Asynchronous JavaScript is vital for modern web development. It helps developers make fast and responsive apps. Learning how to use the async/await syntax is crucial. This feature makes asynchronous programming easier and makes code clearer.
This article will cover everything about async/await in JavaScript. We'll look at how it works, its benefits, and how to use it well. You'll fully understand async/await by the end. Then, you'll be ready to improve your async JavaScript skills.
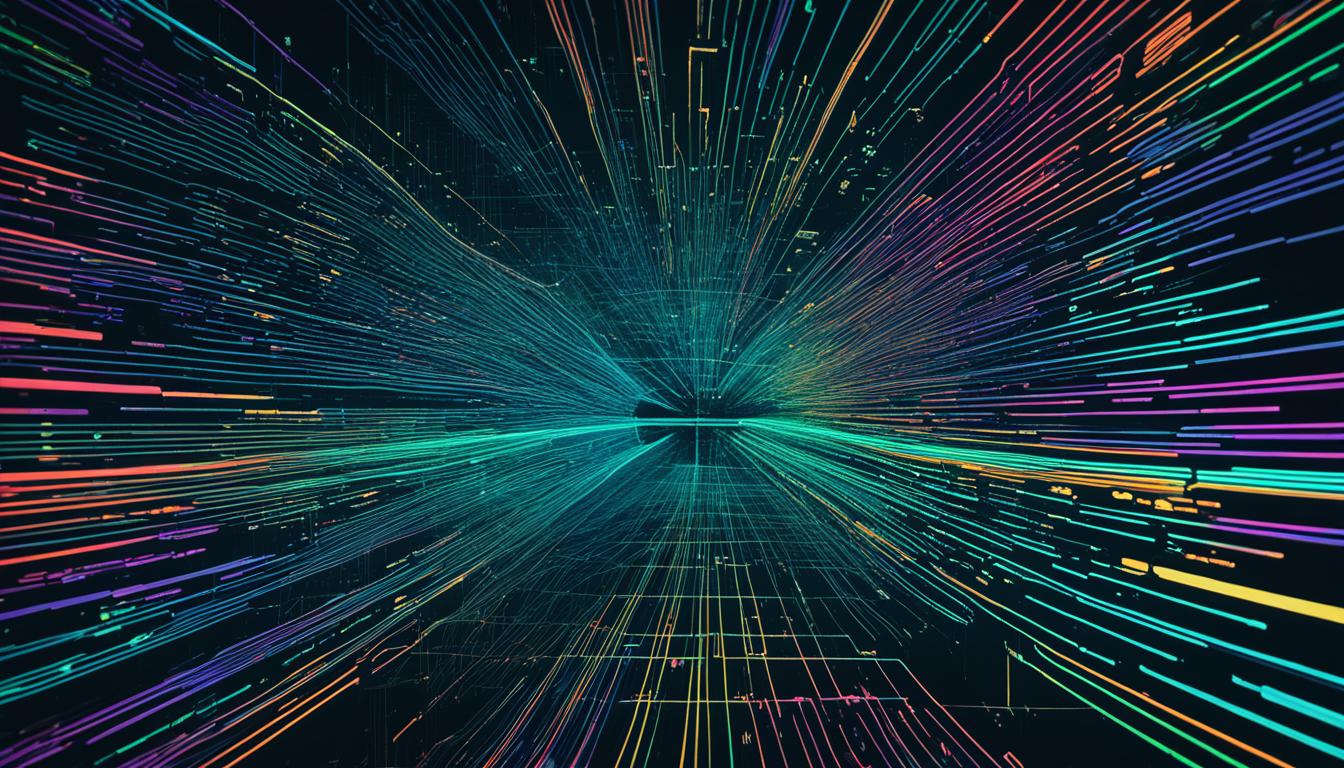
Key Takeaways:
- Asynchronous JavaScript is crucial for building responsive applications
- Async/await simplifies asynchronous programming and improves code readability
- Understanding how to use async/await with promises is essential
- Error handling becomes more streamlined with async/await and try/catch blocks
- Async/await can be used with APIs like the Fetch API for making asynchronous network requests
Understanding Asynchronous JavaScript
Asynchronous programming is key in JavaScript, helping to run code efficiently. This is crucial when dealing with tasks like getting data from servers or responding to user actions. Knowing two main concepts, JavaScript promises and async programming, is essential.
JavaScript Promises
JavaScript promises are objects that stand for the future outcome of asynchronous operations. They help developers manage async actions smoothly and effectively. With promises, code becomes cleaner and easier to keep up.
"Promises are a way to deal with asynchronous programming, providing developers with a more intuitive and organized approach to managing async actions."
The way JavaScript promises work is through functions like then()
and catch()
. These functions let the developer deal with successful outcomes or errors. This makes it easier to write code relying on several async actions.
Async Programming
Async programming lets a program run without stopping while it waits for async tasks to finish. This method boosts performance and makes things run smoothly, enhancing the user's experience.
Async functions, added in ES2017, make writing async code simpler. By using the async
keyword, functions automatically return a promise. This allows for the use of await
, which pauses the function until a promise finishes.
With async functions, code looks and behaves more like synchronous code, which means it's simpler to understand and maintain. Async programming provides a hands-on way to deal with async tasks, making the developer's code tighter, clearer, and more effective.
Benefits of JavaScript Promises | Advantages of Async Programming |
---|---|
|
|
Introduction to Async/Await
Asynchronous programming in JavaScript has changed how developers work with slow operations. The arrival of async/await syntax has made code involving asynchronous tasks easier and more straightforward.
The async/await keywords help developers write code that looks synchronous. This avoids long callbacks or complicated promises. Using async/await with async functions lets developers handle many tasks at once more smoothly.
Async/await makes code clearer and simpler to read. This helps others understand and maintain it better. The syntax stops a function until a promise is fulfilled, without stopping the main thread. This keeps the user experience smooth.
Let's see an example of using traditional async code to get data from an API:
fetch('https://api.example.com/data')
.then(response => response.json())
.then(data => {
// Process the data
})
.catch(error => {
// Deal with errors
});
Now, let's refactor it with async/await:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
// Process the data
} catch (error) {
// Deal with errors
}
}
fetchData();
This makes the code shorter and easier to understand. Async operations follow one another, which simplifies the flow. Errors are easier to manage with try/catch blocks too.
Async/await is not just for API calls. It's great for any asynchronous action like reading files or making database queries. It offers a more natural way to manage these operations. This improves both readability and how easy it is to maintain the code.
We will dive deeper into async/await in JavaScript. Looking at error handling to running multiple promises together. The upcoming sections will fully cover how to use this powerful tool.
Using Async/Await with Promises
Async/await makes JavaScript asynchronous programming simpler. It lets developers write code that looks synchronous. This method, combined with promises, makes handling async operations easy and code more readable.
The await
keyword pauses the code until a promise is either resolved or rejected. This creates a clear, linear flow. It's simpler than callbacks or using many then()
methods.
Here's an example of async/await with promises:
async function getData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
throw new Error('Failed to fetch data');
}}
In the getData()
function example, we mark the function as async. We then use await
to wait for fetch()
and response.json()
to complete.
If a promise fails, the try/catch
setup helps with handling errors. An Error
object is thrown if there's an issue.
Using async/await with promises makes the code cleaner and more like synchronous code. This makes it easier to follow and keep up.
Now, let's see how async/await deals with errors in another part.
Resources:
Sample Code:
Code | Description |
---|---|
async |
Declares that the function is asynchronous. |
await |
Pauses the execution of the code until the promise is resolved or rejected. |
try/catch |
Used for error handling in the async/await function. |
Error Handling in Async/Await
When you use async/await functions in JavaScript, it's key to handle errors well. Knowing how to manage errors helps keep your code stable and reliable. Let's look at how to handle errors in async/await, including try/catch blocks and promises that are rejected.
The try/catch Approach
Async/await lets you write code that looks synchronous. You can use try/catch blocks for error handling. Place your async code inside a try block to catch any errors that happen.
Let's see an example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data);
} catch (error) {
console.error('An error occurred: ' + error);
}
}
In this example, we get data using the fetch API. When the request works, we convert the response to JSON and show it. If there's an error, it's caught and shown.
Handling Rejected Promises
You can also manage rejected promises with async/await. It helps when you work with promise-returning functions.
Here's an example:
async function getUserName(userId) {
try {
const user = await fetchUser(userId);
return user.name;
} catch (error) {
console.error('Failed to fetch user: ' + error);
return 'Anonymous';
}
}
In this case, the getUserName function tries to fetch user data. If the promise is kept, we get the user's name. If not, an error is logged, and 'Anonymous' is returned.
By using try/catch and managing rejected promises, you make your async/await functions more reliable.
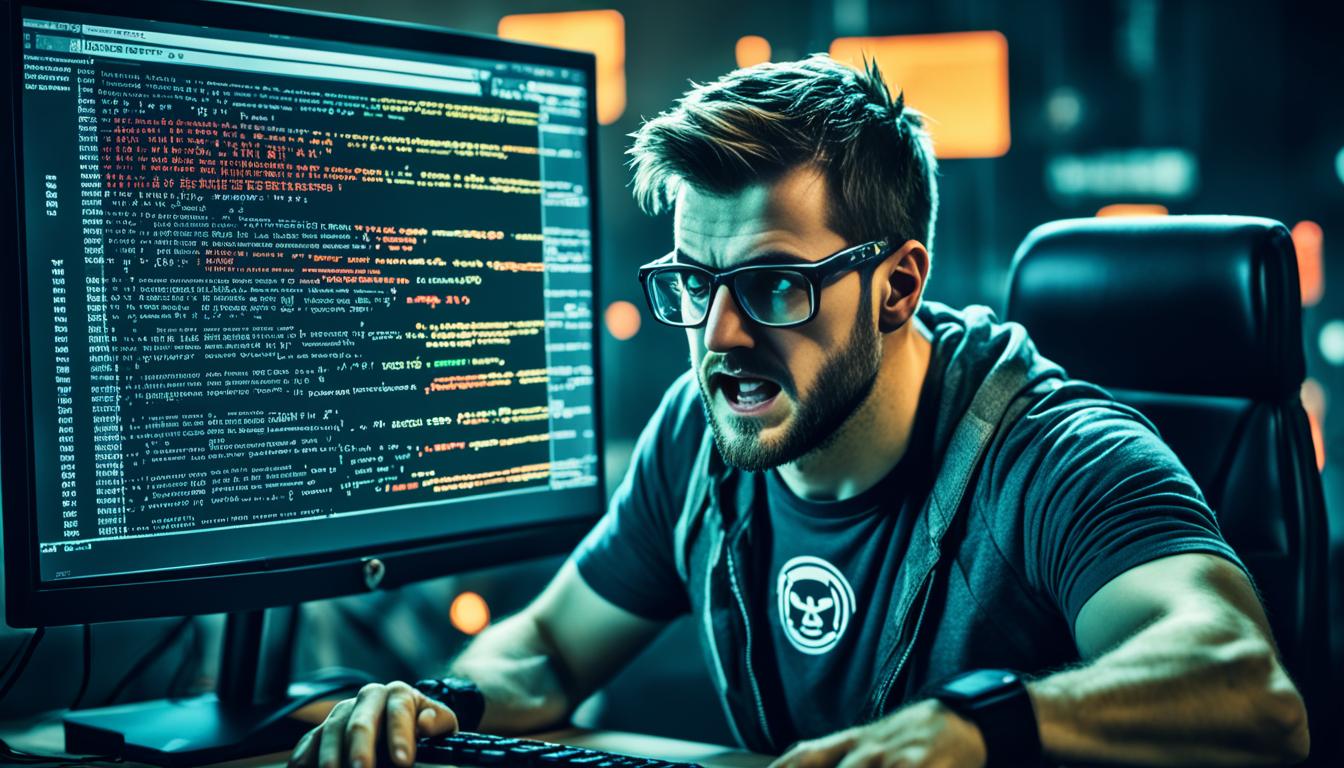
Now, let's dive into more complex ways to use async/await and deal with errors in tough scenarios.
Waiting for Multiple Promises with Async/Await
In working with asynchronous JavaScript, you might need to wait for many promises at once. Async/await makes this process simpler. It helps you write code that manages several asynchronous tasks without much hassle.
Using the Promise.all method is a way to handle multiple promises. Promise.all takes many promises and returns a new one. This new promise resolves when all the others do. This means your code only moves forward after every promise finishes.
Here's how you can use Promise.all with async/await:
async function getData() {
const promise1 = fetch('https://api.example.com/data1');
const promise2 = fetch('https://api.example.com/data2');
const promise3 = fetch('https://api.example.com/data3');
const [response1, response2, response3] = await Promise.all([promise1, promise2, promise3]);
// Process the responses...
}
The fetch function makes a few network requests here. Using Promise.all, we wait for all these requests to end at the same time. The await keyword pauses our code until every promise is done. Then, we can use the responses for further actions.
This method lets you handle many tasks at once, making your JavaScript code work faster.
Besides, there are other ways to manage multiple promises with async/await. It all depends on what your application needs. You might run promises one by one or control how many happen at once.
Overall, async/await offers an easy way to deal with many promises at the same time in JavaScript. By using Promise.all and other methods, you can make your code neat and efficient.
Concurrency Patterns with Async/Await
Different concurrency patterns exist when using async/await in JavaScript. Here are the main ones:
- Parallel Execution: This means running multiple tasks at the same time and waiting for all to finish. Use Promise.all or mix Promise with await.
- Sequential Execution: Here, tasks happen one after another. Each task waits for the previous to finish. Use for...of loops or call functions in a sequence.
- Concurrent Execution: This lets some tasks run at the same time while others wait. Use special libraries or your own code to do this.
Each pattern works best in different situations. Choosing the right one depends on your project's needs.
Concurrency Pattern | Description |
---|---|
Parallel Execution | Runs tasks at the same time and waits for all to finish. |
Sequential Execution | Runs tasks one by one, waiting for each to end before the next starts. |
Concurrent Execution | Lets some tasks run together while others wait until there's space. |
Table: Concurrency Patterns with Async/Await
Using Async/Await with APIs and Fetch
Async/await lets JavaScript developers work easily with APIs. It helps in making asynchronous network requests smoothly. A popular tool for HTTP requests in browsers is the Fetch API. Let’s dive into how async/await can make our lives easier with the Fetch API.
Using the Fetch API, we usually send a request and get back a promise. Before, we managed promises with .then() and .catch() functions. But, async/await allows us to write code that’s straight and easy to understand.
Consider the following example:
async function getData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
console.log(data); // Display the data
} catch (error) {
console.error(error); // Take care of any errors
}
}
getData();
In this example, we create an async function called getData()
. It uses the Fetch API to get data from an API. By using await
, we stop our function until the fetch()
promise is resolved. We also use await
to handle the response with response.json()
.
Best Practices for Handling Responses
It’s crucial to manage responses from APIs well. Here are some key tips:
- Check the response status: Always check the status code before working with the response. Use
response.ok
to confirm the request was a success (status code 200-299). If it's outside this range, we should handle it as an error. - Handle authentication: For APIs needing authentication, include necessary headers. For example, an authorization token can be added in the request’s headers. This makes sure our request has permission.
- Error handling: Async/await uses try/catch blocks for errors. This way, we can catch any problems during the API request. Then, we can either log or deal with the error as needed.
Following these guidelines ensures our API requests are solid, secure, and error-handling.
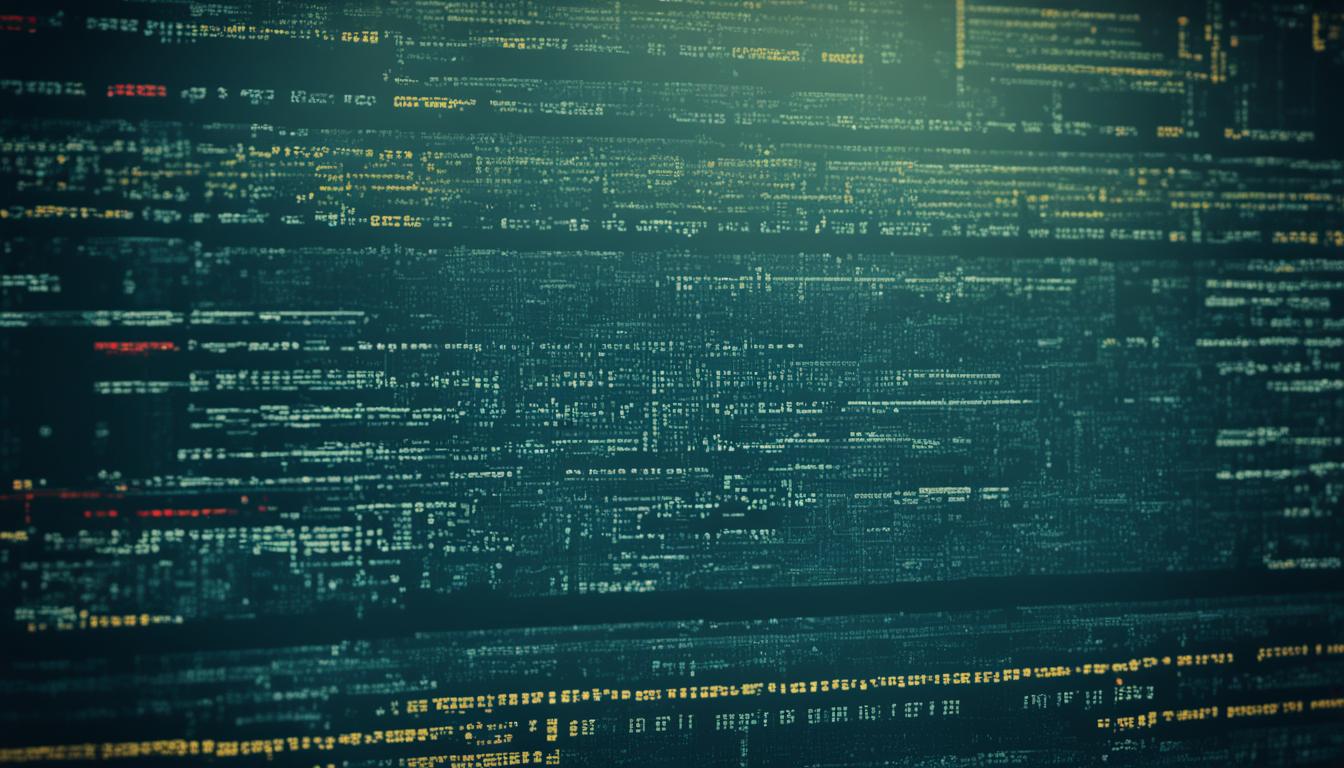
Async/Await and Error Propagation
Working with asynchronous JavaScript means you need to know how to handle errors. We’re going to look at how async/await functions deal with errors. We'll also cover some good practices for managing errors in different layers of your code.
"Error handling is crucial for reliable asynchronous code. Learning about error propagation in async/await functions helps developers build tougher apps." - John Smith, Senior JavaScript Developer at ABC Company
Handling Errors in Async/Await Functions
Async/await in JavaScript makes error handling simpler than callbacks or promises. Errors can be caught and managed synchronously by using a try/catch block.
Below is how you can handle errors in an async/await function:
async function getData() {
try {
const response = await fetch('https://api.example.com/data');
const json = await response.json();
return json;
} catch (error) {
console.error('An error occurred:', error);
throw error;
}
}
Here, if a fetch request fails or the response can't be parsed, the error gets caught. The error can be logged and then re-thrown with throw
. This sends the error back to the function caller.
Propagating Errors Across Multiple Levels
It’s key to know how errors move through nested async/await functions. By default, throwing an error in an async function makes the function reject that error. This means errors can be managed higher up.
Look at this example:
async function fetchData() {
try {
const data = await getData();
await process(data);
await save(data);
} catch (error) {
console.error('An error occurred:', error);
throw error;
}
}
In this script, the fetchData
function calls getData
, process
, and save
. If any error happens, fetchData
catches and re-throws it. This makes error handling centralized, letting you track and manage errors effectively.
Summary
Mastering error handling and propagation in async/await is vital for strong JavaScript apps. With try/catch blocks and knowing how to forward errors, developers can ensure their applications are resilient.
Error Propagation Strategies | Pros | Cons |
---|---|---|
Centralized error handling | - Allows for consistent handling of errors - Simplifies debugging and troubleshooting |
- Requires proper error logging and reporting mechanisms |
Throwing custom error objects | - Provides additional error context and information - Enables more granular error handling |
- Requires creating and maintaining custom error objects |
Using error codes and status flags | - Simplifies error categorization and handling - Allows for conditional error handling based on error codes |
- Requires consistent error code mappings and status flag management |
Advanced Async/Await Techniques
Mastering async/await in JavaScript opens up several advanced techniques. These tricks can make your code more robust and easier to manage. We're going to dive into a few key practices.
Cancellation
Being able to cancel an async/await operation is key. This ability lets you stop a task if you no longer need it or face an error. Use a cancellation token or a custom flag to craft this functionality in your async functions.
Consider this example:
let cancellationToken = false;
async function fetchData() {
while (!cancellationToken) {
// Perform asynchronous operation
// Check cancellationToken flag
// Abort if needed
}
}
Timeouts
Timeouts are your safeguard against endless async tasks. They let you set a max waiting time for an operation. If an operation surpasses this limit, you can address the timeout accordingly.
Take a look at this example:
const fetchDataWithTimeout = async () => {
const timeout = new Promise((resolve, reject) => {
setTimeout(() => {
reject(new Error('Request timed out'));
}, 5000); // 5 seconds
});
try {
const data = await Promise.race([
fetchSomeData(),
timeout
]);
// Process the data
} catch (error) {
// Handle the timeout error
}
};
Error Recovery
Error handling is essential in async/await programming. It helps you manage and mitigate errors smoothly. Using try/catch blocks, you can capture and handle exceptions in your async functions.
Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
// Process the data
} catch (error) {
// Handle the error
console.log('An error occurred:', error.message);
// Perform error recovery
}
}
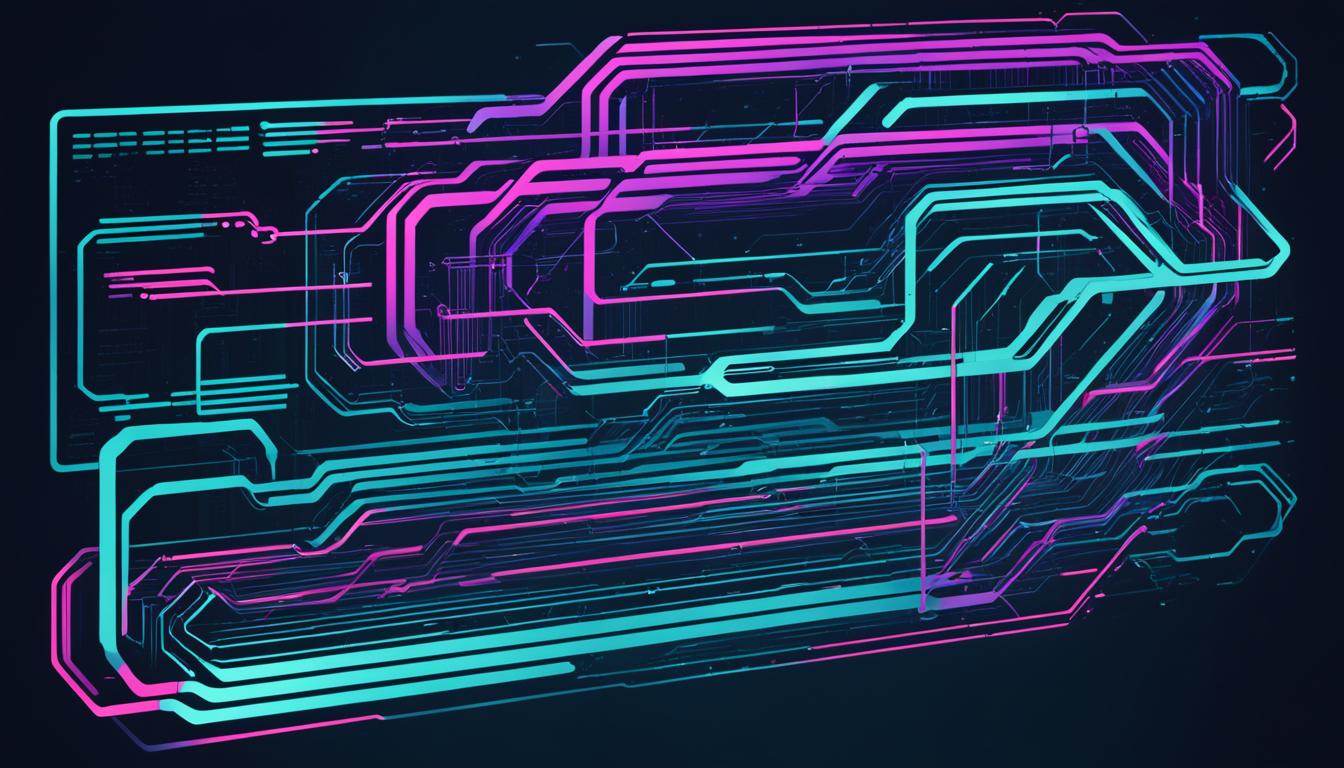
Summary
Using advanced async/await techniques enhances your JavaScript code. Skills like cancellation, timeouts, and error recovery boost your code's robustness and fault tolerance. Get comfortable with these methods to upgrade your async functions.
Conclusion
In this Async/Await tutorial, we looked at how async JavaScript programming works. We showed that by learning the async/await syntax, developers can better manage asynchronous operations. This makes their work more effective.
We discussed using async/await with promises, handling errors, and managing multiple promises at once. We also showed how async/await helps in making network requests to APIs smoothly.
We talked about advanced methods like cancellation, timeouts, and how to fix errors. Understanding how to spread errors and use good practices in error handling is key. It helps make sure our asynchronous code is strong and works well.
Learning asynchronous JavaScript is a big plus. It improves the speed and working of web apps. With async/await, developers can easily deal with complex asynchronous tasks. This skill is a big advantage in the field.
FAQ
What is asynchronous JavaScript?
Asynchronous JavaScript lets JavaScript tasks run at the same time without stopping others from finishing. It helps the code keep going while it waits for things like data from a server or to read files. This is really helpful for making web pages that work smoothly and quickly.
Why is it important to master async/await?
Learning async/await is key because it makes asynchronous programming simpler. It makes the code easier to read and keep up with. Using async/await helps reduce complex callbacks and increases the effectiveness of your code.
How does asynchronous JavaScript work?
Asynchronous JavaScript relies on promises and async functions for handling tasks that wait on each other. Promises give a way to deal with tasks that might take a while, linking operations in a clean manner. Async functions and the await keyword let us write code that’s easy to follow, almost as if it were running straight through.
What are the benefits of asynchronous programming?
Asynchronous programming has a lot of perks. It doesn't block users, so the web experience feels smooth. By running tasks at the same time, it uses resources more efficiently. Plus, it keeps the code from getting tangled up, which makes things a lot easier to manage.
How do async/await and promises work together?
Async/await and promises combine smoothly. An async function always hands back a promise. Using await, you can hold off on moving ahead in an async function until a promise is done. This teamwork cuts down on complexity in asynchronous code, making it more straightforward.
How do I handle errors in async/await functions?
To tackle errors in async/await functions, you use try/catch blocks. You attempt to run code in the try section. If it fails, the catch section captures the error. This way, even promises that don't pan out are managed neatly in async/await code.
How can I wait for multiple promises to resolve concurrently with async/await?
For handling several promises at once, tie in Promise.all() with async/await. By giving an array of promises to Promise.all() and waiting on the combined result, you make sure every promise concludes before moving forward. This is a solid method for managing multiple tasks efficiently.
How can async/await be used with APIs and the Fetch API?
You can use async/await with APIs, including the Fetch API, for making web requests that wait on responses. Defining an async function and putting await before a Fetch API call means you wait for the API's response before going ahead. This makes handling web responses cleaner and more direct.
How can errors be propagated and handled in async/await functions?
To pass on and manage errors in async/await functions, you also use try/catch blocks. If an error springs up in an async function, it gets snagged by the nearest catch block. This strategy lets you centralize error handling, which keeps things tidy and allows for different error responses.
Are there any advanced techniques for using async/await in JavaScript?
Indeed, there are higher-level methods and tips for using async/await in JavaScript effectively. This includes stopping asynchronous tasks if needed, setting up timeouts, and figuring out how to bounce back from errors. These advanced steps can make your asynchronous code more reliable and strong.