Welcome to the world of AI programming in JavaScript! The async/await syntax changes how we build AI applications. If you're starting an ML project or jumping into AI, knowing async/await is key. It unlocks JavaScript's full power for AI work.
Async programming is a must in AI, helping manage tough, lengthy tasks. With async/await, coders can write code that doesn't block the main thread. This is perfect for AI, dealing with big data, and training models.
This article will cover async/await basics, JavaScript's AI role, and hands-on guides. We're using libraries like TensorFlow.js. Let's dive deep into JavaScript's AI programming power!
Key Takeaways:
- Async/await is crucial for building AI applications using JavaScript.
- Asynchronous programming is essential for handling complex and time-consuming AI tasks.
- JavaScript's versatility makes it an ideal choice for AI development.
- Tutorials on Node.js machine learning and TensorFlow.js will be covered.
- Explore popular AI libraries to enhance your JavaScript AI projects.
Introduction to Building AI with JavaScript
JavaScript is more than a tool for interactive websites. It's also great for artificial intelligence (AI) apps. We'll look at how AI and JavaScript work together and the benefits of JavaScript in AI.
The Benefits of Building AI with JavaScript
JavaScript is popular for web development because it's easy to use, has lots of community support, and many libraries. These perks make it great for AI too.
JavaScript is easy to learn, making AI programming accessible to more people. You don't need to be an expert to start.
JavaScript runs in web browsers, so you don't need complicated setups. AI apps can run online, reaching users everywhere. This is especially good for AI web apps that need to work in real-time.
Principles of AI Programming in JavaScript
Understanding AI programming basics is crucial. It’s about creating algorithms that mimic human intelligence. This lets systems learn, reason, and make decisions.
Using JavaScript, AI programmers apply techniques like machine learning and computer vision. These help systems process data, gain insights, and make predictions.
The Potential of JavaScript in AI
JavaScript's role in AI is growing thanks to new AI tools. Developers can use these to make advanced AI apps with JavaScript.
The rise of Node.js lets developers use JavaScript for server-side tasks. This is great for AI apps that process lots of data or offer AI services.
As JavaScript gets better, its use in AI will grow. Expect more tools that make creating AI apps easier.
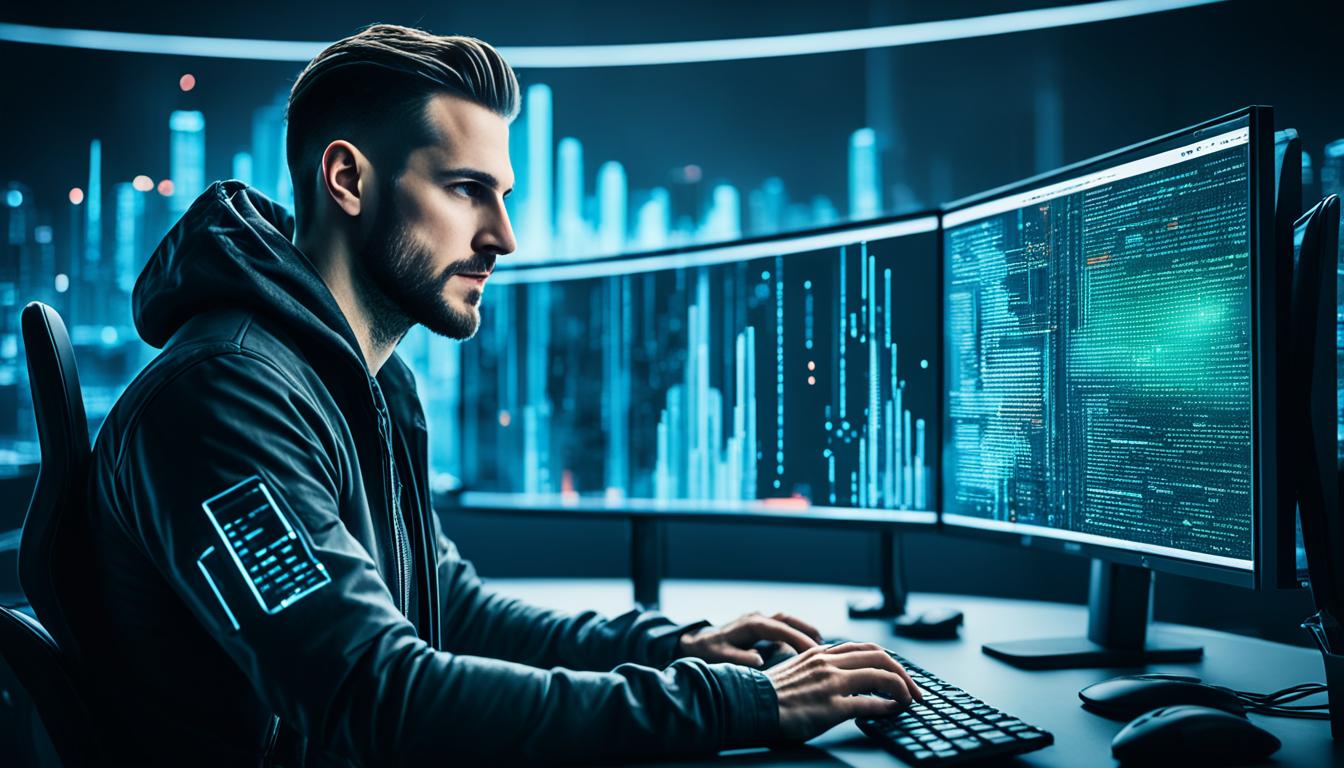
Leveraging Async/Await in JavaScript for AI Projects
The power of async/await in JavaScript greatly helps with AI project development. It makes coding for AI applications easier by using asynchronous programming. Understanding how to use async/await can make a big difference.
Async/await lets developers write code that looks more straightforward but handles asynchronous tasks. You mark a function with "async" to say it does something later, returning a promise. The "await" pauses the function until the promise gets resolved. This makes the code follow a more natural, step-by-step approach.
For AI projects in JavaScript, managing tasks like data fetching, model training, and real-time processing smoothly is crucial. These tasks can be complex and slow. Async/await helps ensure they don’t slow down everything else. This leads to better app performance and responsiveness.
Benefits of Async/Await in AI Development
Using async/await in JavaScript for AI has many benefits:
- Simplified Syntax: It makes asynchronous look like synchronous code, which is easier to read and keep up with.
- Error Handling: By using try/catch with await, developers can catch and manage errors from asynchronous operations easily.
- Better Readability: The code's flow is more straightforward, making it cleaner and easier to maintain.
- Improved Debugging: Debugging is easier as errors are clearly linked to their source await statement.
Consider this example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
// Process the data
}
catch(error) {
console.error('Error fetching data:', error);
}
}
In the code above, fetchData
gets data from an API using fetch
. Async/await keeps the flow clear and errors are well managed. The data is then processed as needed.
Benefits of Async/Await in AI Development | Advantages of Async/Await in JavaScript for AI Projects |
---|---|
Simplified Syntax | Code is easier to read and maintain. |
Error Handling | Errors during asynchronous tasks are caught and managed easily. |
Better Readability | The code's structure is clearer and logic flow is easier. |
Improved Debugging | Tracing and debugging errors become simpler. |
Getting Started with Node.js Machine Learning
Node.js is a powerful JavaScript runtime used for machine learning projects. It combines JavaScript's flexibility with machine learning's abilities, allowing developers to create smart, interactive apps. We will guide you through starting with Node.js for machine learning in JavaScript.
Setting up Node.js
The first step is installing Node.js. Go to the official Node.js website (https://nodejs.org) and download the version for your system. After downloading, follow the instructions to set Node.js up on your machine.
Configuring Machine Learning Libraries
After Node.js is installed, the next step is setting up machine learning libraries. There are many JavaScript ML projects for AI development, such as:
- TensorFlow.js - This library lets developers build and train models in the browser.
- Brain.js - It offers a simple API for neural networks, making model creation and training easy.
- ml5.js - A beginner-friendly library for JavaScript machine learning with pre-trained models and simple APIs.
These libraries can be installed via npm or included in your HTML with a script tag. Always follow each library's documentation for correct installation or inclusion.
Building AI with Node.js
With Node.js and the needed libraries set up, you're ready to build AI apps. Node.js lets you run JavaScript code outside the browser, which is perfect for AI development on the server side.
"Node.js's non-blocking, event-driven architecture is efficient for concurrent requests. It's excellent for AI projects that deal with lots of data."
This setup allows you to create AI applications capable of complex tasks. They can process natural language, recognize images, and perform predictive analytics.
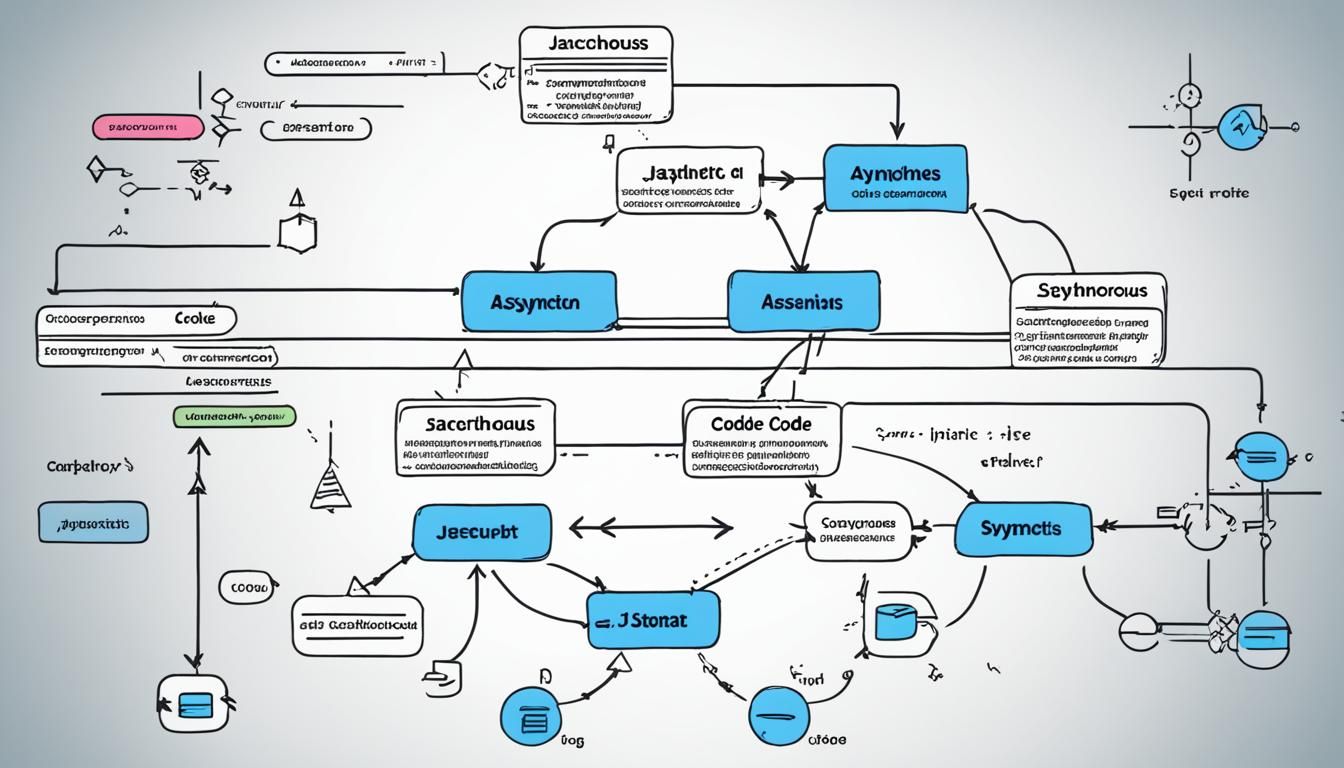
Image: A graphic showing Node.js in machine learning. It highlights how JavaScript and AI development work together seamlessly.
Next, we'll look at different JavaScript AI libraries. We'll cover their features, advantages, and how they're used in AI development.
Exploring JavaScript AI Libraries
Developers have many options for building AI with JavaScript. They can use JavaScript AI libraries for creating smart applications. TensorFlow.js is one of the most used libraries.
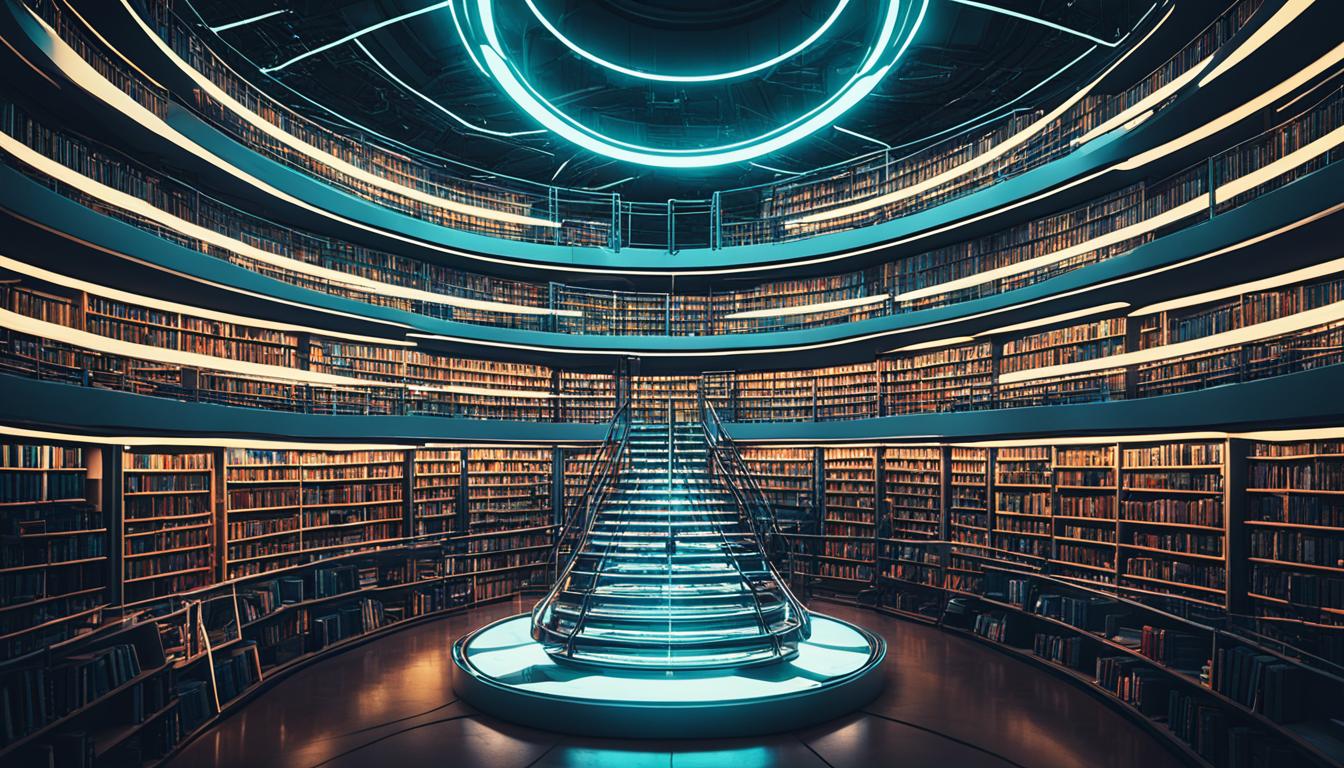
TensorFlow.js is an open-source library for developing machine learning models in the browser or on Node.js. It's easy to use and supports image and text classification, among other tasks.
With TensorFlow.js, developers can work with pre-trained models or create their own. It also has tools for data processing and model visualization. This makes adding AI features to JavaScript apps much easier.
"TensorFlow.js is changing the game in JavaScript AI development. Its versatility and user-friendliness make it perfect for developers who want to use machine learning in their JavaScript projects."
There are other JavaScript AI libraries to check out:
- Brain.js: This library works well for neural networks and can handle different learning tasks.
- Deeplearn.js: Made by Google, it offers deep learning operations that are very fast, thanks to GPU acceleration.
- ConvNetJS: It's a simple library for deep learning that supports many network types. It's also light and works in browsers.
- Synaptic.js: This library is great for creating neural networks for pattern recognition and predicting time series.
Each library offers unique benefits, letting developers pick the best one for their AI projects.
JavaScript AI Libraries Comparison
Here’s a table comparing these libraries:
Library | Features | Advantages | Use Cases |
---|---|---|---|
TensorFlow.js | Built-in support for training and deploying models, pre-trained models, visualization utilities | Well-documented, community support, easy integration with JavaScript applications | Image and text classification, object detection, natural language processing |
Brain.js | Simple API, flexibility, supports neural networks, both supervised and unsupervised learning | Lightweight, runs efficiently in the browser, good for small to medium-sized datasets | Pattern recognition, time series analysis, regression |
Deeplearn.js | Low-level GPU-accelerated operations, high performance, optimized for deep learning | Powerful toolset for performance-critical tasks, integration with TensorFlow ecosystem | Deep learning models, computer vision, natural language processing |
ConvNetJS | Support for various neural network architectures, simplicity, browser compatibility | Lightweight, easy to learn and use, good for educational purposes | Image recognition, object detection, reinforcement learning |
Synaptic.js | Intuitive API, supports various neural network types and architectures | Lightweight, runs efficiently in the browser, fast prototyping | Pattern recognition, time series prediction, optimization problems |
Deep Learning in JavaScript with TensorFlow.js
TensorFlow.js is a leading tool for creating AI models in JavaScript. It brings TensorFlow's power, a top deep learning library, into the JavaScript world. This lets developers use deep learning algorithms in web browsers or Node.js.
With TensorFlow.js, deep learning in JavaScript is more accessible. It opens up the field to web developers and AI fans. You can now use deep learning in your JavaScript projects without needing to change languages or tools.
Starting with TensorFlow.js to make deep learning models is both fun and educational. It suits all, from newbies to pros. TensorFlow.js has an easy-to-use interface and great guides to help you start fast.
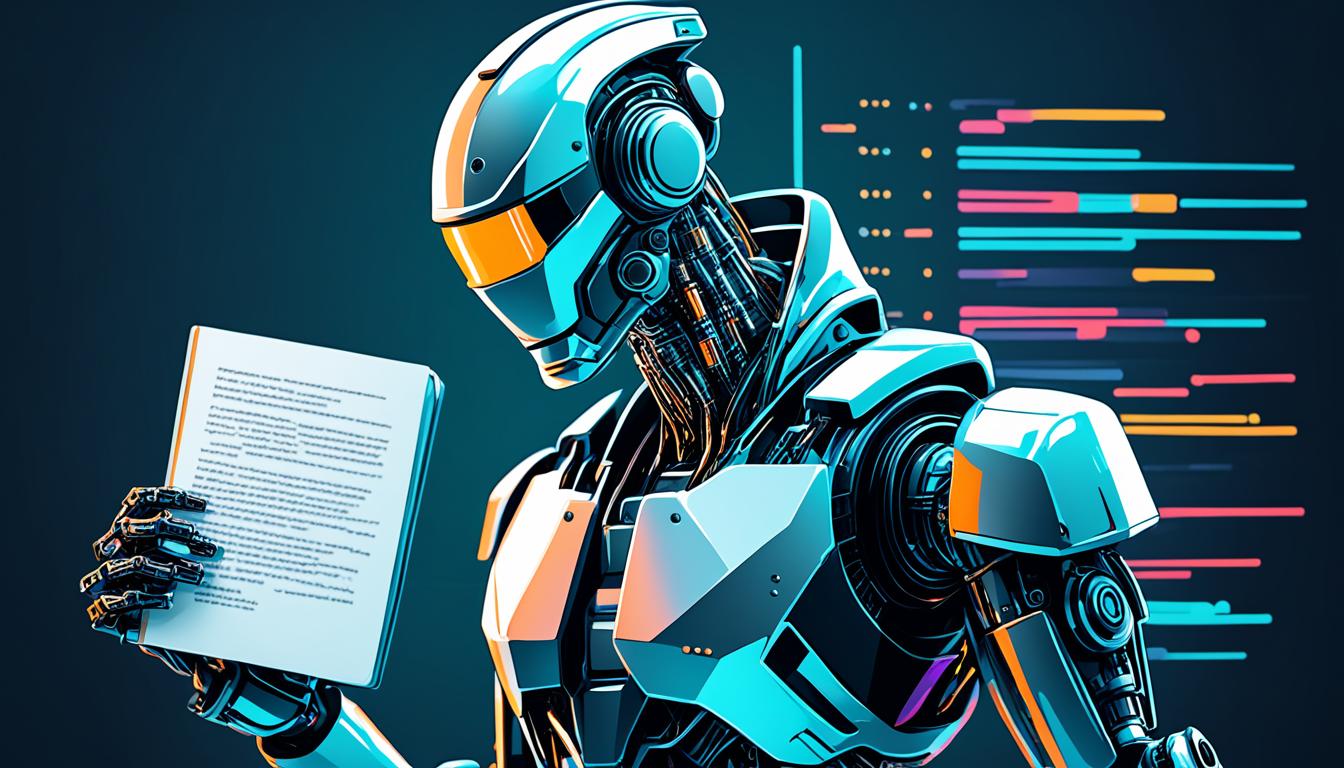
Getting started with TensorFlow.js
Starting with TensorFlow.js begins with installing the library. Check the TensorFlow.js website for how to set it up in browsers or Node.js.
After installation, you're ready to create deep learning models in JavaScript. TensorFlow.js has lots of ready-to-use models. These include tools for image recognizing, understanding feelings in text, and processing languages. It also lets you train your models, offering everything you need for building, data handling, and fine-tuning.
Building a deep learning model
We'll see how to build a model that tells cats from dogs:
- Load and preprocess the dataset of cat and dog images.
- Create a model's architecture with TensorFlow.js layers.
- Train your model with this dataset.
- Check how well your model does.
- Finally, use it to predict new images.
These steps help you build an image classifying model in JavaScript. TensorFlow.js's detailed docs and examples guide you through, making it easy for beginners.
Advantages of deep learning in JavaScript with TensorFlow.js
Deep learning with TensorFlow.js in JavaScript has many plus points:
- Accessibility: Many developers use JavaScript, and TensorFlow.js makes deep learning open to them.
- Flexibility: You can work in browsers or Node.js thanks to TensorFlow.js. This adds versatility to where and how you build models.
- Integration: It fits well with other JavaScript tools, letting you mix deep learning with web tech you already use.
- Performance: By using the GPU, TensorFlow.js speeds up deep learning, making models learn and predict quicker.
TensorFlow.js brings AI's power to you, allowing remarkable apps that use machine learning. Whether in a browser or server-side, it unlocks AI's potential.
Advantages | Disadvantages |
---|---|
Accessibility to a large developer community | Limited performance compared to native languages |
Flexible development environment | Depending on browsers or Node.js to run |
Integration with existing JavaScript libraries and frameworks | Issues might occur with old JavaScript versions |
Uses GPU for quicker deep learning tasks | Heavy models might need more optimization |
Challenges and Limitations of JavaScript in AI Development
JavaScript is popular for its ease of use and versatility. But, it faces hurdles in AI development. Developers may find performance issues and a lack of AI-specific features challenging. Yet, these can be tackled with the right strategies.
Performance Limitations
When it comes to AI, JavaScript's speed can be a problem. It's because JavaScript is interpreted, not compiled like Python. This makes it slower for tasks like training complex AI models.
Also, JavaScript can't easily do parallel processing due to being single-threaded. Web workers help, but they might not match the performance of AI-designed languages.
Limited AI-specific Features
JavaScript lacks some AI-specific features. It has tools like TensorFlow.js but they may not be as powerful as those in AI-focused languages.
Challenges also arise when working with special hardware like GPUs. This makes JavaScript less suitable for advanced AI projects than languages such as Python.
Potential Workarounds
Developers can overcome JavaScript's limits in AI with certain approaches:
- Code Optimization: Optimizing code can boost performance in AI tasks. Using efficient algorithms and managing memory well are key.
- Integration with Other Languages: Combining JavaScript with AI-specific languages can be effective. For instance, using Node.js to connect with Python AI libraries.
- Exploring Alternatives: For demanding AI projects, looking into other languages like Python might be better. Python's AI libraries offer more features.
Assess your AI project's needs carefully before choosing JavaScript. By playing to JavaScript's strengths and using workarounds, meaningful AI apps can still be made.
In summary, JavaScript faces challenges in AI but it's still useful. Developers can navigate these challenges with proper strategies and make the most of JavaScript in AI.
Exploring AI Applications with JavaScript
JavaScript has grown beyond web development to power AI solutions. Its flexibility makes it a top choice for AI projects in many fields.
Natural language processing (NLP) is one area where JavaScript shines. Tools like Natural and Brain.js let developers create chatbots and virtual assistants. These systems can understand and reply to human language, changing how companies interact with customers.
JavaScript is also key in computer vision. With TensorFlow.js and OpenCV.js, developers can build advanced image and facial recognition systems. This opens the door to exciting uses in healthcare, entertainment, and security.
Recommendation systems use JavaScript to offer personalized suggestions on websites and apps. These AI algorithms study user habits to make accurate recommendations. This technology is reshaping e-commerce and digital entertainment.
In the financial sector, AI and JavaScript work together for fraud detection and risk management. They help spot suspicious behaviors, protecting users from fraud. This combo is crucial for keeping financial transactions safe.
Healthcare benefits from JavaScript-powered AI too. It's used in disease prediction, medical image analysis, and aiding clinical decisions. This technology is improving diagnostics, saving time, and enhancing patient care.
HTML coding is being revolutionized by JavaScript's AI abilities. Developers can automate HTML code creation, simplifying web design and enabling quick prototyping.
JavaScript's AI applications are transforming many industries and tackling complex challenges. With a rich ecosystem of libraries, the possibilities for AI-powered innovations are endless. As developers explore more uses, we'll see new ways AI and JavaScript change our world.
Industry | AI Application |
---|---|
Customer Service | AI chatbots and virtual assistants |
Computer Vision | Image recognition systems and facial recognition technologies |
Personalization | Recommendation systems for e-commerce and entertainment |
Finance | Fraud detection and risk assessment |
Healthcare | Predictive disease analysis and medical image analysis |
Web Development | Automated HTML code generation |
Future Trends in AI Development with JavaScript
AI is reshaping technology's future, with JavaScript becoming a key player. This combo lets developers create cutting-edge AI solutions. Let's dive into the exciting trends in AI development using JavaScript.
1. Integration of JavaScript with Big Data
JavaScript and big data are joining forces. As we generate more data, analyzing it is key for AI. JavaScript helps developers use big data for smarter AI applications.
2. Expansion of Natural Language Processing
JavaScript is crucial for natural language processing (NLP) growth. It supports building apps that understand human language. This means better chatbots and voice assistants are on the way.
3. Advancements in Neural Networks and Deep Learning
Neural networks are pushing AI forward. Thanks to libraries like TensorFlow.js, developers can build complex models in browsers. This speeds up AI app development and deployment.
4. Cross-platform Development with JavaScript
JavaScript is making AI apps work across devices. Tools like Electron.js and React Native let developers use one codebase for all platforms. This makes AI apps more accessible.
5. Integration with IoT and Edge Computing
JavaScript fits perfectly with the growing IoT world. It's great for AI in IoT devices. Node.js, for example, helps devices make smart decisions fast.
"The future of AI development with JavaScript is full of immense possibilities. With the right tools, frameworks, and advancements, developers can propel AI applications to new heights, transforming industries and improving the way we interact with technology."
In conclusion, AI development with JavaScript is on a promising path. With its help, developers will revolutionize industries by building smarter applications.
Conclusion
The journey of crafting AI with JavaScript has been thrilling. In this article, we've delved into JavaScript's strength in AI creation. We noted the importance of grasping asynchronous JavaScript for making efficient AI tools.
JavaScript's adaptability lets developers forge AI projects that truly make a difference. We discussed using Node.js for machine learning and TensorFlow.js. This shows JavaScript is a robust base for AI solutions.
While AI and JavaScript are growing together, they face hurdles. Yet, with knowledge and the right tactics, developers can surpass these issues. If you're just starting or already know your way around, diving into AI with JavaScript is filled with endless opportunities.
FAQ
Can JavaScript be used for building AI applications?
Yes, you can use JavaScript for AI. It has many libraries and frameworks. These help developers use machine learning and deep learning in their projects.
What are the advantages of using JavaScript for AI programming?
JavaScript is easy to learn, which makes it great for all developers. It has a strong community, lots of resources, and works on the client and server side.
What is the significance of async/await in JavaScript for AI development?
Async/await makes handling asynchronous programming easier. In AI, it helps manage complex data and computations. It makes AI applications run smoother and respond faster.
How can Node.js be leveraged for machine learning projects in JavaScript?
Node.js lets developers run server-side code for machine learning. They can use libraries, process data, and build AI on the backend with it.
What are some popular JavaScript AI libraries?
TensorFlow.js, Brain.js, and ml5.js are top JavaScript AI libraries. They help with machine learning, neural networks, and deep learning in JavaScript.
How can TensorFlow.js be used for deep learning in JavaScript?
TensorFlow.js brings deep learning to JavaScript. Developers can build and train models in the browser. It has a simple API for neural networks and works with CPU or GPU.
What are the challenges of using JavaScript for AI development?
The main issues are performance, missing AI features, and working with other languages. Yet, developers can solve these problems through optimization, picking the right libraries, and cross-language communication.
What are some AI applications that can be built with JavaScript?
With JavaScript, you can make chatbots, recommendation engines, sentiment analysis, image recognition, and NLP tools.
What does the future hold for AI development with JavaScript?
AI in JavaScript has a bright future. As the language adds new features and libraries, its AI potential will grow. This will lead to more innovation in AI.