Welcome to the world of Javascript! In this article, we will dive deep into the concepts of closure and scopes in Javascript. These topics play a crucial role in understanding how Javascript works and are essential for any developer looking to write efficient and organized code.
Before we proceed, let's take a moment to define what we mean by closure and scopes in Javascript. Closures refer to the ability of a function to retain access to variables from its outer scope, even after the function has finished executing. On the other hand, scopes determine the visibility and accessibility of variables within a specific part of the code.
In the following sections, we will explore how closures work and their significance in Javascript. We will also delve into the scope chain and how it affects the access to variables. Furthermore, we will discuss lexical scope and nested functions, which are fundamental concepts related to closures and scopes in Javascript.
To provide you with a comprehensive understanding, we will cover these topics in a step-by-step manner. By the end of this article, you will have a solid grasp of closure and scopes, empowering you to write cleaner and more efficient Javascript code that can tackle various programming challenges.
How Closures Work in Javascript
In this section, we will explore the inner workings of closures in Javascript. Closures play a crucial role in the language and provide a powerful tool for developers to manage and manipulate variables within functions.
So, what exactly are closures? A closure is a combination of a function and the lexical environment within which that function was declared. It allows a function to retain access to variables even after it has finished executing. This unique characteristic of closures enables programmers to create more flexible and efficient code.
When a function is defined inside another function, the inner function has access to variables in its outer function. This is because the inner function forms a closure, which encapsulates both the function and its surrounding data, including any variables that were in scope at the time of its creation.
Let's consider an example to illustrate how closures work. Take a look at the following code snippet:
// Defining the outer function
function outerFunction() {
var outerVariable = "I am outside!";
// Defining the inner function
function innerFunction() {
console.log(outerVariable);
}
// Executing the inner function
innerFunction();
}
// Calling the outer function
outerFunction();
When the outerFunction
is called, the innerFunction
is executed within the lexical environment of the outer function. Consequently, the console.log(outerVariable)
statement within the inner function logs "I am outside!" to the console. This is possible because the inner function retains access to the outerVariable
even after the outer function has finished executing.
Closures are incredibly useful in many real-world programming scenarios. They enable developers to control variable access, create private variables, implement data hiding, and achieve encapsulation. Understanding how closures work is fundamental to becoming a proficient Javascript developer.
Benefits of Closures
Closures offer several advantages when it comes to Javascript development. Here are a few key benefits:
- Closures allow for the preservation of variables in memory, even after their respective functions have completed execution.
- They enable the creation of private variables and functions that cannot be accessed from the outside, providing better data protection and security.
- Closures facilitate the creation of higher-order functions, which are functions that can accept other functions as parameters or return functions as values.
- They provide a clean and organized way to manage and manipulate variables, allowing for more efficient and maintainable code.
Understanding the concept of closures and their benefits is crucial for any Javascript developer who wants to write clean, efficient, and robust code.
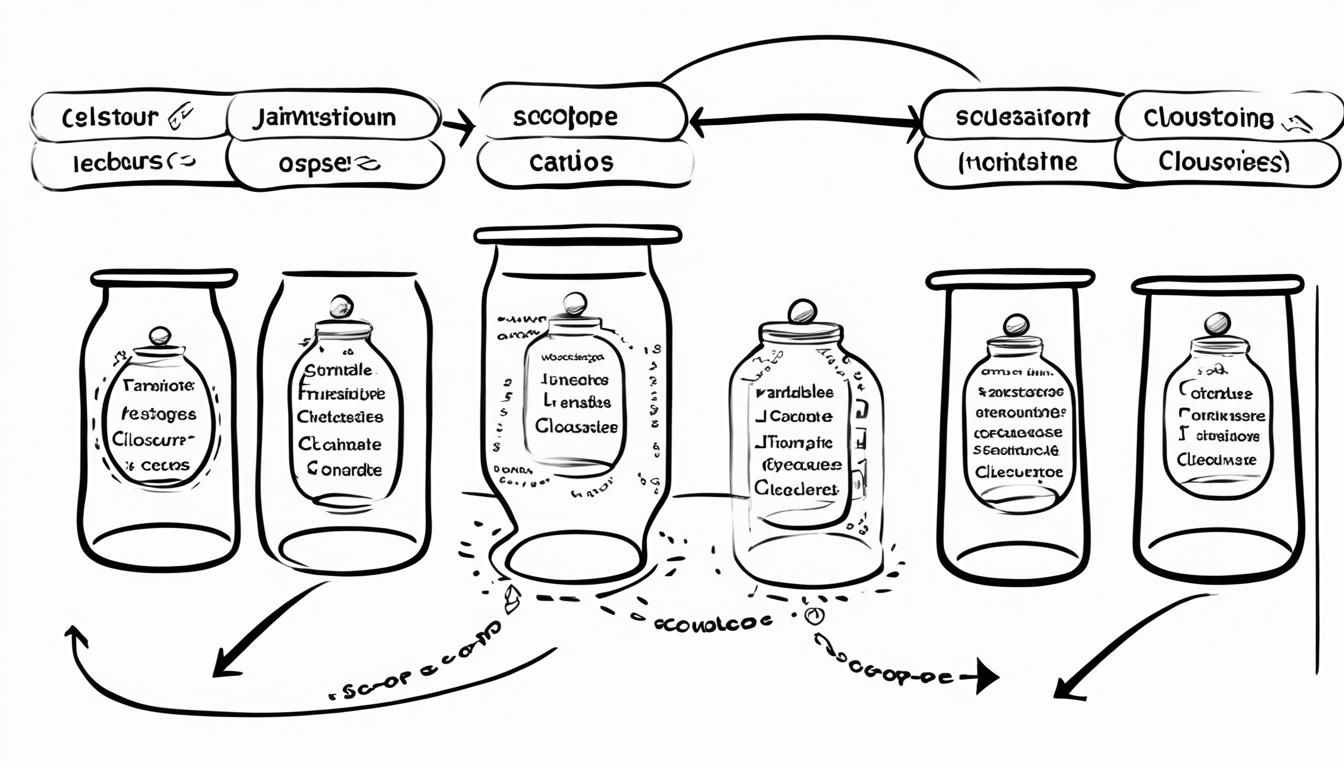
In the next section, we will delve deeper into the scope chain in Javascript, exploring how variables are accessed and resolved based on their location in the code.
Understanding Scope Chain in Javascript
In this section, we will explore the concept of the scope chain in Javascript, which plays a crucial role in determining the accessibility of variables within a program. Understanding how the scope chain works is essential for writing efficient and organized code.
Scopes in Javascript represent the regions within a program where variables and functions are defined and can be accessed. The interpreter follows the scope chain to find a variable's value based on its location in the code.
There are several types of scopes in Javascript:
- Global Scope: Variables defined outside any function have global scope and can be accessed from anywhere in the program.
- Local Scope: Variables defined within a function have local scope and can only be accessed within that function.
- Function Scope: Each function creates its own scope, allowing variables defined within the function to be accessed only within that function.
- Block Scope: Introduced in ES6, block scope is created by using the let and const keywords within blocks of code, such as if statements or loops.
The scope chain is formed by nesting functions or blocks within each other. When the interpreter encounters a variable, it first looks for the variable within the current scope. If the variable is not found, it moves up the scope chain until it reaches the global scope.
Let's understand this concept with an example:
var x = 10; // Global variable
function outer() {
var y = 5; // Local variable
function inner() {
var z = 3; // Inner local variable
console.log(x + y + z); // Accesses global, local, and inner local variables
}
inner();
}
outer(); // Output: 18
The image above visually represents the concept of the scope chain and how variables are accessed within different scopes in Javascript.
Understanding the scope chain is crucial when working with nested functions or complex code structures. It allows us to effectively organize our code and prevent naming conflicts between variables.
Lexical Scope and Nested Functions
In Javascript, lexical scoping is a fundamental concept that allows functions to access variables from their outer scopes. This enables the creation of nested functions, which play a crucial role in organizing code and enhancing its readability. By understanding how lexical scope and nested functions work together, developers can write more efficient and maintainable code.
Lexical scope, also known as static scope, determines the accessibility of variables in a function based on their location in the source code. When a function is defined, it creates its own scope, which includes variables declared within the function using the var
keyword. Additionally, the function has access to variables defined in its outer scopes, such as variables declared in the parent function or the global scope.
The following example demonstrates the concept of lexical scope:
function outerFunction() {
var outerVariable = 'Hello';
function innerFunction() {
var innerVariable = 'World';
console.log(outerVariable + ' ' + innerVariable);
}
innerFunction();
}
outerFunction();
Lexical Scope | Nested Functions |
---|---|
Defines variable accessibility based on location in code | Encapsulate logic and reduce code duplication |
Allows access to variables from outer scopes | Can access both local and outer variables |
Uses parent scope as a fallback if a variable is not found | Improves code modularity and readability |
Conclusion
In conclusion, a thorough understanding of closure and scopes in Javascript is essential for writing efficient and organized code. By exploring the intricacies of closures, the scope chain, lexical scope, and nested functions, developers can gain a deeper understanding of how variables are accessed and manipulated within their code.
Closures, which allow functions to retain access to variables even after they have finished executing, provide a powerful mechanism for encapsulating data and creating modular code. This can lead to more maintainable and reusable applications, as functions can operate independently, yet still interact with the necessary data.
The scope chain in Javascript dictates the accessibility of variables based on their location in the code. Understanding this concept enables developers to avoid conflicts and unintended modifications to variable values. Additionally, developers can leverage lexical scope, which grants functions access to variables in their containing scopes, to write more concise and expressive code.
By mastering closure and scopes in Javascript, developers can enhance their ability to build robust applications. These concepts serve as the foundation for creating clean and organized code, improving code quality, and making debugging and troubleshooting more efficient. With a solid understanding of closure and scopes, developers can unlock the full potential of Javascript and harness its expressive power.
FAQ
Q: What are closures in Javascript?
A: Closures in Javascript are functions bundled together with their surrounding state, including variables and references to code. They allow functions to retain access to variables even after they have finished executing.
Q: How are closures created in Javascript?
A: Closures are created in Javascript when a nested function is defined inside another function, and the nested function references variables from its outer scope. This forms a closure, as the nested function retains access to the variables even after the outer function has returned.
Q: What is the scope chain in Javascript?
A: The scope chain in Javascript refers to the hierarchy of scopes through which the interpreter searches for variables. When a variable is accessed, the interpreter starts from the innermost scope and moves outward until it finds the variable or reaches the global scope.
Q: How does lexical scope work in Javascript?
A: Lexical scope in Javascript determines the accessibility of variables based on their location in the code. Variables defined in an outer scope are accessible to functions and nested functions defined within that scope. Lexical scope ensures that functions have access to the variables they need, even if they are defined in different scopes.
Q: What are nested functions in Javascript?
A: Nested functions in Javascript are functions defined inside another function. These nested functions have access to variables from their outer scope and can be called within the outer function or returned to be called later. They play a significant role in creating closures and implementing lexical scope in Javascript.