When it comes to JavaScript programming, understanding hoisting is crucial. But what exactly is hoisting? In simple terms, hoisting refers to the behavior of moving variable and function declarations to the top of their containing scope during the compilation phase. This means that regardless of where the declarations appear in the code, they are processed before the code is executed.
To illustrate hoisting, let's consider an example:
console.log(hoistedVariable); // Output: undefined
var hoistedVariable = "Hello, hoisting!";
console.log(hoistedVariable); // Output: Hello, hoisting!
In the above code snippet, even though the variable hoistedVariable
is referenced before its declaration, it doesn't throw an error. This is because the declaration is hoisted to the top, giving it the value "undefined" until it is assigned the actual value.
Understanding the rules of hoisting is essential for writing efficient and maintainable JavaScript code. Here are some key rules to keep in mind:
- Variable declarations are hoisted but not their assignments.
- Function declarations are hoisted along with their definitions.
- Only declarations are hoisted, not initializations.
By grasping the concept of hoisting and following best practices, developers can optimize their code and improve their programming practices. It's important to note that while hoisting can be beneficial, it's essential to use it judiciously and maintain code readability.
Continue reading to explore more hoisting examples and rules in the next section.
Exploring Hoisting Examples and Rules
In this section, we will dive deeper into the concept of hoisting in JavaScript by exploring various examples and discussing the rules that govern hoisting behavior. By understanding how hoisting works and the guidelines to follow when using it in code, readers will gain valuable insights into this important aspect of JavaScript programming.
Hoisting in JavaScript refers to the behavior where variable and function declarations are moved to the top of their respective scopes during the compilation phase. This allows developers to use these variables and functions before they are actually declared in the code. It's important to note that only the declarations are hoisted, not the initializations.
Hoisting Examples
Let's consider a few examples to illustrate the concept of hoisting:
Example 1:
In this example, we have a variable declaration num that is initialized with the value 10. We then attempt to log the value of num to the console before the declaration. Despite the declaration being below the console.log statement, the JavaScript engine hoists the declaration, allowing the code to execute without any errors:
console.log(num); // Output: undefined
var num = 10;
console.log(num); // Output: 10Example 2:In this example, we have a function declaration sayHello that is called before it is declared:
sayHello(); // Output: "Hello, there!"
function sayHello() {
console.log("Hello, there!");
}
Example 3:
In this example, we have a function expression sayHi that is assigned to a variable greeting. We then attempt to invoke the function before the assignment:
greeting(); // Output: TypeError: greeting is not a function
var greeting = function sayHi() {
console.log("Hi, there!");
}
Hoisting Rules
Now that we have seen some examples, let's discuss the rules that govern hoisting in JavaScript:
- Variable declarations are hoisted but not their initializations: When a variable is hoisted, its declaration is moved to the top. However, the initialization remains in its original position. This means that the value of the variable will be
undefined
until it is assigned a value. - Function declarations are fully hoisted: Function declarations are hoisted in their entirety, including the function body. This means that the function can be called before it is declared in the code.
- Function expressions are not hoisted: Function expressions, which are assigned to variables, are not hoisted. They must be declared before they are used in the code.
Understanding the rules of hoisting is crucial for writing clean and efficient JavaScript code. By being aware of how hoisting works, developers can avoid unexpected results and ensure their code behaves as intended.
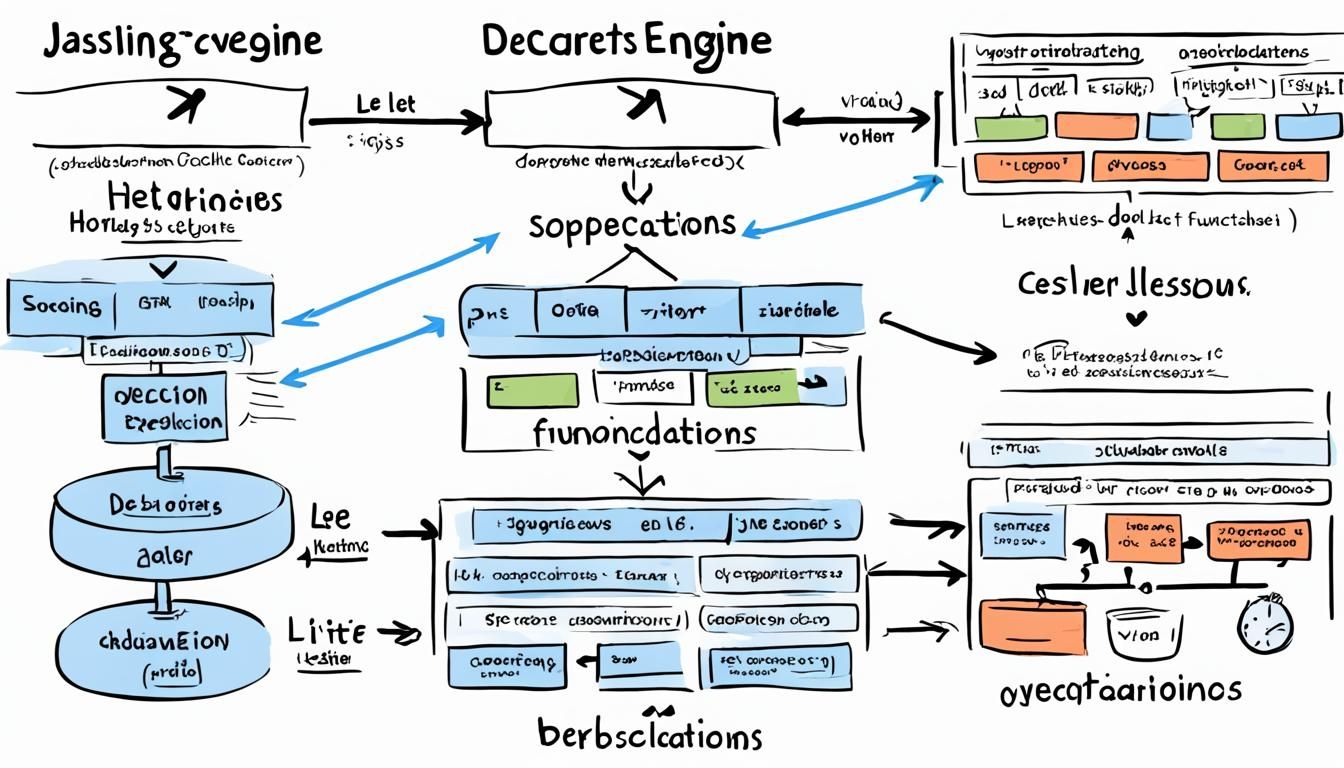
Now that we have explored hoisting examples and discussed the rules, we can move on to the next section to further understand the importance of hoisting in JavaScript development.
The Importance of Understanding Hoisting in JavaScript
Mastering hoisting in JavaScript is crucial for developers. By gaining a strong understanding of hoisting, they can write more efficient and maintainable code, leading to improved software development practices.
Hoisting allows variables and function declarations to be moved to the top of their scope during the compilation phase. This means that even if they are declared later in the code, they can be used before they are declared in the actual execution.
One of the main benefits of hoisting is that it simplifies the code structure. It allows developers to declare variables and functions wherever they are logically needed, instead of being restricted to a specific order. This promotes better code organization and readability.
Another advantage of hoisting is that it can prevent errors caused by the access of undefined variables. Since hoisted variables are automatically initialized with a value of undefined, developers can use them without encountering reference errors. This helps in catching bugs early in the development process.
Understanding hoisting is also essential for writing modular code. By hoisting function declarations to the top, it becomes easier to separate concerns and reuse code across multiple files or modules.
In programming, hoisting refers to the process of moving variables and function declarations to the top of their scope. This allows them to be used before they are actually declared.Understanding hoisting is crucial for writing efficient and maintainable code.
In summary, a solid understanding of hoisting is essential for developers looking to enhance their JavaScript skills. By mastering this concept, they can write more organized, error-free, and modular code, thus improving overall software development practices.
Conclusion
This comprehensive guide has provided valuable insights into the concept of hoisting in JavaScript. By understanding hoisting and following best practices, developers can optimize their coding process and achieve better outcomes in their programming projects.
Hoisting, a fundamental aspect of JavaScript, allows variables and function declarations to be accessed before they are actually defined within a given scope. This understanding can significantly enhance a developer's ability to write clean and efficient code.
By leveraging the power of hoisting, developers can improve the readability of their code by declaring variables and functions at the beginning of their respective scope. This not only ensures smoother code execution but also makes it easier for other developers to understand and maintain the codebase.
Furthermore, by adhering to the rules and best practices of hoisting, developers can avoid unexpected behaviors and minimize potential bugs in their code. Understanding hoisting rules, such as function declarations being hoisted before variable declarations, helps developers write more robust and predictable code.
FAQ
Q: What is hoisting in JavaScript?
A: Hoisting in JavaScript is a mechanism where variable and function declarations are moved to the top of their respective scopes during the compilation phase, before the code execution. This means that regardless of where variables and functions are declared in the code, they can be used before they are declared.
Q: Can you provide some examples to illustrate hoisting?
A: Certainly! Here are a few examples: Example 1:
console.log(x); // Output: undefined
var x = 10;
Example 2:
hoistedFunction(); // Output: "Hello, hoisting!" function
hoistedFunction() {
console.log("Hello, hoisting!");
}
In both examples, even though the variables and functions are used before they are declared, JavaScript moves the declarations to the top, resulting in expected behavior.
Q: What are the rules for hoisting in JavaScript?
A: The rules for hoisting in JavaScript are as follows:
1. Variable declarations are hoisted but not their initializations. This means that variable names are known throughout the scope, but their values are undefined until assigned.
2. Function declarations are hoisted along with their body. This allows you to use functions before they are defined in the code.
3. Function expressions, such as arrow functions and anonymous functions assigned to variables, are not hoisted. They must be defined before being used. It's important to note that hoisting only applies to declarations, not initializations or assignments.
Q: What are the benefits of understanding hoisting in JavaScript?
A: Understanding hoisting in JavaScript is crucial for developers because:
1. It helps avoid unexpected behaviors when using variables and functions.
2. It allows for more flexible code organization by using functions before their actual declarations.
3. It improves code readability and maintainability by making it clear where variables and functions are declared. By grasping the concept of hoisting, developers can write efficient and reliable JavaScript code.
Q: Are there any best practices for using hoisting in JavaScript?
A: Absolutely! Here are some best practices for utilizing hoisting in JavaScript:
1. Always declare variables and functions before using them to avoid ambiguity.
2. Declare variables at the top of their respective scopes to enhance code readability.
3. Avoid relying on hoisting excessively, as it can make the code harder to understand.
4. Clearly separate variable declarations from variable assignments for cleaner code. By adhering to these best practices, developers can harness the power of hoisting while maintaining code quality and clarity.