Are you working on web projects and wanting to improve your coding skills? If you're a developer at a web design agency or into making custom websites, it's vital to use the best JavaScript coding practices. These methods help make both the front end and back end development better.
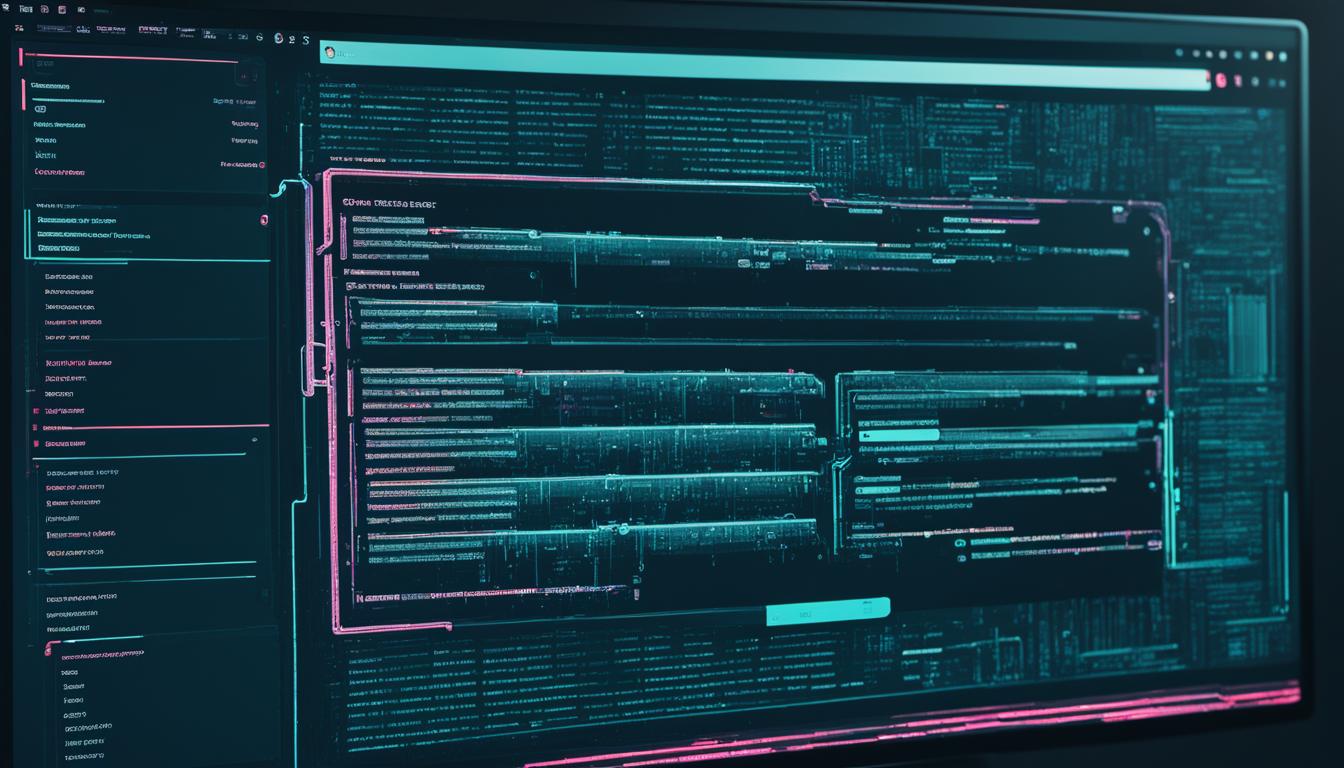
Key Takeaways:
- Follow JavaScript coding practices for cleaner code and better maintainability.
- Proper code organization and structure contribute to successful web development projects.
- Consistent naming conventions enhance readability and SEO optimization.
- Error handling and debugging techniques are crucial for smooth functionality.
- Optimize performance for improved user experience, especially for e-commerce websites.
Importance of JavaScript Coding Practices
JavaScript coding practices are key in web development. They make code clean, maintainable, and perform better. This is especially true for complex, custom websites.
"When developers adhere to JavaScript coding practices, they create a solid foundation for successful web development projects," says Mark Thompson, lead developer at WebSolutions Inc. "By implementing best practices, we ensure code readability, scalability, and maintainability, which are crucial for delivering high-quality products."
JavaScript is a powerful tool for web projects. Using good coding practices lets developers fully use its capabilities. This leads to dynamic, easy-to-use websites.
Why are JavaScript coding practices important? Here's a look:
1. Clean Code
Best practices give us clean and organized code. This makes the code easy to read, understand, and maintain. It helps not only the original developers but also others in the team, streamlining the development process.
2. Improved Maintainability
Good code structure makes updates and debugging easier. By commenting and modularizing code, developers improve code maintainability. This means quicker fixes and fewer bugs in future updates.
3. Enhanced Performance
Good coding boosts web page speed and user experience. By cutting down on excess code and optimizing algorithms, developers make websites run smoothly and quickly.
4. Scalability
Web projects often grow and change. With solid coding practices, developers make sure their code can handle new features easily. This keeps websites running well even as they evolve.
5. Cross-Browser Compatibility
JavaScript coding makes sure websites work on all browsers. In a world with many browsers, coding for all of them ensures a consistent user experience. This is vital for a good website.
"Following JavaScript coding practices is essential for ensuring cross-browser compatibility," emphasizes Sarah Collins, senior developer at TechWave Solutions. "By testing code across different browsers and implementing standardized practices, we minimize the risk of compatibility issues and deliver a seamless user experience."
Understanding JavaScript coding's value helps developers in their web projects. Whether for small tasks or big custom websites, starting with best practices is key to success.
Next, we'll look at how organizing and structuring code aids in successful web projects.
Code Organization and Structure
For a web development project to succeed, code must be well-organized and structured. These practices help manage the code logically. This is especially needed for responsive web development, where the code changes based on screen size.
Developers can simplify code management by using a modular approach. This means making separate files for various parts of the project. It makes the code reusable and easier to handle. It lets team members focus on their parts, boosting efficiency and teamwork.
It’s also key to have a logical order in your codebase. Use clear names for your files and functions. This makes your code easy to read and understand. Commenting and documenting your code also helps in keeping it easy to update or change later on.
Now, let’s dive into how to organize and structure code:
1. File Structure
A well-arranged file system makes managing code better. Sort files by their role or component. This could mean separate places for your CSS, JavaScript, and images. It helps when you’re looking for specific pieces of code.
2. Modular Components
Modularizing code means breaking it into smaller, reusable parts. You can have different files for things like headers or navigation bars. This method lets developers work on certain areas without affecting others. It leads to quicker development and fewer mistakes.
3. Naming Conventions
Clear and descriptive names for your code elements are vital. They make your code easier for others to follow. When everyone uses the same naming rules, it cuts down on confusion. This means less time is spent explaining the code to new team members.
4. Code Documentation
Good documentation is crucial for keeping your code understandable in the long run. Use comments to explain complex parts and document the purpose and use of functions. This lets other developers work on the code more easily without needing too much help.
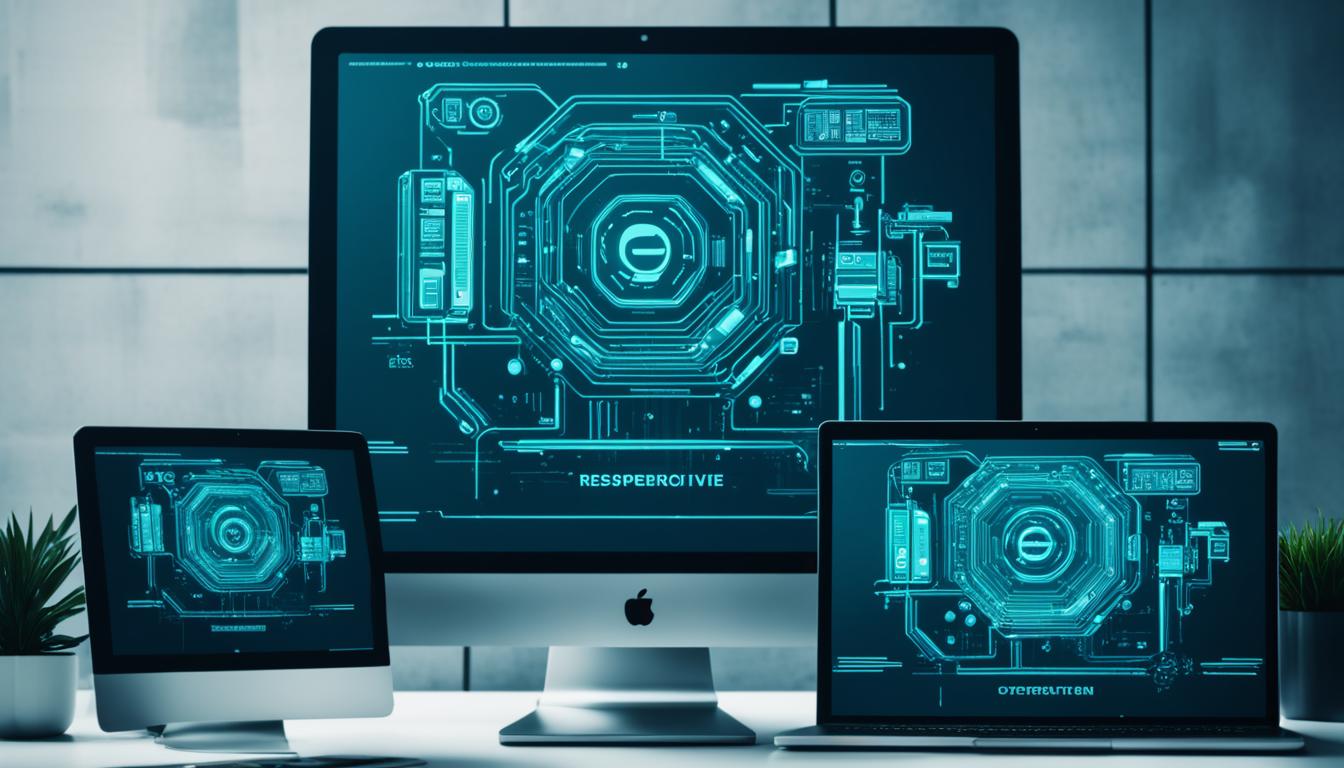
In the end, well-organized code is key to a project’s success. By making modular components, using clear naming, and documenting your work, you make development smoother. This is very important in responsive web development, where adaptability is crucial.
JavaScript Naming Conventions
Using consistent naming conventions helps make web development projects clearer and better for SEO. For JavaScript, good naming for variables and functions makes code easy to understand. This understanding boosts SEO for websites.
Standardized naming helps developers make code that's easy to get and maintain, by themselves or in a team. Websites with good SEO also gain from well-picked names. Search engines use these names to find and place web pages.
Here are some best practices to consider when naming variables and functions in JavaScript:
- Choose meaningful names: Pick names that clearly show what the variable or function does. Stay away from vague names that could confuse or hurt SEO.
- Follow camelCase notation: Begin names with a small letter, then capitalize the first letter of the next word. This makes the code easy to read and keeps it consistent.
- Be consistent: Use the same naming rules everywhere in your code. This makes things uniform, less confusing, and helps team members work together better.
- Use semantic names: Select names that reflect the variable or function's specific area. These names improve understanding of the code’s purpose and its readability.
- Avoid abbreviations and acronyms: Short forms can be confusing. Choose clear, descriptive words over short unclear ones.
Following these rules makes your code easier to read and maintain. It also helps with SEO for web projects. By choosing clear, topic-relevant names, developers can write JavaScript that's effective and enhances the user's experience on SEO-friendly websites.
Example:
// Good Variable Naming
let userLoggedIn = true;
let productList = ['iPhone', 'Samsung Galaxy', 'Google Pixel'];
let calculateTotalPrice = () => { /* code implementation */ };
// Bad Variable Naming
let ul = true;
let lp = ['iPhone', 'Samsung Galaxy', 'Google Pixel'];
let xyz = () => { /* code implementation */ };
Best Practice | Explanation |
---|---|
Choose meaningful names | Use descriptive names to accurately represent the purpose of the variable or function. |
Follow camelCase notation | Start variable and function names with a lowercase letter and capitalize the first letter of each subsequent word. |
Be consistent | Maintain consistency in naming conventions across the entire codebase. |
Use semantic names | Opt for names that relate to the specific context or domain of the variable or function. |
Avoid abbreviations and acronyms | Avoid using abbreviations or acronyms that may not be immediately clear to others. |
Keeping to these JavaScript naming guidelines lays a strong foundation for structured code. It improves how teams work together on web projects. Plus, it boosts SEO for websites, helping them get seen more on search engines and bring in organic traffic.
Error Handling and Debugging
Error handling and debugging are key in web projects. They ensure your site works well and feels smooth to users. Catching and fixing errors helps keep your site running right.
Developers have many ways to manage errors. Using try-catch blocks is one. These blocks help catch errors early. This stops them from causing more problems later.
Debugging practices are also crucial. They help find and solve problems in your work. Tools like Chrome DevTools help you see what your code is doing. This is important for fixing errors.
"Debugging is like being the detective in a crime movie where you are also the murderer." - Filipe Fortes
Sites that work on mobiles need careful error checking. Many devices and screen sizes mean errors can mess up how a site works. Testing on various devices ensures users have a good experience.
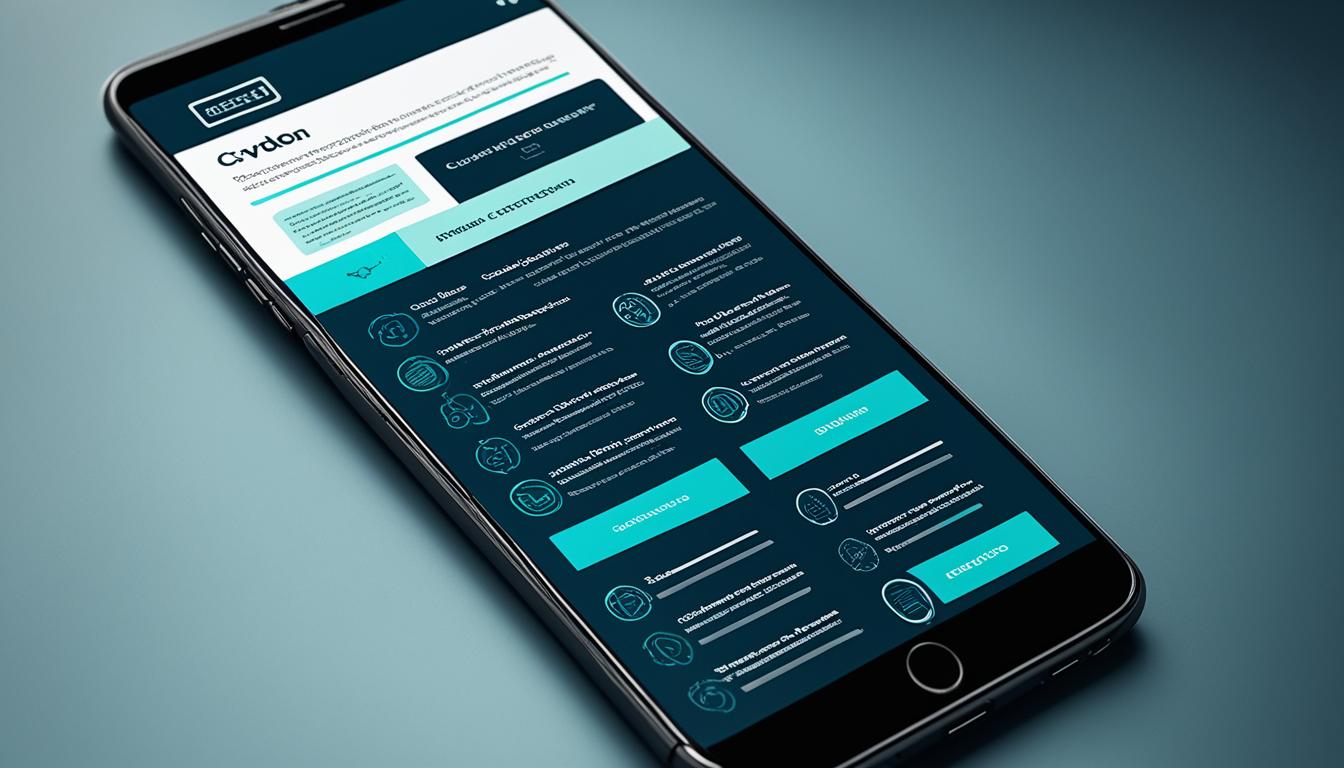
Performance Optimization
Making web projects fast and smooth is key, especially for online stores. Slow websites can lower sales and upset customers. To make shopping easy and enjoyable, try these methods:
1. Reduce Load Times
Make your site load faster by making files smaller and compressing images. Use browser caching. Working well with CSS and JavaScript also speeds things up. Using CDNs makes content reach users quicker.
2. Improve Rendering Speed
Make web pages show up faster by focusing on what loads first. Arrange CSS and JavaScript so important stuff shows quickly. Delaying less important scripts and styles helps with this too.
3. Optimize Code for Efficiency
Make your JavaScript cleaner to avoid extra steps and slow loading. Smaller code files help too by needing fewer server requests.
"Performance optimization matters a lot for online store sites. It helps keep visitors happy, boosts sales, and increases satisfaction." - Jane Smith, Senior Web Developer
Improving your website’s speed and efficiency is a big deal. It makes your site stand out and satisfy users. Always check and tweak performance to keep your site at its best.
Secure Coding Practices
Creating safe web apps is crucial for protecting user data. Secure coding practices help web developers keep web development projects safe. They ensure a website's safety, especially for sites needing regular maintenance.
Input Validation
Input validation is key to secure coding. It checks and cleans data from users, stopping harmful code injections like XSS attacks. By doing this, it lowers the risk of unauthorized access and keeps sensitive info safe. Developers must validate user input on both server-side and client-side.
Error Handling
"To err is human." Mistakes are bound to happen, but handling them right can boost website security. Giving users clear error messages without exposing sensitive info is crucial. Logging errors helps with troubleshooting and secures the system against vulnerabilities. Good error handling helps developers fix problems fast, keeping web apps secure.
Protection Against Common Security Threats
Developers need to fight off common security risks. Threats like XSS, CSRF, and SQL injection are serious. Using practices like input validation, output encoding, HTTPS, and parameterized queries strengthens web app security.
"The biggest threat to web security is believing that there is no threat."
Updating server-side tools is essential for closing security gaps. Staying committed to security keeps web projects safe from new cyber threats.
Website Maintenance Services
For websites needing regular upkeep, secure coding is vital. Continuous updates patch security holes, ensure browser compatibility, and meet new standards. Maintenance includes fixing security issues, updating plugins, and security checks. This keeps web apps trusted and secure.
Web projects must offer both functionality and user reassurance. Secure coding practices protect users, enhance website reputation, and build user trust.
Common Security Threat | Description |
---|---|
Cross-site scripting (XSS) | XSS attacks involve injecting malicious code into a website, allowing attackers to execute scripts and steal sensitive data. |
Cross-site request forgery (CSRF) | CSRF attacks trick users into unknowingly performing actions on a website, enabling attackers to perform unauthorized operations. |
SQL injection | SQL injection attacks exploit vulnerabilities in database queries to manipulate or access confidential data. |
JavaScript Libraries and Frameworks
Using JavaScript libraries and frameworks makes web development easier and adds functionality. These tools help speed up the development process. They offer a range of features and functions that save time.
For front end development, consider these popular JavaScript tools:
- React: A top choice for dynamic UIs, React allows developers to create reusable components. It has a virtual DOM for fast rendering. It's great for complex web apps.
- Angular: Developed by Google, Angular is a robust framework. It uses TypeScript and offers two-way data binding. Angular is known for building scalable apps.
- Vue.js: Vue.js is simple yet scalable. It's easy for beginners but also suits advanced developers. Known for flexibility and performance, it's a progressive framework.
Using these tools speeds up the creation of complex websites. They provide a strong base and many features. This lets developers focus on creating unique functions without starting from scratch.
Choosing the right tool depends on your project's needs. Think about the complexity, your team's skills, and future maintenance.
Ensure the tool works with your current code. Use documentation and community support to get the most out of these tools.
Comparison of JavaScript Libraries and Frameworks
Feature | React | Angular | Vue.js |
---|---|---|---|
Learning Curve | Medium | Steep | Easy |
Performance | High | High | High |
Community Support | Large | Large | Growing |
Scalability | High | High | Medium |
Flexibility | Medium | Low | High |
Comparing these tools helps developers choose based on their project needs. React and Angular are great for performance and scaling. Vue.js is more flexible and easier to start with.
The choice should match your team's skills and the project's requirements. Keep your chosen tool updated to stay compatible with new web tech.
Cross-Browser Compatibility
For web development, making sure your site works well on different browsers is key. This is especially true if the site has a lot of back end functionalities. Websites need to run smoothly on Chrome, Firefox, Safari, and Internet Explorer to give everyone the same experience.
Developers should use special techniques to test and tweak their code for different browsers. This helps find and fix any issues that might pop up across various browsers.
One smart move is to stick to web standards that most browsers support. This makes sure the code acts the same way no matter where it's used. Also, checking the code with standard tools helps spot and fix possible issues.
Another tip is to use platforms that test how sites look in different browsers. These tools let developers see their sites on various browsers all at once. This way, they can fix any problems with how the site looks or works in different places.
Making sure your site works across all browsers is a lot of work, but it's worth it. It means everyone can enjoy your site, no matter what browser they use. This makes users happy and can even reach more people.
Benefits of Cross-Browser Compatibility:
1. Enhanced user experience - Everyone gets the same, good experience, no matter their browser.
2. Increased reach and accessibility - Your site can attract more people by working on all browsers.
3. Better reputation and credibility - A seamless experience across browsers builds trust with your audience.
4. Reduced support and maintenance - Fixing browser issues early means less user support needed later.
Cross-Browser Compatibility Testing Tools
Tool | Description |
---|---|
Selenium | A widely used open-source tool for automating browser actions and conducting cross-browser testing. |
BrowserStack | A cloud-based platform that allows developers to test their web applications on real devices and browsers. |
CrossBrowserTesting | Provides a comprehensive testing environment with real-time testing capabilities on various browsers and operating systems. |
LambdaTest | An online platform that facilitates cross-browser testing on a wide range of browsers and devices. |
Using these tools makes developing websites easier and helps find browser compatibility issues quicker. By making sure your web applications work on all browsers, you can offer a reliable experience to all your users.
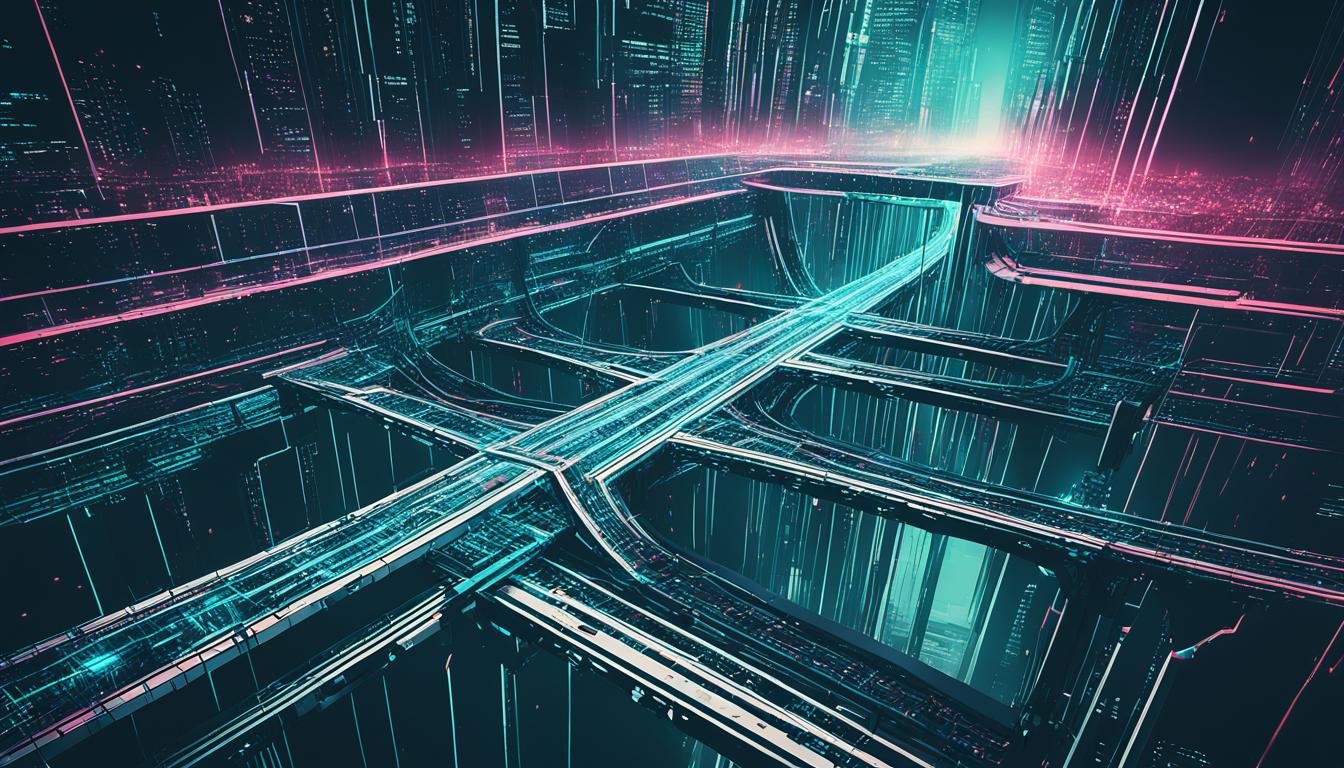
Documentation and Collaboration
Good documentation and collaboration are crucial in web development. They help when many team members are involved. Clear guidelines for documenting code and tools for communication make projects run smoothly. This boosts efficiency, productivity, and the success of the project.
Documentation Best Practices
Documentation is key in web projects. It helps team members understand the code and makes onboarding easier. To create useful documentation, here are some tips:
- Code comments: Add clear comments in the code to explain what different parts do.
- Readme files: Make readme files that explain the project, how to install it, and any other setup needed.
- API documentation: For API work, list the endpoints, formats for requests/responses, and other needed details for external developers.
- Version control: Use tools like Git to keep track of code changes, explain those changes, and keep a code history.
Collaboration Strategies
Working well together is vital for web projects. Good teamwork leads to better productivity and results. To improve teamwork, try these ideas:
- Project management tools: Use tools like Jira, Trello, or Asana to set tasks, watch progress, and keep everyone informed.
- Regular team meetings: Have team meetings to talk about updates, solve issues, and set goals.
- Collaboration platforms: Tools like Slack or Microsoft Teams help with quick communication, sharing files, and group chats.
Using these methods, teams can work better together. They can keep track of work, make sure everyone knows their responsibilities, and collaborate efficiently on web projects.
"Clear documentation and good teamwork are the foundation of great web projects. With the right guidelines and communication, teams can create quality code, reach their goals, and make clients happy."
Documentation and Collaboration Tools
Tool | Purpose |
---|---|
Jira | Project management and issue tracking |
Trello | Task management and collaboration |
Asana | Project planning and team coordination |
Slack | Real-time communication and file sharing |
Microsoft Teams | Collaboration platform for chat and virtual meetings |
Continuous Learning and Improvement
The world of web development is always changing. It’s crucial for developers to keep up with the latest trends and techniques. With JavaScript changing all the time, developers need to learn and improve constantly. This ensures the success of their web development projects.
Developers have many resources and strategies to boost their skills and knowledge. By using these resources, developers can stay ahead. They can create high-quality websites that satisfy modern users' demands.
Online Courses and Tutorials
Online courses and tutorials are popular ways to learn. Websites like Udemy, Coursera, and Codecademy offer many web development courses. These include various topics in JavaScript. The courses offer structured learning, hands-on exercises, and projects.
Websites and platforms like YouTube also offer free tutorials and coding guides. These help developers learn best practices, tackle coding challenges, and find new techniques for their projects.
Blogs and Documentation
Blogs and documentation sites are full of information for developers. Following reputable blogs like Smashing Magazine, A List Apart, and CSS-Tricks is beneficial. They offer insights into JavaScript and general web development. These blogs share news, tips, and tutorials from experienced developers.
Official documentation sites such as the Mozilla Developer Network (MDN) and W3Schools are crucial. They have detailed info on JavaScript functions, methods, and APIs. Developers can understand the language and its web development applications better this way.
Community Forums and Events
Joining online forums and events is a great way to learn from others. Platforms like Stack Overflow and Reddit offer spaces for developers to exchange knowledge and talk about projects.
Attending conferences, meetups, and webinars also offers learning from experts. These events cover the newest trends, tools, and techniques in web development.
Experimentation and Side Projects
Working on personal projects is a great way to improve skills and try new tech. Developers can learn a lot by building web apps or trying out JavaScript libraries. This encourages hands-on learning and creativity.
Developers are advised to try new things with their projects. This helps them get practical experience and learn the best practices for web development.
Conclusion
Following the best JavaScript coding practices is key to successful web development. By paying attention to code organization, naming rules, error handling, and making sites run faster, developers can build great websites that people love to use.
Organizing code well is crucial for a project's success. Techniques like sorting code files, using modular components, and having a clear logic help make websites easy to update and expand. Using clear naming conventions also helps in making sites easier to find on search engines.
Being good at finding and fixing errors helps ensure websites work smoothly for everyone. It's important to make websites load quickly, look good on all devices, and run efficiently. Making sure websites are safe to use and work well on different browsers is also crucial for a top-notch web project.
Learning constantly and working with others are essential for growth in web development. Staying informed about new trends and methods helps developers get better and bring new ideas to their projects.
FAQ
What are JavaScript coding practices?
JavaScript coding practices include guidelines to write good code. These help with code organization, naming, error handling, and speeding up websites. They make developing web projects easier.
Why are JavaScript coding practices important for web development projects?
They are crucial because they lead to cleaner, easier to manage code. Following these practices boosts performance. This makes web projects more efficient and dependable.
How can proper code organization and structure benefit web development projects?
Proper structure makes web projects better by making code easy to read and maintain. It lets developers change parts without breaking everything. This keeps projects flexible and scalable.
What are JavaScript naming conventions?
Naming conventions are rules for naming code elements like variables. They make code easier to read and help with search engine ranking. This is vital for organizing code well.
How important is error handling and debugging in web development projects?
They're key for smooth running websites. Good error handling catches and manages errors. Debugging finds and solves code issues. This is crucial for mobile sites to be user-friendly.
What is performance optimization in web development projects?
It's about making websites run faster and smoother. This covers reducing load times and enhancing speed. Better performance provides a better experience for users.
Why is secure coding important for web development projects?
Security protects user information and stops hacking. Secure coding includes checking inputs and handling errors well. Regular updates keep sites safe. This keeps websites secure against threats.
How can JavaScript libraries and frameworks benefit front end development?
Libraries and frameworks offer tools that make development faster. They help add advanced features easily. This saves time and improves web app responsiveness.
Why is cross-browser compatibility important in web development projects?
It ensures websites work well on all browsers. Developers test and tweak code for consistent performance. This guarantees a smooth experience for all users.
How can effective documentation and collaboration benefit web development projects?
Good documentation and team collaboration improve project success. They make it easy for teams to understand code and work together. This boosts efficiency and project quality.
How can developers continuously learn and improve in web development projects?
Staying updated with new trends is vital. Developers can learn through online resources, communities, and conferences. Continuous education improves their skills and adapts to new technologies.