Error handling in JavaScript is a crucial aspect of developing robust and reliable applications. By effectively managing errors, developers can ensure smooth user experiences, prevent crashes, and maintain the integrity of their code. In this article, we will explore different techniques and best practices for handling errors in JavaScript.
One of the primary methods for error handling in JavaScript is the use of try-catch blocks. This allows developers to capture and handle errors that occur during the execution of their code. The try block contains the code that may throw an error, while the catch block handles any errors that are caught. By handling errors gracefully, developers can provide meaningful error messages to users and prevent the application from crashing.
Javascript error management is essential for maintaining the stability and reliability of applications. By identifying and handling errors effectively, developers can ensure that their code runs smoothly and correctly. It is also essential to implement best practices for error handling, such as logging errors and reporting them for debugging purposes.
In the following sections, we will dive deeper into error handling best practices, error logging and reporting techniques, and conclude with a summary of the key takeaways. By mastering error handling in JavaScript, developers can enhance the performance and reliability of their applications.
Best Practices for Error Handling in JavaScript
In order to write robust and reliable JavaScript code, it is essential to implement best practices for error handling. By effectively managing errors, developers can ensure a smooth user experience, prevent crashes, and facilitate the debugging process. This section explores some of the key techniques and recommendations for error handling in JavaScript.
1. Logging Errors
One of the fundamental practices in error handling is logging errors. By capturing error information, developers can gain valuable insights into the nature and cause of errors. Logging can assist in identifying recurring issues, tracking error trends, and facilitating debugging efforts. It is recommended to use a dedicated logging library or create a custom logging mechanism to capture and store error data effectively.
2. Displaying Error Messages
When an error occurs, it is important to provide meaningful error messages to users. Clear and concise error messages help users understand what went wrong and how to resolve the issue. Avoid exposing sensitive information in error messages and maintain a user-friendly tone. Consider providing additional guidance or suggestions for error resolution, if applicable.
3. Handling Exceptions
Exception handling is a critical aspect of error management in JavaScript. Using try-catch blocks allows developers to catch and handle exceptions gracefully. It enables the execution of fallback code or alternative pathways when errors occur. It is recommended to handle specific types of exceptions using separate catch blocks to provide specific error handling logic for different scenarios.
"Good error handling not only improves the reliability and stability of your code but also enhances the overall user experience of your application."
4. Graceful Degradation
Implementing graceful degradation is another best practice for error handling. This approach involves anticipating potential errors and implementing fallback mechanisms to ensure the application can continue functioning even if certain features or functionalities encounter errors. By gracefully degrading the application's behavior, developers can prevent crashes and provide a smoother experience to users.
5. Testing and Debugging
Thorough testing and debugging are crucial steps in error handling. Regularly test your code and simulate various error scenarios to ensure you have adequate error handling mechanisms in place. Utilize debugging tools and techniques to pinpoint and resolve errors efficiently. Incorporate automated testing frameworks and tools to streamline the error identification and resolution process.
By adhering to these best practices and incorporating them into your JavaScript development process, you can enhance the reliability, stability, and user experience of your applications.
Best Practices for Error Handling in JavaScript | Description |
---|---|
Logging Errors | Implement a robust logging mechanism to capture and store error data |
Displaying Error Messages | Provide clear and concise error messages to users for effective troubleshooting |
Handling Exceptions | Use try-catch blocks to catch and handle exceptions gracefully |
Graceful Degradation | Implement fallback mechanisms to ensure the application continues to function despite errors |
Testing and Debugging | Thoroughly test and debug your code to identify and resolve errors |
Error Logging and Reporting in JavaScript
When it comes to building robust JavaScript applications, error logging and reporting play a crucial role in identifying and resolving issues. By logging errors, developers can gain insights into potential bugs, application crashes, and unexpected behavior. This section explores the importance of error logging for debugging purposes and discusses various error logging techniques in JavaScript.
Importance of Error Logging
Effective error logging serves as a valuable tool for developers to track and troubleshoot issues in JavaScript applications. By recording error messages, stack traces, and other relevant information, developers can identify patterns and gain a deeper understanding of the root causes of errors.
Some of the key benefits of error logging in JavaScript include:
- Improving Debugging: Error logs provide developers with a detailed record of encountered issues, making it easier to reproduce and debug errors.
- Enhancing Stability: By monitoring and addressing logged errors, developers can enhance the stability and reliability of their applications.
- Identifying Critical Issues: Error logs highlight critical errors that need immediate attention, allowing developers to prioritize and address them accordingly.
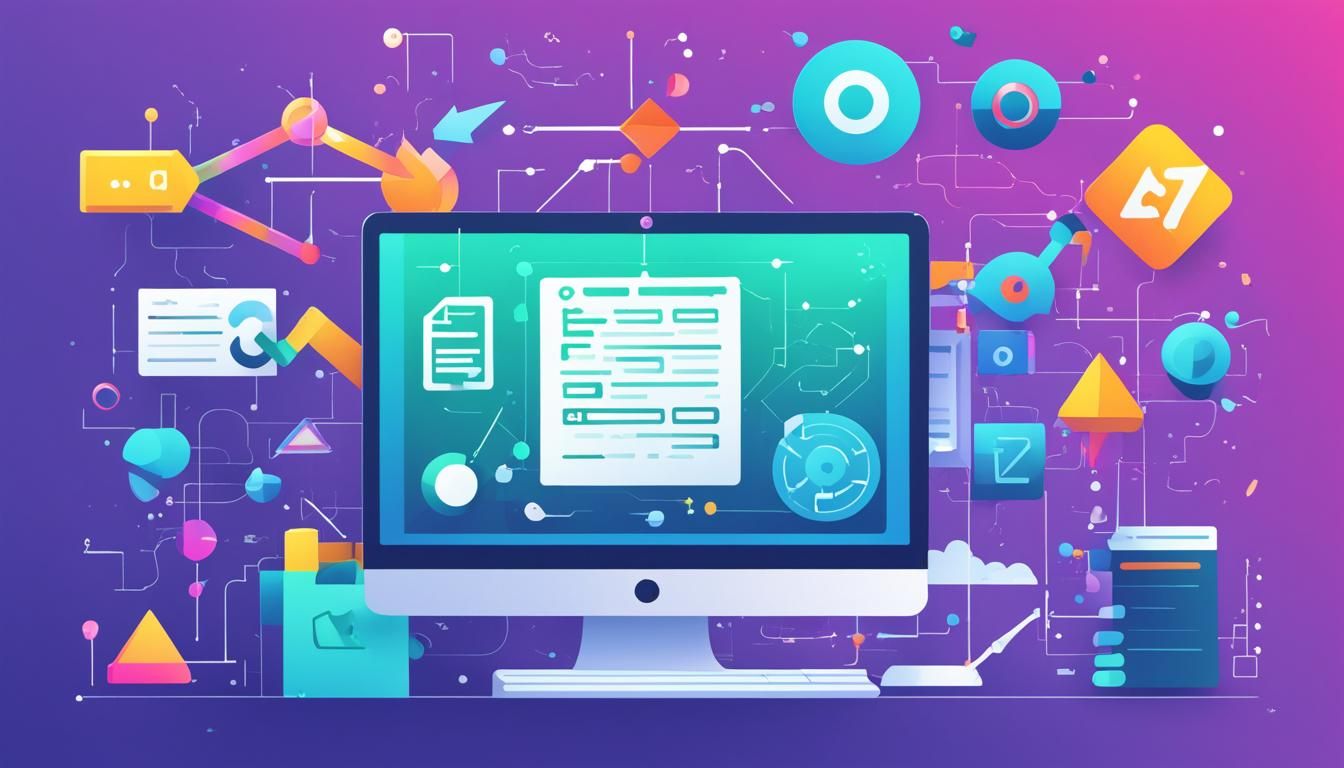
Error Logging Techniques
JavaScript offers several error logging techniques that developers can employ to capture and track errors efficiently. Common error logging techniques include:
- Console Logging: Using console.log or console.error statements, developers can log errors and other relevant information to the browser console for real-time debugging.
- Error Event Listeners: By attaching error event listeners to the window object, developers can capture unhandled errors and log them to a remote server or the browser console.
- Error Tracking Libraries: Utilizing error tracking libraries, such as Sentry or Bugsnag, developers can gain comprehensive insights into errors, including error frequencies, affected users, and associated stack traces, enabling efficient bug resolution.
Error Reporting and Monitoring Tools
To simplify error reporting and monitoring in JavaScript applications, a variety of tools and services are available. These tools provide comprehensive error tracking features, real-time alerting, and advanced analytics to help developers effectively manage errors. Some popular error reporting tools include:
- Rollbar: Rollbar offers real-time error monitoring and alerting, allowing developers to identify, track, and resolve errors before they impact users.
- Sentry: Sentry is a powerful error tracking platform that provides detailed error reports, event aggregation, and release tracking to help developers identify and resolve issues quickly.
- Bugsnag: Bugsnag offers real-time error monitoring and crash reporting, along with intelligent error grouping and comprehensive error analytics to support efficient debugging.
By utilizing these specialized tools, developers can streamline the process of error logging, reporting, and resolution, saving valuable time and resources.
With the right error logging and reporting practices in place, developers can proactively address issues, improve the overall quality of their applications, and deliver a seamless user experience.
Conclusion
In conclusion, mastering error handling in JavaScript is crucial for writing reliable and maintainable code. By effectively managing errors, developers can prevent crashes and ensure a smooth user experience.
Throughout this article, we discussed the best practices for error handling in JavaScript. We explored techniques such as logging errors, displaying error messages, and handling exceptions gracefully. These practices not only help in identifying and resolving errors quickly but also aid in debugging and improving the overall quality of JavaScript applications.
It is important for developers to stay vigilant in managing errors effectively. By following the discussed best practices, they can ensure that their code is robust and resilient. Additionally, leveraging tools and libraries available for error logging and reporting can further enhance the error handling process.
FAQ
Q: What is error handling and why is it important in JavaScript?
A: Error handling in JavaScript refers to the process of managing and responding to errors that occur during the execution of a JavaScript program. It is important because it helps in identifying and resolving errors, enhancing the overall user experience, and ensuring the robustness and reliability of the code.
Q: What are some common error handling techniques in JavaScript?
A: Some common error handling techniques in JavaScript include using try-catch blocks to catch and handle specific types of errors, using error objects to obtain detailed information about an error, and using the throw statement to create custom errors.
Q: How can I display error messages to users in JavaScript?
A: To display error messages to users in JavaScript, you can use the alert() function to show a pop-up alert with a custom error message, or you can dynamically update the HTML content of an element on the webpage to display the error message.
Q: What are some best practices for error handling in JavaScript?
A: Some best practices for error handling in JavaScript include logging errors to a central system for tracking and debugging, providing meaningful error messages that help users understand the issue, and handling exceptions gracefully to prevent crashes or unexpected behavior.
Q: How can I log errors in JavaScript?
A: You can log errors in JavaScript by using console.log() to output error messages to the console, or by integrating a logging library such as log4javascript or Winston to log errors to a file or a remote server for better error tracking and analysis.
Q: Are there any tools available for error reporting and tracking in JavaScript applications?
A: Yes, there are various tools and libraries available for error reporting and tracking in JavaScript applications. Some popular tools include Sentry, TrackJS, and Bugsnag, which provide features like real-time error monitoring, smart error grouping, and issue tracking to help developers track, analyze, and resolve errors more efficiently.