Node JS Fetch
The Node JS Fetch (experimental) is a global fetch api that is native to NodeJS v18+ and provides a way to retrieve resources from other servers using the HTTP protocol. The implementation comes from undici and is inspired by node-fetch which was originally based upon undici-fetch. It can be used to fetch resources across the network, and to process the response data.
// NodeJS Fetch Example using async/wait
const getDocs = async() => {
const res = await fetch('https://nodejs.org/api/documentation.json')
if (res.ok) {
const data = await res.json()
console.log(data)
}
}
// NodeJS Fetch Example using promises
const getDocs = () => {
fetch('https://nodejs.org/api/documentation.json')
.then(response => response.json())
.then(data => console.log(data))
.catch(error => console.log(errror)
}
NodeJS Test Runner (Experimental)
The NodeJS Test Runner is a tool that helps you write and run unit tests for your NodeJS applications. It allows you to simply run tests and provides features such as assertion support and code coverage reporting. This provides a native alternative to Jest, Supertest, MochaJS and others.
import test from 'node:test';
import assert from 'node:assert';
test('synchronous passing test', (t) => {
// This test does not throw an exception.
// PASS
assert.strictEqual(1, 1);
});
test('synchronous failing test', (t) => {
// This test throws an exception.
// FAIL
assert.strictEqual(1, 2);
});
Using the test()
method allows subtests to be created, and like the main test, they can be async
in order to use promises. Subtests follow the familiar structure of other test packages.
test('top level test', async (t) => {
await t.test('subtest 1', (t) => {
assert.strictEqual(1, 1);
});
await t.test('subtest 2', (t) => {
assert.strictEqual(2, 2);
});
});
NodeJS Test Runner is a full feature, native, and easy to use and will hopefully become full featured soon.
V8 Support
The V8 JavaScript engine is a powerful tool that provides support for event-driven, asynchronous applications. It offers a wide range of features and options, making it the perfect choice for NodeJS development. NodeJS v18 uses version 10.1 of V8 engine, which provides:
- Added
findLast
andfindLastIndex
methods on arrays. - Additional Improvements in
Intl.Locale
API. - The
Intl.supportedValuesOf
function.
What is NodeJS?
Node JS has released the new version of Node, version 18 and it offers a lot of recent updates for developers to take advantage of. These new features are extremely useful for developers as they help improve productivity and performance.
NodeJS is a platform built on Chrome's JavaScript runtime for easily building fast, scalable network applications. NodeJS uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
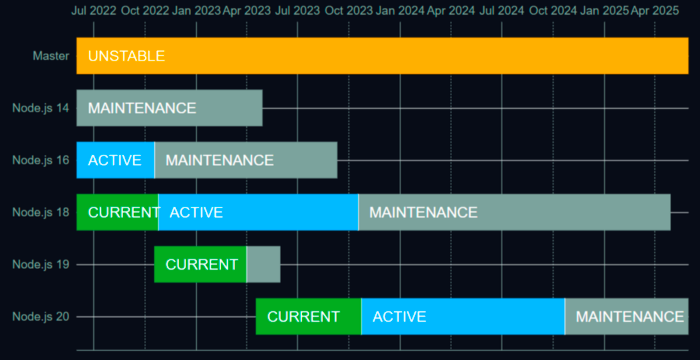
Conclusion
NodeJS is increasingly gaining popularity for its asynchronous and event-driven programming model. This, along with its ability to execute on the server-side, makes it an attractive platform for developing web applications. The NodeJS Foundation has been working to improve the quality of the NodeJS codebase and ecosystem. In this article, we looked at some of the recent improvements to the NodeJS Fetch API, Test Runner, and V8 Support.