Welcome to the world of asynchronous programming in JavaScript! If you're looking to write cleaner and more efficient code, understanding Async/Await in JavaScript is essential. With the introduction of ES6, JavaScript gained a powerful feature that simplifies working with asynchronous functions.
By leveraging the async/await syntax, you can streamline your async operations and make your code easier to read and maintain. Whether you are new to JavaScript or an experienced developer, mastering Async/Await will take your coding skills to the next level.
In this section, we will explore the fundamentals of Async/Await in JavaScript, diving into the syntax and highlighting the benefits of using it in your projects. Through easy-to-understand examples, we will demonstrate how Async/Await can enhance the performance of your applications. By the end of this section, you will have the essential knowledge to harness the full potential of Async/Await in JavaScript.
Let's embark on this journey of mastering Async/Await in JavaScript and unlock a new level of coding efficiency!
Understanding the Basics of Async/Await
In this section, we will dive deeper into the concepts behind Async/Await in JavaScript. We will cover the basics of working with async functions and understanding the syntax of Async/Await. You will learn how to handle promises with Async/Await and explore real-world examples that showcase the benefits of using this approach. By the end of this section, you will have a solid understanding of how Async/Await works and how to implement it in your JavaScript projects.
The Async/Await feature in JavaScript allows you to write asynchronous code in a more concise and readable manner. It builds upon the foundation of Promises and provides a simpler way to handle asynchronous operations.
Let's start by explaining the fundamental concepts of Async/Await:
- Async Functions: An async function is a special type of function that can be used with the await keyword. When invoked, an async function returns a Promise. This allows us to work with asynchronous operations synchronously, making the code more readable and maintainable.
- Await Keyword: The await keyword is used inside an async function to pause the execution until a Promise is resolved or rejected. It only works inside async functions.
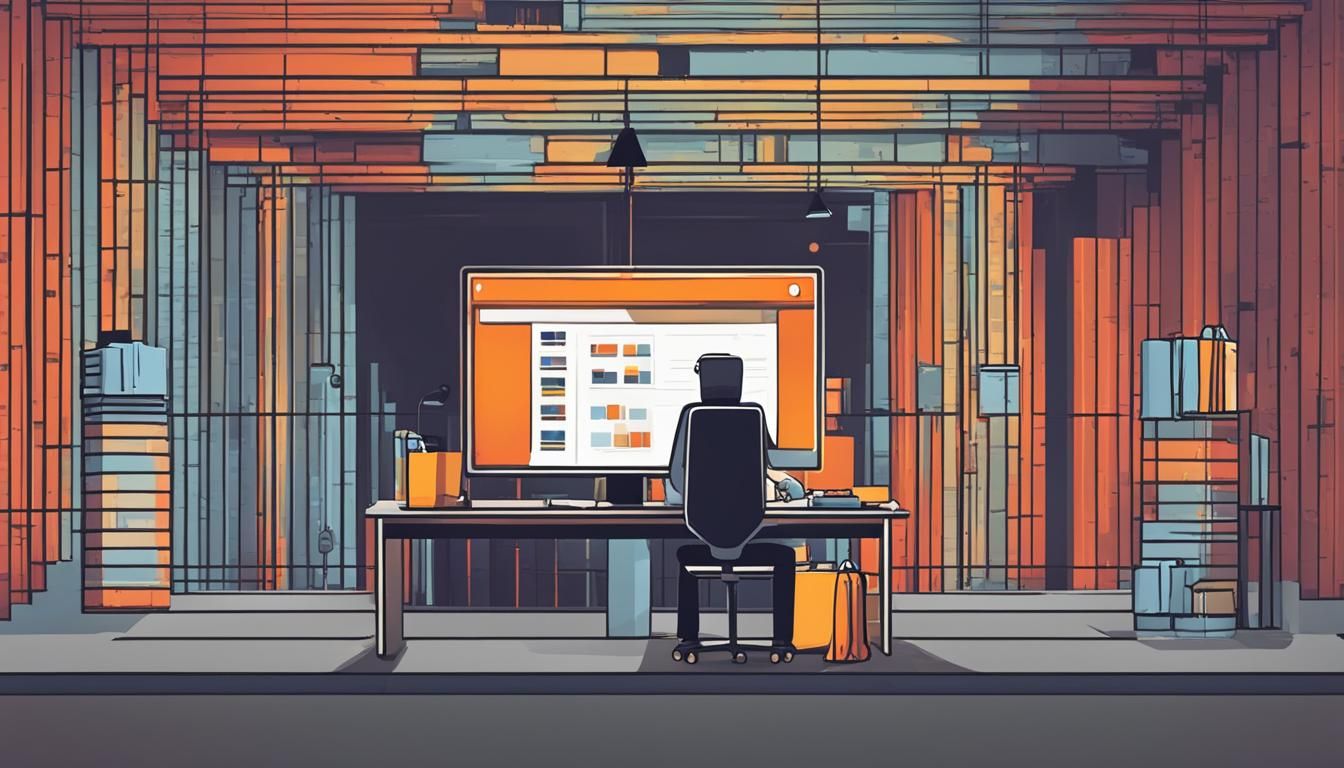
Let's take a look at a simple example that demonstrates the power of Async/Await:
Async/Await Example:
async function getData() {
try {
const response = await fetch('https://api.example.com/data');
const data = await response.json();
return data;
} catch (error) {
console.error(error);
throw new Error('Failed to fetch data');
}
}
getData()
.then(result => console.log(result))
.catch(error => console.error(error));
In this example, we define an async function called getData
. It uses the await
keyword to wait for the response of a fetch request and then converts the response data to JSON. If any errors occur, the catch
block handles them gracefully.
The getData
function is then called and the returned Promise is handled using .then
and .catch
methods. This approach allows us to write asynchronous code in a more linear and intuitive way.
By utilizing Async/Await, you can simplify the handling of promises and create cleaner, more maintainable code. It is particularly useful when dealing with complex asynchronous operations or when multiple async operations need to be executed in a specific order.
Real-World Examples:
To further illustrate the benefits of Async/Await, let's explore a few real-world examples:
- Fetching Data from an API: In web development, it's common to make API requests to retrieve data. With Async/Await, you can easily handle the asynchronous nature of the requests and simplify the code in the process.
- Working with Libraries: Many JavaScript libraries and frameworks use Promises for asynchronous operations. Async/Await provides a more straightforward syntax for working with these libraries, making your code more readable and maintainable.
- File Uploads: Uploading files asynchronously can be a complex task. Async/Await simplifies the handling of file uploads by allowing you to wait for each step of the process, making it easier to track the upload progress and handle any errors that may occur.
As you can see, Async/Await is a powerful feature that enhances the readability and maintainability of your JavaScript code. By understanding the basics of how Async/Await functions and utilizing real-world examples, you can harness its full potential in your JavaScript projects.
The Key Differences: Await vs. Async in JavaScript
In JavaScript, the 'await' and 'async' keywords play crucial roles in achieving asynchronous behavior in your code. Understanding the differences between these two keywords is essential for effectively using the Async/Await feature. Let's explore how 'await' and 'async' work together and how they can enhance the performance of your JavaScript applications.
The keyword 'async' is used to define an asynchronous function. When a function is declared as 'async', it automatically returns a promise. This promise allows the function to be paused and resumed, ensuring that the code within it executes in the expected order. By utilizing 'async' functions, you can write asynchronous code in a more structured and readable manner.
The 'await' keyword, on the other hand, is used to wait for a promise to resolve before proceeding further. When 'await' is used within an 'async' function, it temporarily pauses the execution of that function until the awaited promise is resolved or rejected. This allows you to write code that appears synchronous while still benefiting from the performance optimizations of asynchronous operations.
By using 'await' and 'async' together, you can create more efficient and readable asynchronous code. The 'await' keyword allows you to easily handle promises without the need for extensive 'then' and 'catch' statements. It simplifies the process of working with asynchronous code and allows you to write logic that closely resembles synchronous programming.
"Using 'await' and 'async' in JavaScript can greatly enhance the performance and readability of your code. It provides a powerful way to handle asynchronous operations while keeping your code structured and maintainable."
When to Use 'await' and 'async'
Understanding when to use 'await' and 'async' is crucial for optimizing your JavaScript code. Here are some scenarios where these keywords are commonly used:
- When making HTTP requests to APIs: 'await' allows you to wait for the response from an API before proceeding with further code execution.
- When working with databases: 'await' enables you to wait for database queries to complete, ensuring data integrity and avoiding race conditions.
- When dealing with time-consuming operations: 'await' allows you to pause execution until a time-consuming task, such as image processing or file uploads, is complete.
By using 'await' and 'async' appropriately in these situations, you can enhance the performance and reliability of your JavaScript applications.
Conclusion
Mastering Async/Await in JavaScript is crucial for developers who want to improve the quality and efficiency of their code. By utilizing this powerful feature, you can simplify complex asynchronous operations and enhance the overall performance of your applications.
In this article, we covered the essentials of Async/Await, giving you a solid foundation to build upon. We explored the basics, delving into the syntax and usage of async functions and the benefits of working with promises in conjunction with Async/Await. Real-world examples showcased the advantages of this approach, highlighting its ability to streamline code and enhance readability.
We also discussed the differences between 'await' and 'async', clarifying their individual roles within JavaScript. Understanding these distinctions, such as when to use 'await' to pause the execution of a function and 'async' to declare an asynchronous function, is crucial for effectively utilizing Async/Await.
Armed with this knowledge, you are now equipped to harness the full potential of Async/Await in your JavaScript projects. By implementing cleaner, more maintainable code, you can build faster and more efficient applications that deliver an enhanced user experience.
FAQ
Q: What is Async/Await in JavaScript?
A: Async/Await is a feature introduced in JavaScript ES6 that allows developers to write asynchronous code in a more synchronous and readable manner. By using the 'async' keyword with a function, and the 'await' keyword before an asynchronous operation, developers can pause the execution of code until the operation completes, without blocking the entire program.
Q: How does Async/Await work?
A: When an async function is called, it returns a Promise. The 'await' keyword is used to pause the execution of the function until the Promise is resolved or rejected. While waiting for the Promise to resolve, the JavaScript engine can continue executing other tasks. Once the Promise is resolved, the function resumes execution, and the value returned by the Promise is available for further processing.
Q: What are the benefits of using Async/Await?
A: Using Async/Await can make asynchronous code easier to read and understand. It eliminates the need for callback functions or chaining multiple promises. Async/Await also allows the developer to handle errors more effectively using try/catch blocks, making error handling more straightforward compared to using plain Promises.
Q: Can I use Async/Await with any asynchronous operation?
A: Yes, you can use Async/Await with any function that returns a Promise, such as making HTTP requests, interacting with databases, or reading/writing files. By using the 'await' keyword before these operations, you can ensure that the function waits for them to complete before moving on to the next line of code.
Q: What happens if an error occurs in an async function?
A: If an error occurs within an async function, you can handle it using a try/catch block. If an error is thrown inside the 'try' block, the execution jumps to the corresponding 'catch' block. This allows you to gracefully handle the error and prevent your program from crashing.
Q: Is there a limit to the number of 'await' statements I can use in an async function?
A: No, there is no limit to the number of 'await' statements you can use in an async function. You can use multiple 'await' statements to handle multiple asynchronous operations in a single function. Each 'await' statement will pause the execution of the function until the respective Promise is resolved or rejected.