In the world of web development, event handling plays a crucial role in creating dynamic and interactive user experiences. Whether it's responding to a button click, hovering over an element, or submitting a form, JavaScript event handling empowers developers to capture and respond to these user interactions effectively.
In this comprehensive tutorial, we will explore the ins and outs of event handling in JavaScript. From understanding event listeners and their significance in handling events, to diving into event delegation and event bubbling, we will equip you with the knowledge and skills to become a master of event handling.
Event handling in JavaScript involves listening for specific events, such as clicks or keypresses, and executing corresponding code when these events occur. By utilizing event listeners, developers can create dynamic responses to user actions, enhancing the functionality and interactivity of web pages.
Event delegation and event bubbling are two essential concepts that complement event handling. Event delegation allows developers to handle events on parent elements rather than individual child elements, resulting in more efficient and manageable code. On the other hand, event bubbling enables events to propagate through the DOM hierarchy, allowing multiple elements to respond to the same event. Understanding these concepts is crucial for effective event handling in JavaScript.
Throughout this tutorial, we will also discuss best practices for event handling, providing you with valuable tips and techniques to optimize your code and enhance the performance of your web applications.
Whether you are a beginner looking to grasp the fundamentals of event handling or an experienced developer aiming to level up your skills, this tutorial has got you covered. Let's dive into the world of event handling in JavaScript and unlock its potential for creating seamless and interactive user experiences.
Understanding JavaScript Event Listeners
In this section, we will delve deeper into the world of event handling in JavaScript and explore the significance of JavaScript event listeners. Event listeners play a crucial role in the dynamic behavior of web pages by allowing developers to detect and respond to specific user actions. By attaching event listeners to HTML elements, developers can create interactive and responsive web interfaces.
JavaScript event listeners are functions that listen for a specific event to occur and then trigger a response or execute a set of instructions. These events can be user-initiated actions, such as clicking a button, hovering over an element, or submitting a form. Event listeners can be attached to any HTML element, such as buttons, links, input fields, or even the entire document.
Event listeners are added to HTML elements using the addEventListener()
method, which takes two parameters: the event type and the function that should be executed when the event occurs. Here's an example:
<button id="myButton">Click Me</button>
<script>
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button clicked!');
});
</script>
In the example above, an event listener is added to a button element with the ID 'myButton'. When the button is clicked, the function provided as the second parameter of the addEventListener()
method is executed, which displays an alert with the message 'Button clicked!'. This demonstrates how event listeners can be used to respond to user interactions in real-time.
JavaScript provides a wide range of event types that can be listened for. Some commonly used event types include:
- click: Fires when a user clicks on an element.
- mouseover: Fires when a user hovers over an element.
- submit: Fires when a form is submitted.
- keydown: Fires when a user presses a key on the keyboard.
- load: Fires when a page or an image finishes loading.
It is important to note that event listeners can be attached to multiple elements, allowing developers to handle events on a group of related elements without duplicating code. This concept is known as event delegation and is often used to optimize event handling in JavaScript.
When utilizing JavaScript event listeners, it is essential to follow best practices to ensure efficient and maintainable code. Here are some event handling best practices:
- Keep event handling code separate: Separate your event handling code from other JavaScript functions to maintain a clear code structure.
- Use event delegation when appropriate: If you have multiple elements that require the same event handling, utilize event delegation to attach a single event listener to a parent element.
- Remove event listeners when no longer needed: If an element or group of elements no longer requires event handling, make sure to remove the associated event listeners to prevent memory leaks.
- Optimize performance: Be mindful of the impact of event handling on performance. Avoid attaching excessive event listeners or performing resource-intensive tasks within event callbacks.
By following these best practices, developers can create efficient and robust event handling systems that enhance user interactions on web pages.
Next, we will explore event delegation and event bubbling in JavaScript, which are advanced techniques for managing events.
Summary Table: JavaScript Event Listeners
Event Type | Description |
---|---|
click | Occurs when a user clicks on an element. |
mouseover | Triggers when a user hovers over an element. |
submit | Fires when a form is submitted. |
keydown | Triggered when a user presses a key on the keyboard. |
load | Fires when a page or an image finishes loading. |
Event Delegation and Event Bubbling in JavaScript
Event delegation and event bubbling are fundamental concepts in JavaScript event handling. By understanding these concepts, developers can effectively manage events and optimize their code. This section will explore event delegation and event bubbling in JavaScript, providing insights into their practical applications.
Event delegation in JavaScript allows developers to handle events on parent elements instead of individually assigning event listeners to each child element. This technique offers several benefits, including improved performance and reduced memory consumption. By leveraging event delegation, developers can streamline event handling and efficiently manage dynamic content.
Event bubbling in JavaScript refers to the process by which events propagate through the Document Object Model (DOM) hierarchy. When an event occurs on a specific element, it triggers the same event on its parent elements, propagating up the DOM tree. This mechanism enables developers to capture events at higher-level elements and respond accordingly. By utilizing event bubbling, developers can simplify event handling and enhance code modularity.
Let's illustrate these concepts with an example. Consider a scenario where a list of items is displayed on a web page. Each item has a delete button to remove it from the list. Instead of attaching event listeners to each individual delete button, event delegation allows us to attach a single event listener to the parent container element:
Example: Event delegation
const itemList = document.getElementById('item-list');
itemList.addEventListener('click', function(event) {
if (event.target.classList.contains('delete-button')) {
// Delete the item
const item = event.target.closest('.item');
item.remove();
}
});
In the code snippet above, the event listener is attached to the itemList
element, which is the parent container. When a click event occurs, the listener checks if the clicked element has the class delete-button
. If it does, the corresponding item is deleted from the list. This way, we avoid attaching event listeners to each delete button individually and instead handle the event at the parent level.
On the other hand, event bubbling allows us to capture events at higher-level elements as they propagate through the DOM hierarchy. This enables us to handle events for multiple related elements efficiently. Let's take a look at an example that demonstrates event bubbling:
Example: Event bubbling
const container = document.getElementById('container');
container.addEventListener('click', function(event) {
if (event.target.matches('.nested')) {
// Handle the event for nested elements
console.log('Nested element clicked!');
} else if (event.target.matches('.container')) {
// Handle the event for the container element
console.log('Container element clicked!');
}
});
In the code snippet above, the event listener is attached to the container
element. When a click event occurs on a nested element with the class nested
, the listener logs "Nested element clicked!" to the console. If the click event occurs on the container element itself (with the class container
), the listener logs "Container element clicked!". By leveraging event bubbling, we can handle events for both the nested elements and the container element with a single event listener.
Event Delegation in JavaScript | Event Bubbling in JavaScript |
---|---|
Allows handling events on parent elements | Enables events to propagate through the DOM hierarchy |
Improves performance and memory consumption | Simplifies event handling and enhances code modularity |
Efficiently manages dynamic content | Captures events at higher-level elements |
By understanding event delegation and event bubbling, developers can optimize their JavaScript code and enhance the interactivity of their web applications. These concepts offer efficient ways to handle events, improving performance and code maintainability. Experimenting with event delegation and event bubbling techniques will empower developers to build robust and responsive web interfaces.
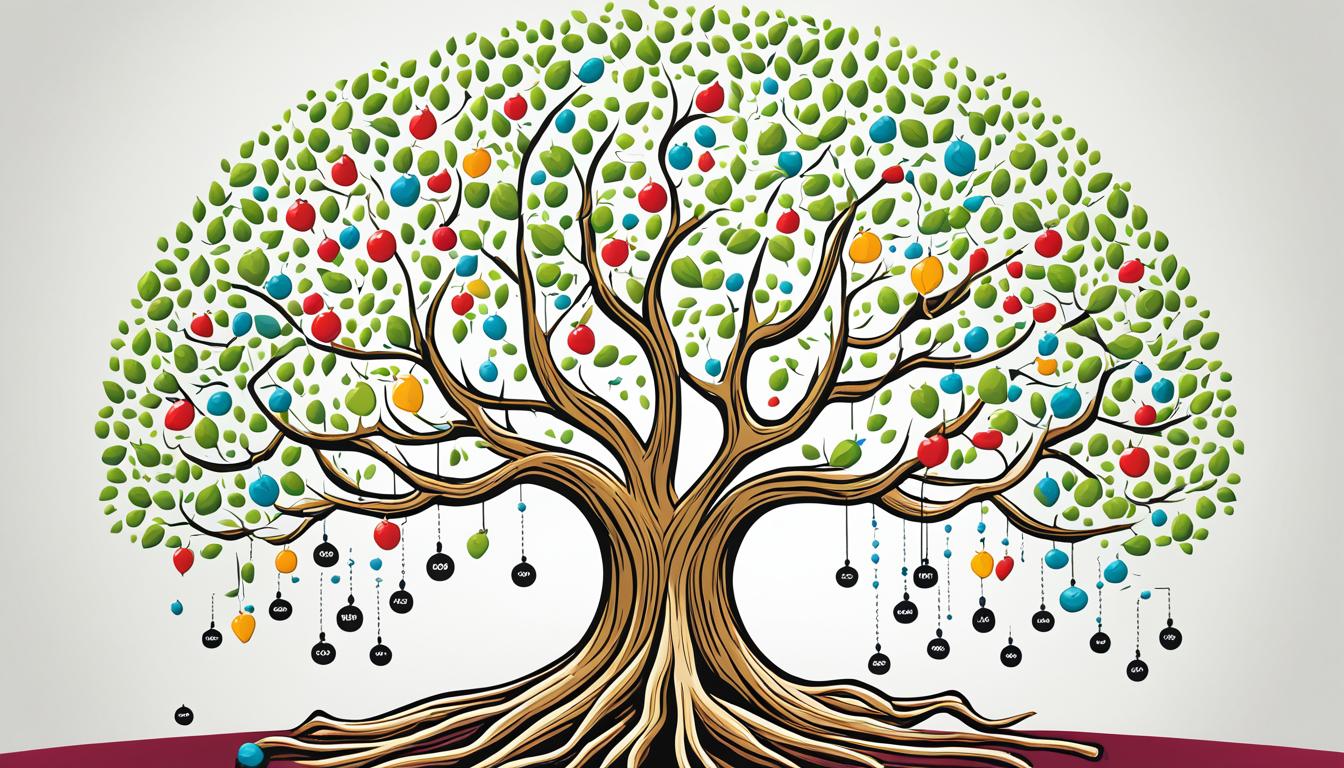
Conclusion
In this article, we have explored the essentials of event handling in JavaScript. We started by understanding the role of event listeners in detecting and responding to user interactions on web pages. By attaching event listeners to HTML elements, developers can create dynamic and interactive experiences for their users.
We then delved into event delegation, a powerful concept that allows handling events on parent elements instead of individual child elements. By utilizing event delegation, developers can optimize their code and improve performance by reducing the number of event listeners required.
Lastly, we discussed event bubbling, which enables events to propagate through the DOM hierarchy. Event bubbling can be leveraged to handle events at multiple levels of the DOM, providing more flexibility in event handling.
By following the best practices highlighted throughout the article, developers can ensure effective event handling in their JavaScript applications. With a solid understanding of event listeners, event delegation, and event bubbling, they can create seamless and interactive user experiences on their web pages.
FAQ
Q: What is event handling in JavaScript?
A: Event handling in JavaScript refers to the process of detecting and responding to user interactions, such as clicks, mouse movements, or keyboard actions, on a web page. By using event listeners, developers can associate specific actions or functions with these events, creating dynamic and interactive web experiences.
Q: What are JavaScript event listeners?
A: JavaScript event listeners are functions that are attached to HTML elements to detect and respond to specific events. They "listen" for a particular user action, such as a click or a submit, and execute the associated code when the event occurs. Event listeners can be added to individual elements or applied globally to the entire document.
Q: What is event delegation in JavaScript?
A: Event delegation in JavaScript is a technique that involves attaching event listeners to a parent element instead of handling events on individual child elements. This approach allows for more efficient event handling, especially in cases where there are multiple child elements involved. The event listener on the parent can then use event propagation to determine which specific child element triggered the event.
Q: What is event bubbling in JavaScript?
A: Event bubbling in JavaScript is a mechanism where an event triggered on a nested HTML element propagates up through its parent elements in the DOM hierarchy. This means that when an event occurs on a child element, the event is also triggered on its parent elements sequentially. Event bubbling can be useful in scenarios where you want an event to be handled by multiple elements in a nested structure.
Q: What are some best practices for event handling in JavaScript?
A: Here are some best practices for event handling in JavaScript: - Use event delegation whenever possible to minimize the number of event listeners and improve performance. - Avoid adding unnecessary event listeners to the document or body element. - Separate your JavaScript logic from your HTML markup by using unobtrusive event handling. - Consider using event.stopPropagation() to prevent event bubbling when necessary. - Always clean up event listeners when they are no longer needed to avoid memory leaks. - Utilize event delegation to handle events on dynamic or newly created elements. - Be mindful of event order and consider using event.preventDefault() to prevent default browser behavior when required. - Employ asynchronous event handling techniques, such as promises or async/await, to handle events that rely on asynchronous operations.
Q: Is there a tutorial on JavaScript event handling?
A: Yes, we have a beginner-friendly tutorial on JavaScript event handling. It covers the basics of adding event listeners, handling events with functions, and demonstrates practical examples of event delegation and event bubbling. The tutorial will guide you through the process step-by-step, helping you gain a solid understanding of event handling in JavaScript.