In the world of JavaScript programming, control flow plays a vital role in determining the order in which statements are executed. It governs the flow of a program, allowing developers to create logical and efficient code. By understanding control flow in JavaScript, developers can optimize their code and ensure smooth execution.
In this article, we will explore the concept of control flow in JavaScript and its significance. We will delve into the different control flow structures, such as conditional statements and loops, and examine how they can be utilized to enhance the efficiency of your code.
To grasp the full potential of control flow, it is essential to have a clear understanding of its implementation in JavaScript. By the end of this article, you will have a thorough comprehension of control flow, enabling you to write more structured and efficient JavaScript code.
Conditional Statements and Loops in JavaScript
In JavaScript, conditional statements and loops play a crucial role in controlling the flow of code execution. Understanding how to use these control structures effectively can greatly enhance coding efficiency and flexibility. In this section, we will explore the purpose and syntax of conditional statements, various types of loops, and additional control structures that enable developers to handle flow control in JavaScript.
Conditional Statements
Conditional statements allow developers to perform different actions based on different conditions. The most commonly used conditional statement in JavaScript is the if-else statement. The if-else statement evaluates a condition and, if it is true, executes a particular block of code. If the condition is false, it can execute an alternative block of code specified by the else statement.
Another useful conditional statement in JavaScript is the switch statement. The switch statement allows developers to compare a variable or expression to multiple possible values and execute different blocks of code based on the match.
Loops
Loops are used to repeat a block of code multiple times until a certain condition is met. JavaScript provides various types of loops, each designed for specific use cases.
The for loop is commonly used when you know the number of iterations required. It consists of three parts: initialization, condition, and increment or decrement. The code block inside the for loop will be executed as long as the condition is true.
The while loop is useful when the number of iterations is unknown and is determined by a condition. The loop continues as long as the condition is true.
The do-while loop is similar to the while loop, but the code block is executed first before checking the condition. This guarantees that the code block is executed at least once, even if the condition is false from the beginning.
Control Structures
In addition to conditional statements and loops, JavaScript provides control structures that modify the behavior of these control flow elements.
The break statement is used to exit a loop or switch statement prematurely. It allows developers to terminate the loop or switch block before the condition for exiting naturally occurs.
The continue statement is used to skip the current iteration of a loop and move on to the next iteration. It allows developers to bypass certain parts of the code within the loop without terminating it.
By harnessing the power of conditional statements, loops, and control structures, developers can effectively handle flow control in JavaScript and create dynamic and responsive applications.
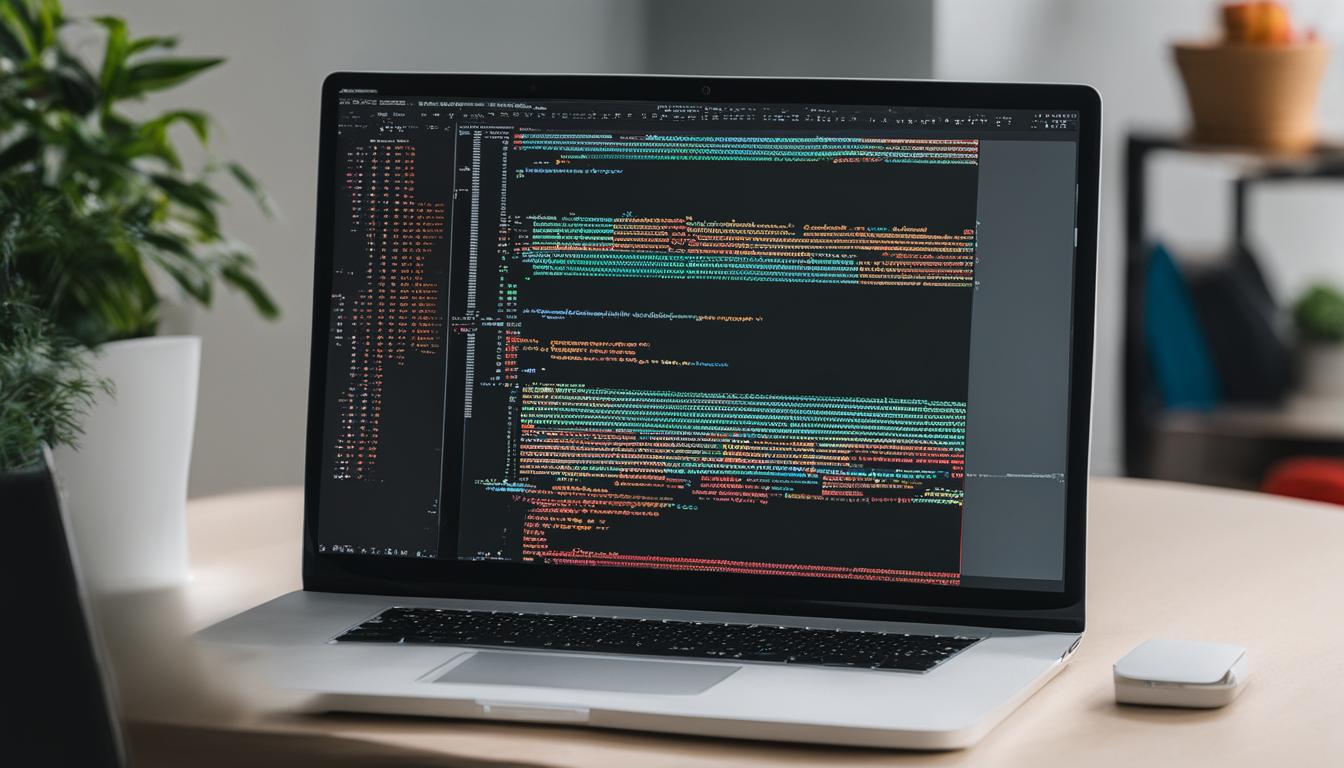
Conclusion
In this article, we have explored the essentials of control flow in JavaScript. Control flow plays a crucial role in determining the sequential execution of code and ensuring that programs run smoothly. By understanding and effectively utilizing control flow mechanisms, developers can create more robust and efficient programs.
We discussed how conditional statements provide a way to make decisions in code based on certain conditions. Whether it's using an if-else statement to execute different blocks of code or leveraging the power of a switch statement to handle multiple cases, conditional statements allow developers to control the flow of their program based on specific conditions.
Additionally, we examined the power of loops in JavaScript. Loops provide a mechanism to repeatedly execute a block of code until a certain condition is met. Whether it's a for loop for a predetermined number of iterations or a while loop that continues until a specific condition is no longer true, loops are invaluable tools for automating repetitive tasks and iterating over collections of data.
Control structures such as break and continue statements can also be utilized to modify the behavior of loops and conditionals. These control structures allow developers to selectively interrupt loop execution or skip iterations based on specific conditions, providing greater flexibility and control over the code execution flow.
FAQ
Q: What is control flow in JavaScript?
A: Control flow refers to the order in which statements and instructions are executed in a program. In JavaScript, control flow determines the flow of execution based on conditions and loops.
Q: What are conditional statements in JavaScript?
A: Conditional statements, such as if-else statements and the switch statement, allow developers to make decisions in their code based on different conditions. These statements execute different blocks of code depending on whether a certain condition is true or false.
Q: How do loops work in JavaScript?
A: Loops in JavaScript, such as for loops, while loops, and do-while loops, repeat a block of code multiple times until a certain condition is met. This provides a way to iterate over arrays, perform repetitive tasks, and handle flow control in a more efficient manner.
Q: What are control structures in JavaScript?
A: Control structures in JavaScript, such as break and continue statements, allow developers to modify the behavior of loops and conditionals. The break statement terminates the execution of a loop or switch statement, while the continue statement skips the rest of the current iteration and proceeds to the next one.
Q: How can control flow enhance coding efficiency?
A: By understanding and properly utilizing control flow structures in JavaScript, developers can create more efficient and robust programs. Control flow structures enable the execution of specific code blocks based on conditions, iteration over arrays, and handling specific scenarios, ultimately leading to better control over the flow of execution and more streamlined code.