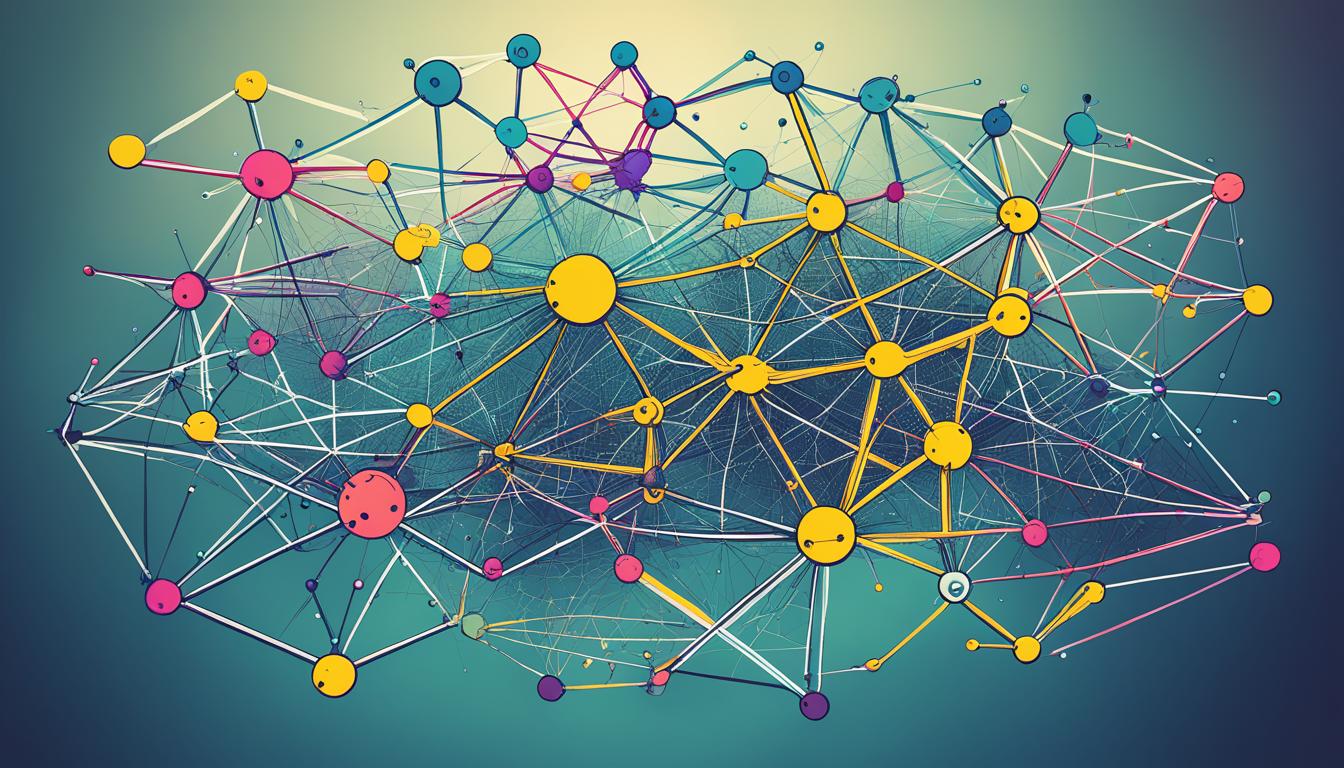
Application | Use Case |
---|---|
Image Recognition | Identifying objects, faces, and patterns in images with high accuracy |
Natural Language Processing | Language translation, sentiment analysis, and chatbots |
Recommendation Systems | Personalized recommendations in e-commerce, entertainment, and content platforms |
Financial Predictions | Stock price prediction, market trend analysis, and investment forecasting |
Fraud Detection | Real-time identification of suspicious transactions and activities |
Overcoming Challenges in JavaScript Neural Networks
Neural networks in JavaScript have huge potential. Yet, developers often face challenges during their setup. Knowing how to handle these issues is key to successful neural network applications in JavaScript.
Memory and Processing Limitations
Working with neural networks in JavaScript brings up issues like limited memory and processing power. Training complex models and managing big datasets need a lot of computational resources. JavaScript runs on users' devices, which adds more constraints.
To tackle this, developers use optimization strategies. They cut down on unnecessary calculations, pick efficient data structures, and try parallel computing in browsers. Using JavaScript machine learning frameworks also helps. These frameworks are made for better performance and memory use.
Data Preprocessing and Feature Engineering
Preparing data for neural networks in JavaScript is vital. This step includes handling different data formats and making the data represent the problem well. It's important for training neural networks effectively.
Developers use JavaScript libraries for easier data preprocessing. These libraries come with tools for transforming data and feature scaling. This makes the preprocessing step less of a hassle.
Model Selection and Hyperparameter Tuning
Choosing the correct neural network model and hyperparameters is crucial. It can be tough to know which architecture and settings will work best. But it's necessary for good model performance.
Developers solve this by testing models and evaluating them. They use JavaScript neural network libraries that automate this process. These tools help in selecting the best model and settings.
Debugging and Error Handling
Debugging is a crucial part of neural network development in JavaScript. It's complex because of the detailed data and model structures.
Developers get better at this by using neural network-specific debugging strategies. These include visual tools and monitoring metrics. Debugging tools designed for neural networks also help find and fix errors quickly.
Model Interpretability and Explainability
Neural networks are complex, making them seem like a black box. It's often hard to explain how they make decisions. This can be a problem when you need clarity.
Techniques like feature importance analysis and model visualization help. Using tools like Grad-CAM also makes neural networks easier to understand. This makes explaining their decisions more straightforward.
By facing these challenges head-on, developers can harness the power of neural networks in JavaScript. This unlocks new possibilities in AI and advanced web applications.
Challenges | Strategies |
---|---|
Memory and Processing Limitations | Optimize code, leverage machine learning frameworks |
Data Preprocessing and Feature Engineering | Utilize JavaScript libraries for data preprocessing |
Model Selection and Hyperparameter Tuning | Experiment, evaluate models, automate hyperparameter tuning |
Debugging and Error Handling | Use debugging techniques and tools specific to neural networks |
Model Interpretability and Explainability | Apply feature importance analysis, model visualization, Grad-CAM |
Future of Neural Networks in JavaScript
Technology keeps advancing, leading to a bright future for neural networks in JavaScript. There are new developments that show lots of promise for AI on the web. We will look at the exciting prospects for JavaScript neural networks.
Advancements in JavaScript Libraries
JavaScript libraries for neural networks are getting better. Developers will see more features, higher performance, and ease of use. These improvements will allow for creating advanced neural network models. This leads to better predictions and insights.
Integration with Web Technologies
JavaScript neural networks will blend well with other web tech. This makes it possible to build AI apps that work in browsers. Imagine using real-time image recognition or chatting with AI, all thanks to JavaScript neural networks enhancing the web.
Expansion of Deep Learning Capabilities
Deep learning helps recognize complex patterns. Soon, JavaScript will offer tools for more complex AI tasks. This really opens the door for innovative web AI applications.
Collaborative AI Systems
JavaScript neural networks will become more user-friendly and powerful. We will see AI systems that learn from each other. This boosts the power of AI by sharing knowledge, making all models smarter.
Applications in IoT and Edge Computing
JavaScript neural networks will be part of IoT and edge computing. They'll be in smart and edge devices, bringing AI closer to us. This means faster, smarter decision-making right where it's needed.
Ethical Considerations and Responsible AI
As these networks grow, so does the focus on ethics and privacy. There's a big push to make AI fair and safe. Making AI responsibly will shape its future in JavaScript.
Looking ahead, the future for neural networks in JavaScript shines bright. With new advancements, developers can make web AI that changes businesses and how we interact with technology.
Conclusion
Neural networks in JavaScript can change web-based AI projects in big ways. By using JavaScript, developers can easily create smart applications. This lets them dive into machine learning model insights.
JavaScript is great for machine learning because it fits well into web development. It offers a known language for using neural networks. Developers can pick from many libraries and frameworks, finding what works best for them.
The future of neural networks in JavaScript looks bright. It holds the promise of new AI discoveries. By using neural networks, we can explore exciting possibilities. These include image recognition and understanding natural language. This empowers developers to make advanced AI applications.
FAQ
What are neural networks?
Neural networks are like a brain model developed for machines. They have artificial neurons linked together. These networks process info, learn, and make smart guesses.
Why should I implement neural networks in JavaScript?
JavaScript is popular for making websites and is very useful. Using JavaScript, you can add smart features to your website apps using neural networks.
Are there any JavaScript libraries or frameworks for implementing neural networks?
Yes, for neural networks, JavaScript has libraries like TensorFlow.js, Brain.js, and Synaptic.js. They make it easier to build and improve your smart models.
Can JavaScript handle deep learning tasks?
Absolutely, JavaScript is equipped for deep learning. Libraries such as brain.js and TensorFlow.js allow you to create deeper neural networks. This means you can solve more complex problems.
What are the advantages of using neural networks in JavaScript?
Neural networks in JavaScript are great for web apps, work fast on browsers, and have a supportive community. They make apps smarter without much trouble.
What are some practical applications of JavaScript neural networks?
You can use JavaScript neural networks for cool stuff. Like recognizing images, understanding language, finding trends, recommending things, and spotting oddities. All within web projects.
What challenges might I encounter when implementing neural networks in JavaScript?
You might find it tricky because of slower client-side processes, dealing with big data, and making things work on all browsers. Yet, with the right tools and tricks, you can overcome these hurdles.
What does the future hold for neural networks in JavaScript?
The outlook is bright for JavaScript neural networks. With tech advancements, expect better performance and more web apps using AI. This will lead to innovative solutions.