Welcome to the world of Javascript data structures! In this section, we will explore the essential data structures that form the foundation of every Javascript developer's toolkit. By mastering these data structures, you will be equipped with the knowledge and skills to efficiently store and manipulate data in your code.
JavaScript is a powerful programming language widely used for web development. To harness its full potential, it's crucial to understand how to leverage data structures effectively. With the right data structures, you can optimize code performance, improve efficiency, and build robust applications.
In this article, we will cover a range of data structures, including arrays, objects, linked lists, stacks, queues, trees, and graphs. We will delve into each data structure's advantages, use cases, and algorithms that work best with them. By the end of this section, you will have a solid understanding of when and how to implement these data structures in your Javascript projects.
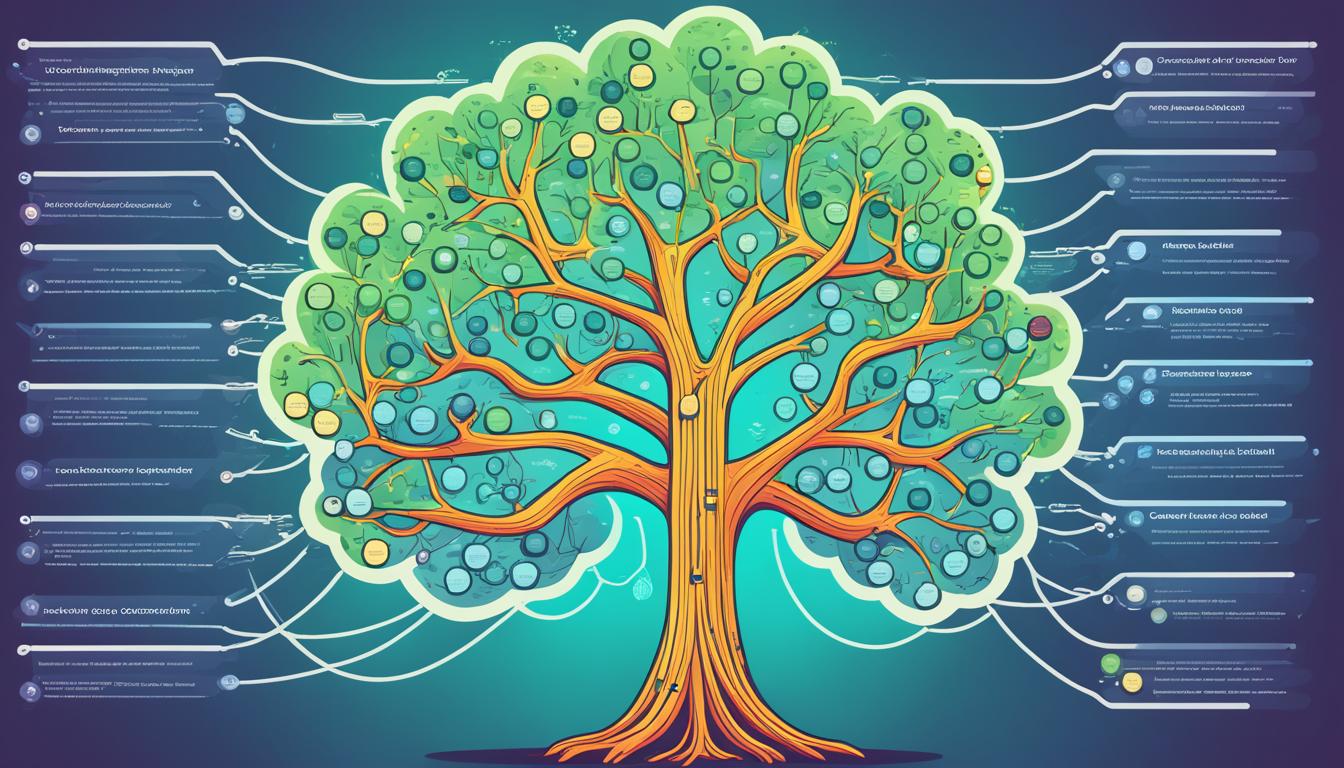
Whether you're a beginner or an experienced developer, understanding data structures in Javascript is essential to writing efficient and maintainable code. So, let's dive in and unlock the power of Javascript data structures together!
Javascript Arrays and Objects
In this section, we will dive deep into Javascript arrays and objects, two fundamental data structures. Arrays provide a way to store and organize multiple values in a single variable, making them highly versatile for various applications. Objects, on the other hand, enable us to store and manipulate complex data by grouping related properties and values together.
Let's first explore JavaScript arrays. Arrays are indexed collections of elements that can hold values of different types. They allow you to store and access multiple values using a single variable. With arrays, you can easily add, remove, and modify elements to dynamically manage data. Here are some common methods and properties that can be used with arrays:
- .push(): Adds one or more elements to the end of an array.
- .pop(): Removes the last element from an array.
- .splice(): Adds or removes elements from an array at a specified position.
- .length: Returns the number of elements in an array.
Now, let's turn our attention to objects in JavaScript. Objects are collections of key-value pairs, where each key is a unique identifier and each value represents a property. They are commonly used to represent real-world entities and complex data structures. Here's an example of how an object can be defined:
const person = {
name: "John Doe",
age: 30,
occupation: "Software Engineer"
};
Objects offer various methods to manipulate and access data, including:
- Object.keys(): Returns an array of an object's enumerable property names.
- Object.values(): Returns an array of an object's enumerable property values.
- Object.entries(): Returns an array of an object's enumerable property key-value pairs.
By understanding the power of arrays and objects in JavaScript, you can efficiently handle complex data and manipulate it effectively. These data structures form the backbone of many JavaScript applications, enabling efficient data management and processing.
Example: Accessing Array Elements
Suppose we have an array of numbers:
const numbers = [1, 2, 3, 4, 5];
To access an element in the array, we can use the index of the desired element:
console.log(numbers[0]); // Output: 1
console.log(numbers[2]); // Output: 3
Method | Description |
---|---|
.push() | Adds one or more elements to the end of an array. |
.pop() | Removes the last element from an array. |
.splice() | Adds or removes elements from an array at a specified position. |
.length | Returns the number of elements in an array. |
Linked Lists, Stacks, and Queues in Javascript
In this section, we will delve into more advanced data structures in Javascript: linked lists, stacks, and queues. These data structures are essential for efficient data management and manipulation, providing valuable tools for developers. By mastering these concepts, you will be one step closer to becoming a proficient Javascript programmer.
Linked Lists:
A linked list is a linear data structure consisting of nodes, where each node contains a data element and a reference (or link) to the next node. This dynamic structure allows for efficient memory management and flexible insertion and deletion operations. Linked lists are particularly useful when the size of the data is unknown at the time of implementation.
Stacks:
A stack is an ordered collection of elements in which the insertion and removal of items can only occur at one end, called the top. It follows the Last-In-First-Out (LIFO) principle, where the last element added is the first one to be removed. Stacks are commonly used in solving problems involving recursion, backtracking, and expression evaluation.
Queues:
A queue is an ordered collection of elements that follows the First-In-First-Out (FIFO) principle, meaning the element added first will be the first one to be removed. Queues are ideal for scenarios such as job scheduling, breadth-first search, and managing resources in real-time systems.
Now, let's take a closer look at the implementation and use cases of these data structures in Javascript.
Linked List Implementation:
To create a linked list in Javascript, you can define a constructor function to represent the nodes and create methods to perform operations such as insertion, deletion, traversal, and searching. Here's an example:
class Node {
constructor(data) {
this.data = data;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
}
// Methods for insertion, deletion, traversal, and searching// ...
}
Stack Implementation:
To implement a stack in Javascript, you can use an array and define methods to mimic the stack operations. Here's an example:
class Stack {
constructor() {
this.items = [];
}
push(element) {
this.items.push(element);
}
pop() {
if (this.isEmpty()) {
return "Underflow";
}
return this.items.pop();
}
// Methods for peek, isEmpty, size, and clear// ...
}
Queue Implementation:
For implementing a queue in Javascript, you can use an array and define methods to simulate the queue operations. Here's an example:
class Queue {
constructor() {
this.items = [];
}
enqueue(element) {
this.items.push(element);
}
dequeue() {
if (this.isEmpty()) {
return "Underflow";
}
return this.items.shift();}
// Methods for front, isEmpty, size, and clear// ...
}
Use Cases of Linked Lists, Stacks, and Queues:
Linked lists, stacks, and queues serve various purposes in software development. Here are some common use cases for each:
Data Structure | Use Cases |
---|---|
Linked Lists |
|
Stacks |
|
Queues |
|
By understanding the implementation and use cases of linked lists, stacks, and queues, you can optimize your code and efficiently manage data in Javascript.
Trees and Graphs in Javascript
As we delve into the final section of this comprehensive guide to mastering data structures in JavaScript, we now turn our attention to the fascinating concepts of trees and graphs. These powerful data structures offer unique capabilities for efficient searching, organizing, and representing complex relationships between data points.
Trees provide a hierarchical structure, where each element (or node) has a specific relationship with its parent and children nodes. This allows for efficient searching and organizing of data, making trees ideal for tasks like representing hierarchical file systems or organizing data in a sorted manner. With a solid understanding of trees in JavaScript, you can easily navigate and manipulate data in an optimized and organized manner.
On the other hand, graphs provide a flexible way of capturing relationships between different data points, where nodes (also known as vertices) are connected by edges. This offers a powerful tool for representing complex networks, social connections, or even geographic maps. By mastering graphs in JavaScript, you'll gain the ability to efficiently traverse, search, and manipulate interconnected data, opening up endless possibilities for solving intricate problems.
By gaining expertise in trees and graphs, you will be equipped with efficient and versatile tools to solve a wide range of programming challenges. Whether you're working on large-scale data analysis, optimizing code performance, or building complex algorithms, a solid foundation in these data structures will enable you to tackle even the most intricate problems with ease.
FAQ
Q: What are some commonly used data structures in Javascript?
A: Some commonly used data structures in Javascript are arrays, objects, linked lists, stacks, queues, trees, and graphs.
Q: How can I efficiently store and manipulate data in Javascript?
A: You can efficiently store and manipulate data in Javascript by utilizing appropriate data structures such as arrays and objects. Arrays are useful for storing collections of data, while objects allow you to store data in key-value pairs.
Q: What are the advantages of linked lists, stacks, and queues?
A: Linked lists offer efficient memory management and are particularly useful when dealing with large amounts of data. Stacks and queues, on the other hand, help in handling data in a specific order. Stacks follow the Last In First Out (LIFO) principle, while queues follow the First In First Out (FIFO) principle.
Q: How can I implement linked lists, stacks, and queues in Javascript?
A: You can implement linked lists, stacks, and queues in Javascript by creating custom classes or using built-in data structures and methods provided by the language.
Q: What are trees and graphs, and why are they important in Javascript?
A: Trees are hierarchical data structures that allow for efficient searching and organizing of data. Graphs, on the other hand, represent complex relationships between data points. Trees and graphs are important in Javascript as they enable you to solve intricate problems and optimize your code for maximum efficiency.
Q: How can I traverse, search, and manipulate trees and graphs in Javascript?
A: You can traverse, search, and manipulate trees and graphs in Javascript by implementing algorithms such as depth-first search (DFS) and breadth-first search (BFS). Additionally, you can use recursive techniques and various traversal methods to perform operations on these data structures.