JavaScript functions are key parts in programming. They let you group code you can use again and again. This is helpful for both new and seasoned developers. Mastering JavaScript functions is key to being good at JavaScript.
We're going to make JavaScript functions easy to get. We'll look into how they're built and used. By the end of this, you'll really know how functions work in JavaScript.
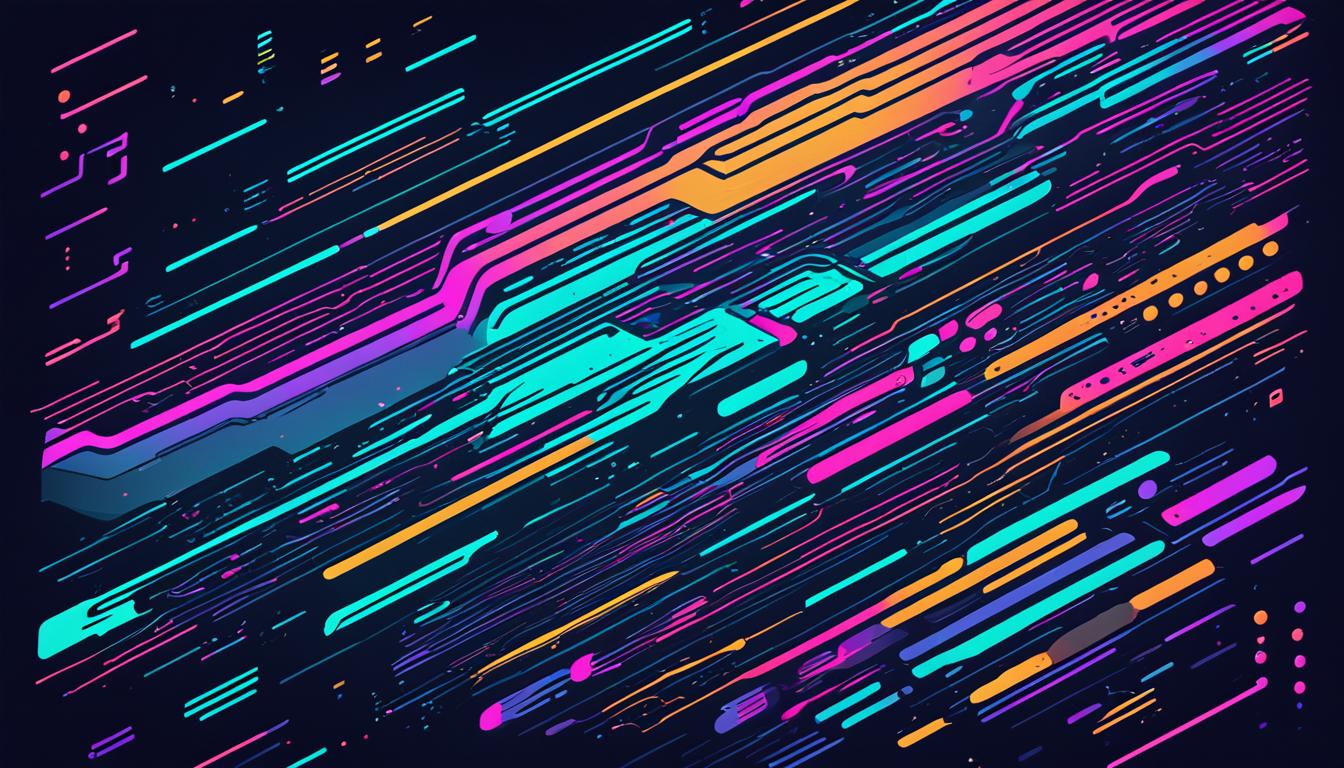
Key Takeaways:
- JavaScript functions are essential building blocks in programming.
- Understanding the syntax of JavaScript functions is crucial for writing effective code.
- Functions allow you to encapsulate and reuse code, improving code organization and maintainability.
- Learning JavaScript functions will enhance your ability to write efficient and well-structured code.
- By the end of this section, you'll have a solid understanding of JavaScript function syntax.
What Are JavaScript Functions?
JavaScript functions are crucial to the JavaScript programming language. They act as reusable code blocks that do specific tasks. They help organize and structure code, making it easy to manage.
A JavaScript function bundles statements and gives them a name. When you call it, it runs its code. Sometimes, it returns a value. Functions can do calculations, change data, or interact with users.
Functions and methods are similar, but there's a small difference. Functions stand alone, while methods are tied to objects or classes. Object-oriented programming uses methods to influence how objects behave.
Thinking of JavaScript functions, imagine them as a cooking recipe. This recipe lists steps to make a dish. Like reusing a recipe, functions allow code reuse.
Functions, like recipes, guide how code runs.
Difference Between Functions and Methods
Functions and methods both perform tasks, but differ slightly. Functions are independent blocks of code, usable anytime. They aren't linked to any object or class.
Methods, on the other hand, are tied to objects or classes. They're used in object-oriented programming to affect an object or class's behavior. You call them using dot notation, like object.method()
or class.method()
.
Essentially, methods are part of something, while functions stand alone.
Consider the difference using a car analogy. Starting the car is a method related to the car object. But, doing math, like finding a square root, uses a standalone function. It's not tied to an object.
Grasping this difference helps programmers write and organize their code better.
JavaScript Function Syntax
JavaScript functions let developers group code into reusable blocks. Understanding their syntax and structure is key. We will look into JavaScript function syntax to help you create and use functions well.
Function Declaration
To declare a JavaScript function, use the function keyword, then add the function name and parentheses (). The function name is how you will call it later. Here's how you declare a function:
function myFunction() { // code to be executed }
The function name here is myFunction. Inside the curly braces {}, you write the code to run when called.
Function Parameters
Parameters make functions more flexible by letting you pass values into them. They go inside the parentheses () of the function name. You can add more than one parameter by separating them with commas. Here is an example:
function greet(name) { console.log("Hello, " + name + "!"); }
In this case, the greet function has a parameter called name. You can give a value for name when you call the function. This value gets used inside the function.
Return Values
Functions can give back values with the return statement. You can save this returned value or use it right away. To return something, use return followed by the value you wish to return. Look at this example:
function multiply(a, b) { return a * b; }
Here, the multiply function accepts two parameters, a and b, and gives back their product. You can use this returned value later or assign it to a variable.
Putting It All Together
With your new understanding of JavaScript function syntax, let's check out an all-inclusive example:
function calculateArea(length, width) { var area = length * width; return area; }
The calculateArea function uses length and width as inputs. It then calculates the area and returns it with a return statement. Now, you're ready to make your own functions thanks to your solid understanding of their syntax. Next, we will dive deeper into JavaScript function parameters.
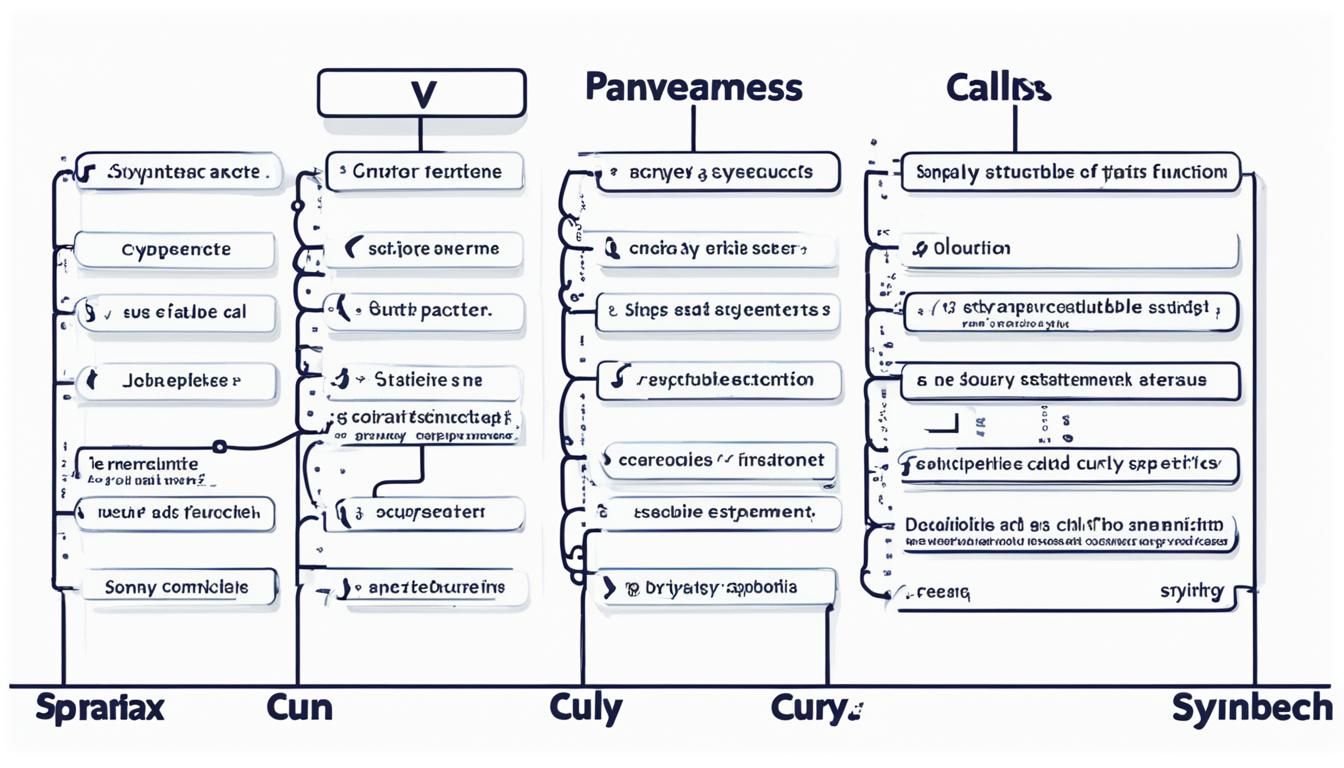
Understanding JavaScript Function Parameters
JavaScript lets you pass values to a function with parameters. These parameters are like variables. They let you use different values each time you call the function. This makes your functions flexible and useful for various tasks.
You can set up a function with one or more parameters between the parentheses. Think of parameters as placeholders for values you'll use later. Here's how you write them:
function functionName(parameter1, parameter2, parameter3) {
// function body
}
You can name JavaScript function parameters anything. But choosing clear names is important. This helps show what they do. Functions can have any number of parameters, from none to many.
When you use a function, you fill in these placeholders. These real values are called arguments. You put them inside the parentheses, like this:
functionName(argument1, argument2, argument3);
Let's look at a simple example:
// Function declaration
function greet(name) {
console.log("Hello, " + name + "!");
}
// Function call
greet("Alice");
greet("Bob");
This `greet` function has one parameter, `name`. It says hello in a personal way. You can call `greet` with any name. This makes the code reusable.
Multiple Parameters and Default Values
For a function with several parameters, just separate them with commas:
// Function with multiple parameters
function addNumbers(a, b) {
return a + b;
}
// Function call
let result = addNumbers(5, 3);
console.log(result); // Output: 8
In this case, `addNumbers` has two parameters, `a` and `b`. It adds them up. When called with 5 and 3, it returns 8.
You can also set default values for parameters. If you don't provide an argument, the function uses this default. Here's how:
// Function with default parameter value
function greet(name = "Friend") {
console.log("Hello, " + name + "!");
}
// Function calls
greet();
greet("Alice");
greet("Bob");
With a default `name` value, `greet` says hello differently. If no name is given, it uses "Friend".
The Benefits of Using JavaScript Function Parameters
Function parameters are great for several reasons:
- They make functions flexible and reusable for different situations.
- By separating inputs from the function body, they help you focus on specific tasks. This makes your code neater and easier to maintain.
- They let your functions work with various data, making your code more powerful.
Knowing how to use function parameters well boosts your coding skills. It lets you customize functions to do exactly what you need.
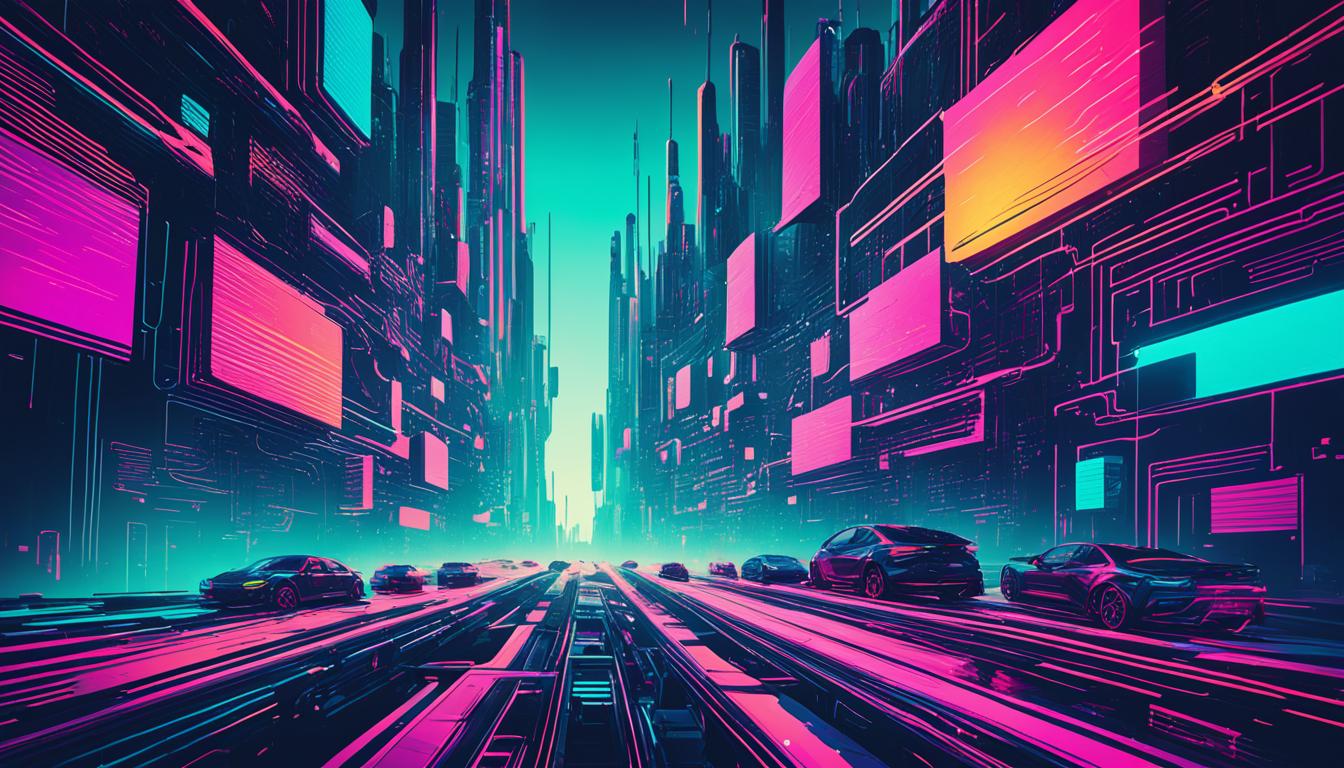
Parameter Type | Description |
---|---|
Numeric | The parameter accepts numerical values such as integers or decimals. |
String | The parameter accepts textual values enclosed in single or double quotes. |
Boolean | The parameter accepts `true` or `false` values. |
Array | The parameter accepts an ordered list of values enclosed in square brackets. |
Object | The parameter accepts a collection of named values enclosed in curly braces. |
Function | The parameter accepts a reference to another function, allowing for dynamic behavior. |
JavaScript Function Examples
Let's dive into examples of JavaScript functions. They show their use from simple math to complex tasks. Each will show how functions help meet goals in programming.
Example 1: Math Operations
Functions often perform math operations. Look at this function that finds a rectangle's area:
function calculateRectangleArea(width, height) {
return width * height;
}
The calculateRectangleArea
function uses width
and height
. It multiplies them. You can call the function and save the result:
let area = calculateRectangleArea(5, 10);
console.log(area); // Output: 50
This makes it easy to get a rectangle's area with width and height.
Example 2: String Manipulation
Functions can change strings too. See this function that makes the first letter uppercase:
function capitalizeFirstLetter(word) {
return word.charAt(0).toUpperCase() + word.slice(1);
}
This capitalizeFirstLetter
function takes a word
. It capitalizes the first letter. Here's how to use it:
let capitalizedWord = capitalizeFirstLetter('example');
console.log(capitalizedWord); // Output: Example
So, you can capitalize any word's first letter in your code.
Example 3: Data Validation
Validating data is another function use. Consider this even-number checker:
function isEven(number) {
return number % 2 === 0;
}
The isEven
function checks if a number divides by 2 evenly. It returns true
for even, false
for odd. Here's its use:
let result = isEven(10);
console.log(result); // Output: true
This way, it's simple to see if numbers are even or odd.
Example 4: Event Handling
Functions also handle JavaScript events. Look at this button-click listener:
let button = document.querySelector('#myButton');
function handleClick() {
console.log('Button clicked!');
}
button.addEventListener('click', handleClick);
handleClick
runs when you click a button named myButton
. It then logs a message. You can change what the function does.
Example 5: DOM Manipulation
Functions can change the DOM. Try this visibility toggler:
function toggleVisibility(elementId) {
let element = document.getElementById(elementId);
if (element.style.display === 'none') {
element.style.display = 'block';
} else {
element.style.display = 'none';
}
}
The toggleVisibility
function finds an element by ID. Then it hides or shows that element. Use this function to control element visibility.
These examples highlight functions' range and power in JavaScript. Customizing them for tasks can make your code more effective.
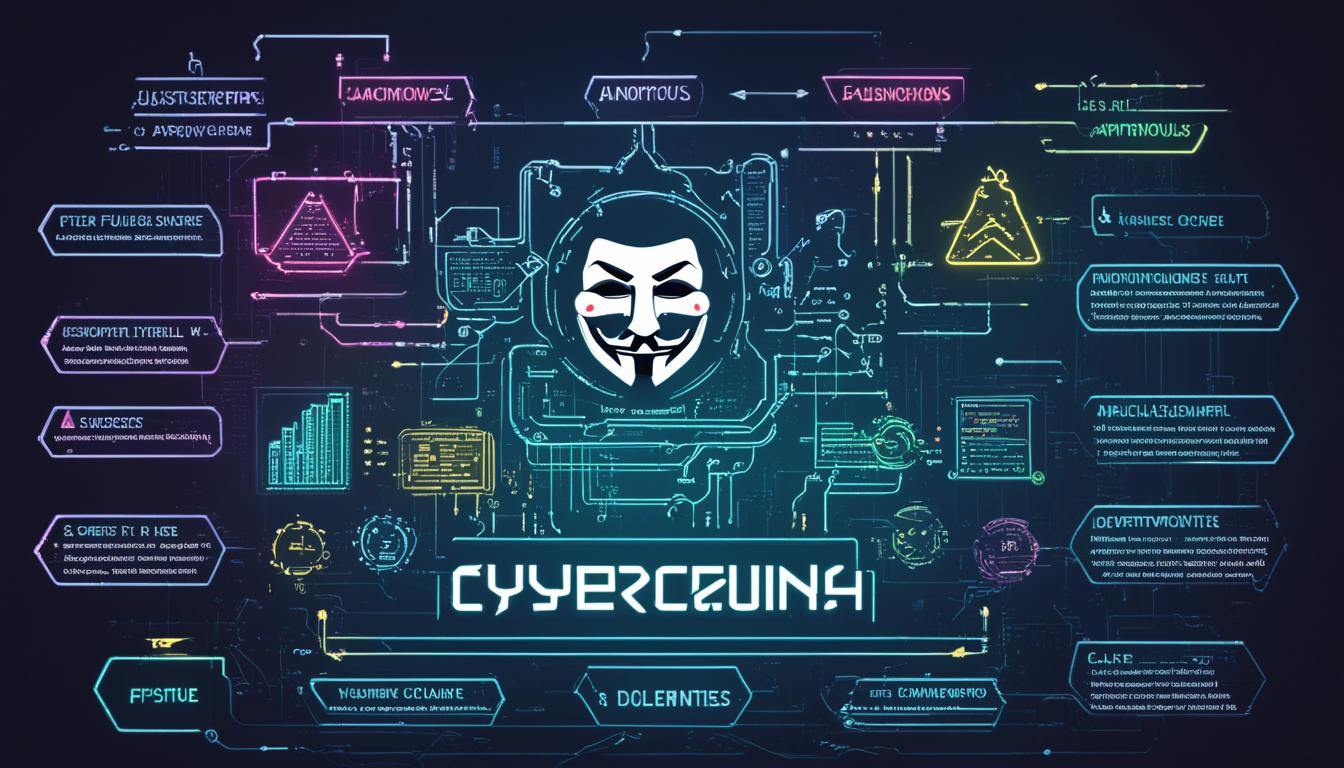
We have covered JavaScript functions from basics to advanced uses. Now, you can use them to improve your websites and make them interactive.
Best Practices for JavaScript Functions
Writing JavaScript functions well means your code runs efficiently and is easy to keep up to date. We will look at important guidelines and tips to make high-quality functions. Following these ideas helps your code run better and enhances your JavaScript apps.
1. Utilize Descriptive Naming Conventions
Using clear names for functions is critical. Names should show the purpose and function of your code. This helps both you and others who might work with your code in the future.
"A function name should reflect its purpose and what it does."
For example:
Function Name | Not Recommended | Recommended |
---|---|---|
Calculate | calc | calculateTotalPrice |
Sort | srt | sortArray |
Validate | chk | validateEmail |
Clear function names make your code readable and easy to follow.
2. Keep Functions Concise
It's best to keep functions short. Long functions can be hard to follow. Try to break complex tasks into smaller, focused functions.
Short functions also make your code more modular and reusable. This way, you can use the same functions in different parts of your code.
3. Prioritize Code Readability
Code that's easy to read is key for good maintenance and debugging. To make code more readable, use:
- Consistent indentation and formatting.
- Comments for complex parts.
- Appropriate capitalization and spacing for names.
4. Avoid Global Variables
Avoiding global variables prevents conflicts and keeps code manageable, especially in big projects or with teamwork. Use local variables in your function’s scope instead.
5. Test and Debug Regularly
Always test and debug your functions before making them live. Testing finds and fixes errors, making your functions reliable.
By adhering to these best practices, your JavaScript functions will be better in efficiency, readability, and upkeep. Implementing these tips not only uplifts your code but also smoothens teamwork and boosts your JavaScript app's performance.
JavaScript Function Return Values
When you work with JavaScript functions, knowing about return values is key. A return statement lets a function send a value back. This value can be used for more actions or decisions. Return values move data from the function to the calling code.
The way to use a return statement is easy. Just write the value you want to return after "return". Look at this JavaScript function for example:
function calculateSum(a, b) {
let sum = a + b;
return sum;
}
This example has a variable "sum" that holds the result of "a" and "b" added together. The return statement then sends "sum"'s value back to where the call was made.
Return values are very useful in programming. They let functions give results for other parts of the code to use. Functions can do tough math, get data from databases, or handle user input. Return values help use these results.
Did you know? A return statement sends back values and ends the function. Code after the return won't run.
Importance of Return Values
Return values are key in controlling how a program runs. They let you:
- Decide actions based on the value returned
- Set the returned value to a variable to use it more
- Give the returned value to another function
Think about a function that finds a number's square:
function calculateSquare(number) {
return number * number;
}
let result = calculateSquare(5);
console.log(result); // Output: 25
In this case, the calculateSquare
function's return value goes to result
. We can then use the squared number for other things.
Return values let you get and use a function's output. This makes your code more adaptable and useful.
JavaScript Function Hoisting
In JavaScript, function hoisting lets you use functions before you declare them. It happens during the compilation phase. This means we can call functions ahead of their declaration in our code.
The compiler looks for function declarations first when reading a JavaScript file. It moves them to the top of their environment. So, you can call a function anywhere within its scope, even before it's written out in your code.
Remember, only function declarations get hoisted, not function expressions. Function expressions are when you assign a function to a variable, and these don't move to the top.
Let's see an example of how function hoisting works:
hoistedFunction(); // The function is called before its declaration
function hoistedFunction() {
console.log('Hoisted function');
}
// Output: Hoisted function
In the example, we call hoistedFunction before declaring it. But thanks to function hoisting, there's no error, and it prints "Hoisted function" on the console.
Understanding function hoisting helps prevent surprises in your JavaScript projects. Knowing about it makes your code cleaner and easier to follow.
Benefits of JavaScript Function Hoisting
Function hoisting brings many advantages to JavaScript coding:
- It makes code more logical and easier to read because you can declare and use functions in any order.
- It gives more flexibility in how you organize your code. You can put function declarations at the end, creating a cleaner look.
- Knowing about function hoisting can save you from bugs and errors, making your coding more effective.
Conclusion
In this article, we've covered the basics of JavaScript functions. Their syntax, usage, and best practices were examined. This helps you create efficient, well-structured functions.
Knowing about JavaScript functions lets you make webpages more dynamic and interactive. It's key for both new and seasoned programmers. It is a must for building strong, scalable apps.
It's important to use function parameters and return values. They make your functions more flexible and reusable. Keep your functions short and use clear names. This will make your code easy to read and maintain.
Also, it's crucial to know about function hoisting. It lets you call functions before they're actually defined. Understanding this can help prevent confusion in your code.
As you keep working with JavaScript, try out different function examples. Improve your programming skills. Using JavaScript functions well can really improve the web experiences you create.
FAQ
What are JavaScript functions?
JavaScript functions are like small machines in your code. They do a particular job when called upon. They help in organizing your code into smaller pieces, making it tidy and manageable.
What is the syntax of a JavaScript function?
A JavaScript function starts with the keyword function, followed by a name. You use parentheses for parameters, and curly braces hold the code. For example: ```javascript function functionName(parameter1, parameter2) { // code block } ```
How do JavaScript function parameters work?
Parameters in JavaScript functions are like placeholders. They hold the values given to the function when you call it. This allows you to work with different data in the function. They are put inside the function's parentheses.
Can you provide some examples of JavaScript functions?
Sure! Let's look at some JavaScript function examples: - ```javascript function addNumbers(num1, num2) { return num1 + num2; } ``` This adds two numbers and gives the result back. - ```javascript function greetPerson(name) { return "Hello, " + name + "!"; } ``` This one creates a greeting message using a name.
- ```javascript function checkEvenOrOdd(number) { if (number % 2 === 0) { return "Even"; } else { return "Odd"; } } ``` It checks if a number is even or odd and tells you.
What are the best practices for writing JavaScript functions?
When writing JavaScript functions, remember: - Use clear names that show what the function does. - Make your functions short and to the point. Avoid long ones as they're tough to follow.
- Keep your code neat and well-formatted for easy reading. - Comments are helpful. Use them to explain the function's purpose. - If you can, reuse functions to reduce repeating code.
How do JavaScript function return values work?
Function return values send information back to where the function was called. Use the return statement to provide this information. This is how you get data from a function to use elsewhere in your code.
What is function hoisting in JavaScript?
Function hoisting lets you use a function before you've written it in your code. This is because JavaScript moves function declarations to the top. But, function expressions don't get this treatment. It's key to know how this works to avoid mistakes.