Welcome to the thrilling world of web development with Express.js! It's perfect whether you're a pro or just beginning. Express.js stands out for its power and efficiency in server-side web development. It's a top choice for developers thanks to its simple yet strong features.
We'll delve into Express.js essentials here and offer tips to improve your web development prowess. We'll cover everything from the basics to deploying your projects. Get ready to upgrade your skills!
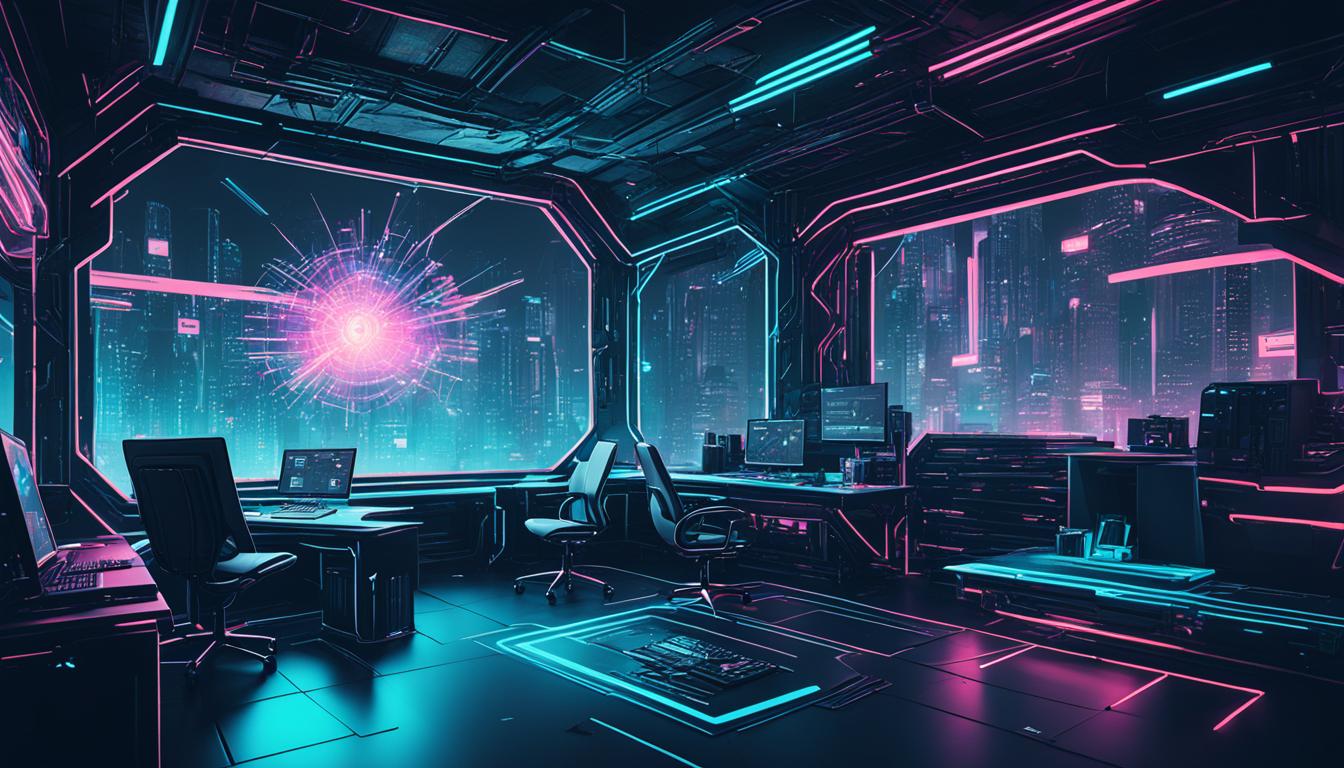
Key Takeaways:
- Express.js is a popular framework for server-side web development.
- It provides a robust and efficient solution for building web applications.
- Express.js is closely related to Node.js and leverages its capabilities.
- Understanding the basics of Express.js will help kickstart your web development journey.
- Stay tuned for valuable tips and best practices throughout the article!
What is Express.js?
Express.js is a powerful web framework used with Node.js. It offers a simple yet broad set of features for server-side apps. This makes it great for developers who want to build efficient and scalable web services.
It's based on Node.js, which is known for its fast, event-driven architecture. This lets developers create quick and responsive web applications easily.
With Express.js, making routes, handling requests and responses, and using middleware is straightforward. Its modular design helps with reusing code. This makes apps easier to maintain and grow.
Express.js has excellent middleware support. Middleware functions let developers run code before and after processing requests and responses. They can do logging, authentication, error handling, and more with middleware.
It also has many plugins and extensions. Developers can add many features like database connections and session management easily. This makes Express.js versatile for various web development tasks.
Overall, Express.js is a strong, easy-to-use framework for server-side development. Its simplicity, ability to scale, and rich ecosystem are why many developers choose it for their projects.
Getting Started with Express.js
If you're diving into server-side web development with JavaScript, the Express.js framework is a top pick. It's designed for creating web apps efficiently. Here, we'll guide you through the basics of getting started with Express.js.
This includes installing the framework and setting up your app's core structure.
Installation
To begin, you need to install Express.js in your development space. Make sure Node.js is already set up on your computer. You can then add Express.js by running a command in your terminal:
npm install express
This command installs Express.js and its required parts.
Basic Setup
With Express.js installed, next is structuring your app. Your app starts with a file, often called app.js
or server.js
. In this file, you bring in the Express.js module and kick-start your app like this:
const express = require('express');
const app = express();
Now, you're all set to craft routes, manage requests, and more within Express.js.
Essential Tools
Several key tools work well with Express.js, enhancing your coding journey. Here are a few:
- Body-parser: It's a middleware that interprets the data from incoming requests.
- Morgan: This logs request details, helping with app monitoring and troubleshooting.
- Helmet: It boosts your app's security by setting various HTTP headers.
- Passport: This tool makes adding authentication straightforward.
These and other tools in the Express.js environment can make your development smoother. They also help with app security and functionality.
With a grasp on Express.js basics, you're ready to learn more about its features. Next, we'll look into middleware and its role in your app.
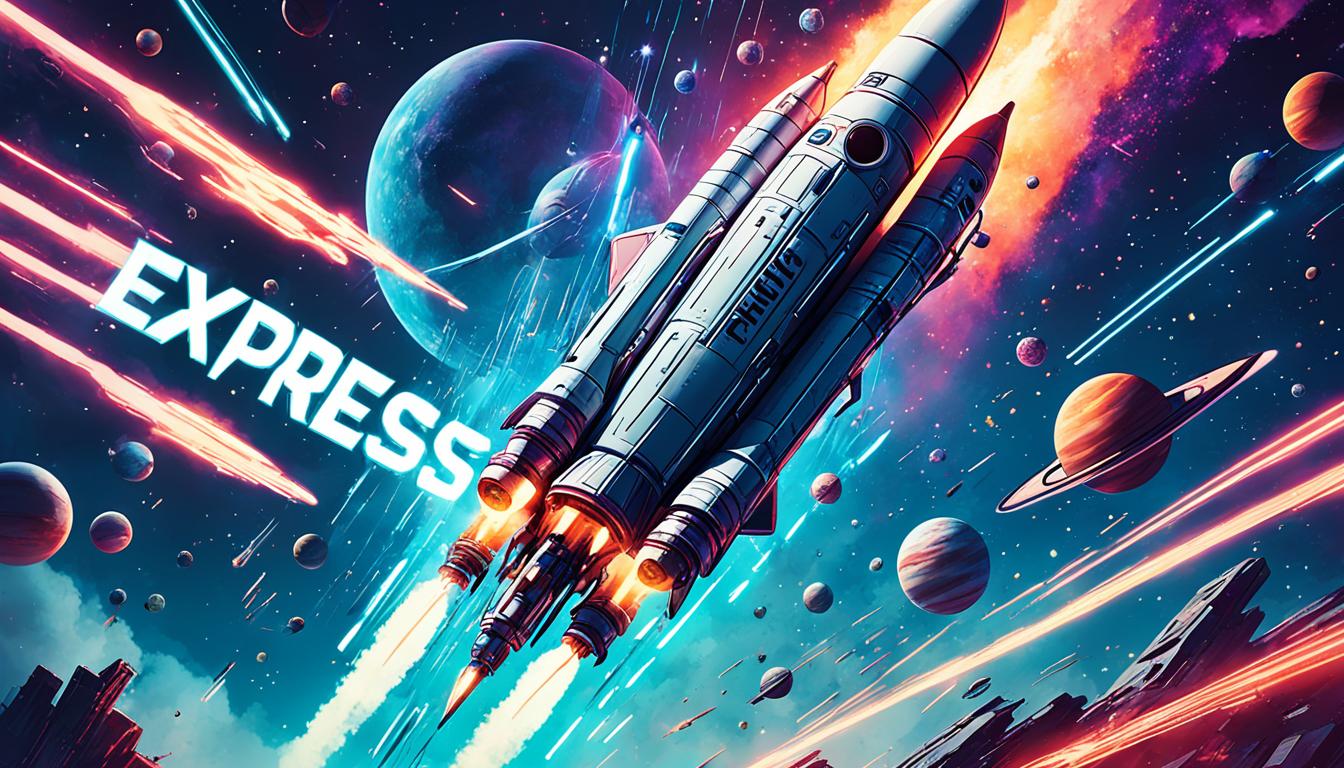
Understanding Middleware in Express.js
In Express.js, middleware is key. It connects server code and client code. It creates a layer that handles requests and responses well. This makes building complex web apps easier by using small, separate functions.
Express.js middleware steps in to edit requests and responses. It runs specific tasks before and after the server processes a request. These tasks include logging, checking who's accessing something, making sure data is right, and dealing with errors.
Middleware works in steps in Express.js. A request passes through a series of middleware functions, known as the middleware stack. Each one can change the request or response. This lets developers change how the server acts by adding, removing, or changing features.
- Types of Express.js Middleware:
Express.js has different middleware for various tasks in web development:
- Built-in Middleware: Express.js has built-in functions for basic tasks. These help with static files, reading request bodies, and managing cookies.
- Third-Party Middleware: There’s a lot of extra middleware you can get through npm. These add more features like user checks with passport and better security with helmet.
- Custom Middleware: You can also make your own middleware for what you need. This is great for checking data, who can access what, and managing how often your API is used.
Middleware in Express.js works one after the other. This gives you control over how requests and responses are handled. Usually, you'll stack several middleware functions together. Each one adds something to your app. It's important to put them in the right order so they run correctly.
Using middleware well lets developers split their code up, make their apps more secure, and easier to keep up. Express.js's middleware system offers tools to build server-side apps that can grow and work smoothly.
Routing in Express.js
Routing is key in web development, especially with Express.js. It lets developers set paths and endpoints for the server to react to. This makes handling requests easy and ensures the right data gets back to the user. Mastering routing lets developers make efficient, well-organized apps.
Express.js uses HTTP methods like GET, POST, PUT, and DELETE for routing. This way, developers can manage various request types and take specific actions. For instance, a GET request could pull data from a database, while a POST request might add a new record.
Routes in Express.js can have parameters, making them flexible. These parameters, pulled from the URL, help in fetching certain data or doing specific tasks. This feature makes it easy for developers to use parameters in their server-side logic.
Defining Routes in Express.js
To define routes, developers use app.METHOD() functions in Express.js. "METHOD" stands for any HTTP method like GET or POST. Each route links a URL path to a function that runs when the path is accessed.
Here's a simple route definition for a GET request on the root path:
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello, Express.js!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
When the root path ('/') gets a GET request, the callback function sends back 'Hello, Express.js!'. You can set up more complex routes and do more within the callback functions, based on your app's needs.
Handling Different HTTP Methods
Express.js lets developers handle various HTTP methods. This is great for building RESTful APIs or doing CRUD operations.
Here's how to set up routes for different HTTP methods:
app.get('/users', (req, res) => {
// Get all users
});
app.post('/users', (req, res) => {
// Create a new user
});
app.put('/users/:id', (req, res) => {
// Update a user with the given ID
});
app.delete('/users/:id', (req, res) => {
// Delete a user with the given ID
});
This setup lets the server handle GET, POST, PUT, and DELETE requests for '/users'. It runs the right function for each HTTP method to carry out needed tasks.
Passing Parameters in Routes
Developers can include parameters in routes with Express.js. These are marked with a colon (:) and a name. You can get to them using the req.params object.
Here's an example of a route with a parameter:
app.get('/users/:id', (req, res) => {
const userId = req.params.id;
// Perform operations using userId
});
In this case, the server handles GET requests for '/users/:id', where :id is the parameter. The callback uses req.params.id to operate with the user ID. It could fetch user info or do other tasks.
Knowing how to work with routing in Express.js is vital for developing strong server-side apps. By setting up routes, managing HTTP methods, and using parameters, developers can make flexible endpoints. These endpoints meet specific user needs and deliver the right content or services.
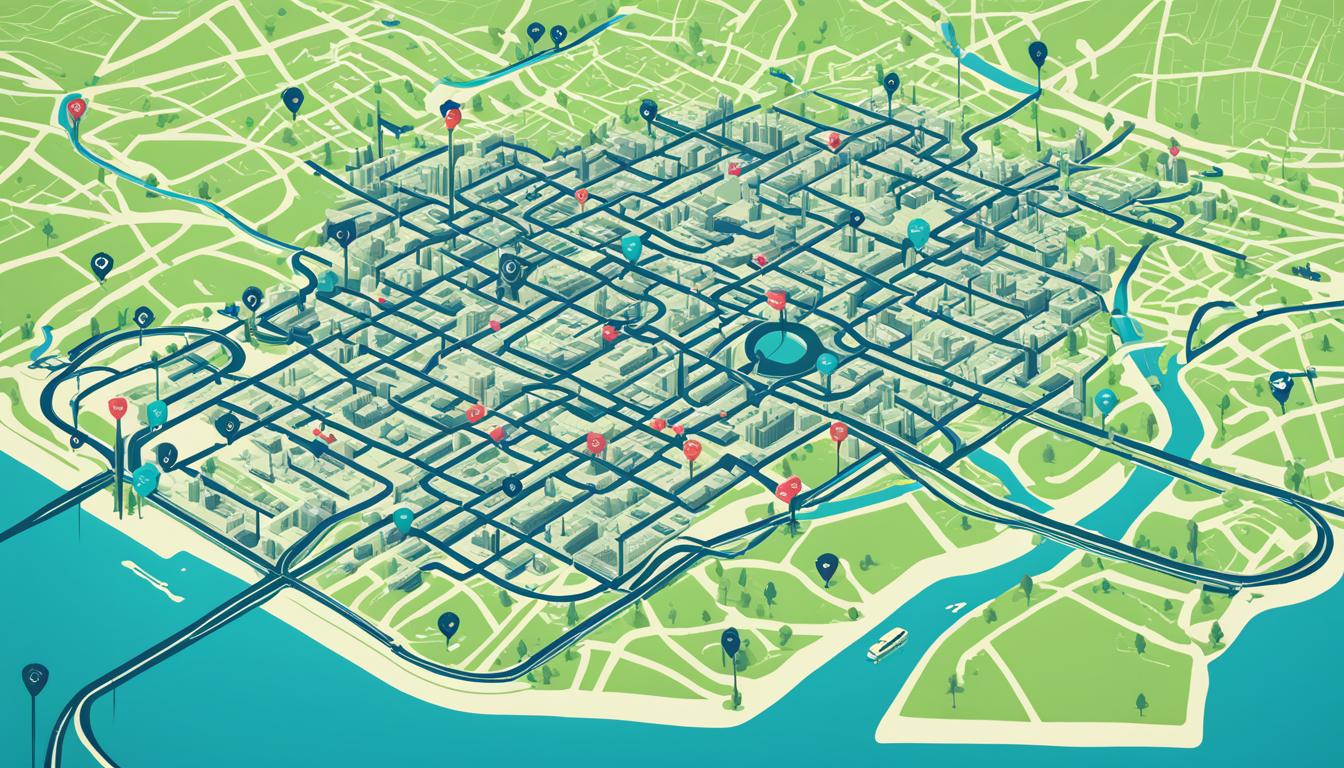
Building APIs with Express.js
Express.js is a powerful choice for web development and server-side programming. It offers a solid platform for API creation. This lets developers build applications that are smooth and scalable.
Express.js stands out for its simple design. This simplicity means developers can focus on their API's key features. They can avoid getting bogged down by complex details.
Handling API Requests
Express.js makes handling API requests straightforward. Developers set up routes and callbacks to respond to client requests correctly. It supports various HTTP methods like GET, POST, PUT, and DELETE. This ensures smooth server and client interactions.
Authentication and Security
Express.js views security as key when creating APIs. It lets developers use authentication middleware for user verification. This controls API route access. Also, integrating third-party authenticators is easy. This boosts security and makes logging in user-friendly.
Implementing Common API Functionalities
Express.js eases adding common API features such as pagination and filtering. It uses query parameters for filtering by specific criteria. This helps clients find the data they need. For managing large data, it enables pagination through limits and offsets.
Error Handling and Middleware
Handling errors well is vital, and Express.js offers strong error management tools. By using middleware, developers can globally tackle errors. This means APIs send meaningful error messages and statuses, improving the user experience.
"Express.js is a reliable, efficient framework for API development. Its rich features, easy syntax, and smooth integration make it perfect for web projects."
- Jane Johnson, Senior Full-Stack Developer
Express.js helps developers create durable, scalable APIs for various applications. It simplifies handling requests, managing authentications, and adding functionalities. Express.js gives the tools needed to make API development smoother and high-performing.
Key Features of Express.js for Building APIs | Benefits |
---|---|
1. Routing and handling different HTTP methods | Easy interaction with the client, allowing for seamless communication. |
2. Authentication and security mechanisms | Enhanced security and control over API access, protecting sensitive data. |
3. Support for implementing common API functionalities | Efficient data retrieval through filtering and pagination. |
4. Error handling and middleware functionality | Global error handling and improved user experience for API consumers. |
Advanced Features and Best Practices
As developers get better with Express.js, they find new cool features. These help make web projects better in terms of safety, speed, and how fun they are to use. By tapping into these, the quality of apps skyrockets.
Error Handling
It's really important to deal with errors the right way in web apps. Express.js has tools like error-handler middleware for this. This stops your app from crashing when things go wrong. Also, writing down errors helps fix and improve your app.
"Express.js offers a range of error-handling middleware options and logging libraries to facilitate the management of errors in web applications."
Logging
Logging is key to understanding your app and solving problems. With libraries like Morgan and Winston, Express.js lets developers keep track of HTTP requests, errors, and more. This makes finding issues and enhancing security easier.
Security
For security, Express.js has many tools and tips to keep apps safe. Things like checking input, making sure users are who they say, and using security tools like Helmet help a lot. They keep data safe and block hackers.
Performance Improvements
There are ways to make Express.js apps run faster. Using browser and server caching with Redis cuts down wait times. Also, async programming helps servers do their job better and faster.
By using these tips and tools, developers can really make their Express.js apps shine. This means apps that are strong, safe, and fast.
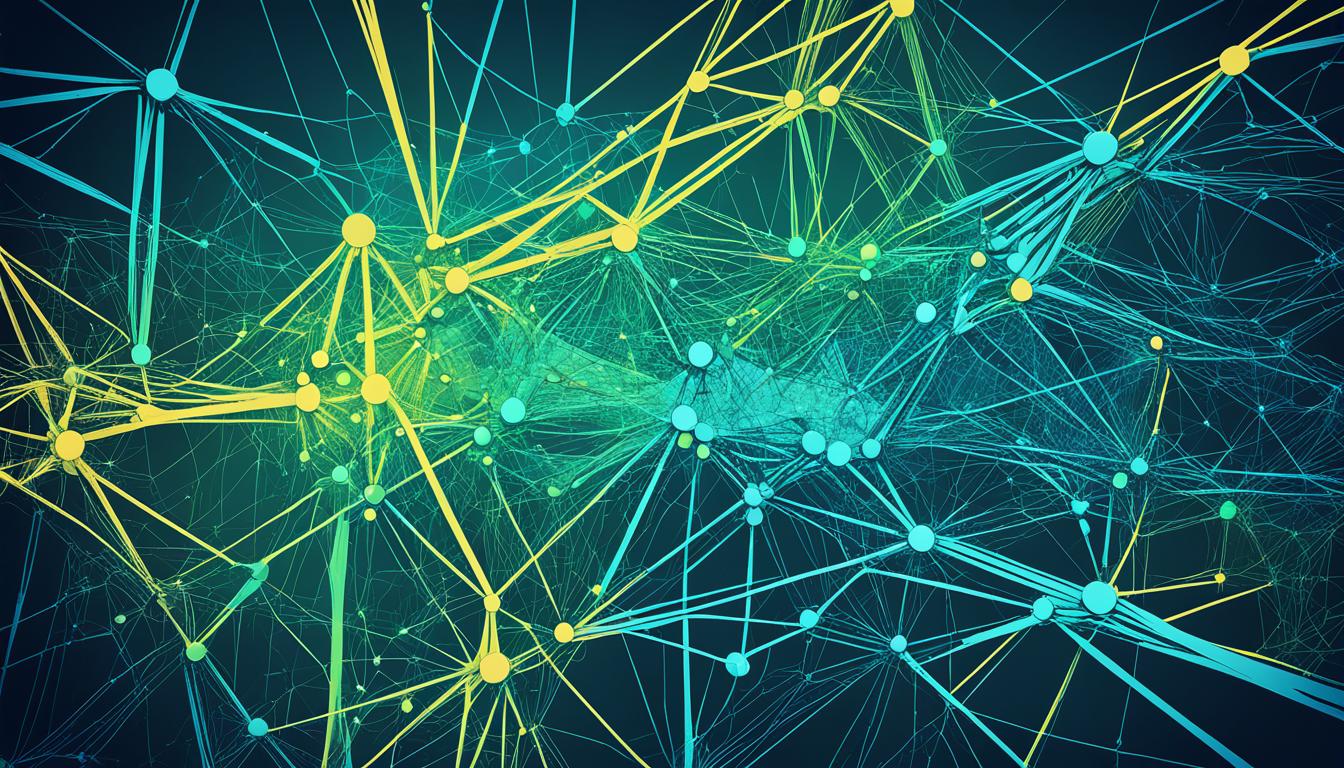
Advanced Features | Best Practices |
---|---|
Error Handling Middleware | Implement robust logging |
Logging Libraries (e.g., Morgan, Winston) | Secure sensitive data |
Security-focused Middleware (e.g., Helmet) | Optimize caching |
Caching Mechanisms (Browser and Server-side) | Utilize asynchronous programming |
Integrating Express.js with Frontend Frameworks
Combining Express.js, a robust server-side JavaScript framework, with frontend frameworks like React and Angular, results in powerful web applications. This blend offers the backend strengths of Express.js with the dynamic interfaces of frontend systems. Thus, developers can craft interactive and efficient apps.
Follow these steps to integrate Express.js with popular frontend frameworks:
Integrating Express.js with React
1. Start a new React project using the create-react-app tool.
2. Inside the React project, make an API folder.
3. Install axios to manage HTTP requests from React.
4. Add a proxy in React's package.json for API requests to Express.js.
5. Create an Express.js route for API requests from React.
6. The Express.js route will handle and respond to API requests.
Integrating Express.js with Angular
1. Use Angular CLI to start a new Angular project.
2. Add an Express.js server to the Angular project.
3. Install HttpClient in Angular for requests to Express.js.
4. Make an API service in Angular for the API calls.
5. Create routes in Express.js for Angular's API requests.
6. Express.js routes will manage and reply to API requests.
Integrating Express.js with React and Angular gives developers strong backend and frontend tools. It ensures smooth communication between backend and frontend. This helps in making web applications that are rich in features and responsive.
Integrating Express.js with frontend frameworks helps developers use both technologies' strengths to make innovative web applications. It's great for creating RESTful APIs or managing CRUD operations. This integration brings many possibilities for web development.
Express.js makes web development efficient and straightforward. Frontend frameworks boost the user experience with lively interfaces. By using the powers of Express.js, web development, and JavaScript, developers can make solid applications. These applications meet the demands of today's digital age.
Deploying Express.js Applications
There are several ways to deploy Express.js apps. It's key to pick a method that ensures your project runs well. You might choose a cloud service, a virtual private server (VPS), or even a dedicated server. This guide covers important tips, best practices, and how to scale your deployment.
Deployment Options
Express.js apps can be deployed in different ways, based on your needs. Some popular choices include:
- Cloud Services: Use platforms like AWS, Google Cloud, or Microsoft Azure. They offer scalability, balance loads automatically, and are reliable.
- Dedicated Servers: This option gives the most control and lets you optimize performance for your Express.js app.
- Virtual Private Servers (VPS): VPS hosting is both affordable and offers a good balance of control.
- Containerization: Using Docker or Kubernetes for containers makes your Express.js app portable, scalable, and consistent.
Scaling Strategies
When your app gets bigger, you need good strategies to manage more traffic. Some scaling tips include:
- Load Balancing: Spread out traffic across several servers to perform better and manage more users.
- Caching: Use caching to save time on accessing data and lessen the load on your app.
- Database Optimization: Make database actions faster through better queries, indexes, and caching.
- Horizontal Scaling: Add more servers as needed, either manually or automatically, to manage more traffic.
Best Practices
It's important to follow best practices for smooth deployment and running of your Express.js app. Key advice includes:
"Use automation like CI/CD pipelines or scripts to make deployments consistent and less prone to errors."
"Set up monitoring and logging to understand performance, find problems, and solve them efficiently."
"Make sure your app is secure by encrypting data and using authentication to keep data safe."
Sample Deployment Architecture
Here is a basic setup for deploying an Express.js app:
Component | Description |
---|---|
Load Balancer | Distributes requests to different servers to balance loads and improve availability. |
Application Servers | Runs several instances of the Express.js app to manage incoming requests. |
Database | Stores data and manages actions from the Express.js app. |
Cache Server | Keeps commonly accessed data ready to speed up performance and lower backend load. |
This setup helps your Express.js app scale well, stay available, and perform optimally.
Deploying your Express.js apps will be easier with this knowledge. Choose the best deployment method, scale your app smartly, follow good practices, and plan your deployment well. Doing so will create a stable and efficient environment for your project.
Performance Optimization in Express.js
Optimizing your Express.js web applications is key to a smooth user experience. Specific techniques like caching, database optimization, and asynchronous programming can boost your app's speed. These methods enhance your Express.js app's performance significantly.
Caching
Caching is a major way to speed up Express.js apps. It lets you store commonly accessed data or views on your server. This reduces database load and speeds up response times. Tools like Redis or Memcached make data retrieval fast and improve your app's feel.
Database Optimization
Improving database queries is crucial for Express.js apps. Using indexing, selecting specific fields, and avoiding unneeded joins helps in faster data fetching. Also, use connection pooling to avoid the cost of setting up new connections for each request. This makes your app respond quicker.
Asynchronous Programming
Asynchronous programming can greatly speed up your Express.js apps. Using JavaScript promises or async/await helps manage multiple requests at once. This leads to better resource use and quicker responses. It keeps your app running smoothly by avoiding delays due to I/O operations.
"Performance optimization in Express.js is like fine-tuning a high-performance sports car. By carefully implementing caching, optimizing database queries, and leveraging asynchronous programming, developers can achieve lightning-fast response times and deliver an exceptional user experience."
- Jane Smith, Senior Software Engineer at Company XYZ
To make your Express.js app fast and responsive, use these optimization techniques. But remember to test and monitor performance. This helps find and fix any issues to make your app even better.
Technique | Description |
---|---|
Caching | Storing frequently accessed data or rendered views on the server to reduce database load and improve response times. |
Database Optimization | Optimizing database queries through indexing, querying specific fields, and avoiding unnecessary joins to enhance performance. |
Asynchronous Programming | Utilizing JavaScript promises or async/await syntax to handle multiple requests concurrently and prevent blocking. |
Knowing and using these methods can unleash the full power of the Express.js framework. It lets developers make server-side JavaScript web apps that are very fast.
Conclusion
Express.js is a strong tool for creating websites, especially on the server-side. It works well with JavaScript and Node.js. This makes it flexible and efficient for making powerful and scalable web apps.
We talked about what Express.js is, including its features and how it handles middleware and routing. We also looked at how to make APIs and some advanced tips to make development better.
With Express.js, creating APIs, working with front-end technologies, and launching apps is straightforward. Its simplicity and flexibility are great for JavaScript developers making server-side apps.
Keep learning about Express.js by looking into more resources, tutorials, and guides. Whether you're starting out or have experience, Express.js is a reliable framework for your web development projects.
FAQ
What is Express.js?
Express.js is a framework for making web apps and APIs. It's built on Node.js. This setup offers a simple way to create server-side applications.
Why should I use Express.js?
Developers like Express.js for its ease of use and flexibility. It helps in making scalable applications. Plus, there's a huge community support and many plugins.
How do I get started with Express.js?
First, ensure Node.js is installed on your device. Then, install Express.js using npm. You can now start your Express.js project and build your application.
What is middleware in Express.js?
Middleware is a function that can edit requests and responses in Express.js. It's used for tasks like authentication and logging. It helps in managing requests efficiently.
How does routing work in Express.js?
Routing lets you manage different HTTP requests and set up routes. Use methods like `app.get()` to define routes. Express.js matches these to execute the right handler.
Can I build APIs with Express.js?
Yes, you can. Thanks to its simplicity, Express.js is great for APIs. It supports request handling, authentication, and other API features.
Are there any advanced features and best practices in Express.js?
Certainly! Express.js offers tips for better apps. It includes error handling and security. Using these can make your apps more reliable and fast.
Can I integrate Express.js with frontend frameworks like React or Angular?
Absolutely! Express.js works well with frameworks like React and Angular. This lets you build strong full-stack apps, combining backend with great UIs.
How do I deploy Express.js applications?
You have many options for deployment. Services like Heroku, AWS, or DigitalOcean are popular. Techniques like load balancing improve your app's ability to handle more visitors.
How can I optimize the performance of my Express.js application?
Improving performance in Express.js involves caching, optimizing databases, and using asynchronous programming. These steps help your app run faster and smoother.