Welcome to the world of JavaScript, where objects play a crucial role in programming. Whether you are a beginner or an experienced developer, understanding objects in JavaScript is essential for building robust and efficient code.
In this section, we will delve into the basics of objects in JavaScript and explore their significance in programming. We will cover the fundamental concepts of creating objects, working with object properties and methods, and how objects contribute to JavaScript's object-oriented nature.
So, what exactly are objects in JavaScript? In simple terms, an object is a collection of related data and functionalities. It encapsulates properties (variables) and methods (functions) that allow you to create, manipulate, and interact with complex data structures.
Objects in JavaScript are versatile and can represent real-world entities, such as a user, a car, or a product. They provide a powerful way to organize and manage data, enabling developers to write clean, modular, and reusable code.
Now that we've explored the basics, let's dive deeper into creating and manipulating objects in the next section.
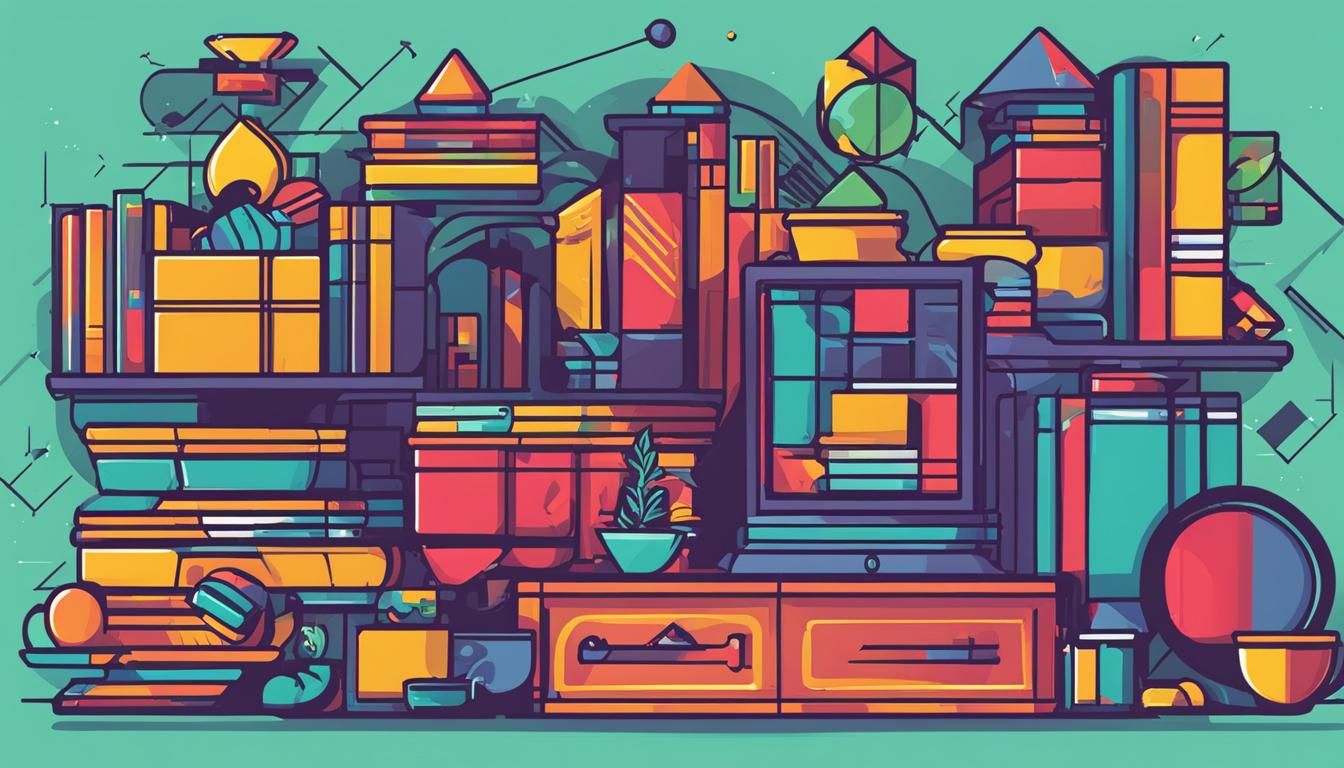
Creating and Manipulating Objects in JavaScript
In the world of JavaScript programming, objects play a crucial role in organizing and manipulating data. This section will explore the process of creating and manipulating objects in JavaScript, providing you with the essential knowledge to harness the power of object-oriented programming.
Creating Objects
There are various techniques to create objects in JavaScript, each serving different purposes. Two common approaches include using object literals and constructor functions.
"Object literals" allow you to define and initialize an object in a single expression. This approach is perfect for creating simple objects.
"Constructor functions", on the other hand, provide a blueprint for creating multiple objects with similar characteristics. By using the new
keyword and invoking the constructor function, you can create new instances of the object.
Let's take a look at an example of creating an object using an object literal:
let person = {
name: "John Doe",
age: 30,
profession: "Web Developer"
};
Accessing and Modifying Object Properties
Manipulating object properties is a fundamental aspect of working with objects in JavaScript. To access and modify object properties, you can use dot notation or bracket notation.
Dot notation is typically used when the property name is known and does not contain special characters or spaces.
// Accessing object properties using dot notation
let name = person.name;
console.log(name); // Output: "John Doe"
// Modifying object properties using dot notation
person.age = 31;
However, if the property name contains special characters or spaces, or if it is dynamically determined, bracket notation should be used.
// Accessing object properties using bracket notation
let profession = person["profession"];
console.log(profession); // Output: "Web Developer"
// Modifying object properties using bracket notation
person["age"] = 32;
Object Manipulation
Besides accessing and modifying object properties, JavaScript provides various methods for manipulating objects. These methods enable you to add or remove properties, merge objects, and clone objects, among other operations.
Here is an example demonstrating how to add a property to an existing object:
person.city = "New York";
And here is an example showcasing how to remove a property from an object:
delete person.age;
In addition to these basic object manipulation techniques, JavaScript offers more advanced methods to modify objects. These methods provide efficient ways to transform, combine, and manipulate objects to suit your specific programming needs.
Visualizing Object Manipulation in JavaScript
Method | Description |
---|---|
Object.assign() | Combines multiple objects into a single object. |
Object.keys() | Returns an array of an object's enumerable properties. |
Object.values() | Returns an array of an object's enumerable property values. |
Object.entries() | Returns an array of an object's enumerable property key-value pairs. |
Object.freeze() | Prevents any modifications to an object. |
By leveraging these powerful built-in methods, you can efficiently manipulate objects in JavaScript and achieve the desired outcomes in your application.
Object Prototypes and Prototype Methods in JavaScript
In JavaScript, object prototypes and prototype methods play a significant role in object-oriented programming. Understanding these concepts allows developers to create hierarchical relationships between objects and leverage reusable code.
Object prototypes serve as blueprints for creating new objects. Each object in JavaScript has an internal [[Prototype]] property, which connects it to its prototype. By accessing this prototype, objects can inherit properties and methods from higher-level prototypes.
One way to create object prototypes is through constructor functions. These functions define the structure and initial properties of an object. When a new object is created using the new
keyword, it inherits the constructor function's prototype.
Additionally, JavaScript provides built-in prototype methods that can be accessed and utilized by objects. These methods are defined in the prototype and inherited by all instances of that object type. Prototype methods offer a convenient way to add functionality to objects and promote code reusability.
The following example illustrates how object prototypes and prototype methods work:
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.greet = function() {
return "Hello, my name is " + this.name + " and I'm " + this.age + " years old.";
}
const john = new Person("John", 30);
console.log(john.greet());
In the code snippet above, we define a Person
constructor function with two properties: name
and age
. We then add a greet
prototype method, which returns a greeting message using the object's name and age. By creating a new object john
using the Person
constructor, we can call the greet
method on the john
object.
Object prototypes and prototype methods provide a powerful mechanism for organizing and extending functionality in JavaScript. By leveraging inheritance and reusable code, developers can build complex applications with ease.
Key Concepts | Description |
---|---|
Object Prototypes | Blueprints for creating new objects |
[[Prototype]] Property | Connects objects to their prototypes |
Constructor Functions | Create object prototypes |
Prototype Methods | Inherited methods for object instances |
Conclusion
In conclusion, understanding objects in JavaScript is crucial for effective object-oriented programming. By gaining knowledge and proficiency in object creation, manipulation, and prototype methods, developers can greatly enhance their JavaScript coding abilities. Objects serve as the building blocks of JavaScript applications, allowing for the organization and encapsulation of data and functionality.
Object-oriented programming in JavaScript provides a powerful way to structure code, making it more manageable, modular, and reusable. With objects, developers can create custom data types and define their own properties and methods, enabling the creation of complex and scalable applications. By leveraging the features of objects, JavaScript programmers can achieve code that is efficient, maintainable, and extensible.
Furthermore, understanding object prototypes and prototype methods allows developers to harness the power of inheritance in JavaScript. By utilizing prototypes and prototype chains, they can create hierarchies of objects that inherit properties and methods from their parent objects. This not only promotes code reusability but also fosters code organization and reduces redundancy.
In summary, mastering object-oriented programming in JavaScript opens up a world of possibilities for developers. By comprehending the essentials of object creation, manipulation, and prototype methods, programmers can elevate their JavaScript skills and build robust, scalable, and maintainable applications.
FAQ
Q: What are objects in Javascript?
A: In Javascript, objects are complex data types that allow you to store and manipulate multiple values as a single entity. An object consists of properties and methods, which represent its characteristics and behaviors.
Q: How are objects created in Javascript?
A: There are different ways to create objects in Javascript. One common way is to use object literals, which allow you to define an object and its properties in a concise manner. Another way is to use constructor functions to create objects, which provides the ability to create multiple instances of an object with shared properties and methods.
Q: What are object properties in Javascript?
A: Object properties are the values associated with an object. They represent the characteristics or attributes of the object. Properties can be of any data type, such as strings, numbers, booleans, arrays, or even other objects.
Q: How can I access and modify object properties in Javascript?
A: You can access object properties using dot notation or bracket notation. Dot notation is used when you know the name of the property beforehand, while bracket notation allows you to access properties dynamically. To modify object properties, simply assign a new value to the property using the assignment operator (=).
Q: What are object methods in Javascript?
A: Object methods are functions that are defined as a property of an object. They allow you to define behavior or actions that the object can perform. Methods are accessed and executed in a similar way to object properties but with the addition of parentheses () at the end.
Q: How can I manipulate objects in Javascript?
A: Objects can be manipulated in Javascript using various methods. Some common methods include adding or deleting properties, looping through the properties of an object, or copying the properties of one object to another. These methods provide flexibility in modifying and transforming objects to suit your needs.
Q: What are object prototypes in Javascript?
A: Object prototypes are the mechanism used for inheritance in Javascript. A prototype is an object from which other objects inherit properties and methods. By creating prototype chains, objects can access and inherit properties and methods from their prototypes, allowing for code reuse and object hierarchy.
Q: What are prototype methods in Javascript?
A: Prototype methods are functions defined on an object's prototype. They are shared among all instances of that object and are accessible through the prototype chain. Prototype methods are a powerful tool for implementing object-oriented programming concepts in Javascript, enabling the reuse of code and promoting efficient memory usage.