Welcome to our guide on Node Package Manager (NPM), the top choice for managing Node.js projects.
Node.js changed the JavaScript development scene by allowing the creation of scalable apps. But, dealing with modules and dependencies can be tough. NPM makes it easier.
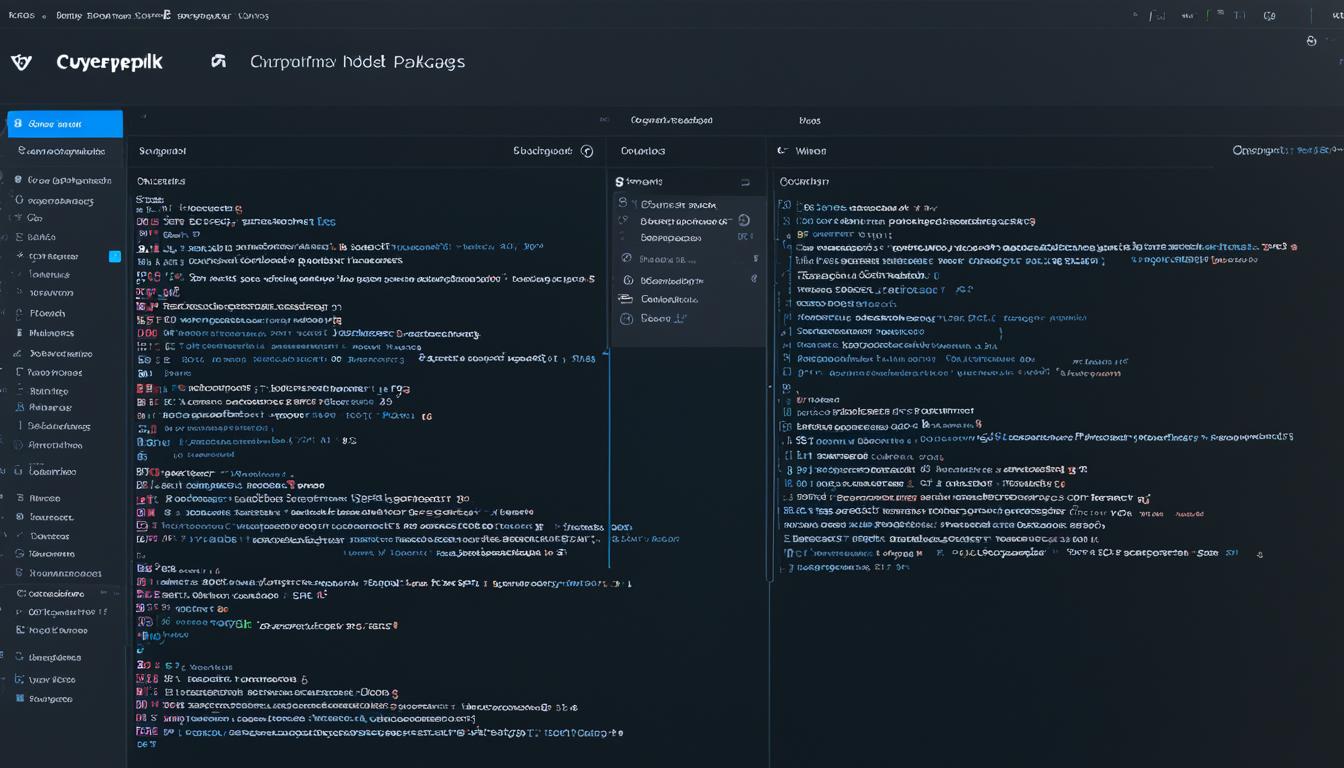
NPM is the core of the Node.js world, giving you access to over 1 million packages. It lets you easily add what your project needs, from authentication tools to database management and frontend frameworks.
This guide will show you the key parts of NPM. You'll learn how to use it to manage your packages better and make your Node.js development smoother.
Key Takeaways:
- Node Package Manager (NPM) is essential for handling modules and dependencies in Node.js projects.
- As a powerful package manager for JavaScript apps, NPM makes it easy to use libraries and frameworks.
- With a library of over 1 million packages, NPM offers vast resources for developers.
- It helps with installing, managing, and versioning dependencies, leading to a better development flow.
- Creating and sharing your NPM packages can help the open-source community and allow you to work with others.
Understanding Node.js and NPM
Node.js is well-known for letting developers use JavaScript on the server-side. It's great for creating scalable web apps, real-time apps, and APIs. Its huge ecosystem and many third-party packages and libraries are key to its success.
"Node.js has revolutionized the way web applications are built. Its event-driven, non-blocking I/O model allows for high-performance applications, and NPM plays a crucial role in managing the dependencies required for these applications."
The Node Package Manager (NPM) is essential for Node.js developers around the world. It offers a central place for reusable modules. This makes it easier to add, share, and manage project dependencies. Using third-party libraries and frameworks becomes simpler, which saves time and effort.
NPM is key not only for Node.js but also for JavaScript apps. It helps developers handle dependencies, control versions, and manage projects more effectively.
Benefits of using NPM as a JavaScript package manager
- Easy dependency management: NPM helps you manage project dependencies easily. It makes sure you have the right versions of libraries.
- Large package ecosystem: There's a vast collection of open-source packages in NPM. This lets developers use existing solutions to speed up development.
- Version control: NPM lets developers set specific package versions. This ensures projects work the same in different settings.
- Automatic dependency resolution: NPM automatically handles package dependencies. This makes managing complex projects easier.
Understanding Node.js and NPM is vital for anyone building web applications, command-line tools, or backend APIs with JavaScript. Next, we'll show you how to install NPM. This will get you started on using its powerful features and capabilities.
Getting Started with NPM Installation
Setting up your development environment starts with installing NPM on your local machine. By following a few easy steps, you can have NPM running quickly.
Step 1: Check for Node.js Installation
First, make sure Node.js is on your machine. Since NPM comes with Node.js, you need Node.js installed first. To check, open your terminal and type:
node -v
If you see a version number, Node.js is installed. If not, go to https://nodejs.org and follow the steps for your system.
Step 2: Verify NPM Installation
Once Node.js is confirmed, check if NPM is installed. In the terminal, enter:
npm -v
If you see a version number, NPM is installed. If it's missing, follow your system's install method.
Step 3: NPM Installation Methods
Different systems have different ways to install NPM. Here are the usual methods:
- Method 1: Node.js Installer: If Node.js isn't on your device, the Node.js installer is a good start. It includes NPM. Just download and run the installer from https://nodejs.org. Node.js and NPM will install together.
- Method 2: Package Manager: You can use a package manager like Homebrew for macOS or Chocolatey for Windows. These commands will install Node.js and NPM:
Operating System | Package Manager | Command |
---|---|---|
macOS | Homebrew | brew install node |
Windows | Chocolatey | choco install node |
Before these commands, install Homebrew or Chocolatey. Visit https://brew.sh or https://chocolatey.org for steps.
- Method 3: Node Version Manager (NVM): For managing multiple Node.js and NPM versions, NVM is excellent. It lets you switch versions easily. To install, follow the guide at the official GitHub page (https://github.com/nvm-sh/nvm).
Step 4: Final Test
To confirm NPM installation, run this in the terminal:
npm -v
If you see a version number, the install was a success. You are now set with NPM for your Node.js projects.
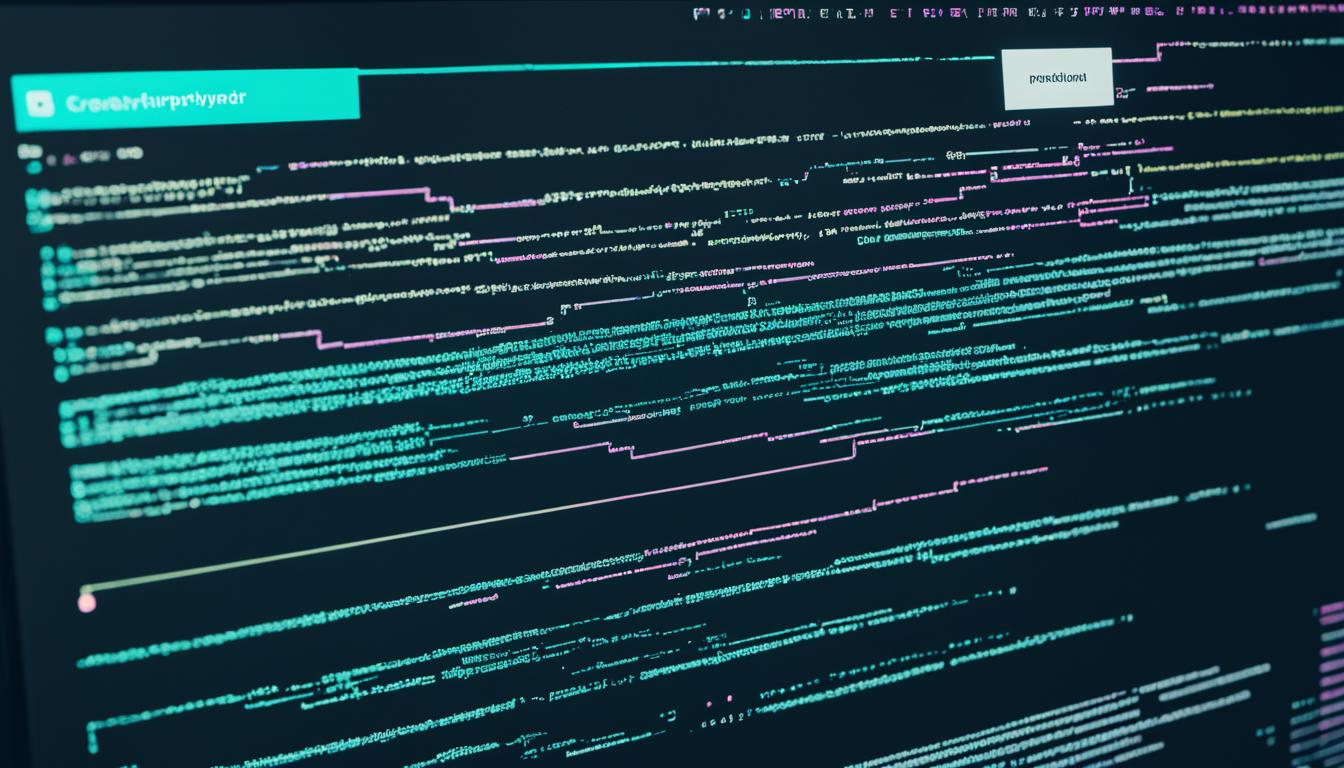
NPM is a powerful tool for managing your Node.js project's modules and dependencies. Next, we'll look at the huge variety of NPM packages in the Node.js package registry. You'll learn how to search for and pick packages for your projects.
Discovering NPM Packages
Working with Node.js applications gets easier when you use NPM packages. The Node.js package registry is full of packages that make your projects better and faster.
By using NPM packages, you tap into solutions others have made. This saves you from having to create everything from scratch. Look for what you need in the NPM registry and add it to your project.
Finding NPM packages is simple. You can use the NPM website or the command line. Search using keywords, the package name, or the author's name.
Choosing the right package is crucial. Here are some things to consider:
- Popularity: More downloads and stars often mean the package is good and has strong community support.
- Documentation: Good packages have clear documentation. This includes how to use it, API details, and how to install it.
- Activity: Make sure the package gets regular updates. This means it's likely to be free of bugs and have the latest features.
- Dependencies: Check the package’s dependencies for compatibility with your project. They shouldn't complicate things.
Consider reading reviews or comments from others who have used the package. This can give you insight into their experiences and any issues they faced.
To add a package to your project, use the npm install
command. Mention the package name and version if needed.
Now you know how to find and evaluate NPM packages. Next, we'll see how NPM helps manage dependencies in Node.js projects.
Managing Dependencies with NPM
In Node.js, managing dependencies is key for a smooth project. Dependencies are modules or packages your project needs to work well. These can be libraries, frameworks, or plugins that help speed up development.
NPM makes it easy to handle, install, and keep track of these dependencies. This helps save time and effort. Developers use NPM to list what they need in a package.json file.
The package.json file is like a project's list of needs. It tells you every dependency and its version. This makes it easier for teams to work together and keep things consistent no matter where they're working from.
Installing a package with NPM also handles dependency resolution automatically. It gets the right versions needed for the package. This keeps the project compatible and working well.
NPM also lets developers sort dependencies:
- Dependencies: Key for the project to work. They're needed when the project is live to run correctly.
- Dev Dependencies: Used only during development and testing. This can be tools or frameworks to help build and refine the project. These aren't included in the final application.
This way, NPM helps manage packages smartly. It shrinks the application size for deployment and smooths out the development process.
Here's what a package.json file might look like with both dependencies and dev dependencies:
{
"name": "my-project",
"version": "1.0.0",
"dependencies": {
"express": "^4.17.1",
"lodash": "^4.17.21"
},
"devDependencies": {
"mocha": "^9.1.1",
"chai": "^4.3.4"
}
}
With package.json set up, developers can get all dependencies with a quick NPM install command. NPM then gets the packages and checks for any issues.
Good dependency management with NPM means Node.js projects are always current. They get the latest updates, bug fixes, and security patches. This makes the application more stable and reliable.
NPM Dependency Commands
Command | Description |
---|---|
npm install |
Installs all the project dependencies listed in the package.json file. |
npm install <package> |
Installs a specific package and its needed dependencies. |
npm uninstall <package> |
Removes a specific package and its dependencies from the project. |
npm update |
Brings all packages up to date as per the package.json file's versions. |
npm outdated |
Shows which packages are out of date and suggests updates. |
NPM helps developers efficiently manage project dependencies. This is crucial for a project's success, making it stable and ready to grow.
Creating and Publishing NPM Packages
Making your own NPM package lets you share code with Node.js developers. It’s great for modules, tools, or frameworks. The Node.js module manager makes publishing and distributing easy.
To create your NPM package, just follow a few steps:
Step 1: Initialize your package
Start in your project folder's command line and type:
npm init
You'll be asked for package info like name, version, and description. After filling it out, a package.json
file appears in your project.
Step 2: Add package files
Make sure your project folder has all necessary files. Leave out files not needed by the end user.
Step 3: Define dependencies
List any third-party dependencies in your package.json
file's dependencies
section. This helps users install what they need for your package easily.
Step 4: Test your package
Test to make sure your package works well. Using Mocha or Jest, write and run tests. This builds trust in your code's stability.
Step 5: Publish your package
When you’re ready, publish with this command in your project:
npm publish
This uploads your package for others to use.
Pick a unique name for your package to avoid conflicts. Add good documentation and a README to help users understand your package. This way, you also contribute to the open-source community and innovation.
Benefits of Creating and Publishing NPM Packages | Challenges of Creating and Publishing NPM Packages |
---|---|
|
|
Optimizing NPM Performance and Security
Making your Node Package Manager (NPM) work better and safer is key. It makes managing your packages smooth and worry-free. To do this well, follow best practices and these tips. They'll help you get the most out of NPM and keep risks low.
Update to the Latest NPM Version
It's important to always use the newest NPM version. New updates improve performance and make things more secure. The NPM team works on fixing problems and making the manager better with each update.
Manage and Clean Up Dependencies
Projects can get bogged down with old or unneeded dependencies. It's good to check your project's dependencies often. Remove anything that's not needed anymore. This makes your package smaller and speeds up installs.
Having too many dependencies can slow things down. It's smart to think about using different packages or writing your code in a way that uses fewer dependencies. This helps keep your NPM running quickly.
Use Caching
Caching makes installing packages much faster. This is especially true if you're working with others or on multiple projects. NPM's built-in caching saves packages locally. That means quicker access and fewer downloads from the internet.
Implement Authentication and Access Controls
For better security, use authentication and access controls. This means setting up access tokens and defining who can do what. It helps keep your private packages safe and makes sure only the right people can make changes.
Regularly Audit and Update Packages
Security risks are always changing. It's important to keep your packages up-to-date. Watch out for security warnings and pick your packages carefully. Make sure to update any that have known problems right away.
Utilize NPM Audit
"With NPM Audit, you can automatically check your project's dependencies for issues. This tool helps you spot and fix problems early. It keeps your project safe and secure."
NPM Audit gives detailed info on weak spots and how to fix them. Adding NPM Audit checks to your workflow helps you catch vulnerabilities early. This keeps your project secure.
Secure Your NPM Account
To keep your NPM account safe, use a strong, unique password. Adding two-factor authentication (2FA) adds even more security. It stops unauthorized people from accessing your account or changing your packages without permission.
Stay Informed and Engage the NPM Community
The NPM community is full of resources and knowledgeable people. Stay up-to-date by following the NPM blog, joining forums, and going to meetups. This keeps you in the loop and helps you learn from experts.
Getting involved with the NPM community helps you stay ahead. You'll learn new things and make sure your NPM use is both successful and secure.
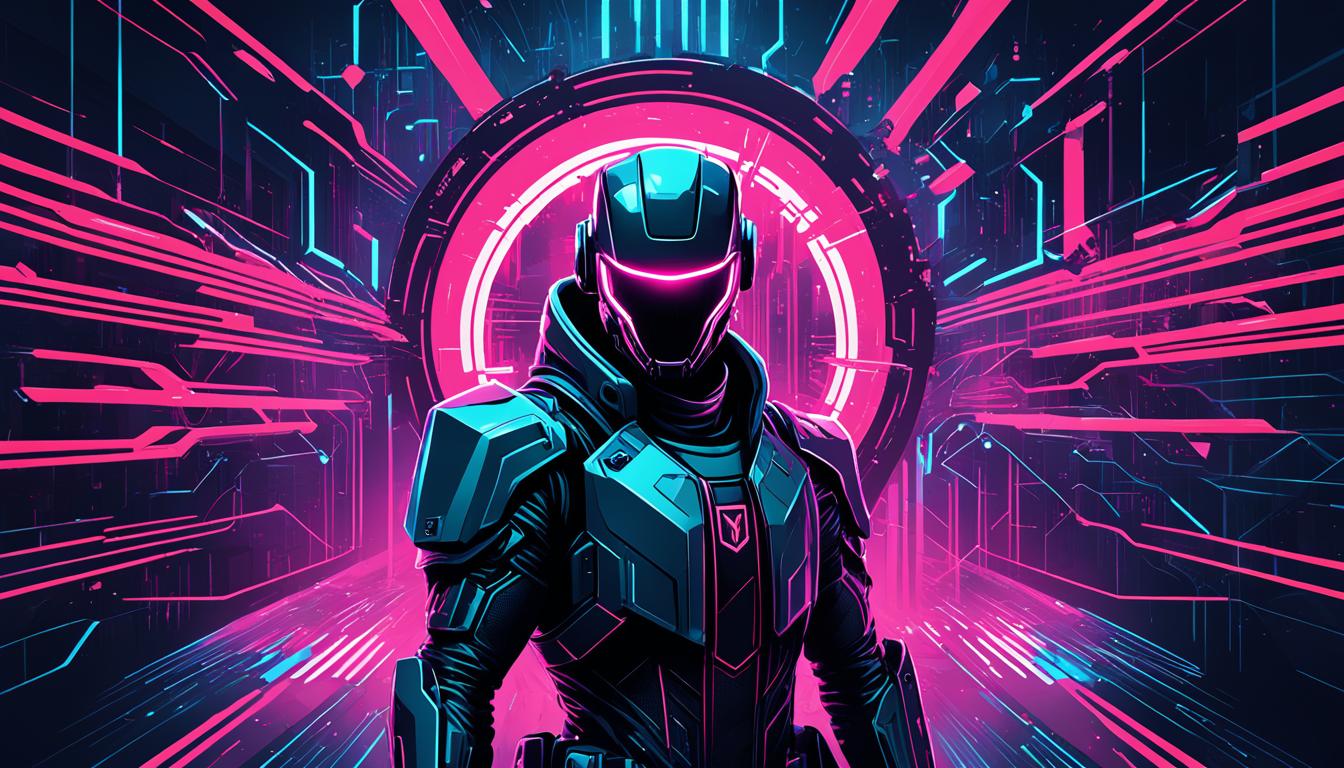
NPM Scripts and Automation
Automating tasks helps make software development more efficient and productive. With Node Package Manager (NPM), developers can automate many tasks. This saves time and effort. Let's look at how NPM scripts can make your development process better.
The Power of NPM Scripts
Developers can create custom commands in the package.json
file with NPM scripts. You can run these scripts with the npm run
command. This makes automating tasks easy.
NPM scripts let you do many things, such as:
- Running unit tests
- Building and bundling assets
- Deploying applications
- Generating documentation
- Executing code linting
- Managing database migrations
This flexibility means developers can set up workflows that fit their project needs.
Creating NPM Scripts
Add a script object in the scripts
part of your package.json
file to make an NPM script. Use a key-value pair. The key is the script name. The value is the command to run.
"scripts": {
"test": "jest",
"build": "webpack --mode production",
...}
For instance, the test
script runs Jest, and the build
script uses Webpack in production mode.
To run a script, use this command:
npm run <script-name>
Managing Script Dependencies
NPM scripts can rely on others, making complex workflows possible. Just start a script command with npm run
to call other scripts in your project.
For example:
"scripts": {
"lint": "eslint",
"lint-and-test": "npm run lint && npm run test"
...}
In this code, lint-and-test
runs lint
then test
. The &&
means the second runs only if the first succeeds.
Customizing NPM Scripts
You can tailor NPM scripts with arguments and environment variables. Pass arguments through the command line. Access environment variables using process.env
in the script.
For arguments, do this:
npm run <script-name> -- <argument>
And for environment variables:
"scripts": {
"start": "node -v"
...}
Here, the start
script shows the Node.js version.
Contributing to Open-Source Projects with NPM
NPM scripts help with contributing to open-source. Many projects have scripts for building, testing, and more. Get to know the scripts in a project's package.json
to start contributing.
Examples of Useful NPM Scripts
Here are common NPM scripts you can use:
- lint: Runs code linting with ESLint or Prettier.
- test: Tests your project.
- build: Triggers the build process.
- start: Starts your app or server.
- deploy: Pushes your app to production.
Automating Your Workflow
With NPM scripts, automate tasks and improve your workflow. This lets you focus on coding rather than setup. NPM helps you achieve more with less effort.
Next, we'll look into advanced NPM features and integrations in section 9. These can boost your development productivity even further.
Advanced NPM Features and Integrations
In this section, we'll delve into the sophisticated features and integrations of Node Package Manager (NPM). These advanced tools boost your project management, making collaboration smoother and adding more functionalities. They lift your development work to higher ground.
1. Scoped Packages
Scoped packages bring organization to NPM by grouping related modules under one name. Great for big projects or teams, they keep things tidy and separate. For instance, using "@myorganization/mypackage" makes your modules unique and easy to find.
2. Custom Scripts
With NPM, you can set up custom scripts in your package.json
file. Those scripts do wonders for automating tasks and making development smoother. They let you run tests, start servers, or push updates with one command, saving you time.
3. Versioning and SemVer
Managing versioning is easier with NPM thanks to Semantic Versioning (SemVer). In your package.json
, symbols like "^" and "~" help you pick version ranges without losing compatibility. SemVer guidelines help keep your project stable as you update dependencies.
4. Package Locking
The package-lock.json file NPM creates ensures every developer gets the same package versions. It locks down the dependency tree for consistent installs. This way, package locking ends conflicts and makes your dev environment reproducible.
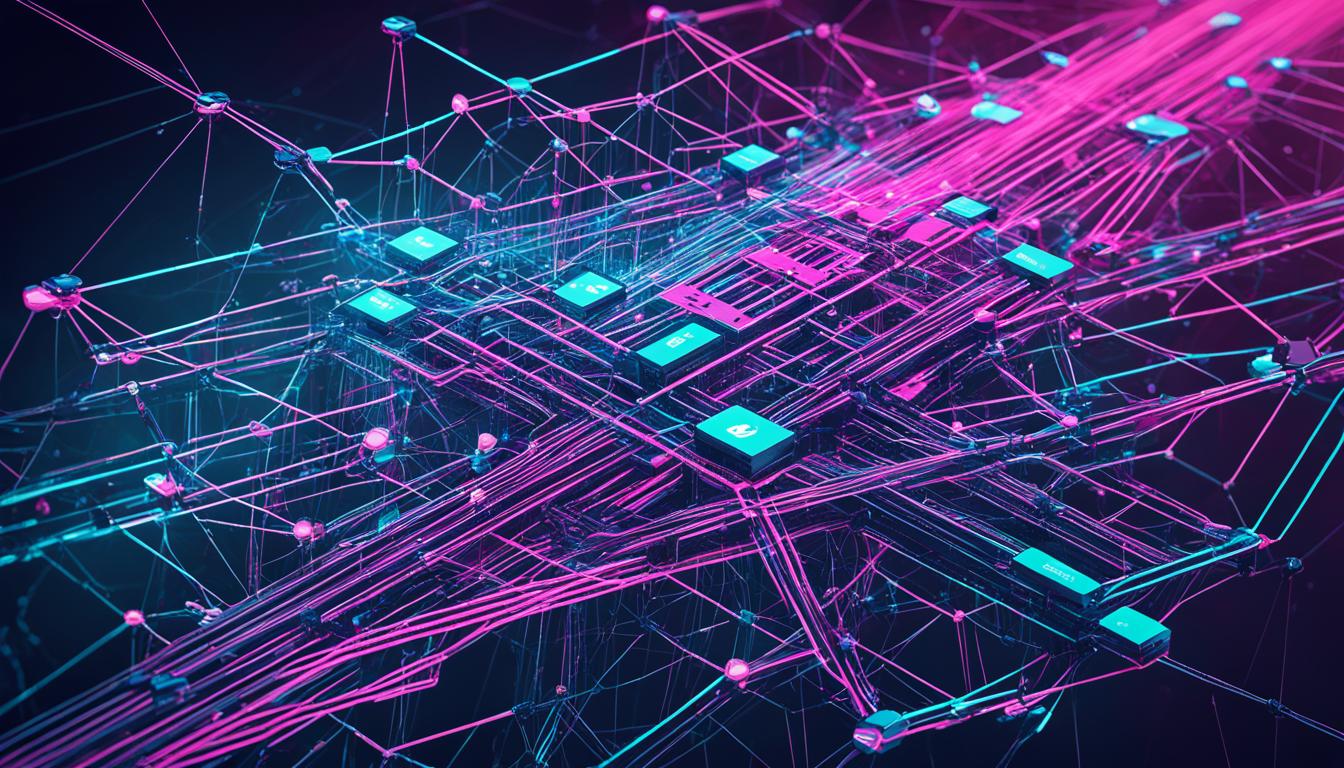
5. Integration with Continuous Integration and Deployment Tools
NPM works well with top CI/CD platforms like Jenkins and CircleCI. Including NPM commands in your CI/CD pipelines automates and streamlines development. This leads to faster project cycles and consistent, ongoing delivery of your software.
Feature | Description |
---|---|
Scoped Packages | Organize and manage related modules with a namespace |
Custom Scripts | Define and execute custom automation tasks |
Versioning and SemVer | Manage package versions using Semantic Versioning |
Package Locking | Ensure consistent package installations across environments |
Integration with CI/CD Tools | Automate builds, tests, and deployments with ease |
These advanced NPM features and integrations empower you to enhance your development process. They boost teamwork, secure your Node.js projects, and ensure stable, reliable outcomes.
Conclusion
In conclusion, the Node Package Manager (NPM) is vital for Node.js projects. It lets developers organize, install, and update dependencies with ease. This ensures a smooth development process.
We've looked at NPM from starting up to exploring its vast package registry. We learned about managing dependencies and making our packages. We’ve also seen how to boost security and performance, use NPM scripts, and benefit from its advanced features.
By using NPM, developers can make their Node.js projects better. It helps with teamwork, makes code reuse easier, and makes development smoother. It's important for both new and skilled developers to use NPM. It helps make scalable, well-maintained, and high-quality Node.js applications.
So, take advantage of NPM and improve your Node.js projects. Dive into its vast package ecosystem, use its features to work smarter, and integrate it into your workflow. NPM is key for easy dependency management, increasing productivity, and moving forward in Node.js development.
FAQ
What is NPM?
NPM is short for Node Package Manager. It's the main package manager for Node.js. It handles the installation, management, and publishing of software packages. This tool makes it easy for developers to add and manage their project dependencies.
How do I install NPM?
First, you need to install Node.js, which comes with NPM. After installing Node.js, NPM will be ready to use on your system. To check the NPM version, open a terminal and type "npm -v".
How do I find and use NPM packages?
To find NPM packages, visit the NPM website or use the "npm search " command. Install a package by typing "npm install ". You can then add it to your Node.js project with the "require" function or by importing it.
How do I manage dependencies with NPM?
Managing dependencies is simple with NPM. Everything is listed in a "package.json" file. To install these dependencies, run "npm install" in your project's root. NPM will take care of the rest, downloading and installing what's needed.
How do I create and publish my own NPM package?
Start by making a package.json file that describes your package. Then, to publish, use "npm publish". Once your package is out there, others can use it in their projects.
How can I optimize NPM performance and security?
For better performance, utilize caching and try "npm ci" for quicker installs. Keep your dependencies updated for security. Use "npm audit" to find and fix security issues.
How can I automate tasks with NPM?
"npm scripts" let you set up custom commands for routine tasks. Add these scripts to your package.json. Then, execute them with "npm run". This helps with building, testing, or deploying your project.
What are some advanced features and integrations with NPM?
NPM comes with features like hooks for action before or after commands. It also works well with other tools, including Git and continuous integration systems.
What is the role of NPM in Node.js projects?
NPM is vital for Node.js projects. It makes package and dependency management easier. Developers save time and maintain consistent project structures. This fosters better teamwork.