When working with JavaScript, especially in asynchronous environments, it is crucial to have a good grasp of Promises. Promises provide a structured and efficient way to handle and manage asynchronous operations. In this article, we will explore the essentials of Promises in JavaScript and how they can simplify your coding workflows.
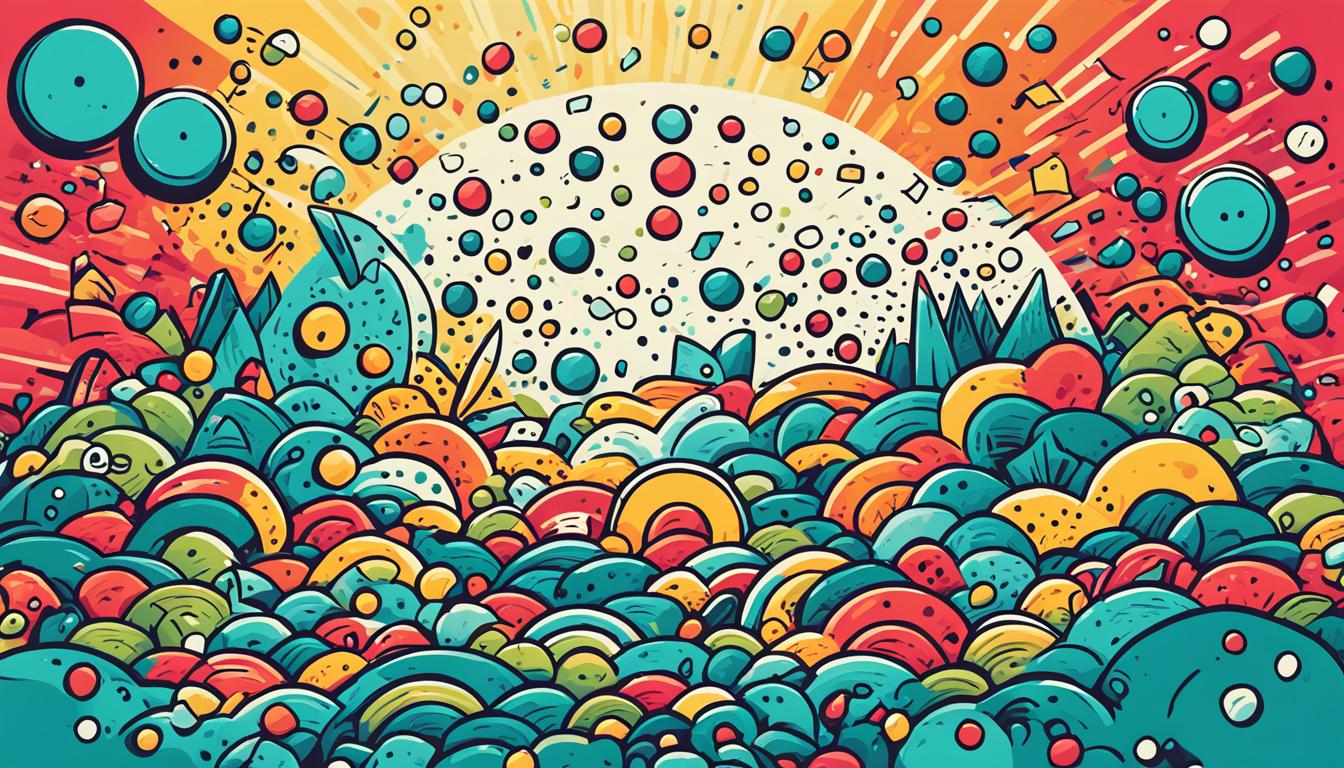
JavaScript Promises, often referred to as JS Promises, are objects that represent the eventual completion (or failure) of an asynchronous operation. They are widely used to handle tasks that take considerable time to execute, such as retrieving data from an API or making network requests.
Asynchronous JavaScript is becoming increasingly important in modern web development. With the rise of single-page applications and the need for seamless user experiences, the ability to efficiently manage asynchronous tasks is critical.
One of the main advantages of using Promises is that they help avoid the "callback hell" problem, where code becomes difficult to read and maintain due to nested callback functions. Promises simplify this workflow by allowing you to chain operations together, making your code more organized and readable.
In the following sections, we will explore how to create and work with Promise objects in JavaScript, including promise chaining and error handling. We will also delve into advanced techniques such as async/await and the Promise.all method.
By the end of this article, you will have a comprehensive understanding of Promises in JavaScript, enabling you to write more efficient and maintainable code when dealing with asynchronous operations.
Let's dive into the world of Promises in JavaScript and discover how they can revolutionize your coding experience.
Working with Promise Objects
In JavaScript, Promise objects play a crucial role in managing asynchronous tasks effectively. They provide a way to handle operations that may take an unpredictable amount of time to complete, such as fetching data from an API or reading a file.
To work with Promise objects, developers need to understand how to create and use them. To create a Promise, the Promise
constructor is used, which takes a callback function as an argument. This callback function, also known as the executor function, is where the asynchronous operation is defined.
Here's an example that demonstrates the creation of a Promise object:
// Create a Promise
const myPromise = new Promise((resolve, reject) => {
// Asynchronous operation
setTimeout(() => {
resolve('Operation completed successfully!');
}, 2000);
});
// Use the Promise
myPromise.then((result) => {
console.log(result);
}).catch((error) => {
console.error(error);
});
Promise chaining in JavaScript allows developers to perform sequential asynchronous operations by chaining multiple Promises together. This is achieved using the then
method, which takes a function as an argument and returns a new Promise. By returning a Promise from the then
method, it enables the chaining of multiple asynchronous operations in a clean and readable manner.
Here's an example that demonstrates Promise chaining:
// Chaining Promises
fetchData()
.then(transformData)
.then(saveData)
.then(() => {
console.log('Data processing completed!');
})
.catch((error) => {
console.error(error);
});
Error handling in Promises is an important aspect that developers need to consider. Promises provide a catch
method that allows error handling within the Promise chain. If any Promise in the chain encounters an error, it will be caught by the nearest catch
method in the chain, preventing the error from bubbling up and crashing the application. Error handling can be done using the throw
statement or by rejecting the Promise with an error object.
Here's an example that demonstrates error handling in Promises:
// Error handling in Promises
fetchData()
.then((data) => {
if (!data) {
throw new Error('Data not found!');
}
return data;
})
.then(transformData)
.then(saveData)
.then(() => {
console.log('Data processing completed!');
})
.catch((error) => {
console.error(error);
});
By understanding the concept of Promise objects and how to work with them in JavaScript, developers can effectively manage asynchronous tasks, handle sequential operations, and gracefully handle errors, leading to more robust and reliable code.
Advanced Asynchronous Techniques
In this section, we will explore advanced asynchronous techniques in JavaScript that can greatly enhance your coding productivity. These techniques include the use of async/await, the Promise.all method, and callback functions within promises.
Async/Await in JavaScript
The async/await syntax is a modern and powerful way to work with Promises in JavaScript. It allows you to write asynchronous code in a more synchronous and readable manner. By using the async keyword before a function and await before a Promise, you can await the resolution of the Promise before moving on to the next line of code.
For example:
async function getUsers() {
try {
const response = await fetch('https://api.example.com/users');
const data = await response.json();
return data;
} catch (error) {
console.error(error);
throw Error('Failed to fetch users');
}
}
In the example above, the getUsers
function uses async/await to fetch user data from an API and handle any errors that may occur. This syntax simplifies the code and makes it more readable.
Promise.all Method in JavaScript
The Promise.all method is another powerful tool in JavaScript for executing multiple Promises concurrently. It takes an array of Promises as input and returns a single Promise that resolves when all the input Promises have resolved. This can be especially useful when you need to make multiple API calls or perform multiple asynchronous operations.
Here's an example:
const promises = [
fetch('https://api.example.com/users/1'),
fetch('https://api.example.com/users/2'),
fetch('https://api.example.com/users/3'),
];
Promise.all(promises)
.then(responses => {
const data = responses.map(response => response.json());
console.log(data);
})
.catch(error => {
console.error(error);
});
In the example above, three API calls are made concurrently using the Promise.all method. Once all the Promises have resolved, the data
array contains the parsed JSON data from each response.
Callback Functions in Promises
Callback functions can also be used within Promises to handle complex asynchronous scenarios. They allow you to perform additional tasks or logic before or after a Promise's resolution. For example, you may need to modify the data returned by a Promise or chain additional Promises based on the result.
Here's an example:
function fetchUser(userId, callback) {
return new Promise((resolve, reject) => {
fetch(`https://api.example.com/users/${userId}`)
.then(response => response.json())
.then(data => {
callback(data);
resolve(data);
})
.catch(error => {
reject(error);
});
});
}
fetchUser(1, userData => {
console.log(userData);
});
The example above demonstrates how a callback function can be used within a Promise to manipulate the fetched user data before resolving the Promise. The callback function is invoked with the retrieved data, allowing you to perform custom logic.
By mastering these advanced asynchronous techniques, you can greatly enhance your JavaScript programming skills and confidently handle complex asynchronous scenarios.
Conclusion
In conclusion, this article has provided a comprehensive overview of Promises in JavaScript. It has covered the basics of Promises and their role in managing asynchronous tasks, as well as advanced techniques for working with Promises. By understanding Promises, developers can write more efficient and maintainable code when dealing with asynchronous operations in JavaScript.
JavaScript Promises offer a powerful solution for handling asynchronous operations, allowing developers to avoid callback hell and write cleaner code. With Promises, developers can easily manage sequential and concurrent async tasks, handle errors gracefully, and simplify the overall coding workflow.
Overall, Promises provide a more intuitive and readable way to work with asynchronous code, enhancing the performance and scalability of JavaScript applications. Whether you are a beginner or an experienced developer, mastering Promises is essential for building modern web applications that deliver enhanced user experiences.
FAQ
Q: What are Promises in Javascript?
A: Promises in Javascript are objects that represent the eventual completion (or failure) of an asynchronous operation. They simplify the handling of asynchronous tasks by providing a more structured way to manage callbacks and handle the results.
Q: How do I create a Promise object in JavaScript?
A: To create a Promise object in Javascript, you use the Promise constructor and pass in a function that takes two parameters: resolve and reject. Inside this function, you can perform the asynchronous operation and then call resolve when it's successful or reject when an error occurs.
Q: How can I chain Promises together?
A: Promise chaining in Javascript allows you to handle sequential asynchronous operations in a more readable and efficient way. You can use the then() method on a Promise to attach a callback that gets executed when the Promise is resolved, and return a new Promise from within that callback to chain additional asynchronous operations.
Q: How can I handle errors in Promises?
A: Error handling in Promises can be done using the catch() method. By attaching a catch() block to a Promise chain, you can handle any rejected Promises and perform error-specific actions. Alternatively, you can use the traditional try/catch syntax within an async function when using async/await.
Q: What is the async/await syntax in JavaScript?
A: Async/await is a modern syntax in Javascript that simplifies asynchronous code execution. It allows you to write asynchronous code in a synchronous manner, making it easier to understand and manage. By marking a function as async and using the await keyword before asynchronous operations, you can ensure that the code waits for each operation to complete before moving on.
Q: What is the Promise.all method in JavaScript?
A: The Promise.all method in Javascript is used to execute multiple Promises concurrently. It takes an array of Promises as input and returns a new Promise that resolves when all input Promises have resolved or rejects if any Promise within the array rejects. This is useful when you have multiple asynchronous operations that can run independently and you need to wait for all of them to complete.
Q: How can I use callback functions within Promises?
A: Callback functions can be used within Promises to handle complex asynchronous scenarios. By passing a callback function as an argument to the then() method, you can perform additional logic or operations based on the resolved value of the Promise. This is especially useful when you need to work with the result of the asynchronous operation before proceeding to the next step.