In the world of JavaScript, functions play a vital role in writing efficient and modular code. Whether you are a seasoned developer or just starting with JavaScript, understanding how functions work is essential.
Functions in JavaScript allow you to encapsulate reusable blocks of code that can be called upon multiple times throughout your program. They provide a way to organize and structure your code, making it easier to read, understand, and maintain.
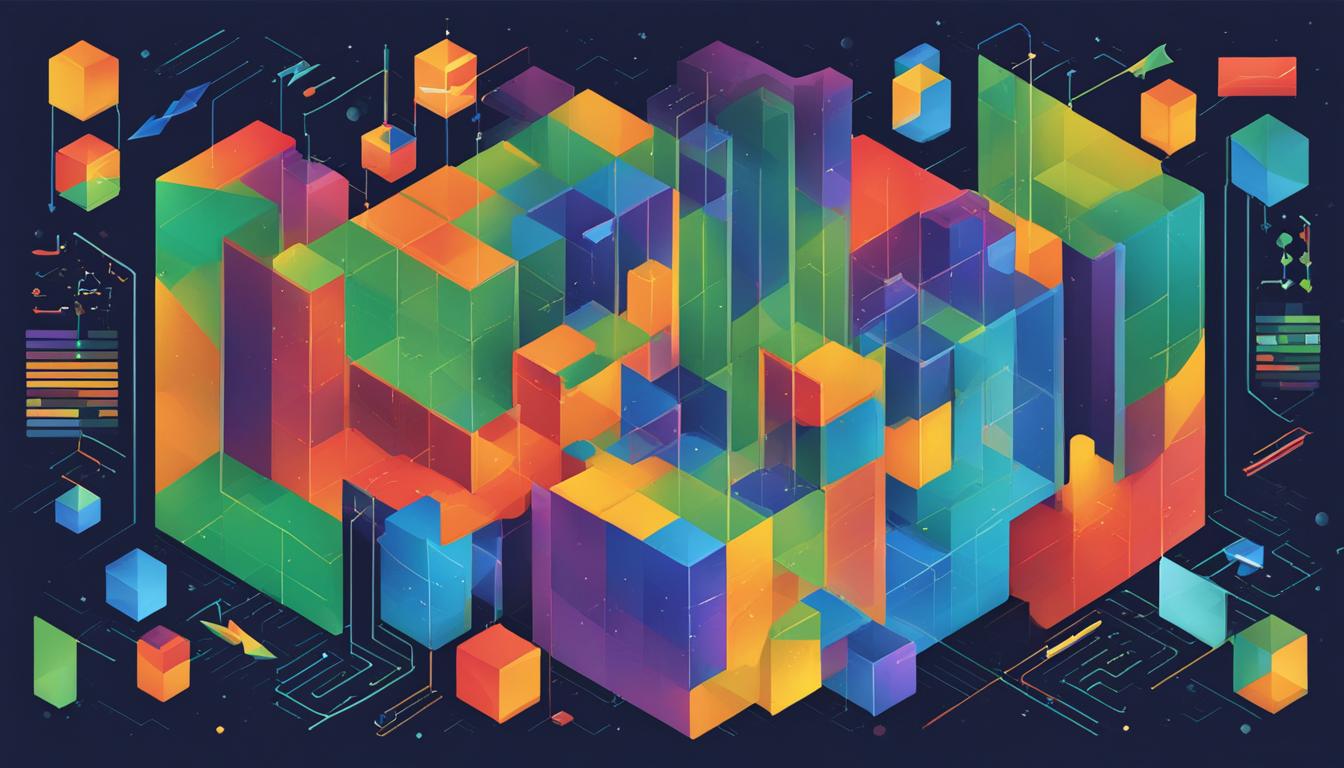
In this section, we will delve into the fundamentals of functions in JavaScript. We will explore the different ways to declare functions, including function declarations, function expressions, and arrow functions. By the end of this section, you will have a solid foundation for creating and implementing functions in your JavaScript projects.
So, let's dive in and explore the essentials of functions in JavaScript.
Exploring Different Types of Functions
In JavaScript, functions are a fundamental building block of code. They allow you to encapsulate reusable blocks of code and make your programs more organized and modular. In this section, we will explore two main types of functions: named functions and anonymous functions.
Named Functions
A named function is defined with a specific name, which allows you to reference and call it by name. They are commonly declared using the function
keyword followed by the chosen name and a set of parentheses. Named functions are useful when you want to reuse the same code block in multiple places or when you need to refer to a function explicitly.
Here is an example of a named function:
<script>
function greet(name) {
return 'Hello, ' + name + '!';
}
var message = greet('John');
console.log(message); // Output: Hello, John!
</script>
In the example above, we define a named function greet
that takes a name
parameter. It returns a greeting message by concatenating the name
with the 'Hello, ' string. We then call the greet
function and pass the argument 'John', which results in the message 'Hello, John!' being logged to the console.
Anonymous Functions
Anonymous functions, also known as function expressions, do not have a specific name. Instead, they are assigned to a variable or passed as an argument to another function directly. They are commonly used in situations where you need to define a function on the fly or when you want to create a function that is only used in a specific context.
Here is an example of an anonymous function:
<script>
var calculate = function(a, b) {
return a + b;
};
var result = calculate(3, 4);
console.log(result); // Output: 7
</script>
In the example above, we assign an anonymous function to the calculate
variable. This function takes two parameters, a
and b
, and returns their sum. We then call the calculate
function with arguments 3 and 4, resulting in the value 7 being logged to the console.
Understanding the differences between named and anonymous functions is crucial for choosing the right approach in different scenarios. Whether you need to reuse a function by name or create a function on the fly, JavaScript provides you with the flexibility to achieve your coding goals.
https://www.youtube.com/watch?v=54NhNhmjQYw
Aspect | Named Functions | Anonymous Functions |
---|---|---|
Declaration | Declared using the function keyword followed by a name | Assigned to a variable or passed as an argument |
Name | Has a specific name | Does not have a specific name |
Reuse | Can be referenced and called by name | Can be invoked through the assigned variable or passed as an argument |
Flexibility | Allows explicit function declaration and clear identification | Offers flexibility in creating functions on the fly or in a specific context |
Understanding Higher-Order and Callback Functions
In JavaScript, higher-order functions and callback functions play a crucial role in writing efficient and flexible code. Let's explore these concepts in detail.
Higher-Order Functions
A higher-order function is a function that can take one or more functions as arguments or return a function as its result. It provides a powerful way to abstract and separate concerns, making your code more modular and reusable.
Here's an example of a higher-order function:
function higherOrderFunc(callback) {
// Perform some operations
callback();
}
In this example, the higherOrderFunc
function takes a callback function as an argument and executes it after performing some operations. This allows you to customize the behavior of the higher-order function by passing different callback functions.
Callback Functions
A callback function is a function that is passed as an argument to another function and is called after a specific event or task completion. It enables you to execute code asynchronously and handle the result or response appropriately.
Here's an example of a callback function:
function callbackFunc() {
// Perform some actions
}
In this example, the callbackFunc
function can be passed as an argument to the higherOrderFunc
function we mentioned earlier. The higherOrderFunc
function will then execute the callbackFunc
function at the appropriate time.
Using higher-order and callback functions allows you to write code that adapts to different scenarios, making it more flexible and reusable. It is especially useful when working with asynchronous tasks, event handling, and data manipulation.
Higher-Order Functions | Callback Functions |
---|---|
Can take one or more functions as arguments or return a function | Passed as arguments to other functions |
Provide a way to abstract and separate concerns | Executed after a specific event or task completion |
Enable code reusability and modularity | Handle asynchronous tasks and event handling |
Understanding higher-order and callback functions is essential for working with JavaScript effectively. These concepts empower you to write code that is more modular, flexible, and capable of handling complex scenarios.
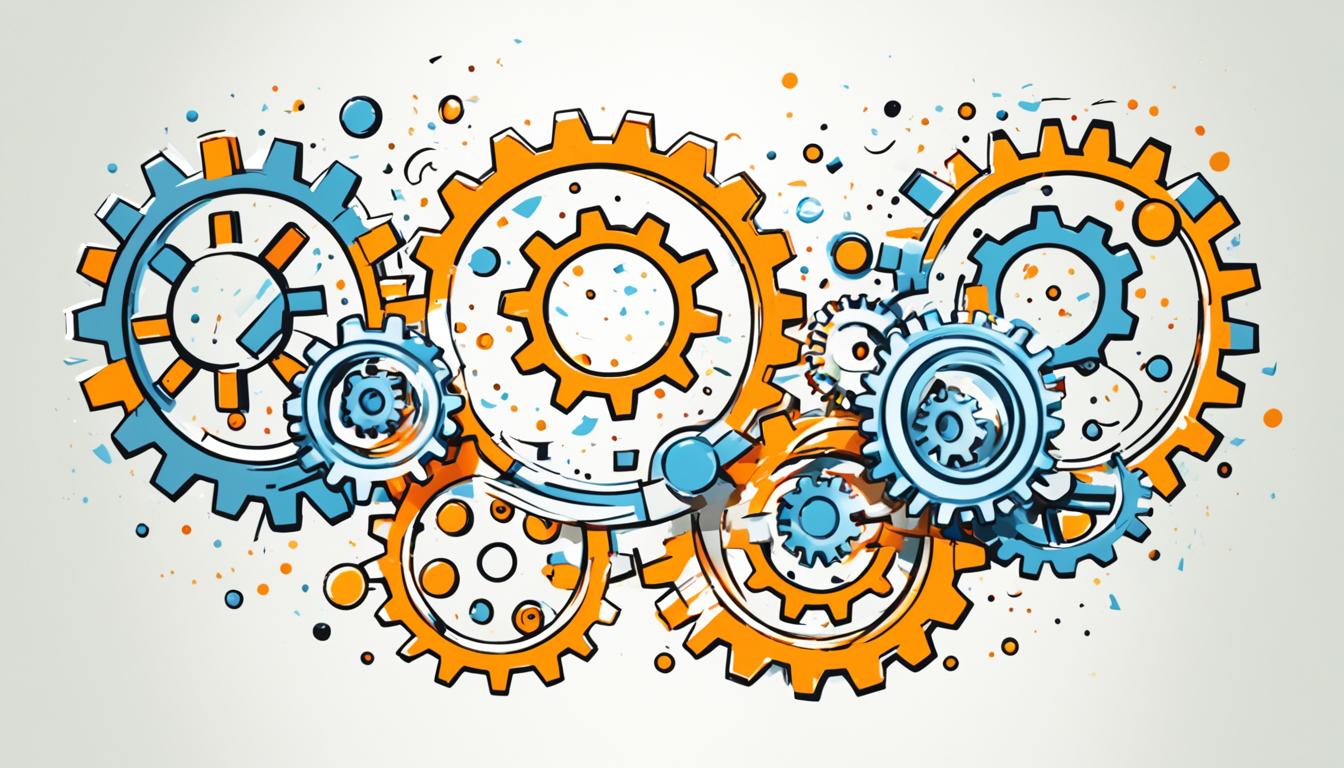
Conclusion
In this article, we have delved into the essentials of functions in JavaScript. We explored the basic concepts of function declarations and expressions, which are fundamental building blocks in JavaScript programming. By understanding these concepts, developers can effectively define and use functions to perform specific tasks and enhance the efficiency of their code.
Furthermore, we discussed more advanced topics like higher-order functions and callback functions. Higher-order functions provide developers with the ability to pass functions as arguments or return them as results, empowering them to create more flexible and reusable code. Callback functions, on the other hand, enable the execution of specific actions at predetermined points, such as after an event or task completion. Incorporating these concepts into your code will allow for the efficient handling of asynchronous operations and the creation of more dynamic web experiences.
By grasping the fundamentals of functions in JavaScript, you can write code that is not only efficient but also modular. Modular code promotes reusability and maintainability, minimizing repetitive code blocks and enhancing overall development productivity. Functions are an integral part of modern JavaScript programming and mastering them will enable you to build robust applications and websites.
FAQ
Q: What are the different ways to declare functions in JavaScript?
A: In JavaScript, there are several ways to declare functions. You can use function declarations, which define a named function using the function keyword, followed by the function name and parentheses. Another option is function expressions, where you can assign a function to a variable. Additionally, you can use arrow functions, which provide a more concise syntax for writing functions.
Q: What are named functions and anonymous functions?
A: Named functions in JavaScript are functions that have a specific name assigned to them. They can be defined using the function keyword followed by the function name. On the other hand, anonymous functions do not have a name and are typically defined using function expressions or arrow functions. Anonymous functions are often used as callbacks or as an inline function when their code is not expected to be reused.
Q: What are higher-order functions and callback functions?
A: Higher-order functions are functions that can take one or more functions as arguments or return a function as a result. They provide a way to abstract and encapsulate reusable behavior. Callback functions, on the other hand, are functions that are passed as arguments to another function and are called after a certain event or completion of a task. They are commonly used in asynchronous programming and can enhance the flexibility and modularity of your code.