In this article, we investigate ES6 methods and their impact on coding. We'll cover the new features of ES6, also known as ECMAScript 6. You'll learn how these can make your code more efficient and clean.
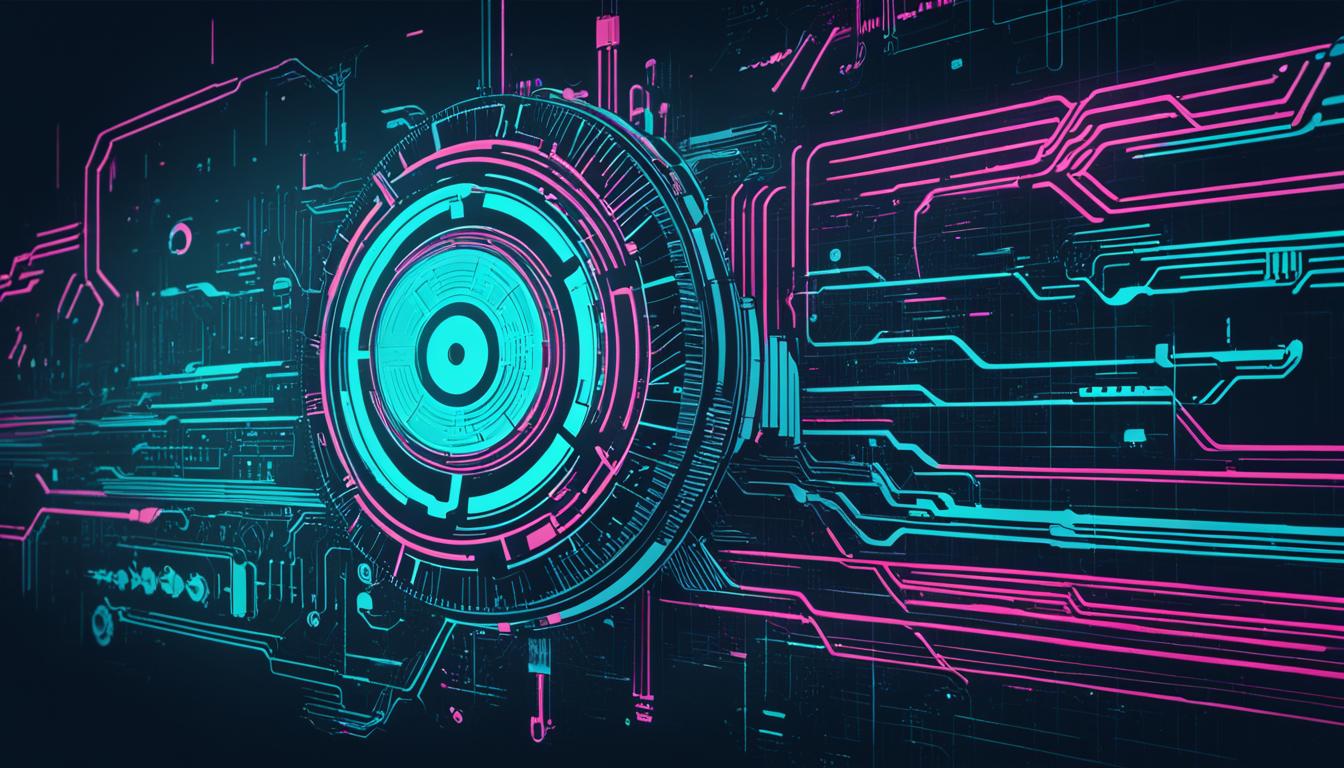
Key Takeaways:
- ES6 methods bring modern features and improvements to JavaScript programming.
- Understanding ES6 features is crucial for leveraging the full potential of ES6 methods.
- ES6 methods can streamline code, enhance readability, and simplify working with complex data structures.
- Array, object, and string manipulation are made easier with ES6 methods.
- ES6 methods enhance asynchronous programming and offer convenient ways to iterate and loop through data.
An Introduction to ES6 Methods
ES6, or ECMAScript 6, started a new chapter in coding with its advanced features. For any JavaScript developer, knowing ES6 methods is key to getting better. We'll look into what these methods are and their benefits compared to old JavaScript. This will help you use ES6 to its full potential in your work.
With its major update, ES6 made JavaScript better by adding features that make coding more efficient and clear. These improvements let developers write short and powerful code. This boosts their work and makes programs better. ES6 methods offer special functions that simplify coding tasks.
Old JavaScript often needed long and intricate code for tasks, but ES6 methods make it easier. They provide ready-to-use functions. This cuts down on unnecessary code, making programs neater and simpler to handle.
Benefits of ES6 Methods
- Improved code readability and maintainability
- Reduced boilerplate code and increased productivity
- Streamlined programming tasks with built-in functions
- Enhanced programming capabilities and expressiveness
Using ES6 methods boosts your JavaScript skills. Next, we'll go deeper into ES6's special features. We'll see how they can further improve your coding. Stay tuned!
Understanding ES6 Features
To really use ES6 methods well, you need to know its main features. ECMAScript 6 (ES6) updated JavaScript in big ways. We'll look at arrow functions, template literals, and destructuring assignments, some of ES6's coolest parts.
Arrow Functions
Arrow functions are a big deal in ES6. They let you write smoother and more direct code, making coding in JavaScript more fun. They have a simpler syntax, cut down on extra function bindings, and keep the 'this' keyword straightforward.
"Arrow functions allow you to write cleaner code and avoid common pitfalls associated with regular function expressions."
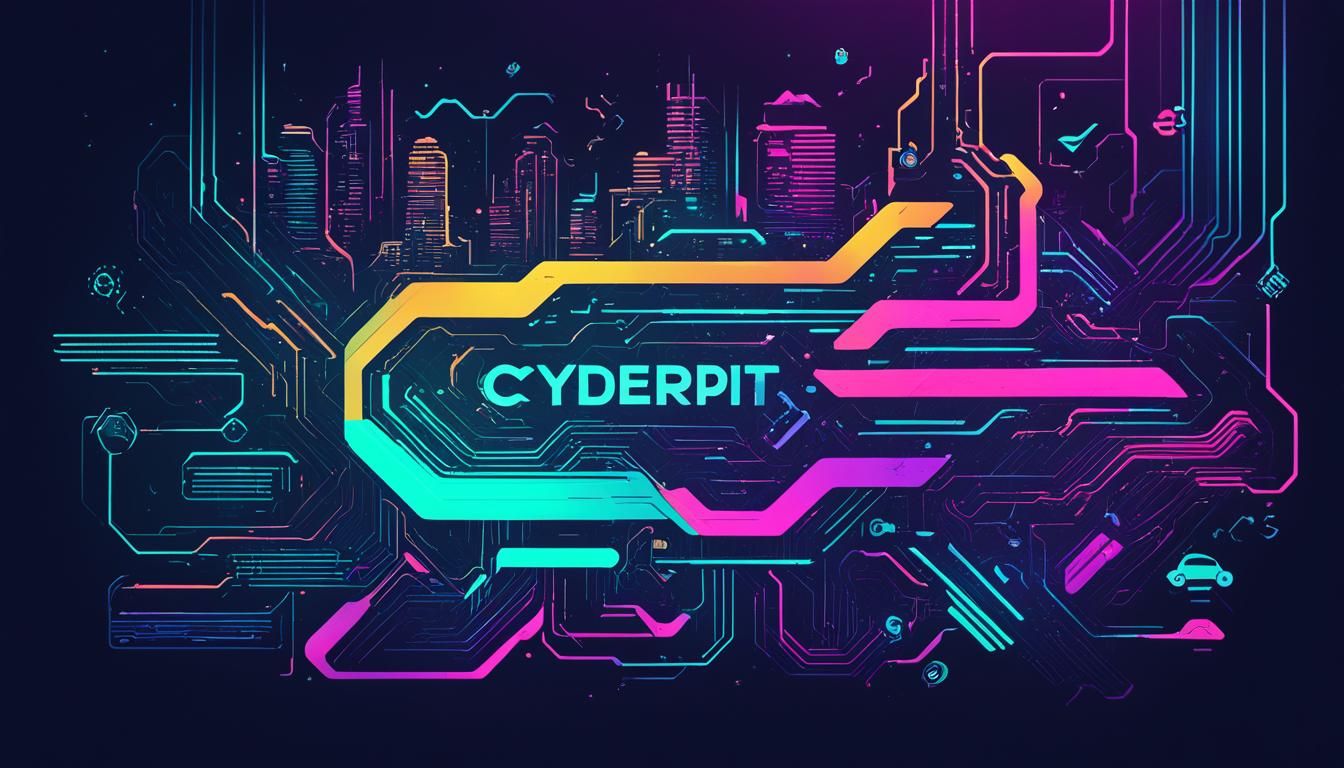
Template Literals
ES6's template literals also make life easier. They let you combine strings and put expressions right inside them. You use backticks instead of quotes, with placeholders ${} for putting expressions directly into strings.
"Template literals make string interpolation and multiline strings effortless and intuitive."
Destructuring Assignments
Destructuring assignments are another smart feature of ES6. They let you pull out values from arrays or objects and put them into variables neatly. This makes dealing with complex data much easier.
"Destructuring assignments provide a more efficient and readable way to extract values from arrays and objects."
ES6 Features Comparison Table
Feature | Description |
---|---|
Arrow Functions | Provide a concise syntax for defining functions. |
Template Literals | Allow for easier string interpolation and multiline strings. |
Destructuring Assignments | Simplify the process of extracting values from arrays and objects. |
Getting Started with ES6 Methods
We're now ready to jump into ES6 methods. This part will show you how to add ES6 methods into your JavaScript work. We will go through it step by step.
ES6 methods bring new powerful features that make your code better. Using them means your code will be cleaner and more efficient. Plus, you stay up-to-date with JavaScript's latest.
If you're new to ES6, don't worry. This section is for you. It teaches how to use ES6 methods in your projects well. You'll learn to make programming tasks easier, make your code easier to read, and work more productively.
Starting with ES6 methods means knowing what methods are there and how they are used. This is key to using the right method at the right time. And it brings out the best in your work.
Here, you'll find guides that take you through using ES6 methods step by step. Each guide gives clear instructions and examples. This helps you understand the ideas and use them in your projects.
By the end of this, you'll be skilled in ES6 methods. You'll be set to add them to your JavaScript coding. Let's begin this exciting journey into the world of ES6 methods!
Array Manipulation with ES6 Methods
ES6 methods have changed the way we handle arrays in JavaScript. They offer powerful tools that make your code cleaner and more expressive. With these methods, you can easily do things like iterate, filter, transform, and reduce arrays. This lets you tackle complex tasks with less effort.
The map method is a key feature of ES6 for array manipulation. It lets you create a new array by changing each item in the original array with a function. This gets rid of the need for for loops and makes it easy to apply a function to every array item. The map method is great for changing array values without altering the original array.
Another handy ES6 method is filter. It helps you make a new array that only contains items that meet a certain condition. With filter, you can quickly remove items you don't want or pick out items that fit specific requirements. This method makes your code easier to read and simplifies complex loops and conditions.
ES6 also gives us the reduce method, which turns an array into a single value. It goes through the array and applies a function to each item, adding up the results. This is super useful for doing things like adding up all the items in an array or finding the biggest item. The reduce method makes complex array tasks much simpler.
To help you understand these methods better, let's look at an example:
const numbers = [1, 2, 3, 4, 5];
// Using the map method to Square each numberconst squaredNumbers = numbers.map(num => num * num);
// Output: [1, 4, 9, 16, 25]
// Using the filter method to filter even numbersconst evenNumbers = numbers.filter(num => num % 2 === 0);
// Output: [2, 4]
// Using the reduce method to find the sum of numbersconst sum = numbers.reduce((acc, num) => acc + num, 0);
// Output: 15
This example shows that ES6 methods provide simple and elegant ways to handle common array tasks. By using these modern JavaScript methods, you can make your code more efficient and expressive. This saves you time and effort in coding.
Now you know how to manipulate arrays with ES6 methods. Next, we'll explore how to work with objects in the following section.
Object Manipulation with ES6 Methods
ES6 has changed the way we program in JavaScript by adding new methods for tampering with objects. These methods include Object.keys, Object.values, and Object.entries. They let developers handle complex data more easily.
Object.keys:
Object.keys helps you pull out an object's keys, giving back a list of its property names. This is super useful for when you have to go through an object or do stuff based on its keys. Here's how it works:
const person = { name: 'Alice', age: 30, profession: 'Engineer'};const keys = Object.keys(person);// Output: ['name', 'age', 'profession']
Object.values:
Object.values operates similarly to Object.keys but it fetches the object's values instead, returning them in a list. It’s great for when you want to work with an object’s values. Check this out:
const person = { name: 'Alice', age: 30, profession: 'Engineer'};const values = Object.values(person);// Output: ['Alice', 30, 'Engineer']
Object.entries:
Object.entries turns an object into a list of key-value pairs, letting you access keys and values at the same time. Here’s an example of how it’s used:
const person = { name: 'Alice', age: 30, profession: 'Engineer'};const entries = Object.entries(person);// Output: [['name', 'Alice'], ['age', 30], ['profession', 'Engineer']]
With these ES6 tools, managing objects becomes a breeze. You can easily grab the info you need and make your code cleaner. These methods support a range of tasks, from iterating over properties to accessing specific ones for complex operations.
Benefits of Object Manipulation with ES6 Methods
- Makes handling objects simpler
- Offers effective ways to gather keys, values, or both from an object
- Improves how your code looks and its upkeep
- Helps with running loop operations on objects
String Manipulation with ES6 Methods
String manipulation is key in JavaScript. It's essential for creating interactive web applications. ES6 brings new, efficient methods to handle strings better.
We'll look at how ES6 improves string manipulation. Time to discover the most helpful ES6 methods for strings:
1. startsWith
The startsWith
method checks if a string begins with certain characters. It returns true or false. It's great for validating or filtering strings.
2. endsWith
endsWith
does the opposite of startsWith
. It checks if a string ends with certain characters. This is useful for various string checks.
3. includes
includes
lets you see if a string has a specific substring. It returns true if the substring is there. This method is perfect for finding or using certain parts of strings.
ES6 methods have changed how we work with strings. They offer simpler, more effective ways to manage strings. Using these methods, your code becomes clearer and your work, faster.
Let's check out how to use these methods in real life:
Scenario | Code Example |
---|---|
Checking if a URL starts with "https://" | const url = "https://www.example.com";
console.log(url.startsWith("https://")); // Output: true |
Checking if a file name ends with ".txt" | const fileName = "example.txt";
console.log(fileName.endsWith(".txt")); // Output: true |
Searching for a keyword in a text | const text = "The quick brown fox jumps over the lazy dog.";
console.log(text.includes("fox")); // Output: true |
These examples show a few ways ES6 string methods can be used. Mix and match methods for more powerful string handling.
Try these methods in your projects. The more you use them, the better you'll get. They can really boost your JavaScript skills.
With a good grasp of ES6 string methods, explore how they help with asynchronous programming next.
Enhancing Asynchronous Programming with ES6 Methods
In today's web development, asynchronous programming is key. It lets us handle long tasks, like API calls, efficiently. JavaScript ES6 has introduced methods that make working with asynchronous tasks easier. They help developers manage and understand their code better. We'll look at three ES6 methods: async/await, Promise.all, and Promise.race.
Async/Await: Simplifying Asynchronous Workflows
The async/await syntax in JavaScript ES6 is a big leap forward. It makes writing asynchronous code feel similar to synchronous code. This syntax improves how we handle promises. It clears up code, making it simpler to use and maintain. With async, a function awaits tasks, pausing until a promise is resolved.
Example:
async function fetchData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.log('Error:', error);
}
}
Promise.all: Handling Multiple Promises
The Promise.all method is great for managing several tasks at once. It processes an array of promises and gives back a single promise. This single promise resolves when all in the array do. This method allows tasks to run at the same time, saving time and boosting performance.
Example:
const promise1 = fetch('https://api.example.com/data1');
const promise2 = fetch('https://api.example.com/data2');
Promise.all([promise1, promise2])
.then(responses => {
const data1 = responses[0].json();
const data2 = responses[1].json();
// Process the returned data
})
.catch(error => console.log('Error:', error));
Promise.race: Handling the Fastest Promise
Sometimes, we need to act on the first task that finishes. That's what Promise.race does. It looks at an array of promises and completes when the first one does. Whether it resolves or rejects first, it gets handled right away.
Example:
const promise1 = fetch('https://api.example.com/data1');
const promise2 = fetch('https://api.example.com/data2');
Promise.race([promise1, promise2])
.then(response => response.json())
.then(data => {
// Process the fastest returned data
})
.catch(error => console.log('Error:', error));
Using async/await, Promise.all, and Promise.race, JavaScript developers can write cleaner, more efficient code. These ES6 methods streamline complicated tasks, making code easier to read and boosting productivity.
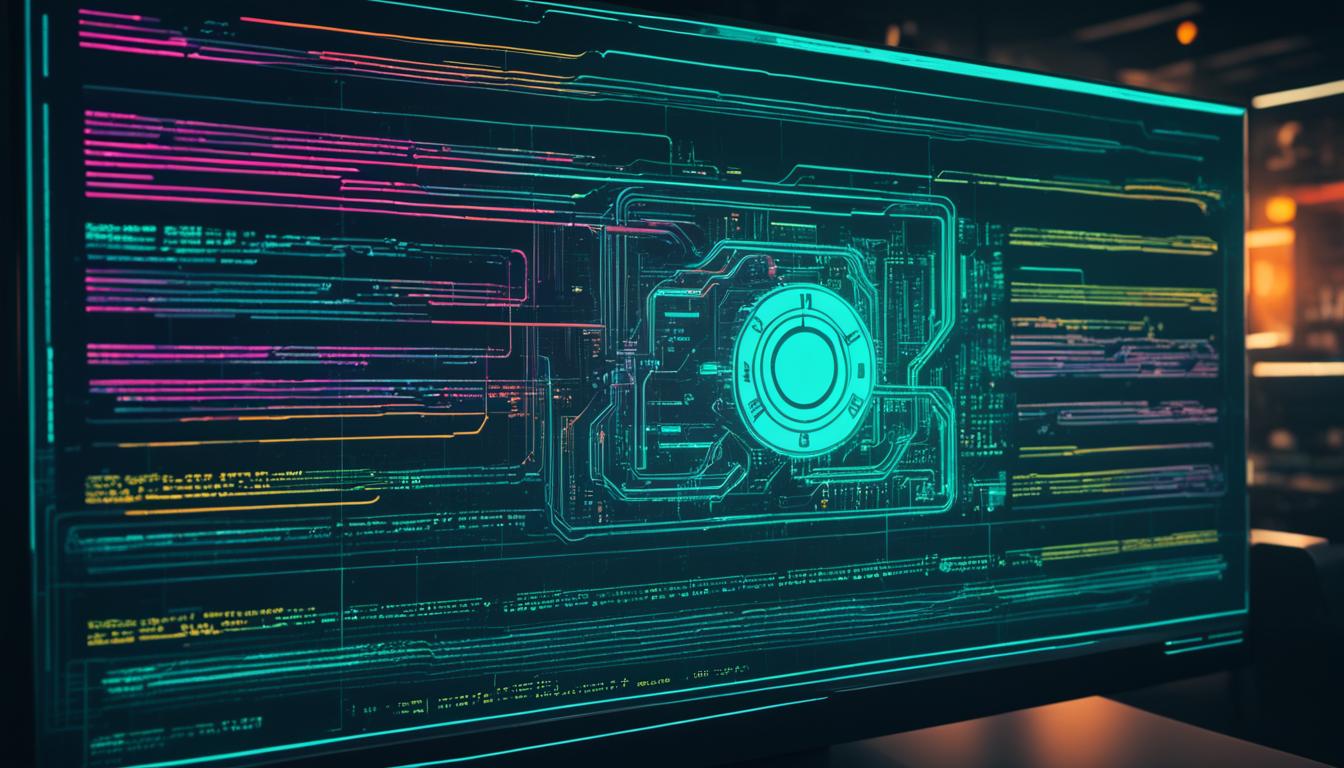
ES6 Method | Functionality |
---|---|
async/await | Simplifies asynchronous workflows by making code resemble synchronous code. |
Promise.all | Handles multiple promises and resolves when all promises in an array are resolved. |
Promise.race | Handles the fastest promise, resolving as soon as the first promise in an array settles. |
Iterating and Looping with ES6 Methods
ES6 brings tools that make going through data easier in JavaScript. Features like forEach, for...of, and entries improve code clarity and efficiency.
Using forEach, you can quickly act on every array item. It gets rid of old-school for loops. This means a neater, more straightforward way to handle data in arrays.
"Using the forEach method not only makes my code more concise and elegant, but it also helps me avoid common issues like off-by-one errors that can occur with traditional for loops. It's a game-changer!" - Emily, JavaScript Developer
For...of loop offers an easy path to go through iterables like arrays or strings. You don't have to manually index every time you need an element or character.
- Arrays would look like this:
Example | Code |
---|---|
Iterating over an array using for...of |
|
- Strings would look like this:
Example | Code |
---|---|
Iterating over a string using for...of |
|
The entries method helps when you need to go over objects and see each property's key and value. It gives an iterable that works with for...of loop for object entries.
Here's an example:
const person = {
firstName: 'John',
lastName: 'Doe',
age: 30
};
for (const [key, value] of Object.entries(person)) {
console.log(`${key}: ${value}`);
}
Output:
firstName: John
lastName: Doe
age: 30
This makes dealing with object properties easier. You can do tasks based on key-value pairs.
ES6 methods like forEach, for...of, and entries, make going through data simpler. They help you write code that's both effective and easy to follow.
Key Takeaways:
- forEach simplifies array iterations, making code clearer.
- for...of loop lets you easily walk through iterables without manual indexing.
- entries is great for working with object properties, showing key and value.
With ES6 methods, your JavaScript skills will grow stronger. You can now use these smart techniques for better coding.
ES6 Methods in Practice: Real-World Examples
You now understand ES6 methods and their features. It's time to see how they work in real life. We'll look at examples that show these JavaScript enhancements in action. This will help you use them in your projects and get the most out of them.
Let's dive right into some specific examples:
Example 1: Array Filtering with ES6 Methods
With filter(), you can pick out elements from an array that meet certain conditions. Here's an example:
```
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((number) => {
return number % 2 === 0;
});
console.log(evenNumbers);
```
This code selects even numbers from the numbers
array, making a new array called evenNumbers
. This new array will have just the values [2, 4]. This shows how you can quickly work with and sort data from larger arrays.
Example 2: Simplifying String Operations with ES6 Methods
ES6 brings new methods for strings that make common tasks easier. Take a look at this example:
```
const message = "Hello, world!";
console.log(message.startsWith("Hello")); // true
console.log(message.includes("world")); // true
console.log(message.endsWith("!")); // true
```
Here, the startsWith(), includes(), and endsWith() methods check a string in different ways. They make it easy to work with strings in JavaScript.
Example 3: Simplifying Asynchronous Operations with ES6 Methods
ES6 has great tools for working with asynchronous tasks. Consider this example with Promise.all():
```
const fetchUserData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("User data fetched");
}, 2000);
});
};
const fetchProductData = () => {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Product data fetched");
}, 3000);
});
};
Promise.all([fetchUserData(), fetchProductData()])
.then((results) => {
console.log(results);
});
```
In this code, fetchUserData()
and fetchProductData()
show asynchronous data fetching. The Promise.all() method handles multiple promises at once. When resolved, the then()
method shows results from both functions. This makes managing asynchronous tasks easier and more organized.
Example 4: Enhanced Object Manipulation with ES6 Methods
ES6 provides better ways to work with objects. Look at how these methods help with object manipulation:
```
const student = {
name: "John",
age: 22,
major: "Computer Science",
};
// Getting the object keys
const keys = Object.keys(student);
console.log(keys); // ["name", "age", "major"]
// Getting the object values
const values = Object.values(student);
console.log(values); // ["John", 22, "Computer Science"]
// Getting the object entries
const entries = Object.entries(student);
console.log(entries); // [["name", "John"], ["age", 22], ["major", "Computer Science"]]
```
This example uses Object.keys(), Object.values(), and Object.entries() to handle the student
object. It makes it easy to access and manipulate object properties.
These examples show how ES6 methods can enhance your programming. As you practice, you'll find more ways to use ES6 methods in your projects.
Remember, practice makes perfect. Keep experimenting with different cases to explore the full potential of ES6 methods.
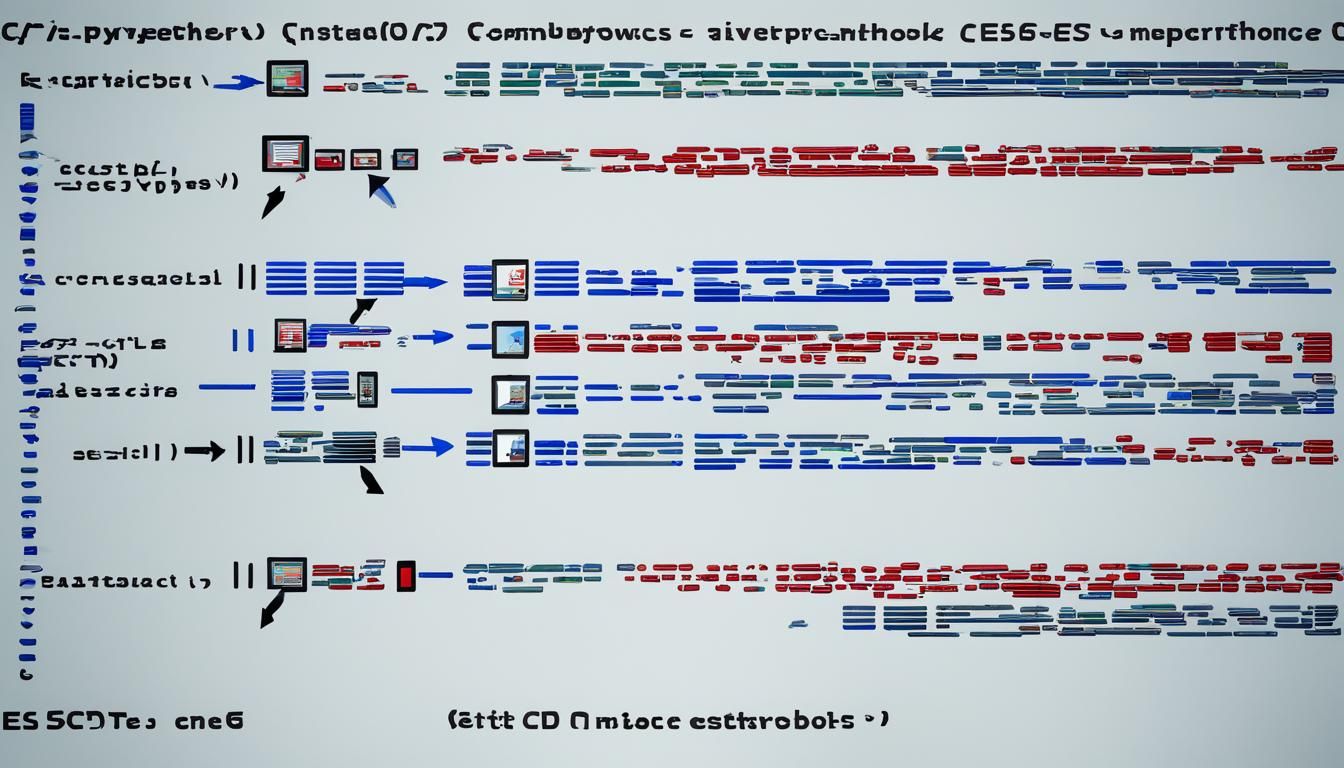
Exploring Advanced ES6 Method Concepts
In this section, we'll dive deeper into ES6 methods. We aim to understand these topics better. This understanding will sharpen your JavaScript skills and help you use ES6 to its full potential.
Method Chaining
ES6 methods let you chain multiple operations together. It's like doing a series of tasks with one item without stopping. For example:
const result = numbers.filter(num => num % 2 === 0)
.map(num => num * 2)
.reduce((sum, num) => sum + num);
This method chaining makes your code neat and more readable. It removes unnecessary variables from your code.
Default Parameter Values
ES6 lets you set default values for function parameters. So, your function works even if some arguments are missing. Here's how it works:
function greet(name = 'friend') {
console.log(`Hello, ${name}!`);
}
greet(); // Output: Hello, friend!
greet('Alice'); // Output: Hello, Alice!
This feature avoids extra checks for missing arguments. It makes your code cleaner and more versatile.
Rest and Spread Operators
The rest and spread operators make array and object handling much easier.
The rest operator (...
) captures many function arguments into one array. It's great for functions with many inputs. Here's an example:
function sum(...numbers) {
return numbers.reduce((total, num) => total + num);
}
console.log(sum(1, 2, 3)); // Output: 6
console.log(sum(4, 5, 6, 7)); // Output: 22
Meanwhile, the spread operator (...
) separates array or object elements. It's useful for copying or merging them:
const numbers = [1, 2, 3];
const copiedNumbers = [...numbers];
console.log(copiedNumbers); // Output: [1, 2, 3]
const obj1 = { x: 1, y: 2 };
const obj2 = { z: 3, ...obj1 };
console.log(obj2); // Output: { x: 1, y: 2, z: 3 }
Take Your ES6 Skills Further
Exploring these advanced ES6 methods will up your JavaScript game. Mastering method chaining, default parameters, and operators will clean up your code. They let you unlock JavaScript's full power in a simpler, efficient way.
Conclusion
You've now finished an in-depth look at ES6 methods, sharpening your modern JavaScript skills. We've walked through the basics and explored different features with real examples. This has set you up to apply what you've learned to your projects.
Armed with these ES6 skills, you're ready to improve your code. You can tackle arrays, work with objects, or make your asynchronous coding better. JavaScript ES6 gives you lots of power.
Keep practicing to get even better. Try out these methods and unlock ECMAScript 6's full powers. The JavaScript world keeps changing, and knowing ES6 puts you ahead in your coding journey.
FAQ
What are ES6 methods?
ES6 methods are the new features in ECMAScript 6 (ES6) that make JavaScript better. They include arrow functions, template literals, and destructuring assignments. This makes the code simpler and easier to read.
How do ES6 methods differ from traditional JavaScript coding?
ES6 methods offer big updates over old-style JavaScript coding. They introduce new ways and features that make complex tasks simple. This makes the code look cleaner and work better.
How can I incorporate ES6 methods into my JavaScript code?
To use ES6 methods, make sure your browser or environment supports ECMAScript 6. You can add ES6 methods to your code or get them from outside modules.
What are some of the array manipulation methods introduced in ES6?
ES6 brought in great array manipulation methods like map, filter, and reduce. They help you change, sift through, and sum up array data easily. This cleans up your code and makes it more straightforward.
Can ES6 methods simplify working with objects?
Yes, ES6 methods offer new ways to handle objects. With methods like Object.keys, Object.values, and Object.entries, you can easily get keys, values, or both. This makes playing with objects much simpler.
How can ES6 methods enhance asynchronous programming?
ES6 methods like async/await, Promise.all, and Promise.race make asynchronous operations easier. They allow you to write asynchronous code that is cleaner, easier to keep up, and less likely to have bugs.
What are some of the ES6 methods used for iterating and looping through data?
ES6 gives you handy methods like forEach, for...of, and entries for going through data in JavaScript. These methods make your code neater and help you be more productive when using collections or iterables.
Can you provide real-world examples of ES6 methods in action?
Sure! You can see ES6 methods in action in many ways, like changing, filtering, and tweaking data in web apps, handling operations that wait for each other, and making complex code simpler.
What advanced concepts related to ES6 methods should I explore?
You should dig into advanced ES6 methods concepts like chaining methods together, default parameter values, and using rest and spread operators. These ideas help you write tidier and sharper code. Plus, they let you fully use ES6 methods.