Welcome to our comprehensive guide on Array and String methods in JavaScript. This guide is perfect whether you're an experienced developer or just starting. Understanding these methods is key for efficient data handling and coding.
Arrays and strings are key in JavaScript. Knowing how to manipulate them boosts your programming skills. By using these methods, you can make complex tasks simpler, improve performance, and write more effective code.
This article dives into the core concepts and uses of Array and String methods. We will look at everything from simple functions to advanced techniques. This will help you master arrays and strings with ease.
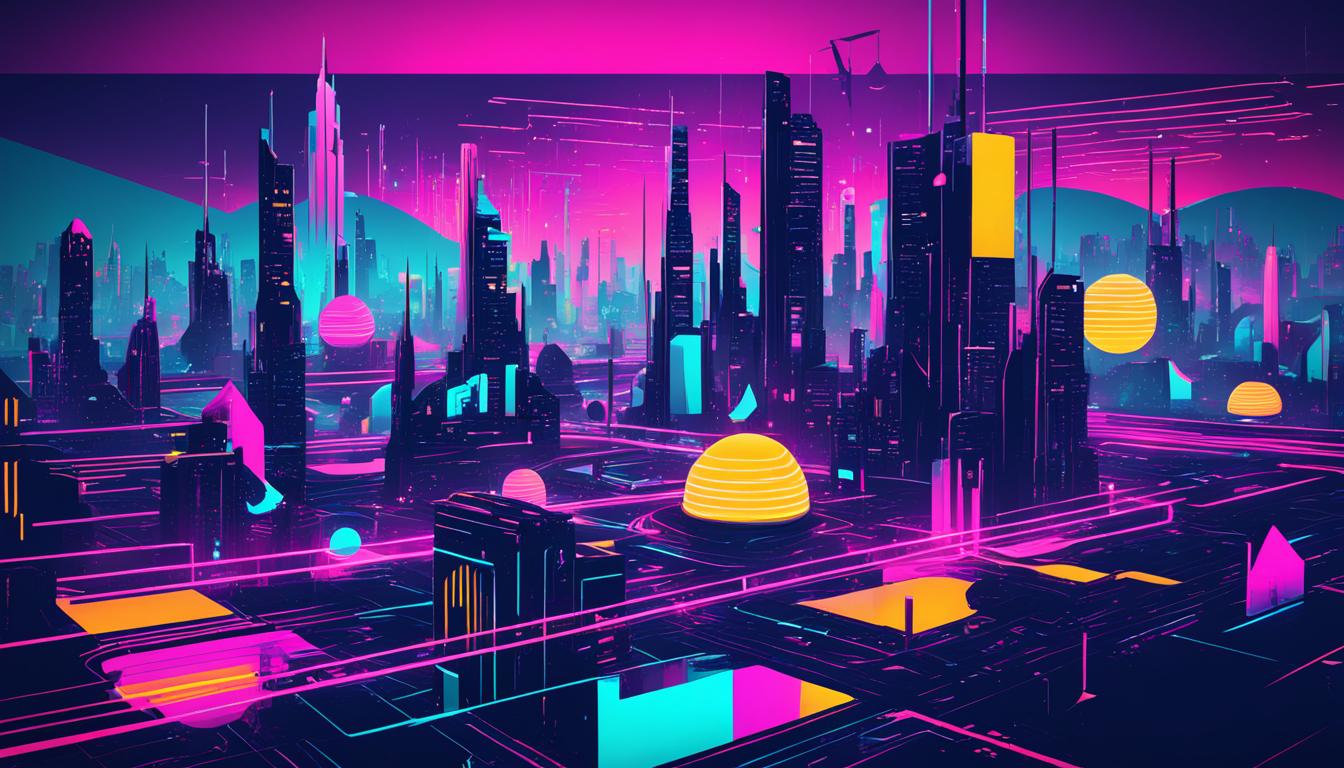
Key Takeaways
- Array and String methods are vital for easy data handling in JavaScript.
- Learning array manipulation improves your efficiency with arrays and strings.
- Studying string functions helps in altering and transforming text effectively.
- JavaScript provides simple methods for common array tasks.
- Becoming proficient in critical string methods boosts your text handling skills.
Understanding Array Manipulation Techniques
Working with arrays in JavaScript is crucial. It's vital to know how to manipulate them. In this section, we will learn about different array functions. This will help us manage arrays and strings well.
Array Functions in JavaScript
JavaScript has many functions for arrays. These functions help with tasks like adding or removing items, sorting, and filtering. Using them makes working with arrays easier and more effective.
Here are some commonly used JavaScript array functions:
- push(): Adds elements to an array's end.
- pop(): Removes the last element from an array.
- splice(): Lets you change an array by adding, removing, or replacing elements.
- concat(): Merges arrays into a new one.
These functions are key to array manipulation in JavaScript. With them, managing arrays and strings becomes straightforward.
Array and String Manipulation Techniques
Manipulating arrays and strings involves tasks like merging, splitting, and filtering. JavaScript offers various ways to do these efficiently.
Let's explore some techniques for arrays and strings:
For transforming arrays, you can use map() and reduce(). They allow changing each element or reducing them to a single value.
To filter arrays, filter() helps. It creates a new array with only the elements that meet a specific condition.
The split() and join() functions are great for working with strings. They divide a string into substrings or merge them into one.
These methods are just a start for array and string handling in JavaScript. Knowing these techniques makes coding more efficient.
Practical Examples and Use Cases
Here are practical examples of array manipulation:
Example | Description |
---|---|
Example 1 | Using the push() function to add new elements to an array. |
Example 2 | Applying the filter() function to select specific elements for a new array. |
Example 3 | Combining strings into a single sentence with join(). |
These examples show how to use array techniques in real situations. Adding these methods to your JavaScript enhances your coding.
With a good grasp of array manipulation techniques, you're set to improve your JavaScript projects. Stay tuned for more on string functions in JavaScript. This will deep dive into string manipulation.
Exploring String Functions in JavaScript
JavaScript string functions let you change and work with text easily. They help you do things like pull out parts of strings, switch out characters, or do more complex tasks. These tools make coding simpler and more effective.
Let's dive into some common string functions in JavaScript:
Basic String Manipulation
For starters, JavaScript lets you join strings, trim extra spaces, and change text case.
Concatenation: The concat()
method lets you merge several strings into one.
Trimming: With the trim()
function, you can get rid of spaces at both ends of a string.
Changing Case: UsetoUpperCase()
andtoLowerCase()
methods to make your text all upper or lower case.
Check out how these basic functions work:
// Concatenation const firstName = "John"; const lastName = "Doe"; const fullName = firstName.concat(" ", lastName); console.log(fullName); // Output: John Doe // Trimming const str = " Hello, World! "; console.log(str.trim()); // Output: Hello, World! // Changing Case const text = "JavaScript is AWESOME!"; console.log(text.toLowerCase()); // Output: javascript is awesome! console.log(text.toUpperCase()); // Output: JAVASCRIPT IS AWESOME!
String Function | Description |
---|---|
concat() |
Combines multiple strings into one. |
trim() |
Removes whitespace from the beginning and end of a string. |
toLowerCase() |
Converts a string to lowercase. |
toUpperCase() |
Converts a string to uppercase. |
Advanced String Operations
JavaScript gives you advanced tools for cutting, replacing, and finding parts of strings, too.
Substring Extraction: You can use the substring()
method to take out part of a string with specific start and end points.
Replacing Text: Change certain words in a string using the replace()
function.
Searching Strings: Find where certain characters or words are withindexOf()
andlastIndexOf()
methods.
Here are examples that show how to use these advanced features:
// Substring Extraction const sentence = "This is a sample sentence."; console.log(sentence.substring(5, 10)); // Output: is a // Replacing Text const textToReplace = "Hello, World!"; const newText = textToReplace.replace("World", "JavaScript"); console.log(newText); // Output: Hello, JavaScript! // Searching Strings const searchStr = "Hello, World!"; console.log(searchStr.indexOf("o")); // Output: 4 console.log(searchStr.lastIndexOf("o")); // Output: 8
String Function | Description |
---|---|
substring() |
Extracts a portion of a string based on specified indices. |
replace() |
Substitutes specific text within a string. |
indexOf() |
Returns the index of the first occurrence of a specified character or substring within a string. |
lastIndexOf() |
Returns the index of the last occurrence of a specified character or substring within a string. |
By learning these functions, you can play with strings in all sorts of ways. This can make your JavaScript coding faster and more fun.
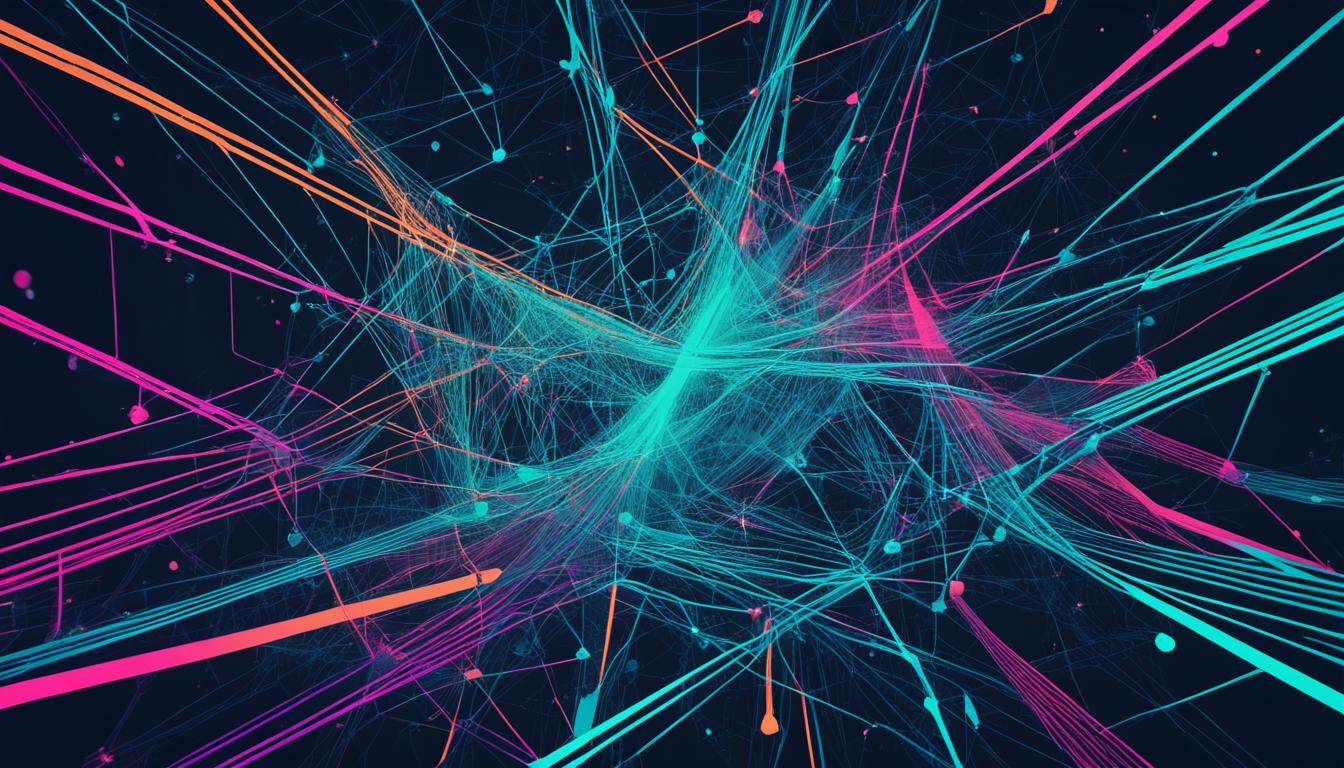
Common Array Operations Made Easy
Working with arrays in JavaScript involves common tasks such as adding or removing elements, sorting, and searching. These key operations simplify your coding tasks. Luckily, JavaScript has handy functions and methods for these actions.
Adding Elements: A typical task is adding elements. You can use the push()
method to add new elements at an array's end. For instance:
let myArray = [1, 2, 3];
myArray.push(4); // Appends 4 to the end of the array
console.log(myArray); // Output: [1, 2, 3, 4]
Removing Elements: For element removal, the pop()
method takes off the last element. Alternatively, splice()
removes elements from specific spots. For example:
let myArray = [1, 2, 3, 4, 5];
myArray.pop(); // Removes the last element (5)
console.log(myArray); // Output: [1, 2, 3, 4]
myArray.splice(2, 1); // Removes one element at index 2
console.log(myArray); // Output: [1, 2, 4]
Sorting: The sort()
method simplifies array sorting. It orders the elements by their Unicode values. You can also sort by custom rules for more specific needs. See this example:
let myArray = [5, 2, 4, 1, 3];
myArray.sort();
console.log(myArray); // Output: [1, 2, 3, 4, 5]
Searching: To find an element's index, use indexOf()
. It returns the element's first index or -1 if not found. Here’s how it works:
let myArray = [1, 2, 3, 4, 5];
let index = myArray.indexOf(3);
console.log(index); // Output: 2
These examples show some of JavaScript's common array functions and methods. Using them, you can manage arrays well in your code.
Essential JavaScript String Methods
In JavaScript, you often work with strings to change character cases, pull out parts of strings, or swap specific words. JavaScript has many string methods to make these tasks easier. Let's learn about these key methods with examples and their practical uses.
Manipulating Character Cases
Changing character cases in strings is a common task. JavaScript has methods to change strings to lower or upper case:
toLowerCase()
: Converts all characters in a string to lowercase.toUpperCase()
: Converts all characters in a string to uppercase.
Here is how you can use them:
const message = "Hello, World!";
const lowercaseMessage = message.toLowerCase();
const uppercaseMessage = message.toUpperCase();
console.log(lowercaseMessage); // "hello, world!"
console.log(uppercaseMessage); // "HELLO, WORLD!"
Extracting Substrings
Extracting parts of strings is also a frequent operation. JavaScript offers two methods for this:
substring(startIndex, endIndex)
: Extracts a substring from a start index to an end index, not including the end index.slice(startIndex, endIndex)
: Likesubstring()
, but can use negative indexes to start from the string's end.
Check out an example of these methods:
const sentence = "JavaScript is amazing!";
const substring1 = sentence.substring(4, 10);
const substring2 = sentence.slice(-8, -1);
console.log(substring1); // "Script"
console.log(substring2); // "amazing"
Replacing Text
Sometimes, you need to replace certain words in strings. For this, JavaScript has the replace()
method. It needs two things: the text to replace and what to replace it with.
Here’s an example:
const sentence = "I love JavaScript!";
const replacedSentence = sentence.replace("JavaScript", "programming");
console.log(replacedSentence); // "I love programming!"
With these essential methods, you can easily change cases, extract parts, or swap words in strings. These are great tools to make your coding more efficient and fun.
Advanced Array Manipulation Techniques
Now that you understand array basics in JavaScript, let's advance. We'll explore complex techniques for managing data structures and sophisticated array operations.
Mapping Arrays
Mapping arrays is a powerful method. It uses a function on every array element, creating a new array of changed values. This is great for modifying or pinpointing certain information.
Imagine you have number arrays and wish to double each. The map()
function can do this:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map(num => num * 2);
Here, map()
doubles each numbers
array item, resulting in doubledNumbers
.
Reducing Arrays
Reducing arrays is another key method. It uses a function to boil down an array to one value. This method is perfect for summing numbers or finding max and min values.
Let's say you want the sum of an array. You'd use reduce()
:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, current) => accumulator + current, 0);
This reduce()
example combines each numbers
value, giving the total sum.
Filtering Arrays
Filtering lets you make a new array with only certain elements. It's handy for extracting specific values or removing undesired ones.
For instance, to keep only even numbers from an array, use filter()
:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(num => num % 2 === 0);
In this case, filter()
selects only even numbers, creating evenNumbers
.
Combining Arrays
Combining arrays merges them into one. This is helpful for pooling data from various sources or adding to an array.
If you want to merge two number arrays, use concat()
:
const numbers1 = [1, 2, 3];
const numbers2 = [4, 5, 6];
const combinedNumbers = numbers1.concat(numbers2);
The concat()
function here merges numbers1
and numbers2
, forming combinedNumbers
.
Mastering these advanced techniques lets you manage and operate on arrays effectively.
Streamlining String Operations with JavaScript
In JavaScript, managing arrays and strings well is key for coding smoothly. This section shows ways to make managing strings easier. This lets you do your work without trouble.
Concatenating strings is a basic task. It combines many strings into one. The concat() method in JavaScript does this. It takes strings as inputs and gives back a new one. Here's how it works:
const string1 = "Hello";
const string2 = "World";
const combinedString = string1.concat(" ", string2);
// Output: "Hello World"
Trimming spaces from strings is another useful task. JavaScript has two tools for this: trim() and trimStart(). trim() cuts spaces from both ends. trimStart() just from the beginning. Check this example:
const text = " Hello World ";
const trimmedText = text.trim();
// Output: "Hello World"
You can also optimize strings with other operations. Like, turning arrays into strings with join(). It merges elements with a separator. This is how you do it:
const fruits = ['Apple', 'Banana', 'Orange'];
const fruitString = fruits.join(', ');
// Output: "Apple, Banana, Orange"
Knowing how to handle strings in JavaScript is very important. By understanding string functions, you can write better code. And your work gets more efficient.
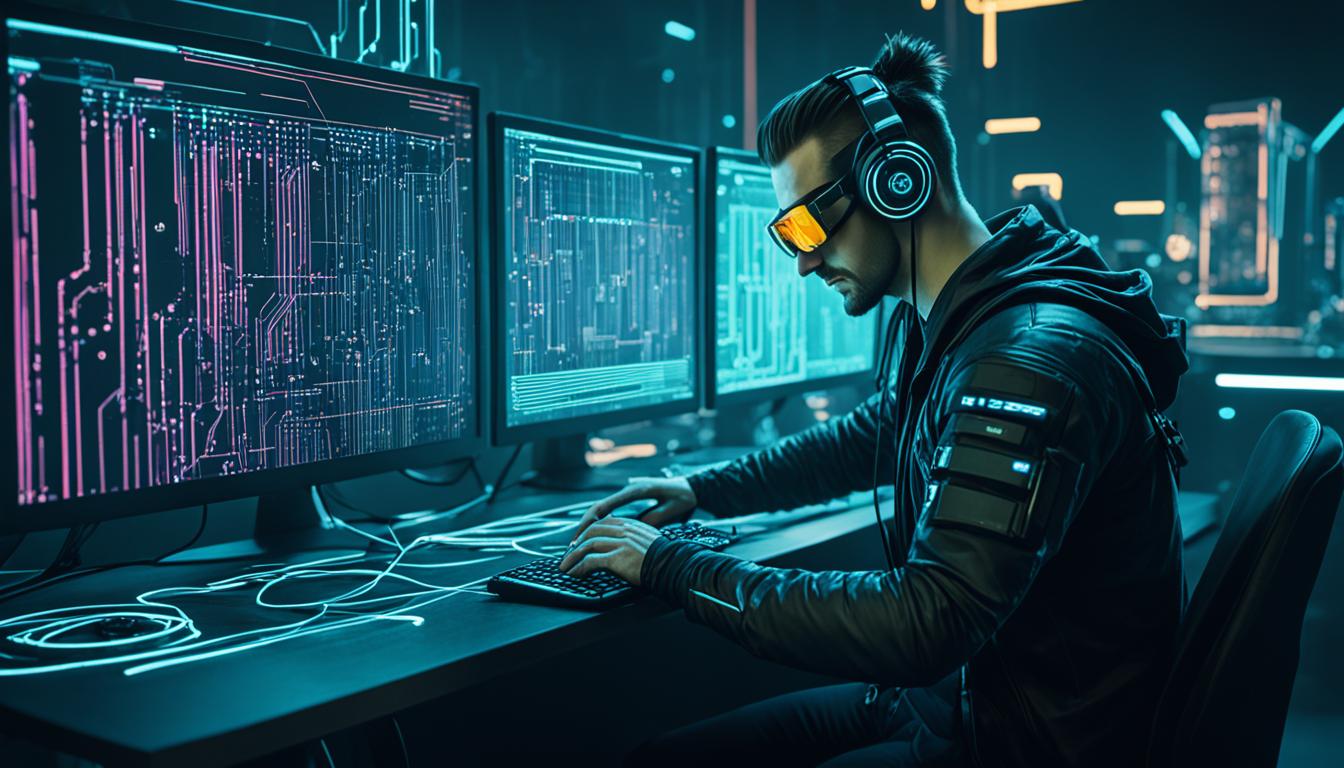
Use JavaScript's string functions and array operations to make coding easier. This improves how you handle data. JavaScript has great tools for merging strings, cutting spaces, or changing arrays to strings.
Mastering Array Iteration Methods
Understanding array iteration methods in JavaScript is key. These include forEach, map, filter, and reduce. They help make working with arrays easier and more efficient.
The forEach method executes a function on every array element. It goes through the array, using the function on each item. This is great for actions needed on every array item without making a new one.
Use the map method to transform each array element into a new array. It changes every item based on a function, giving you a new array. This method is useful when you need to change each item and gather the outcomes.
The filter method creates a new array with only certain elements. You choose which items to keep using a specific function. This is perfect for picking out items that meet certain requirements from an array.
Last, the reduce method turns an array into a single value. Using a function, it combines each item, one by one. This method is good for doing math on array items, like adding them up or finding the biggest number.
Practical Examples of Array Iteration Methods
Let's look at how these methods work with an example:
const numbers = [1, 2, 3, 4, 5];
// Example 1: Using forEach
numbers.forEach((num) => {
console.log(num * 2);
});
Here, the forEach method doubles each number and shows the result. The outcome is:
2
4
6
8
10
With the map method, you can make a new array of doubled numbers:
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // [2, 4, 6, 8, 10]
The filter method lets you get a new array with just the odd numbers:
const oddNumbers = numbers.filter((num) => num % 2 !== 0);
console.log(oddNumbers); // [1, 3, 5]
And using the reduce method, you can add all numbers together:
const sum = numbers.reduce((accumulator, current) => accumulator + current, 0);
console.log(sum); // 15
Comparison of Array Iteration Methods
Method | Description |
---|---|
forEach | Executes a function for each element in the array |
map | Creates a new array by applying a transformation function to each element |
filter | Creates a new array with elements that pass a specified condition |
reduce | Reduces an array to a single value by applying a combining function |
Each method has its own purpose and benefits for array handling. By knowing these methods, you'll be better at using arrays in JavaScript.
Efficient String Manipulation Techniques
In JavaScript programming, knowing how to handle strings well can make your code better. By using the right functions, managing strings becomes simpler and more effective. We'll look at some common methods to make working with strings and arrays easier.
Using slice to Extract Substrings
The slice method helps you get specific parts of a string. It needs a start and an end point. With it, you can pick out parts of a string without changing the whole thing.
Splitting Strings with split
Using split, you can break a string into many pieces with a certain marker. Just input the marker, and you'll have the string in parts. This is handy when you need to work on smaller sections of a string.
Joining Elements with join
The join method lets you combine string arrays into one string. Choose a marker to determine how they merge. This method is great for creating or showing a list of things as one continuous string.
"Techniques like slice, split, and join are key for string handling in JavaScript. They allow developers to easily manage and process strings, boosting code efficiency and effectiveness."
To close, getting good at string manipulation methods helps JavaScript developers work well with strings. The tools slice, split, and join are essential for editing, splitting, and merging string data smoothly.
Method | Description |
---|---|
slice | Extracts a portion of a string based on specified indices. |
split | Divides a string into an array of substrings based on a specified delimiter. |
join | Concatenates elements of an array into a single string using a specified delimiter. |
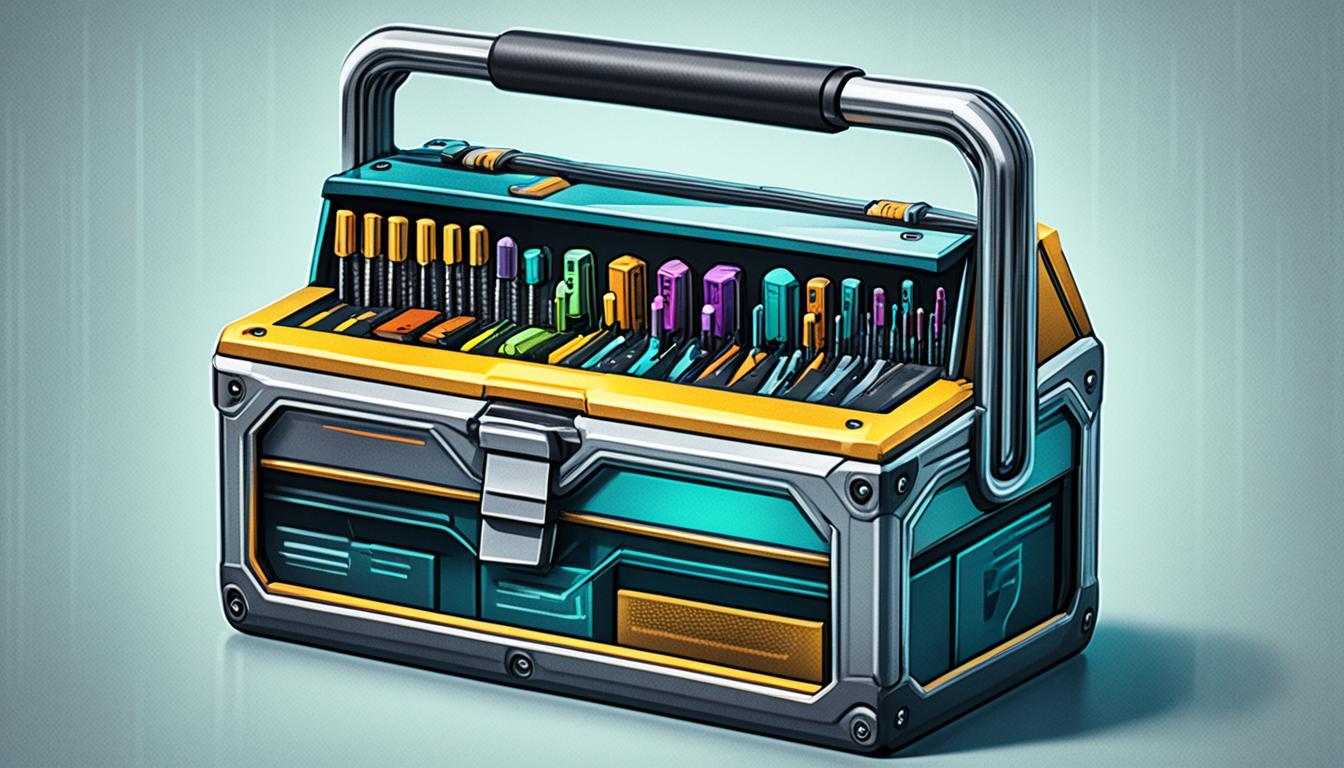
Handling Array and String Methods in JavaScript
When using JavaScript, knowing how to work with arrays and strings is crucial. This means using JavaScript's array and string functions to change them as needed. We'll give you practical examples and tips for using these methods well.
Choosing the Right Methods
Choosing the right functions for arrays and strings is important. JavaScript has many functions for this, each for different needs. Learning these functions helps make your code better and your data handling smoother.
Here are some array functions in JavaScript you should know:
- push(): This function adds new elements to an array's end.
- pop(): It lets you take off and get the last array element.
- splice(): Add or remove elements at any place in an array with this.
- concat(): Combine two or more arrays into one with this function.
Next, let’s look at some string functions in JavaScript:
- toUpperCase(): Changes all letters in a string to uppercase.
- toLowerCase(): Converts all letters in a string to lowercase.
- charAt(): Gets the character at a certain spot in a string.
- replace(): Lets you change certain characters or patterns in a string.
Optimizing Performance
Making your array and string operations perform better is key. Some JavaScript methods work faster and use less memory. Picking the best methods ensures your code runs well.
For arrays, try using map() or reduce() over standard loops for better speed. For strings, try slice() over substring() or substr() for efficiency.
Example of Array and String Methods in Action
Take an array of names. We'll make each name's first letter uppercase:
This uses the map() function to change each name. charAt() and slice() help capitalize the first letter while keeping the rest the same. The result is ["John", "Jane", "Alex"]
.
With the right array and string techniques in JavaScript, you can make coding easier and get better results. Remember to choose the best methods and optimize for a sharper coding edge.
Conclusion
Knowing how to use Array and String methods well is key for good JavaScript coding. By mastering these, developers can handle data better and code faster. This makes them much better at JavaScript.
We looked at a lot of ways to work with arrays and strings in JavaScript. From simple steps to more complex ones, we shared all you need to manage them easily.
Using strong array methods like forEach, map, and reduce makes complex tasks simple. The same goes for string methods like slice, split, and join. They make working with strings easy.
No matter if you're just starting or already know a lot, getting better at these methods will improve your JavaScript skills. So, begin practicing these techniques now and see how much you can do with JavaScript!
FAQ
What are Array methods in JavaScript?
Array methods in JavaScript let developers work with arrays smoothly. You can add, remove, or sort items in an array with these built-in functions. Examples include push, pop, and sort.
How can JavaScript String methods be used?
JavaScript String methods help in editing and handling strings. They include actions like changing case and joining strings. Functions used are toLowerCase, toUpperCase, and concat.
What is the importance of array functions in JavaScript?
Array functions are key for efficient array management in JavaScript. They make adding or finding elements easy. This boosts coding speed and simplifies tasks.
How can I manipulate arrays and strings in JavaScript?
To change arrays in JavaScript, use splice or concat. For strings, toLowerCase and substring are handy. These functions ease handling and modification.
What are some common array and string operations in JavaScript?
Common operations for arrays include sorting and merging. For strings, tasks like altering case and text replacement are usual. These actions are essential for managing data.
What are some practical array and string methods in JavaScript?
Practical array methods like map and filter help with data transformation. String methods such as indexOf assist in finding characters. These improve data handling.
How can I efficiently handle arrays and strings in JavaScript?
Use join to change arrays into strings or split for the opposite. Picking the right method boosts efficiency and saves time.
What are some efficient array and string handling techniques in JavaScript?
For arrays, using map over manual loops saves time. For strings, avoid long concatenations for better speed. These techniques enhance coding efficiency.
What are some advanced array manipulation techniques in JavaScript?
Complex array tasks include mapping to new arrays and combining them. Techniques like filtering based on criteria upgrade data manipulation skills.
How can I streamline string operations with JavaScript?
To streamline string handling, use concat for joining and trim to remove space. These methods quicken string editing.
What are some array iteration methods and how can they be mastered?
Methods like forEach and map assist in array iteration. Mastering them involves practice and understanding their syntax for effective use.
How can I efficiently manipulate strings in JavaScript?
For efficient string manipulation, employ slice to extract parts and join to merge array items. These methods facilitate string adjustments.
How can I handle array and string methods effectively in JavaScript?
Understanding the purpose and function of each method is crucial. Selecting the right method and testing your code ensures success in tasks.